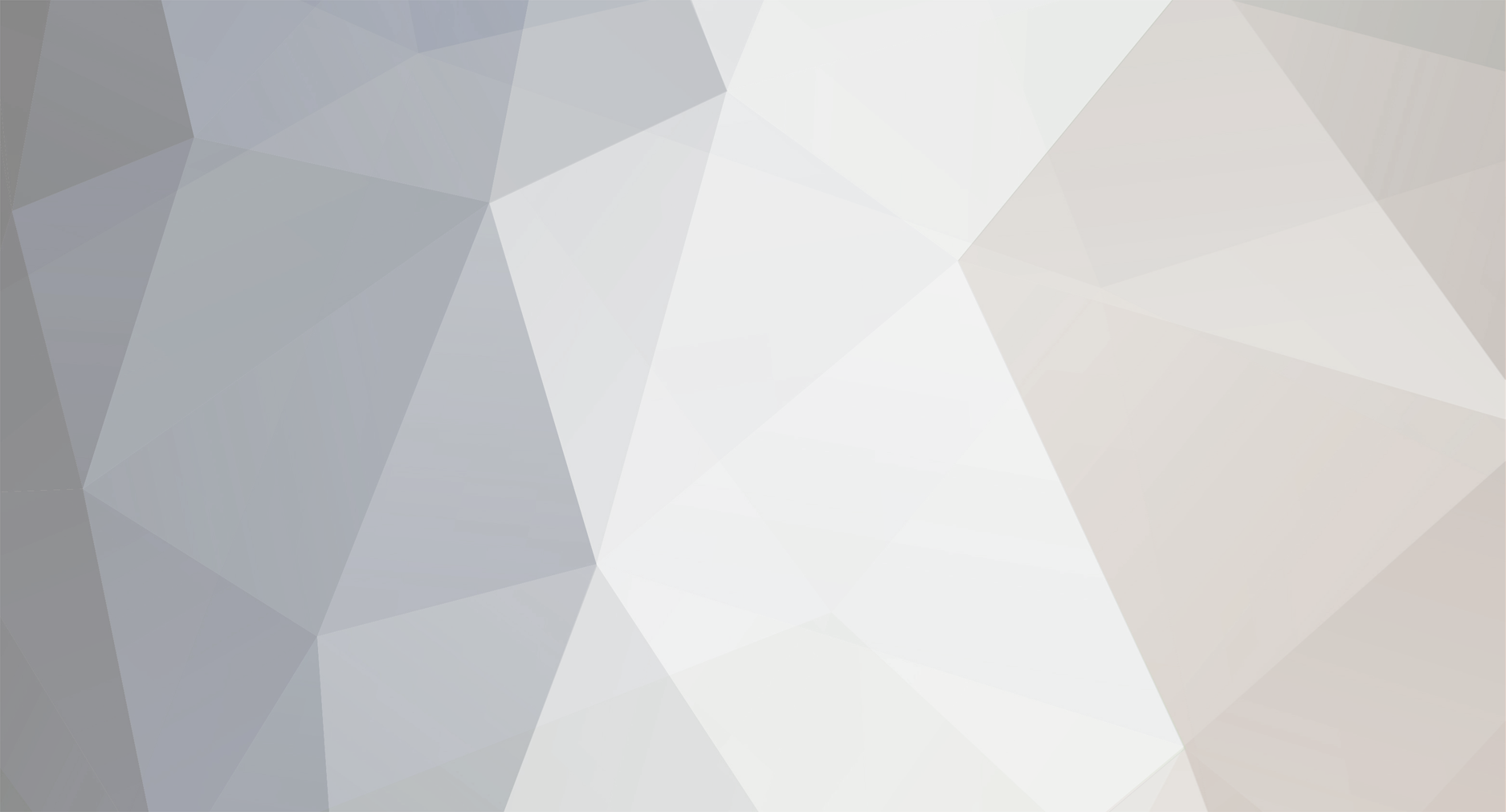
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Okay, first, let's build the form. Before we begin, though, a caveat: Programs like the one you used to construct your site tend to write bad code. It's just the nature of how they work. So, what I'm going to show you isn't necessarily the best way to do things overall, but it should be the easiest to implement as it won't really change the meat of the page's layout. Like anything in computing, there's a trade-off. In this case, it's a trade-off in efficiency for you in the short term vs. shortening your site's code and making things more modular in the long term. Go to line 117, and replace the line there with the following: <td rowspan="2" bgcolor="#000000"><form id="myForm" action="processContact.php" method="post"><table width="740" height="169" border="0"> This will begin the form. The good news is that you already have all of your inputs created. You just need to rename them so they're easier to deal with when you finally process their info. On line 128, replace that line with: <td><input name="name" type="text" size="25" maxlength="30" /></td> Replace 142 with: <td><input name="email" type="text" size="25" maxlength="50" /></td> Replace 156 with: <td><input name="dayPhone" type="text" size="25" maxlength="12" /></td> Replace 170 with: <td><input name="nightPhone" type="text" size="25" maxlength="12" /></td> Replace lines 184 - 190 with: <td><select name="howToReach"> <option value="email">E-mail</option> <option value="dayPhone">Telephone Day</option> <option value="nightPhone">Telephone Night</option> </select></td> And yes, I do want you to remove the form element from that block of code. It's unnecessary now that we have all of this within a form element. Replace 204 with: <td><input name="eventType" type="text" size="25" maxlength="30" /></td> Replace 218 with: <td><input name="eventDate" type="text" size="25" maxlength="20" /></td> Replace 232 with: <td><input name="budget" type="text" size="25" maxlength="10" /></td> Replace 246 with: <td><input name="howDidYouFind" type="text" size="25" maxlength="50" /></td> Replace 260 with: <td><textarea name="comments" cols="25" rows="3"></textarea></td> Replace 274 and 275 with: <td> <input type="submit" name="submit" value="Submit" /> <input type="reset" name="reset" value="Clear" /></td> Finally, replace 286 with: </table></form></td> That should build a capable form without forcing you to rewrite your entire page. Now, before we get to processing all this info, what, exactly, do you want to do with it? I can't help you unless I know how you want to process it.
-
Is this for the form element itself, or everything including the inputs? I ask because I think the superglobals only use the input names as the keys. I'd be more than happy if they recognized ids, too. I agree regarding the form element itself, though. I've been playing with ASP.NET too much lately...you do NOT want to know how it handles the ids of anything told to run on the server. Makes CSS a pain in the ass.
-
I think you're over-thinking this. Let's start at the beginning and work from there. When dealing with the database, file location doesn't really matter so long as the database and file you want to have access it are on the same server. In fact, localhost means 'local to this host.' Your contact page could be buried in nested directories, like say public_html/new/autumn/contact.php. You would still access the database by specifying localhost as the host. With your contact page in particular, you need to use a form element in order to handle the info the user types into the fields. This is a standard HTML element, and is something you'll need to get comfortable with whenever you need to get user-inputted info. A form element looks like this: <form name="form1" action="process.php" method="post"> </form> The form's attributes are VERY important: Name - the name of your form. Useful when using JavaScript Action - the name of the file that processes the data entered into the form. This is the name of the PHP file you want to use to deal with the form data Method - how that data is actually transfered. There are two options: get and post. Get passes the data as name/value pairs within the URL itself (also known as a query string). Ever been to a site that has an address like www.somesite.com/index.php?action=comment&user=1432 ? Everything after the first '?' is a query string. Post passes the data behind the scenes, so it's not visible in the URL. Within the form element should be your inputs. These are the form fields and/or buttons that the user can interact with. So, with these additions, you'd have something like: <form name="form1" action="process.php" method="post"> Name: <input name="name" type="text" /><br /> E-mail: <input name="email" type="text" /><br /> <input name="submit" type="submit" value="Submit" /> </form> Any inputs with the type "submit" tell the browser to submit whatever info is in the other inputs (if any) to the file specified with the action attribute (in our case, process.php) when that button is clicked. It quite literally tells the browser "we're now submitting all the data associated with this form." The form element is the glue that holds it all together. It groups all of the inputs inside of it as the data needing to be sent, then sends only that data to the server when the submit button is pressed. It's then up to you to actually write process.php to handle that incoming data. Let me know if you need help handling that data now that you know how to send it to a PHP script.
-
PHP files should go into your web root folder, which is typically named something along the lines of public_html. As a general rule of thumb, if you're supposed to put HTML files somewhere, then you can put PHP files there as well. Form data is delivered to the script named with the action attribute. Example: <form name="form1" action="processForm.php" method="post"> <!-- bunch of inputs and stuff --> </form> The data entered into that form would be sent to the file processForm.php. If you want to save form data, you'll need to either save it to a database or write it to a file. Using my example above, you'd have to write that code within processForm.php. One of the cool things about PHP is how easy it is to create sticky forms. These are forms that 'remember' what the user previously entered if the user entered invalid/bad/wrong data. The general form of it is: if (data has been submitted) { process it, then redirect the user } else { show the form with any previously entered values in the form's fields } A simple (i.e., not for production use) example would be: <?php require_once("dbconnect.php"); if(isset($_POST['submit'])) //has data been submitted? { if(isset($_POST['name'])) //has a name been submitted? { $name = $_POST['name']; } else { $name = false; } if(isset($_POST['email'])) //has an e-mail address been submitted? { $email = $_POST['email']; } else { $email = false; } if($name && $email) //if everything's been submitted correctly { $query = "INSERT INTO myUsers (name, email) VALUES ('$name', '$email')"; $result = mysql_query($query); header("Location: index.php"); //redirect the user back home } } //if things aren't right, display the form ?> <form name="myForm" action="<?php echo $_SERVER['PHP_SELF']; /* use THIS script to process the form data */ ?>" method="post"> Name: <input name="name" type="text" value="<?php if(isset($name)){ echo $name; } ?>" /><br /> E-mail: <input name="email" type="text" value="<?php if(isset($email)){ echo $email; } ?>" /><br /> <input name="submit" type="submit" value="Submit" /> </form>
-
You need to use session_start(). http://www.php.net/manual/en/function.session-start.php Be sure to heed the first note from that linked page. You must call session_start before ANY output is sent to the browser. This includes any accidental whitespace. So, for best results, session_start should be the first line of your script.
-
I think this would be pretty easy to do. In essence, you'll need to check the readyState of the XmlHttp object. Since a readyState of 1 means the server is loading the response, it makes sense to test against that. You can add it to your existing stateChanged function. function stateChanged() { if(xmlHttp.readyState == 1 || xmlHttp.readyState == "loading") { //display loading image } if (xmlHttp.readyState==4 || xmlHttp.readyState=="complete") { document.getElementById("txtHint").innerHTML=xmlHttp.responseText } }
-
Okay, first, you created an entirely new html document within the middle of your page. You can't do this. HTML doesn't work that way. Second, you need to follow along with what I did. Remember: I removed the showPhoto() function call from the link. Sticking it into the id of a link is illogical, and not at all what I did above. On to actual code... At the top of the page, put this: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <script type="text/javascript" src="http://travelling.dw20.co.uk/AJAX/largephoto.js"></script> <script type="text/javascript"> window.onload = function() { var gallery = document.getElementById('gallery'); var images = gallery.getElementsByTagName('img'); for(var i = 0; i < images.length; i++) { images[i].onclick = function() { showPhoto(this.id); } } } </script> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> <link href="site.css" rel="stylesheet" type="text/css" /> <style type="text/css"> <!-- body { margin-left: 0px; margin-top: 0px; margin-right: 0px; margin-bottom: 0px; background-color: #333333; } --> </style></head> REMOVE the following from the middle of your page: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <script src="/AJAX/largephoto.js"></script> <script type="text/javascript"> var img=null window.onload = function(img) { var testAjax = document.getElementById('testAjax'); testAjax.onclick = function(img) { showPhoto('img'); } } </script> </head> <body> Now, give your main content table an id of gallery: <!--start of main content table --> <table id="gallery" width="100%" border="0" cellpadding="0" cellspacing="0"> Now, here's one of the most important parts. Redo the line of code that houses the thumbnails so it looks like: <img id="thai1001" src='http://travelling.dw20.co.uk/images/photos/thai1/thailand.jpg' height='65px'><img id="losa1002" src='http://travelling.dw20.co.uk/images/photos/losa1/la1.jpg' height='65px'><img id="lossa1001" src='http://travelling.dw20.co.uk/images/photos/losa1/la.jpg' height='65px'><img id="lasv1002" src='http://travelling.dw20.co.uk/images/photos/lasv1/vegas2.jpg' height='65px'><img id="lasv1001" src='http://travelling.dw20.co.uk/images/photos/lasv1/vegas1.jpg' height='65px'><img id="fiji1002" src='http://travelling.dw20.co.uk/images/photos/fiji1/FIJI.gif' height='65px'><img id="fiji1001" src='http://travelling.dw20.co.uk/images/photos/fiji1/fiji2.jpg' height='65px'><img id="aust1001" src='http://travelling.dw20.co.uk/images/photos/aust1/aus1.jpg' height='65px'> The ids should be available to you through PHP. It shouldn't be hard creating the list of images like I have here. That should more or less do it.
-
For your test link, remove the inline onclick call. Instead, change it to: <a id="testAjax" href="#">Hello</a> And add the following to the <head> of the document: <script type="text/javascript"> window.onload = function() { var testAjax = document.getElementById('testAjax'); testAjax.onclick = function() { showPhoto('aust1001'); } } </script> It should work with those changes.
-
You need to fetch the second query's results. You also need to swap your double and single quotes around: $tmp = mysql_query("SELECT staff FROM users WHERE username = '$username'"); $row = mysql_fetch_assoc($tmp); $staff = $row['staff']; switch($staff) { case "1": session_start(); $_SESSION['user'] = $_POST['username']; $_SESSION['permissions'] = "1"; break; case "2": . . . }
-
Nah, that shouldn't matter given the way JavaScript handles type. You could give it a hexadecimal number and it wouldn't care. Is all of your JavaScript within largephoto.js? Because the error reads like one of those runtime collisions I've mentioned a few times. If there's any JavaScript within <head>, then stick it all in an onload callback like so: <head> <script type="text/javascript"> window.onload = function() { //rest of your non-largephoto.js code } </script> </head>
-
Write it like: alert(url); Just to be sure. Putting it in the PHP won't help as the JavaScript is concerned with two things: 1. The actual HTML elements it needs to manipulate 2. The value of the $photo_id Both are available in the HTML file. No, the error is in the JavaScript itself. If all the code is stored within AJAX/largephoto.js, then it's not a runtime collision where the script loads before the rest of the document. Change showPhoto to the following: function showPhoto(str) { alert(str); xmlHttp = createXmlHttp() if (xmlHttp == null) { alert("Browser does not support HTTP Request"); return; } var url = "largephoto.php"; url += "?id=" + str; alert(url); url += "&sid=" + Math.random(); alert(url); xmlHttp.onreadystatechange = stateChanged; xmlHttp.open("GET", url, true) xmlHttp.send(null) }
-
For some reason, it looks like its choking on the photo id being passed to it. Do me a favor: alert the url variable after: url=url+"&sid="+Math.random() And let me know if it's being constructed correctly.
-
Can I see the code for showPhoto, because that's where the error is happening.
-
A good way to do it, since it appears you're using PHP to populate the smaller images, is to create an array of those images and add an onclick event to each one. I'm not sure what element contains these images, so my example will use a div with the id of "gallery." var gallery = document.getElementById('gallery'); var images = gallery.getElementsByTagName('img'); for(var i = 0; i < images.length; i++) { images[i].onclick = function() { showPhoto(this.id); } } . . . <div id="gallery"> <?php while($row = mysql_fetch_assoc($result)) { $photo_id = $row['photo_id']; $url = $row['url']; echo "<img id='$photo_id' src='$url' height='65px' />"; } ?> </div> I'm assuming that you're pulling the thumbnail info from a database, and that there's more than one image here.
-
It does, actually. You've just encountered one of the most common and frustrating JavaScript errors. Here's what's happening: All of your JavaScript functions and variables are being created before the actual document (i.e., the HTML) is rendered. This causes errors because none of the elements referenced by your script exist yet. So, you can't obtain them through getElementById because they're not there. In order to fix this, you need to wait for the document to load, then let JavaScript build its element references. Thankfully, there's an easy way to do this: <script type="text/javascript"> window.onload = function() { //rest of your script goes here } </script> This tells the rest of your script to wait until the document is loaded before getting references to the HTML elements your functions need. As an aside, you're better off initially testing your JavaScript applications in Firefox using the Firebug addon. Both give much better error messages, and Firebug actually highlights the line where the error occurred.
-
Well, first you need to get a hold of the object: var myEmbeddedObject = document.getElementsByTagName('embed')[0]; //may need to replace embed with object Then you attach a callback function to its onclick event handler. I tend to use anonymous (read: nameless) functions to do this as it's quick and easy: myEmbeddedObject.onclick = function() // <-- see? no name for the function { dropdowncontent.init("contentlink", "left-bottom", 300, "click") } As an aside, do you know if any of your JavaScript is working? I ask because I think it may not work as is. As you noticed in my first response, I had something along the lines of: <script type="text/javascript"> window.onload = function() { //stuff } </script> I do this because JavaScript has a tendency to attempt creating/linking functions before the elements they reference are rendered by the browser. It's a common error that virtually every JavaScript beginner makes, and is the cause of many headaches ("What do you mean my div has no properties?? It's right there!"). Also, as a general tip, you should strive for a completely unobtrusive style. This means completely separating your JavaScript from your markup. You're almost there right now, but you should replace things like: <div align="right"><a href="javascript:dropdowncontent.hidediv('subcontent2')">Close</a></div> With: var rightDiv = document.getElementById('rightDiv'); rightDiv.onclick = function() { dropdownconent.hidediv('subcontent2'); } . . . <div align="right"><a id="rightDiv" href="#">Close</a></div> It's a bit more work up front, but it makes it far easier to edit, modify, and maintain code as everything is centralized (all script code in one place, all markup in another). This centralization would allow you to put the script in an external file, usable by other pages, too. Just an idea if you continue down the JavaScript path. Unobtrusive JavaScript is widely considered best practice, and is the 'professional' way of doing things.
-
Hmm...I'm not sure if JavaScript will be able to get a hold of the flash button, as there's no markup within the HTML that represents that button. I could link the function to the entire embedded object, but that's most certainly not what you're looking for. I've never played around with Actionscript, but I hear it's based on ECMAScript, just like JavaScript. Is there a way for Actionscript to write JavaScript dynamically? Or to interact with already existing HTML markup? Because in order for what you want to work, either the guts of the .swf - in particular, the button - need to be exposed to JavaScript, or Actionscript must do the heavy lifting and toggle the div itself.
-
It doesn't work because you're blindly cutting and pasting what I wrote into your code in the hopes that shoehorning it in will work. You should post all of the relevant code pertaining to your problem. My original answer was based on a vague question, not on a particular, concrete problem, which is why it doesn't work out of the box. So, I'll need to see the markup of this button/link, the markup of this div, what 'dropdowncontent' is supposed to represent (I'm assuming the div in question), and anything else remotely relevant to the problem.
-
'Button-y' would be whatever it is you want this function to do when the button/link is clicked. Is this dropdown div supposed to open/close when the button is clicked?
-
Are you getting any JavaScript errors? If so, you should post them as it'll help us diagnose the problem. In the meantime, are you certain that you're successfully creating an XMLHTTP object? I ran into some problems creating one myself due to the way IE does things. Try the following: function createXmlHttp() { var xmlHttp; try { //try the hard way because IE7 doesn't implement XMLHttpRequest() properly var xmlVersions = new Array('MSXML2.XMLHTTP.6.0', 'MSXML2.XMLHTTP.5.0', 'MSXML2.XMLHTTP.4.0', 'MSXML2.XMLHTTP.3.0', 'MSXML2.XMLHTTP', 'Microsoft.XMLHTTP'); //iterate through the array until we get one that works for(var i = 0; i < xmlVersions.length && !xmlHttp; i++) { xmlHttp = new ActiveXObject(xmlVersions[i]); } } catch(e) { //try the easy way for the good browsers xmlHttp = new XMLHttpRequest(); } //return object (if we have one) or null return xmlHttp; }
-
That's a bit vague. Do you mean something like the following? <script type="text/javascript"> window.onload = function() { var contentLink = document.getElementById('contentlink'); contentLink.onclick = function() { //do button-y stuff here } } </script> . . . <a href="#" id="contentlink" rel="subcontent2">Search Box</a>
-
Look at Example 1: http://www.php.net/manual/en/language.operators.logical.php
-
Any ideas, thanks. PS. Ive removed my username and password from the top but it does connect. You need to actually execute the query and fetch the results: $query = "SELECT username FROM users WHERE username = '{$_POST['username']}'"; $result = mysql_query($query); $row = mysql_fetch_assoc($result); $user = $row['username']; Keep in mind, unless the username in the DB is actually 1, your test will fail every time.
-
First, a terminology lesson. Java != JavaScript. At all. They're not related. So, no, JavaScript is not a subset of Java, or anything like that. Java is an object-orientated language which runs on a virtual machine. It was created by Sun Microsystems. JavaScript is a browser-based scripting language originally developed by Netscape. JavaScript 'borrowed' the 'Java' portion of the name as a marketing ploy. No more, no less. I'm not trying to jump down your throat or anything, but it's something that a lot of beginning developers get wrong, and is a pet peeve of mine. To address your problem, you'll need to use AJAX. AJAX is an acronym for Asynchronous JavaScript and XML. The name is a bit misleading as you don't need XML to get it to work. AJAX is necessary for this because of its asynchronous nature. It allows you to send requests to the server, and obtain the results of those requests, without having to reload the page. Since you probably don't want to learn the ins-and-outs of AJAX, you can probably get away with leaning heavily on the jQuery (documentation: http://docs.jquery.com/Main_Page - download: http://docs.jquery.com/Downloading_jQuery) library to help you with the syntax. For this, you'll need a few things: 1. A callback function tied to the onclose event. 2. A flag to send to your PHP script to tell it to save the necessary data. 3. The PHP code that checks for the flag and saves the data. You should do something like: <script type="javascript" src="jquery-1.2.6.min.js"></script> <script type="javascript"> window.onclose = function() { $.post("yourScript.php", {toSave: "yes"}); //replace yourScript.php with the name of your script } </script> As you can see, no XML was used during the transfer of data. Instead, a simple name-value pair was sent to the server as POST data. And then, in your PHP: if(isset($_POST['toSave']) && $_POST['toSave'] === "yes") { //save your data to the db } Just an aside: You may have to use onunload instead of onclose. I'm not sure if onclose works with all browsers.
-
Did you fix the other variables too? As in: echo "<tr>"; echo "<td><font color='#000000' face='Arial' size='2'>{$row['n']}</font></td>"; echo "<td width='184'><font color='#000000' face='Arial' size='2'>{$row['cc']}</font></td>"; echo "<td width='68'><font color='#000000' face='Arial' size='2'>{$row['st']}</font></td>"; echo "<td><font color='#000000' face='Arial' size='2'>{$row['ea']}</font></td>"; echo "<td><font color='#000000' face='Arial' size='2'>{$row['cd']}</font></td>"; echo "<td><a href='{$row['url']}'><font color='#000000' face='Arial' size='2'>{$row['url']}</font></a></td>"; echo "</tr>"; ?? I ask because your orginal code snippet is filled with variables that seemingly come out of nowhere and aren't related to the query results at all. Remember: it's your job to bind query results to a variable. That's what the following is for: $row = mysql_fetch_assoc($data)