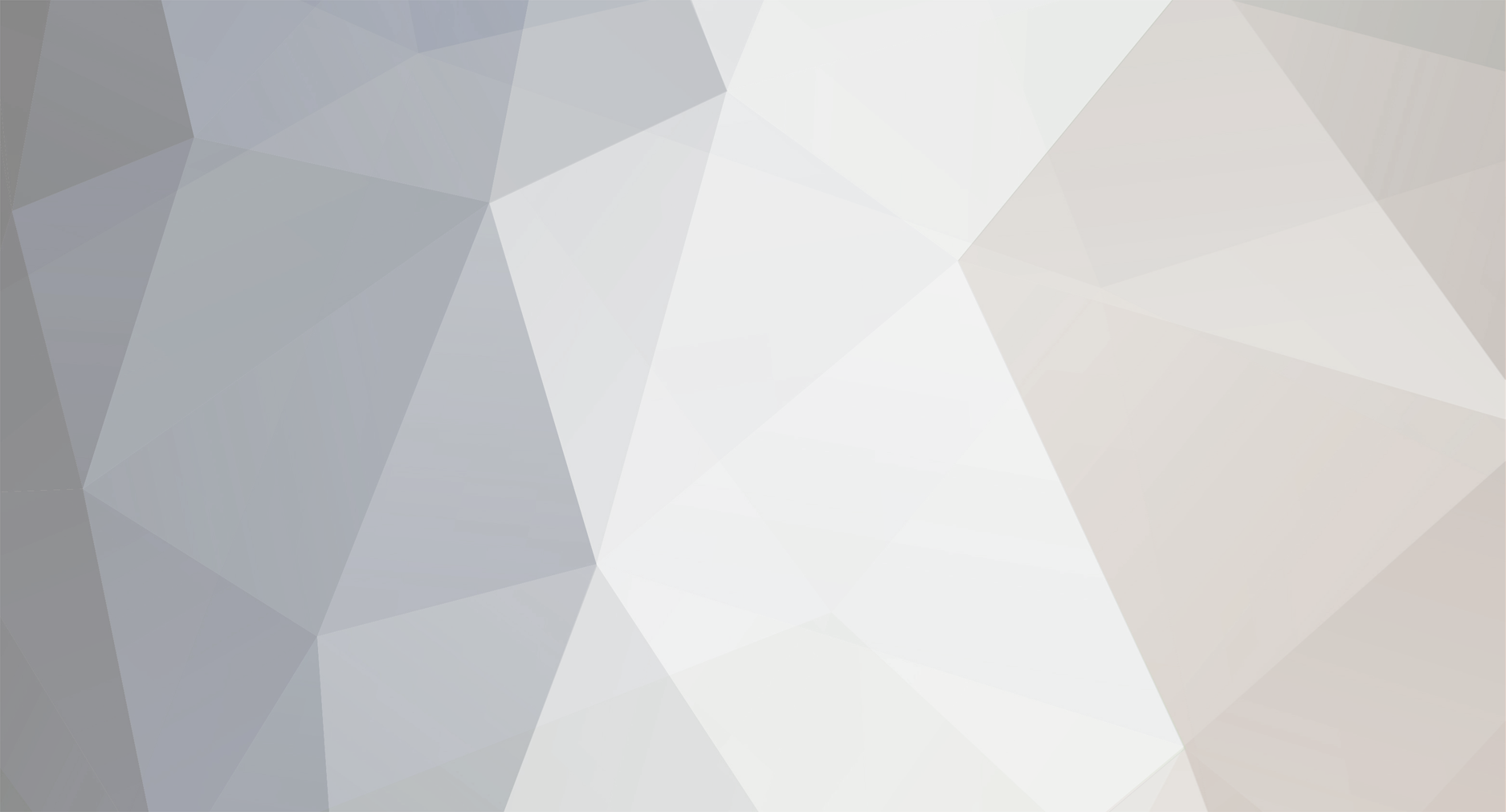
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
First, this is a simple syntax error that can be avoided by reading the manual, or any of the millions of beginner tutorials found through a google search. Link to the manual page you want: http://us2.php.net/manual/en/control-structures.if.php Second, you don't need to assign a variable here. Simply: if ($ulevel = 9) { echo "Admin"; }
-
Is there a more recent version of TinyMCE? The code you linked me to appears to be written in PHP 4, which is pretty gimped in terms of its OO capabilities. PHP 5 is much nicer - abstract classes, data member permission levels (public, protected, private), interfaces, the ability to declare methods as final, etc. I wouldn't be surprised if there isn't a modernized version, given the tenacity of legacy apps, but, still, PHP 5 has been around for 5 years now, so it may be worth a look. Regarding #3, I think that the code is attempting to dynamically create data members. It's kinda sloppy, though, and definitely not the way I'd do it (I have a bit of C++ background, and am currently learning C# myself... I like to declare my members before attempting to use them). That's the only thing I can think of, though, given the lack of declared data members and the empty constructor.
-
The 'this' keyword refers to the current object. In your example code: class MyPhpClass { function myMethod() { $this->variable1 = array(); $this->variable2 = array(); $methodLocalVariable1 = null; $methodLocalVariable2 = 30; } } MyPhpClass has two data members - variable1 and variable2. To clarify, here's a better example: class Person { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getName() { return $this->name; } public function getAge() { return $this->age; } } $Bob = new Person("Bob", 23); $Martha = new Person("Martha", 66); echo $Bob->getAge(); echo $Martha->getAge(); So, using the example above, you should see that Bob and Martha are two instances of the Person class. Despite the class code being the same for each, the values of their properties are different. Why? Because, again, 'this' refers to the current object. When getAge() is invoked on Martha, she is the current object being accessed. Another thing to point out - variables not prefaced with 'this' in an object are temporary. The 'this' implies that you're dealing with a data member/property of an object. Something that is an internal part of the object. $this->name is literally this object's data member entitled 'name.' Furthermore, scope still applies. A temporary variable created in a method will not be globally available to the rest of the class. Yes, the '&' means a reference. In your code example, $this->currentArray holds a reference to $this->array1. With a reference, if you accessed currentArray like so: $this->currentArray[0] = "Hi, folks"; That change would happen in array1 as well. Why? Because currentArray isn't an array, it's an array reference. Anything you do to it actually happens on the array it's referencing. Normal assignment (value assignment) stores a copy of the data rather than a reference. So: $this->currentArray = $this->array1; $this->currentArray[0] = "Hi, folks"; array1 will remain unchanged. Why? Because currentArray contains a copy of array1's data, not a reference to it. Wait, this is preexisting code? Mind sharing the whole thing?
-
Ah, yeah, that sort of thing would definitely draw criticism here. A site should be fully functional without JavaScript. The JS should be used to enhance the existing, underlying site. To use an analogy, a site is like a house. The HTML is the boring, but critical stuff. The foundation, the bathroom(s) and kitchen. The CSS is the floor plan and materials. The fancy oak and the marble tile. The JavaScript is the cool stuff - the HDTV and XBOX 360. It delivers the wow factor, but you can't rely on it for the essentials (to completely beat the analogy into the ground, the HDTV certainly won't keep you warm in winter, nor keep food from spoiling). As long as you view JavaScript as an extra treat rather than a critical component, you'll be fine.
-
JavaScript gets a bad rap for a variety of reasons, the biggest of which are from the early days of the internet when JavaScript was widely misused. Script errors - many of which broke web pages (or worse!) - were common, as was the tendency to bury the user under mounds of popup windows. And, of course, there were security issues tied to it back then, too. That it behaves differently than other web languages makes it a target of many wannabe developers even today as well. As the web matured, so did JavaScript and those that coded in it. Today, it's a cornerstone technology of the web. Many interactive web apps - like the majority of what Google does (GMail, Maps, etc) - are written with JavaScript. That said, you shouldn't use JavaScript for mission-critical tasks like form validation because it can be turned off. JavaScript exists primarily as a way to enhance websites. If used in that manner, it can be very useful. If you want to be a professional, you'll need to learn some JavaScript at some point. It's too widespread to avoid in the modern web. But no, JavaScript is not inherently bad.
-
[SOLVED] Dynamically naming form elements with JavaScript
KevinM1 replied to mayfair's topic in Javascript Help
Simply have a variable in a higher level of scope (in your case, a global) that keeps track of how many times the function has been called. Then, increment it in the function. Ex: var count = 0; function insRow(){ //... b.innerHTML = "<select name='" + (++count) + "'><option value='selected'>Please Select</option><option value='Euros'>Euros</option><option value='US Dollars'>US Dollars</option><option value='Australian Dollars'>Australian Dollars</option><option value='New Zealand Dollars'>New Zealand Dollars</option><option value='Canadian Dollars'>Canadian Dollars</option></select>"; //... } -
Re: API PHP manual: http://www.php.net/manual/en/ PHP function list: http://www.php.net/quickref.php Everything you'd ever want to know about the language is there.
-
You use the correct super global array. If your form's method is post, then you use $_POST. If it's get, you use $_GET. The key names are the names of your form's inputs. So, if you have an input named 'name' (sans quotes), you would access it with $_POST['name']/$_GET['name'] depending on your form's method. You should first check to see if the array even has any values in it, though. Example: Form.html <html> <head> <title>Test form</title> </head> <body> <form action="handleform.php" method="post"> Name: <input type="text" name="name" /><br /> E-mail: <input type="text" name="email" /><br /> <input type="submit" name="submit" value="submit" /> </form> </body> </html> Handleform.php <?php $errMsg = ""; if(isset($_POST['submit'])) //if the form was submitted { if(!empty($_POST['name'])) //if name exists { $name = $_POST['name']; } else { $name = false; $errMsg .= "No name has been entered<br />"; } if(!empty($_POST['email'])) //if email exists { $email = $_POST['email']; } else { $email = false; $errMsg .= "No e-mail address has been entered<br />"; } if($name && $email) { echo "Your name is $name. Your e-mail address is $email"; } else { echo $errMsg; } } ?>
-
Super globals != register_globals. Super globals are your gpc arrays - $_GET, $_POST, $_COOKIE, and the catch-all $_REQUEST. Those aren't going anywhere. register_globals, on the other hand, was a PHP.ini setting that would automatically transform a value in one of the super global arrays into a separate variable of the same key name. So, $_POST['name'] would automatically be available to the coder as $name. It was dangerous because people had a tendency to use that 'feature' as a crutch, and would put these unsanitized variables directly in their database queries. As an aside, addslashes() isn't a very good defense against injection attacks. You should use your database's escape function (such as mysql_real_escape_string()) instead. As for the rest, thorpe's reply is sufficient. The language hasn't changed much in a decade, and certainly not at the variable assignment level. Your errors are logical, not syntactical, in nature.
-
Box doesn't get digitized - only Bridges. :-P
-
Basic plot spoilers from the top of my head: It starts out with something strange going on at a local computer company. The new Master Control Program (MCP) is taking over other systems. The evil CEO (David Warner) is in on it, but it seems as though the MCP is sentient and calling the shots. This strange behavior alerts Bruce Boxleitner's character, and his girlfriend (Cindy Morgan), both of whom work at the company. Once they realize what's going on, they enlist the grudging help of Jeff Bridges (grudging because he and Boxleitner's character had past conflicts, mostly over the girlfriend...yay 80's movie plots), who was a former employee at the company, but was fired for slacking off (writing/playing games) and for getting too close to figuring out the MCP's plans. The three break into the company in the middle of the night. Bridges attempts to stop the MCP, but during the process, the MCP digitizes him, turning Bridges into data that 'lives' inside a virtual, digital world. This digital world is filled with other programs, which are represented as people. The co-stars have analogues here - Warner is in charge of the MCP's evil, and growing, army. Boxleitner is a program named Tron, which was a security program written by the real-life Boxleitner character. And, naturally, the girlfriend is represented by another program as well. The army's culture is taken from both the Nazis and Romans. Innocent programs are captured and sent to prison. Some - those that are useless to the MCP or too disobedient - are killed outright. Others are forced to play various games for a variety of reasons. The outcome for those that lose is always the same - death. Bridges, Boxleitner, and another guy escape prison during the light cycle game. They manage to escape through a crack in the wall. The movie more or less plays out predictably from here - the party gets separated, one guy dies (the extra, 'red shirt' guy), they meet up with the resistance, and ultimately defeat the MCP, which causes Bridges to be de-digitized. Warner is fired as CEO of the company, and I believe the three main characters live happily ever after. The movie is very and unapologetically 1980's. It's not a movie aimed at programmers or computer scientists in general. While the jargon used in the film is no doubt a nod to those that actually know their stuff, it's primarily an action film. A very glowy, neon one. I loved it as a kid because I liked the idea of a complete virtual world living inside my computer. That, and the special effects were cool. It hasn't aged very well, but it boggles my mind that this was done in 1982. I'm sure there were tricks used (there's one brief scene at the end that's obviously done in traditional animation...it makes me wonder if others were, too), but it's still impressive to me. I can't wait for the sequel. I'll be there opening weekend.
-
help solving this simple javascript problem
KevinM1 replied to robert_gsfame's topic in Javascript Help
Hmm...more research has shown that JavaScript's encoding functions aren't compatible with PHP's encoding functions. Thankfully, I've found a 3rd party function that works. Here's the function: function urlencode( str ) { // http://kevin.vanzonneveld.net // + original by: Philip Peterson // + improved by: Kevin van Zonneveld (http://kevin.vanzonneveld.net) // + input by: AJ // + improved by: Kevin van Zonneveld (http://kevin.vanzonneveld.net) // + improved by: Brett Zamir (http://brett-zamir.me) // + bugfixed by: Kevin van Zonneveld (http://kevin.vanzonneveld.net) // + input by: travc // + input by: Brett Zamir (http://brett-zamir.me) // + bugfixed by: Kevin van Zonneveld (http://kevin.vanzonneveld.net) // + improved by: Lars Fischer // + input by: Ratheous // % note 1: info on what encoding functions to use from: http://xkr.us/articles/javascript/encode-compare/ // * example 1: urlencode('Kevin van Zonneveld!'); // * returns 1: 'Kevin+van+Zonneveld%21' // * example 2: urlencode('http://kevin.vanzonneveld.net/'); // * returns 2: 'http%3A%2F%2Fkevin.vanzonneveld.net%2F' // * example 3: urlencode('http://www.google.nl/search?q=php.js&ie=utf-8&oe=utf-8&aq=t&rls=com.ubuntu:en-US:unofficial&client=firefox-a'); // * returns 3: 'http%3A%2F%2Fwww.google.nl%2Fsearch%3Fq%3Dphp.js%26ie%3Dutf-8%26oe%3Dutf-8%26aq%3Dt%26rls%3Dcom.ubuntu%3Aen-US%3Aunofficial%26client%3Dfirefox-a' var hash_map = {}, unicodeStr='', hexEscStr=''; var ret = (str+'').toString(); var replacer = function(search, replace, str) { var tmp_arr = []; tmp_arr = str.split(search); return tmp_arr.join(replace); }; // The hash_map is identical to the one in urldecode. hash_map["'"] = '%27'; hash_map['('] = '%28'; hash_map[')'] = '%29'; hash_map['*'] = '%2A'; hash_map['~'] = '%7E'; hash_map['!'] = '%21'; hash_map['%20'] = '+'; hash_map['\u00DC'] = '%DC'; hash_map['\u00FC'] = '%FC'; hash_map['\u00C4'] = '%D4'; hash_map['\u00E4'] = '%E4'; hash_map['\u00D6'] = '%D6'; hash_map['\u00F6'] = '%F6'; hash_map['\u00DF'] = '%DF'; hash_map['\u20AC'] = '%80'; hash_map['\u0081'] = '%81'; hash_map['\u201A'] = '%82'; hash_map['\u0192'] = '%83'; hash_map['\u201E'] = '%84'; hash_map['\u2026'] = '%85'; hash_map['\u2020'] = '%86'; hash_map['\u2021'] = '%87'; hash_map['\u02C6'] = '%88'; hash_map['\u2030'] = '%89'; hash_map['\u0160'] = '%8A'; hash_map['\u2039'] = '%8B'; hash_map['\u0152'] = '%8C'; hash_map['\u008D'] = '%8D'; hash_map['\u017D'] = '%8E'; hash_map['\u008F'] = '%8F'; hash_map['\u0090'] = '%90'; hash_map['\u2018'] = '%91'; hash_map['\u2019'] = '%92'; hash_map['\u201C'] = '%93'; hash_map['\u201D'] = '%94'; hash_map['\u2022'] = '%95'; hash_map['\u2013'] = '%96'; hash_map['\u2014'] = '%97'; hash_map['\u02DC'] = '%98'; hash_map['\u2122'] = '%99'; hash_map['\u0161'] = '%9A'; hash_map['\u203A'] = '%9B'; hash_map['\u0153'] = '%9C'; hash_map['\u009D'] = '%9D'; hash_map['\u017E'] = '%9E'; hash_map['\u0178'] = '%9F'; // Begin with encodeURIComponent, which most resembles PHP's encoding functions ret = encodeURIComponent(ret); for (unicodeStr in hash_map) { hexEscStr = hash_map[unicodeStr]; ret = replacer(unicodeStr, hexEscStr, ret); // Custom replace. No regexing } // Uppercase for full PHP compatibility return ret.replace(/(\%([a-z0-9]{2}))/g, function(full, m1, m2) { return "%"+m2.toUpperCase(); }); } Once you have the definition in place, run all of your values through it. So: param = urlencode(document.getElementById('Name').value); And so on. Then, in your PHP, use: $name = urldecode($_GET['Name']); $address = nl2br(urldecode($_GET['address']); Etc. -
help solving this simple javascript problem
KevinM1 replied to robert_gsfame's topic in Javascript Help
It looks like your last line should be: window.open("mydata.php?name=" + param + "&address=" + param2); You may need to encode those values so they can be passed through the URL. In mydata.php, you'd obtain those values by: $name = $_GET['name']; $address = $_GET['address']; And, similarly, you may need to decode them here. -
Yeah, I rushed and didn't double-check my array indexes. Also, I had to fix a closure issue (a more advanced JavaScript thing). Now, you don't need to stick everything in window.onload. I like doing things that way for the reasons Dj Kat mentioned. Notice how all the script is in one location rather than strewn about the markup? There's no JavaScript in the markup at all. This makes both the script and the markup easier to edit, debug, etc., and it saves you from sticking function invocations everywhere, which can become a PITA with pages that require the same operations on a multitude of elements. Anyhoo, here's new, functioning code for you: <html> <head> <script type="text/javascript"> window.onload = function(){ var inputCount = document.getElementsByTagName('textarea').length; alert(inputCount); for(var i = 0; i < inputCount; ++i){ (function(){ // <-- fixes the closure issue var oInput = document.getElementById('theInput' + (i + 1)); var oPreview = document.getElementById('previewBox' + (i + 1)); oInput.onkeyup = function(){ oPreview.innerHTML = this.value; } })(); // <-- ditto } } </script> </head> <body> <div id="previewBox1"> Your preview will be displayed here. </div> <div id="contentBox1"> <h2>The Content 1</h2> <textarea id="theInput1"></textarea> </div> <br /> <br /> <div id="previewBox2"> Your preview will be displayed here. </div> <div id="contentBox2"> <h2>The Content 2</h2> <textarea id="theInput2"></textarea> </div> </body> </html>
-
Unfortunately, you're way off. 'This' literally means the current object, which generally means the element you're currently accessing. Passing 'this' to the function doesn't make sense, as the function already has access to 'this' since it's attached to that element's event handler. Also, you can't pass 'this' into getElementById. It expects a string containing the id of the element you're trying to obtain. What you want instead is something like: <html> <head> <script type="text/javascript"> window.onload = function(){ var inputCount = document.getElementsByTagName('textarea').length; for(var i = 0; i < inputCount; ++i){ var oInput = document.getElementById('theInput' + i); var oPreview = document.getElementById('previewBox' + i); oInput.onkeyup = function(){ oPreview.innerHTML = this.value; } } } </script> </head> <body> <div id="previewBox1"> Your preview will be displayed here. </div> <div id="contentBox1"> <h2>The Content 1</h2> <textarea id="theInput1"></textarea> </div> <br /> <br /> <div id="previewBox2"> Your preview will be displayed here. </div> <div id="contentBox2"> <h2>The Content 2</h2> <textarea id="theInput2"></textarea> </div> </body> </html>
-
The ternary operator may not be the best thing to show a beginner who doesn't know about conditional statements....
-
Putting the code in a function, with the condition of whatever it is you need to keep track of passed in as an argument, seems like the most logical solution to me.
-
Inserting a script using innerHTML property
KevinM1 replied to dpacmittal's topic in Javascript Help
Okay...hmm...do you even need to do it dynamically? Is there anything in the Wordpress plugin that's forcing you to attempt injecting the adsense code? -
Inserting a script using innerHTML property
KevinM1 replied to dpacmittal's topic in Javascript Help
Is there any other content? I can't see where a clickable ad is being created. The first script defines some properties, and the second links to a Google script that should allow ads to be displayed. How are adsense ads normally displayed? Wouldn't there need to be a target element of some sort that would actually display the ads? Is there a tutorial somewhere? Because I've never used adsense myself. -
Inserting a script using innerHTML property
KevinM1 replied to dpacmittal's topic in Javascript Help
Can you give me an example of the content? Because I can't see why you couldn't just inject it with innerHTML. -
Inserting a script using innerHTML property
KevinM1 replied to dpacmittal's topic in Javascript Help
Only the adsense content needs to be in the div. Okay...why can't you do something like: <html> <head> <script type="text/javascript"> window.onload = function(){ document.getElementById('test').innerHTML = '<a href="#" id="blah">Clicky<\/a>'; document.getElementById('blah').onclick = function(){ alert("Clicked"); } } </script> </head> <body> <div id="test"></div> </body> </html> It's a simple example, but it shows how you can inject markup into a preexisting element and tie an event handler to it. -
Inserting a script using innerHTML property
KevinM1 replied to dpacmittal's topic in Javascript Help
Does the adsense code need to be in the div, or only the adsense content? -
[SOLVED] Trying to get property of non-object error
KevinM1 replied to spedax's topic in PHP Coding Help
The method returns an array. Have you tried $user[0]->username ?? Also, there are several questionable things going on in general, including: 1. Mixing PHP 4 and PHP 5 OO syntax 2. User allowing direct public access to its properties 3. User being a child of DatabaseObject 4. Using array_key_exists() on an object, which is deprecated behavior as of 5.3. Using property_exists(), or, even better, the Reflection API is the way to go here. -
That's actually not correct. Scripts can go anywhere, and often it's beneficial to put them at the end of the document, or at least after the element they apply to. A very visible example of this is google analytics, which is placed as the last element in the document. I'm surprised they don't just use an in-house version of $(document).ready() to do that. Still, in most cases, from what I've seen/read, convention seems to be that scripts should go in the head, to be executed after window.onload, or the previously mentioned ready() function.
-
+1 for CCleaner