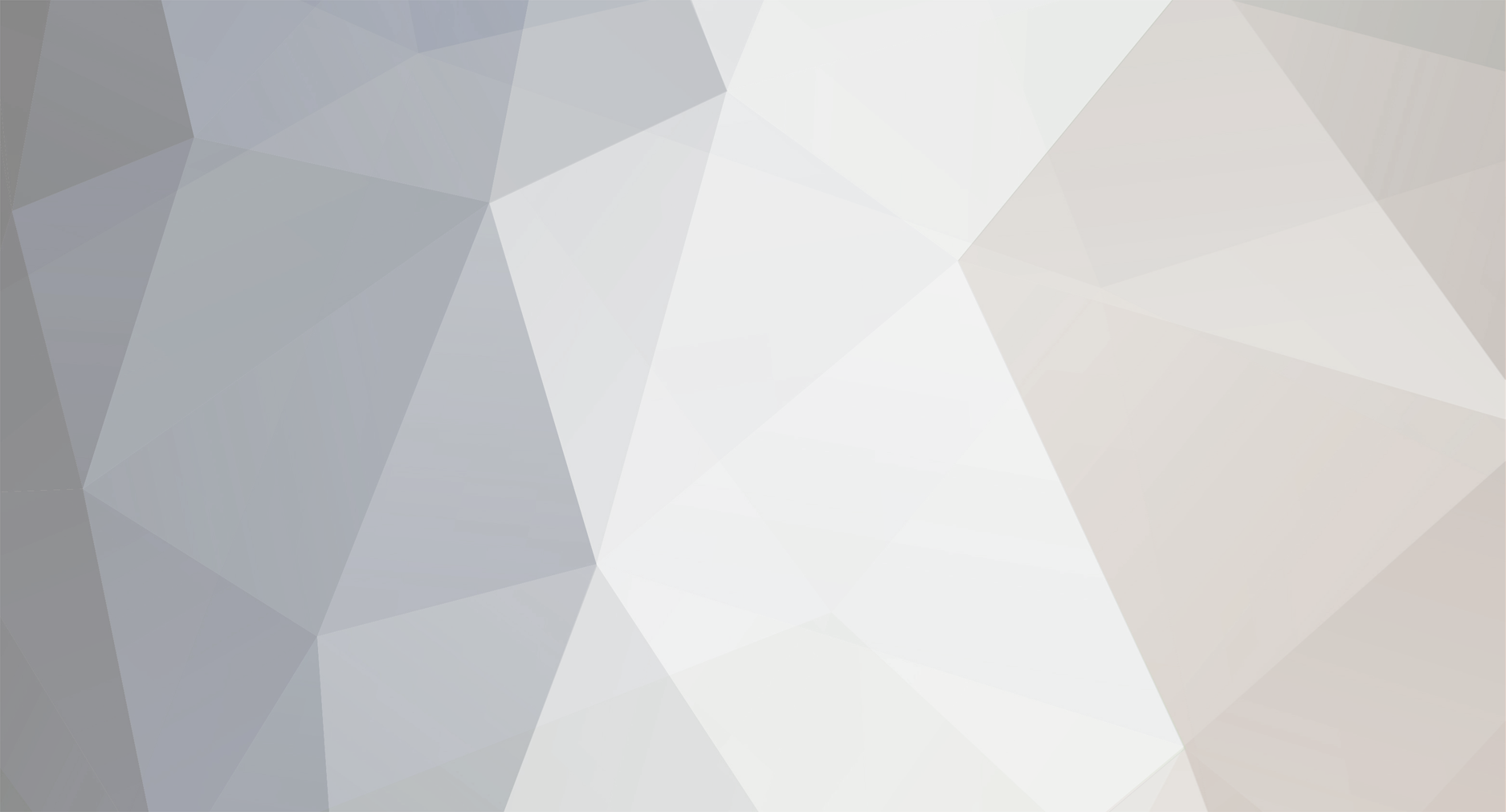
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Oh, I know. I just wanted to give the OP a nudge in the right direction in terms of general class structure.
-
If you still want to roll your own, I suggest separating the responsibilities of your current class. Right now, your class is attempting to do too much. From what I can see, it's attempting to hold all validators (which are other objects of the same type) and perform validation. Also, you're dynamically adding new properties to it with the $object->field = $fieldname stuff, which is a very bad idea. So, here's what you should do to get started: 1. Create a new class whose sole job is to hold an array of validator objects and iterate over them. Something like: class ValidatorArray { private $validators = array(); public function add(Validator $validator) { $this->validators[] = $validator; } public function validate() { foreach($this->validators as $validator) { $validator->validate() } } public function getResults() { foreach($this->validators as $validator) { if(!$validator->didPass()) { echo $validator->getError() . "<br />"; } } } } It may not be exactly what you need, but you should get the idea. 2. Create a Validator class that has the private properties you need to get the job done. No creating new public properties on the fly. class Validator { private $fieldName; private $numChars; private $charCheck; private $passed = false; private $errMsg; public function __construct($fieldName, $numChars, $charCheck, $errMsg) { $this->fieldName = $fieldName; $this->numChars = $numChars; $this->charCheck = $charCheck; $this->$errMsg = $errMsg; } public function validate() { //perform validation logic if(/* data validated */) { $this->passed = true; } else { $this->passed = false; } } public function didPass() { return $this->passed; } public function getError() { return $this->errMsg; } } Again, it may not be exactly what you need, but you should get the idea.
-
Simply output the current post data in the right place: <input name="name" type="text" value="<?php if(isset($_POST['name'])) echo $_POST['name']; ?>" />
-
Simply check to see if there's a value in the form fields. If not, make their values an empty string. Or, if you need to do some math right away, make the values 0.
-
Don't place what I wrote after the body tag. Put it in the head, where most scripts are supposed to go. Really, if you're going to attempt using JavaScript, you should at least know where to place your scripts.
-
I'm not certain of any performance improvements. The MySQLi page on the PHP site doesn't mention any. There are other benefits, though. You already mentioned its OO functionality, and prepared statements are very nice.
-
Why not try something like: <script type="text/javascript"> window.onload = function(){ var documentHead = document.getElementsByTagName('head')[0]; var protoScript = document.createElement('script'); var scriptaculousScript = document.createElement('script'); var lightboxScript = document.createElement('script'); protoScript.type = 'text/javascript'; protoScript.src = 'js/prototype.js'; scriptaculousScript.type = 'text/javascript'; scriptaculousScript.src = 'js/scriptaculous.js?load=effects,builder'; lightboxScript.type = 'text/javascript'; lightboxScript.src = 'js/lightbox.js'; documentHead.appendChild(protoScript); documentHead.appendChild(scriptaculousScript); documentHead.appendChild(lightboxScript); } </script>
-
What is it exactly that you're trying to do?
-
My .NET isn't very good, since I'm still learning it, but to emulate typical PHP firehose behavior, you simply need a command object and a data reader. Here's some of my code. It's C#, but it should give you an idea of what to do: string query = "Select level, name, tpCost, dmgModifier, hitPenalty from DBHackerAttacks"; using (SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["GitSConnectionString1"].ConnectionString)) { SqlCommand cmd; SqlDataReader reader; cmd = new SqlCommand(query, conn); conn.Open(); reader = cmd.ExecuteReader(CommandBehavior.CloseConnection); while (reader.Read()) { HackerAttack hackerAttack = new HackerAttack(reader.GetInt32(0), reader.GetString(1), reader.GetInt32(2), reader.GetDouble(3), reader.GetDouble(4)); this.attacks.Add(hackerAttack.Level, hackerAttack); } } This is .NET 3.5, so some of the things may need to be tweaked. It uses ADO.NET, so you'll need to ensure the proper namespaces are included. With your example, you could either dynamically build a table/other structure, and fill in its cells in the while-loop, or you could pre-make the table/other structure in the plain .aspx file, and, once again, fill its cells with data in the loop.
-
Unfortunately, I cannot. I'm not a Flash developer. I know of Flash, and could probably cobble something simple together at gunpoint, but it's not in my toolbox. The comments/reviews that most major bookstore websites have tend to be pretty informative. You might want to check out the Visual Quickstart books. They tend to be "My first <language>" books, but are often well written and have the info needed to at least be a qualified beginner. They're not insulting like the various "For Dummies" books, and their examples work.
-
Just remember two things: 1. You get what you pay for, so there's no guarantee that free info will teach you the right way to do things 2. Online tutorials tend to only care about getting a specific task completed as opposed to teaching others about the big picture IMO, you should get a good book on the subject (one that's specific to your version of Flash... search around on Amazon or another bookstore site and read the comments/reviews), and use online tutorials, guides, and forums as supplementary material. It may cost you a bit extra, but it'll save you time and headaches in the end.
-
Actionscript is a part of Flash. It's Flash's scripting language. If you have Flash, you wouldn't need to buy any extra software to access Actionscript.
-
show [layer] by clicking on an option from the select button
KevinM1 replied to yami007's topic in Javascript Help
Put quotes around the inputs' attributes in the HTML and the word 'block' in the JavaScript and try it again. -
That's because the time() function returns the current time. Unless time itself stops, the number will always change. And, in that circumstance, you have bigger problems than your script failing.
-
I wouldn't learn Visual C++ for the simple reason that, like captbeagle said, it has some proprietary kinks that make it more difficult to learn. Memory management can be difficult enough without throwing a specialized form of heap management on top of it. Stick with the standard until you know what you're doing.
-
If you know in advance what the value of this third variable will be, you can create a hidden form input. That, or pass the value along in a session.
-
Ooh, nice.
-
EDIT: haku, would something simple like: window.opener.el.value = 'something'; work? I'm just wondering if a child window has knowledge of its parent's variables.
-
Hmm...yeah, you're in a pretty tight corner. Unfortunately, there's no easy, 'clean' solution. The easiest thing I can think of would be to pass in the target element, which you already do, then, for the Mozilla side of things, attach that element's id to the pop-up's url as a query string. So, something like: /* IF MOZILLA */ else { window.open('link-popup.php?sender=' + el.id, 'name', 'height=280,width=550,toolbar=no,directories=no,status=no,menubar=no,scrollbars=no,resizable=no,modal=yes'); } In the pop-up, you can grab a hold of the query string value by writing a function like (note: comes from a 3rd party, so I haven't tested it...): function getQuerystring(key, default_) { if (default_==null) default_=""; key = key.replace(/[\[]/,"\\\[").replace(/[\]]/,"\\\]"); var regex = new RegExp("[\\?&]"+key+"=([^&#]*)"); var qs = regex.exec(window.location.href); if(qs == null) return default_; else return qs[1]; } So, with that in the pop-up, you could modify your updateEditorAMozilla() function like so: /* FUNCTION CALLED FROM POPUP WINDOW TO SET AN A HREF TAG IN EDITOR WHEN USING MOZILLA */ function updateEditorAMozilla(fldVal) { var tagBeginning = fldVal; var tagEnding = "</a>"; var target = getQuerystring('sender'); var el = window.opener.document.getElementById(target); if (el.setSelectionRange) { el.value = el.value.substring(0,el.selectionStart) + tagBeginning + el.value.substring(el.selectionStart,el.selectionEnd) + tagEnding + el.value.substring(el.selectionEnd,el.value.length) } el.focus(); } You'd have to move the Mozilla function into the pop-up, though there's probably a way to keep it in the main page with a little work.
-
Hmm... can you show me all the code of your pop-up window?
-
So, wait...why can't you simply pass along the element to the function you need? You already have a handle on the proper element - it's the argument you pass into the function. What's stopping you from passing that into the pop-up window along with the text you're modifying?
-
How are you calling this function? I have an idea of what you're trying to do, but would like more info just to be sure.
-
Yup, an l-value is a left hand value. It generally means that it's something that can be assigned to, but not always (const variables are considered l-values). l-values can be used as r-values, but not vice versa. More info: http://publib.boulder.ibm.com/infocenter/macxhelp/v6v81/index.jsp?topic=/com.ibm.vacpp6m.doc/language/ref/clrc05lvalue.htm
-
It's sort of a pointer. It's a handle to an object (this time, a string) on the managed (read: .NET) heap. Try using the same safe_cast thing I showed you before to turn it into an int^.