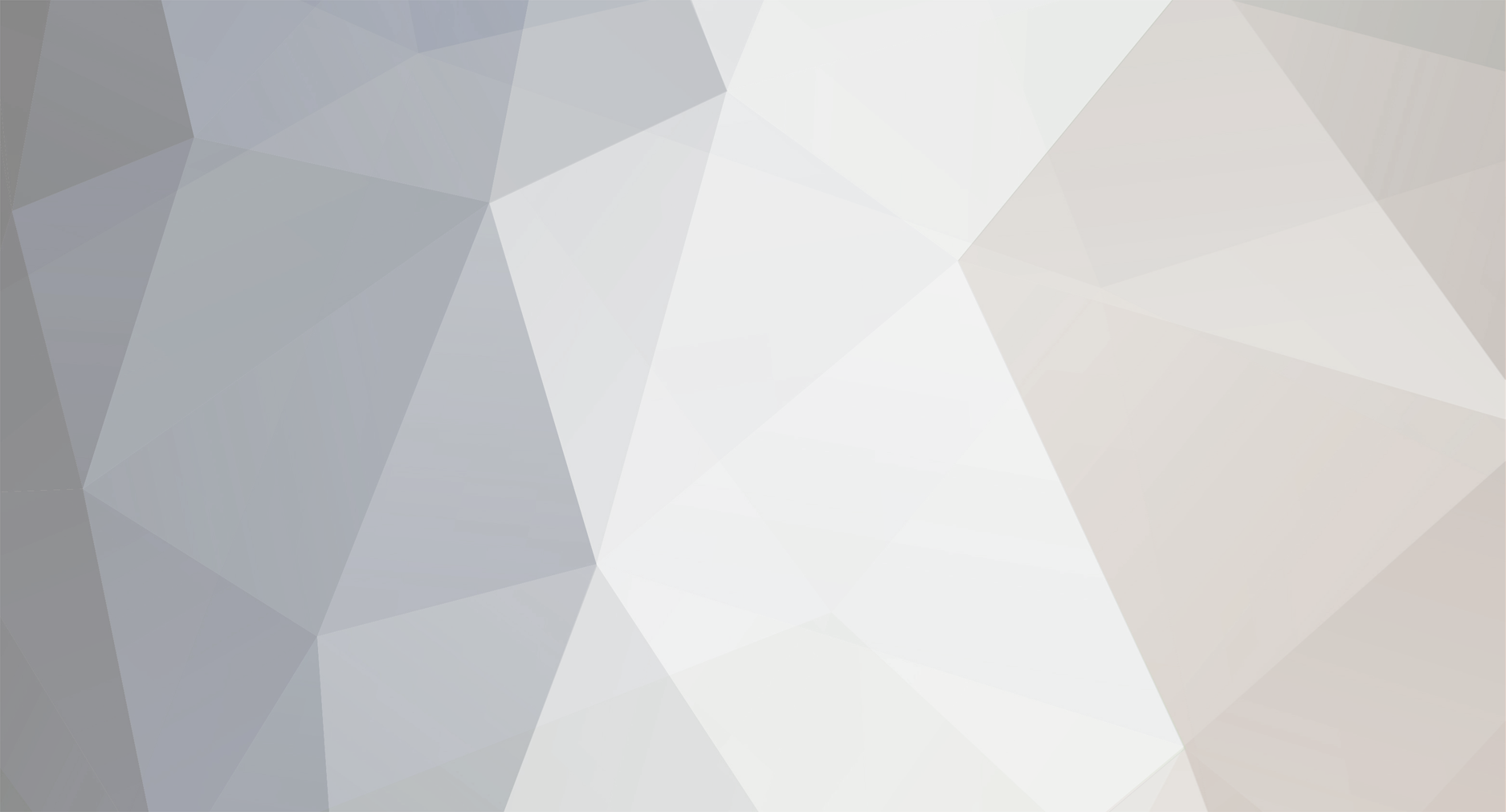
Wintergreen
Members-
Posts
107 -
Joined
-
Last visited
Never
Everything posted by Wintergreen
-
If you're sending a variable to a php page: page.php?variable_name=value And in page.php, you access the variable with $_GET['variable_name']
-
Also, when you're accessing an element's value that was passed from a form, you have to use $_POST['comments'] to access it. Normally you'd make a new variable in the php page for it, so you'd do $comments = $_POST['comments'];
-
Are you getting errors or is it just not displaying anything?
-
Yes, check to see that none of the form elements were empty in the IF statement, and then do your validation and database insert in the ELSE statement. That way if anything is empty, it won't waste time validating data that is incomplete and it won't insert it into the DB. The $_SESSION['message'] thing is just a preference of mine. I just add all the errors onto the end of each other and print them all in one go. It's just easier to remember one variable.
-
Few things to clean up your code a bit. First, you're right, you need an if, else statement. if(empty($_POST['form_element']) || empty($_POST['form_element2']) and so on) { if(empty($_POST['form_element']) $_SESSION['message'] .= "This part was empty<br />"; if(empty($_POST['form_element2']) $_SESSION['message'] .= "This part was empty<br />"; } else { Do your value checking, add slashes etc Do your data insert here } The if statement makes sure there are values in the variables and then uses one variable to print out all the things that needed fixing. The If statement checks ALL the variables from the form to see if they're empty, and if so it goes inside, thereby not touching the DB at all
-
Can you show the code you're using to display it?
-
I think I might as well ask this now. All the str replaces I've seen for filtering out HTML / XML tags have these huge lumps of characters, but I don't know /<(\s*)img[^<>]*>/i works. Can you give a slight explanation?
-
If you've got an array with all the file names stored in it, do a $number_holder = count($array) - 1; If your highest file (f4.pdf) was the last element in the array, access it with $array[$number_holder]
-
So set a $_SESSION variable with the time they posted? That sounds like the easiest way
-
Don't apologize for your English, your question was quite a bit clearer than many of the native speakers I've seen post on here
-
Guess it works as long as all your search strings are set up the same way :)
-
Well, the explode works except for the fact that what you need to break it up by us an escape character, \ . Then, array_search apparently only returns a key value if the search string (@) is the entire entry, not just present in it. So hopefully someone else has something
-
My method, while logical in my head, doesn't work ;)
-
I've got a paging system that works fine, but I'm trying to find as simple a way as possible to display the links. I've looked through some tutorials but they all had all the links printed out, which isn't what I want. I'd like the links to show up like they do in this forum. On a smaller scale, though. So if you're on page one, it shows a few links for pages after then, then ... $last_page If you're on page 25, it shows 1 ... 23 24 25 26 27 ... $last_page. I've been trying for awhile but I just have a bunch of nested if statements and it seems like there really has to be a better way. [code] if($page_number == 1) echo "1"; else echo $first; if($max_page == 3) { if( ($page_number != 1) && ($page_number != $max_page) ) { echo " " . $page_number; } else { echo " " . $nav; } } if($max_page == 4) { if($page_number == 1) { echo " " . $next; } if($page_number == 2) { echo " " . $nav . " " . $next; } if($page_number == 3) { echo " " . $prev . " " . $nav; } } if($max_page > 4) { if($page_number == 1) { echo " " . $next; } if($page_number == 2) { echo " " . $nav . " " . $next; } if($page_number == 3) { echo " " . $prev . " " . $nav; } } if($max_page > 1) { if ($page_number == $max_page) { echo " " . $page_number; } else { echo " " . $last; } } } [/code] Any hints would be wonderful.
-
variable not successfully being passed through address bar
Wintergreen replied to berilac's topic in PHP Coding Help
So at the top of each page, do a quick check to see if the $_GET['page'] is set, and if it's not then just ignore it. If it IS set, then you can do $page = $_GET['page'] and leave the rest of your code as is -
variable not successfully being passed through address bar
Wintergreen replied to berilac's topic in PHP Coding Help
When you pass a variable through the address like that, you access it by using $_GET, so you would check if it's set by if(isset($_GET['page'])) -
Display QUERY results horizontal instead of vertical
Wintergreen replied to adminideas's topic in PHP Coding Help
You could print them all into a table, each entry starting a new <td> -
I'm not sure how long $_POST variables live, but if you're using sessions you could do a check on the form processing page that sees if the user is logged in, and if they're not, put the form data the wrote into some session variables and use them for the value in the original form. You can do a quick check for the form that sees if there is any user data stored in variables, like echo "<input name=blah type = text"; if(isset($_SESSION['title'])) { echo " value=" . $_SESSION['title']; } echo ">"; So if you put something along those lines in the original form, if they try to submit something without logging in you can send them somewhere to have them login and still have the stuff they wrote stored in variables.
-
[code] if(isset($_GET['ID'])) { $query = "SELECT * FROM Videos WHERE VideoID = $_GET['ID']"; $result = mysql_query($query) or die("Query Failed"); $numofrows = mysql_num_rows($result); Then do all your video display code here }[/code]
-
Post requires a form using method=post, so it should be 'safer' than get I guess, but as long as you check the username and password typed by the user for bad input, there should be no problem. I mean the only difference in this case would be that they hadn't typed the username and password into the boxes. One other thing, if your login check is on a separate page, then unless they know the name of the page then no one can really do much
-
The query fails because there's no ID to get, which is what your site relies on to show a video. I tried searching through php.net for the function but don't remember what it's called. There is one that gets the ID of the last entry into the DB. So, since you can't know what the user will search for, at least you can show the latest video or something. So then you'd just do a quick check: [code] if(isset($_GET['ID'])) { $query = "SELECT * FROM Videos WHERE VideoID = '$_GET['ID']'"; } else { $query = and this is where you'd need the function } [/code]
-
if(empty($_POST['element'])) { $_SESSION['message'] = "You need to blah blah"; header("Location: search.php"); }
-
You'd want to use str_replace() http://www.php.net/manual/en/function.str-replace.php
-
how to only the user edit there own profile
Wintergreen replied to rallokkcaz's topic in PHP Coding Help
This is just my way of doing it, but anyway, you'll need to include <? session_start(); ?> at the top of each page, and you'll have to set $_SESSION['user_name'] when they log in since you'll be using this to do your check. So on your site, you can have a page called members.php. And as a link to this, if you want bob to be able to see and edit his profile, but only let other people see it and not edit, you can do something along the lines of having a link like members.php?user=bob. And on the members.php page do [code] <? $user_name = $_SESSION['user_name']; /* This is the logged in user's name */ $user = $_GET['user']; /* This will be the part of the link after the = sign */ ?> [/code] Now you do your read from the DB, ie SELECT FROM users WHERE user_name = $user, then print out the stuff in the format you want. Then after you print it, do a simple check to see if the name of the person logged in is the same as the name of the profile, so [code] if ($user_name == $user) { Print out your form here, containing the info already read from the DB. This let's them edit their userinfo } [/code] And then make a page that will get the $_POST values and write them into the DB and you're done. -
how to only the user edit there own profile
Wintergreen replied to rallokkcaz's topic in PHP Coding Help
What I did with my blog site is do a check for id the user is logged in, as well as for if the user name matches the name of the profile. When the users log in, create a session variable that contains their username, like $_SESSION['user_name'] and then you can use it as a check before you allow them to modify their profile.