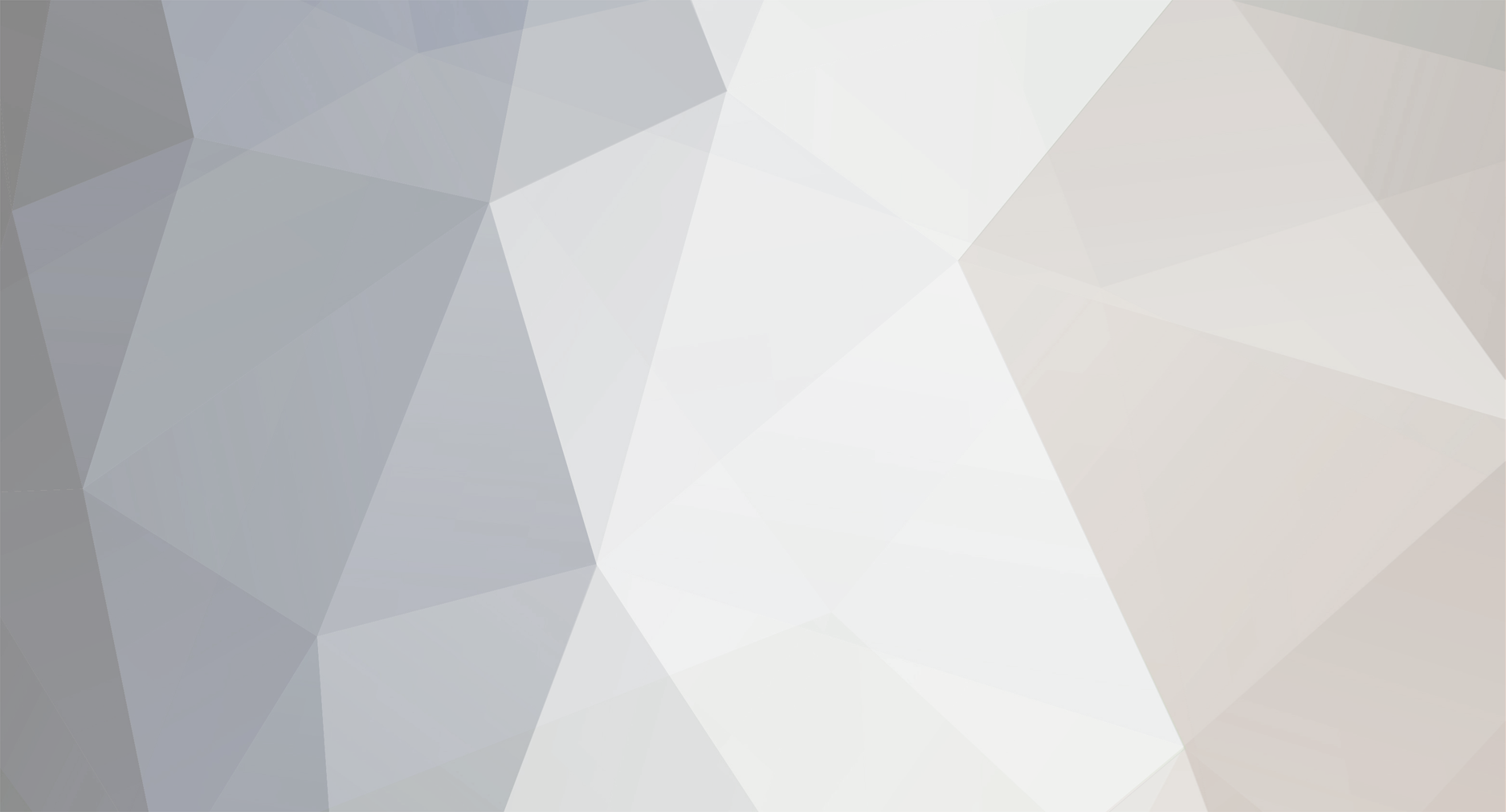
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
I'm going to try to put this as delicately as possible. I cannot imagine a situation where I absolutely have to do PHP work without some sort of connection to the internet. Even on a test server that's not connected/visible to the outside world, at least one computer in my vicinity will have internet access. I mean, I dunno...maybe you wrote this app because you did encounter such a situation. But I'm having a real hard time coming up with a scenario where I have to write server-side code without some sort of internet connection available. Again, not trying to be an ass, and I'm not trying to belabor the point, but I can't think of any realistic situation that would require me to whip out my phone to access something like this.
-
Wicked... you have a USB port connected to your brain?! Man you need to tell me how to do this so I can just carry a flashdrive around with all the info I need. Then I can just like scan in my textbooks and load it into my brain flashdrive and I'll ace my classes and exams. This is going to be awesome! Haha, fair enough. I wrote that under the (probably incorrect) assumption most people have access to laptops these days. (I've gotten a few free ones from clients who buy new ones and would rather throw away the old one then keep it). All the same, if you have an iPhone (which you would need to buy the app anyways) I would assume (never used an iphone) that one could open some local html documents on it. More to the point, you could save the following web address as a bookmark in Safari: http://www.php.net/quickref.php Every PHP function, listed alphabetically. Straight from the source itself. And without the bloat of having a separate application delivering the same info. Not trying to be Captain Buzzkill here, Masna, but I think you're trying to reinvent the wheel. Good for you if people buy it, but I wouldn't (if I had an iPhone).
-
Being a typical American, I'm only fluent in English. I had two years of French in high school, and an 'advanced' semester of it in college, but have since forgotten just about all of it. I don't mind, though. I hated French. Sounds like baby talk to me.
-
First, make an array: $trucks = array() Then fill it up: $trucks[] = new Truck(); That automatically places a new Truck object at the end of the array. Now, you can access it like normal: $trucks[0]->setName('name'); $trucks[0]->setDesc('desc'); Keep in mind, you can do this in a loop if you have a lot of objects you want to add to the array: $trucks = array(); for($i = 0; $i < 100; $i++) { $trucks[$i] = new Truck(); $trucks[$i]->setName('Truck_' . $i); $trucks[$i]->setDesc('Desc_' . $i); }
-
Sometimes you don't want an object, but rather one of its methods. Factory classes are a common example of this: class PersonFactory { public static function getPerson($id) { $query = "SELECT * FROM people WHERE id = '$id'"; $result = mysql_query($query); $row = mysql_fetch_assoc($result); $person = new Person($row['name'], $row['age']); return $person; } } class Person { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } } $myPerson = PersonFactory::getPerson(22); In this case, you don't need (or even want) to have a hold of a PersonFactory object. You just need a specific Person object.
-
You need to escape the quote that would otherwise cause the string to terminate: $var = "The length is 5' x 2\" and blah blah blah";
-
I'm so mad I could rip a napkin. *snicker*
-
[quote author=aximbigfan link=topic=112560.msg1012803#msg1012803 date=1223858191] And, I at least do know how to code fluently. I have written a full DB server and client API 100% from scratch. The system currently supports... * Reading DB keys * Writing DB keys * A dir/file structure * Imaging the DB * Restoring an image of the DB * Renaming (keys, dirs) *.... etc... The system has been up for at least a few weeks with no problems. It gets a Query about once every 5 minutes, since I have an app on my computer that fetches a new desktop wallpaper from the DB.[/quote] This is both impressive and depressing. Impressive for you, depressing for me. May I ask what language(s) your server and API were written in? Man, if only I was 10 years younger. In my day, we didn't have such easy access to programming technology. If you wanted to program, you went to college. No one in the 'real world' (read: non technical households) knew what *nix was, and you couldn't just download various programming platforms to your Windows 95 machine (sans Java, of course). Of course, back then, Perl CGI scripts were the popular way of processing user data, too.... Dammit, you kids get off my lawn! :shakes cane:
-
.NET requires Windows to work, correct? If so, then look at Ektron. They're a local company that sells a CMS written in ASP. Their clients include NASA, PlayStation, and others (http://www.ektron.com/customers.aspx). I'm not a fan of Windows or .NET (although I am enjoying my C# experience so far), but to claim that serious development is only done on a *nix platform is just false. In my area of the world, .NET skills are in far greater demand than PHP. So, I'm learning it in order to move away from freelancing. I figure my resume will look better with proficiency in both Microsoft and open-source technology on it, too.
-
In all seriousness, PHP allows one to switch between scripting and outputting HTML in the same file. Example: <?php $title = "testing"; ?> <html> <head> <title><?php echo $title; ?></title> </head> <body> Wow, look, I'm normal text in an HTML document! </body> </html> The colored bits are PHP. The rest is HTML. This file would be saved as something.php, but that doesn't change the fact that the black-and-white bits are HTML.
-
That is pretty damn awesome. *snicker*
-
Not only that, but that audio file will start automatically when the page is viewed, not when an image is clicked.
-
Uh, yeah, it would. PHP doesn't do client-side events.
-
sending multiple checkbox values to server (PHP)
KevinM1 replied to cougar23's topic in PHP Coding Help
Change the name of all the checkbox inputs to timeSlots[]. This will pass the values to PHP as elements of an array. Then you can echo them all: foreach($_POST['timeSlots'] as $time) { echo "$time<br />"; } -
[quote author=Maq link=topic=112560.msg1009691#msg1009691 date=1223520917] Seems like there are so many young people on these forums. I mean I'm only 22 but I keep seeing kids like 15-20 years old with more years experience than I have... :P [/quote] Hey, you think that's bad? I'm [b]28[/b]. :P
-
It can't be invoked by the client code. Example: class PrivateExample { private $msg = "Hello!"; public function __construct() {} private function getMsg() { return $this->msg; } } $example = new PrivateExample(); $msg = $example->getMsg(); // WON'T WORK Private functions are typically used as bookkeeping functions for the objects in which they reside. That, or in breaking down a complex public function into smaller parts that shouldn't be accessed directly by the world at large. OK I think I'm following you on this. In other words, private functions are functions only someone running Administrator codes can access - meaning, if I'm logged into my admin panel, then I have access to those Private functions? er am I off base here? Thanks Way off base. It has nothing to do with site administration or authorization. We should probably start at the beginning. Objects are datastructures, much like arrays or structs (or even functions). No more, no less. They are defined by classes, which, really, is another word for datatype. So, let's define a class: class Example { public $msg = "Hi, I'm an object property!" public function __construct() { echo "I'm the object constructor. I run every time a new Example object is instantiated!"; } } Pretty straightforward. It has one property ($msg) and one method, its constructor. Now, let's create a few of these objects in our client code: $example1 = new Example(); $example2 = new Example(); Again, simple. Now, what if you wanted to access the objects' property? As it stands now, you can access it directly: echo $example1->msg; Pretty cool, right? And the same goes for overwriting that value: $example1->msg = "I have a lovely bunch of coconuts"; But therein lies the problem. Any piece of code can just change the property directly. What if you didn't want the $msg property to be changed unless you explicitly gave permission to these changes? That's where the private keyword comes in. Let's change the class to: class Example { private $msg = "I'm private now!"; public function __construct() { echo "I'm the same as before"; } public function getMsg() { return $this->msg; } public function setMsg($someText) { $this->msg = $someText; } } $msg itself is now safe from being accidentally accessed by some rogue code. It can still be accessed and changed through the getMsg and setMsg functions. Indeed, those functions force all of the code that wants to access the $msg property to do it in those particular ways, ensuring that the value isn't overwritten by accident elsewhere. The same goes for private methods/functions. Let's say each object needs to access the database upon construction: class Example { private $msg; public function __construct($id) { $initData = $this->accessDB($id); $this->msg = $initData['msg']; } private function accessDB($id) { $query = "SELECT * FROM mytable WHERE id = '$id'"; $result = mysql_query($query); $data = mysql_fetch_assoc($result); return $data; } } // .... $example = new Example(23); The accessDB method is declared as private because it shouldn't be invoked by any other code in the script. Instead, it should only be invoked when the object is constructed.
-
It can't be invoked by the client code. Example: class PrivateExample { private $msg = "Hello!"; public function __construct() {} private function getMsg() { return $this->msg; } } $example = new PrivateExample(); $msg = $example->getMsg(); // WON'T WORK Private functions are typically used as bookkeeping functions for the objects in which they reside. That, or in breaking down a complex public function into smaller parts that shouldn't be accessed directly by the world at large.
-
Isn't Mario Nintendo or am I just getting too old for this stuff! No, you're right. Mario is Nintendo's most recognizable franchise. Pokemon, as the poster above you mentioned, is also Nintendo property.
-
Oh jeez, isn't that the truth. I keep thinking to myself that McCain would be much closer to Obama in the polls if we were having these debates sometime from 1920 - 1960. His rhetoric style borders on vaudeville at times. It just seems so awkward today. To be honest, I felt bad for McCain while watching him move around the stage. It's obvious that his age and war wounds are catching up with him. He just looked like a stiff, frail old man as he moved. It makes me wonder about his overall health, and makes me shudder at the possibility of Palin one day controlling the entire country. Oh well, I already voted. I got my absentee ballot over the weekend and already sent it in, so this debate and the next don't really matter to me.
-
Okay, good. I'm ~90% complete. I do need one extra thing from you, which I think I asked for before we got sidetracked with all of the issues in getting connected to the database. I need an empty website page. It needs to have the look and feel of your other pages (so, same layout, navigation, etc), but without any specific content within it. I need it for two reasons: 1. To display any errors that may occur to the user. 2. To display a "Thank you for signing up" message. Having pages like this will both inform the user of the status of their submission and allow them to continue navigating through your site.
-
So long as it lists anything regarding mysqli, I'll be all set. I could do it the normal mysql way (like we did for the test script), but mysqli has a couple of features which saves me a little bit of work. It's a bit more secure, for one, with its prepared statements. And it automatically escapes potentially dangerous characters without me having to call mysql_real_escape_string() for every inputted value. One last value to check: There should be something within the PHP Core ini which says 'magic_quotes_gpc'. Can you tell me if that's on or off? You should be able to find it by searching for that value ('magic_quotes_gpc') directly.
-
I could never get into the MGS series. It's too schitzo for my tastes. I never liked how it oscillated between being incredibly detailed and realistic to being way, way, way over the top and convoluted like really pretentious anime. And this is coming from someone who enjoyed Neon Genesis Evangelion.
-
It's actually pretty damn solid, at least, with the few games I've tried. The biggest flaw is the lack of any kind of lobby for any of the games. You're either playing online or not. This flaw is most notable with Soul Calibur IV. It takes forever to find a ranked match without lag. It's horrible sitting there for 10 minutes refreshing the list of available games, only to actually play for ~1:30. Although, that could just be a Namco thing. Their online capabilities for Tekken 5: Dark Resurrection are pretty shoddy as well. In the cases of Warhawk, Resistance, and GTA: IV, I haven't had many issues. A few disconnects and freezes, but it's a rarity. I've owned my PS3 since launch, and those issues have come up maybe 10 times total in two years. The PS3 really needs to focus more on the social aspect of online gaming. It's nice that they've introduced Trophies (similar to XBL's Achievements) and their own brand of gamer cards (which take forever to load and display), but there's nothing beyond that, really. Their Home project is supposed to remedy that, but I'm not confident that it'll actually see the light of day anytime soon. It's been in beta forever, with very little forward progress from what I can see. Which is a shame, too, because Warhawk is supposed to have its own war-room, complete with a big table with virtual miniatures so you can plan various attack strategies. The PlayStation Network is great considering it's free. The store is pretty good, too. It has a much better layout than the XBL Store, but it's severely lacking in 3rd party support, especially when it comes to classic PS1 games. I would love to purchase a new copy of Vagrant Story, for example, but the pickings are slim. The best PS1 game currently available is probably Castlevania: Symphony of the Night. Gotta love the various themes/skins available, though. There's a ton out there, all for free.
-
Ack, the error is my fault. D'oh! It should be: <?php phpinfo(); ?>