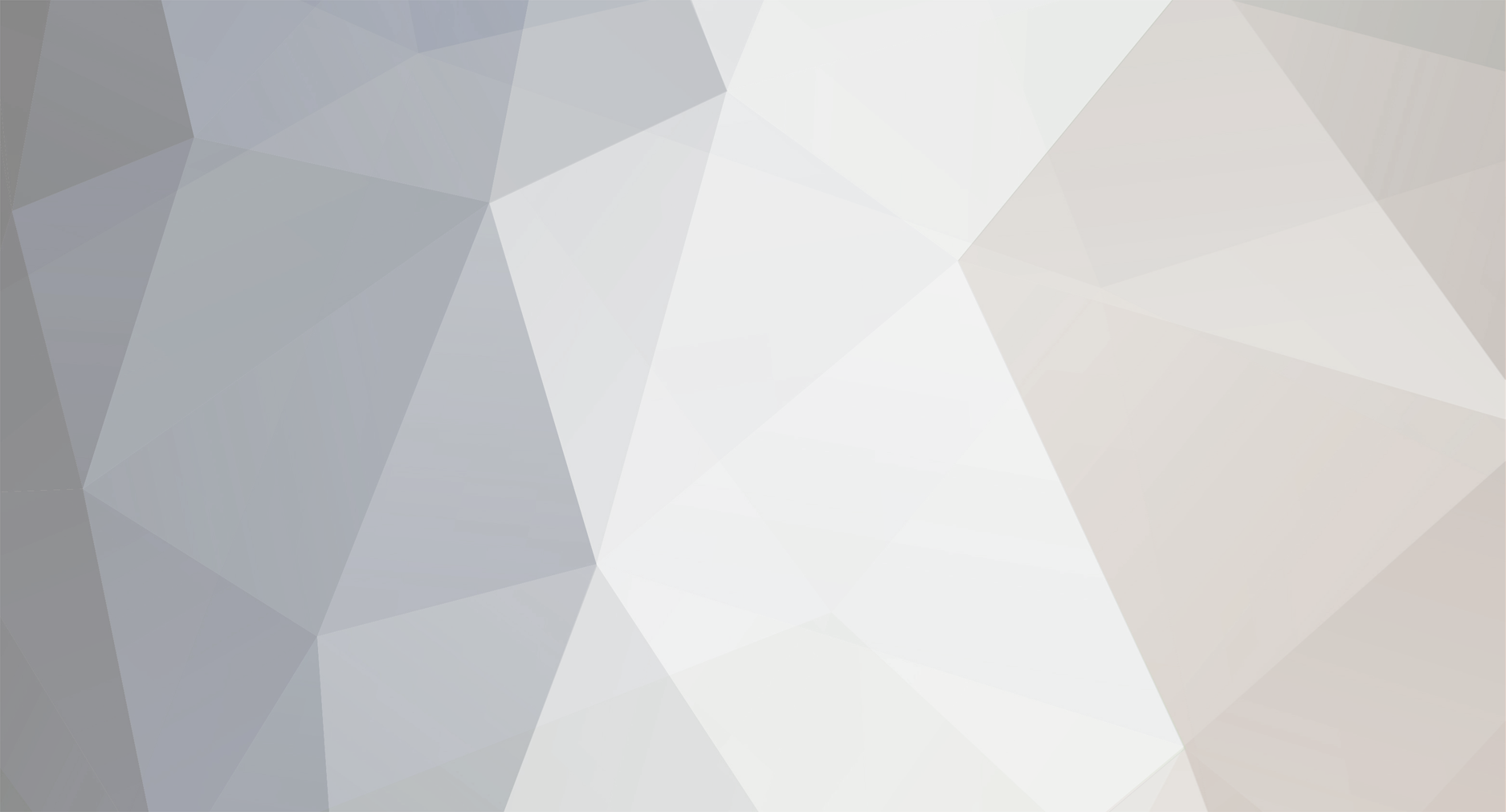
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
This topic has been moved to Miscellaneous. http://www.phpfreaks.com/forums/index.php?topic=351991.0
-
The <select> builder? Sure. Just be sure to fill in all the blanks. For example, don't just blindly throw myValidate() into your code. My examples were largely pseudo-code, intending to show the overall thought process. You'll need to understand what I was trying to tell you and figure out how to put it into what you already have. Simple cut/paste of everything won't work. The query above can likely be used as-is (be sure to test it and debug it if it doesn't work right initially), but the <select> builder needs its while-loop condition added, $name replaced with $fab1, etc.
-
Okay, so: $fab1 = myValidate($_POST['fab1']); // again, replace with whatever validation - if any - that you do . . . $query = "UPDATE testbed SET ab = '$ab', date = '$date', part = '$part', rev = '$rev' , partdesc = '$partdesc' , ncmrqty = '$ncmrqty' , comp = '$comp' , ncmrid = '$ncmrid' , rma = '$rma' , jno = '$jno' , fdt = '$fdt' , cof = '$cof' , fab1 = '" . mysqli_real_escape_string($dbc, $fab1) . "' , fab2 = '$fab2' , fab3 = '$fab3' , non = '$non' , dis = '$dis' , comm = '$comm' , caad = '$caad' , po = '$po' , pod = '$pod' , dri = '$dri' WHERE id = '$id'";
-
Okay, let's see if I can explain it better... Right now, you have a whole section that looks like: $someVar = mysqli_real_escape_string($dbc, trim($_POST['someVar'])); $someVar2 = mysqli_real_escape_string($dbc, trim($_POST['someVar2'])); $someVar3 = mysqli_real_escape_string($dbc, trim($_POST['someVar3'])); . . . What's happening here is that you're passing copies of $_POST values to the escape function, and then storing the results in variables. Those variables now contain escaped characters, meaning something like: $someVar == $_POST['someVar'] May not be true. All I'm saying is, instead of immediately escaping and storing $_POST['name'] in a variable, wait until you need to use it in the INSERT/UPDATE query, and to do it within the query itself so the variable doesn't retain the escaped characters. There's no after query escaping happening here. Not sure where you got that impression. In other words: $name = myValidate($_POST['name']); // myValidate represents whatever validation - if any - you do. Written as a function here for brevity, but could be any kind of statement(s) . . . // insert/update query $query = "UPDATE/INSERT/WHATEVER ... name = '" . mysqli_real_escape_string($dbc, $name) . "' ... "; // note how the escape is happening INSIDE the query, before it's executed . . . // after the select query, to build the <select> HTML form input ?> <select> <?php while(/*something*/) { ?> <option value="$value" <?php if ($name && $name == $value) { echo "selected"; } ?> > <?php } // end while ?> </select>
-
Those books were recommended by one of my friends as well, will definitely need to purchase it/them. I'm assuming it is a several book series? AFAIK, there are two. At least, there were two when I bought them a few years ago.
-
PHP or Jquery or should I use something else????
KevinM1 replied to Tech boy's topic in PHP Coding Help
PHP is server side only. Meaning, it can't 'see' what the client does (including mouse movements/clicks). It also has no native concept of events. -
Reread what I wrote. I do want you to escape it after you run it through your validation code and store it in a separate variable. Escape it in place, like: $query = "UPDATE/INSERT/WHATEVER ... name = '" . mysqli_real_escape_string($dbc, $name) . "' ... "; Why do it this way? Because if you escape it immediately, $name will contain escape characters, so any comparisons to the inserted value when you're building the <select> may not work (remember, you need to check $name against every value returned from your SELECT query to determine which name was entered). Escaping it in place makes a copy* of $name safe in the query, but the escape doesn't persist after that. I hope this makes sense. *The vast majority of PHP functions pass their parameters by value. That means an automatically generated copy of the parameter is passed into the function.
-
Get the Thinking in C++ books. They're great.
-
Can you do any kind of stack trace both with and without the alias? At the very least, you can verify what you think is happening.
-
Okay, so here's what you want to do: Grab a hold of $_POST['name'], or whatever it is you call it, and run it through whatever you use for validation/sanitation except the escape. Store it in a variable: $name = myValidation($_POST['name']); You don't need a function for this. I'm using one for brevity, and because I don't know what you're actually doing for validation. So, replace my function with whatever you're actually doing. Now that you have it in a separate variable, you can use it in multiple ways, so run that new variable through the escape and insert as normal. When it comes time to make your select options, simply do: <select> <?php while(/*something*/) { ?> <option value="$value" <?php if ($name && $name == $value) { echo "selected"; } ?> > <?php } // end while ?> </select> Basically, you just need to check if the last name entered matches a name in the list. If so, mark it as selected.
-
I'm not quite sure what you want the workflow to be. What I get is: 1. You want the <select> to be filled with names from the user table 2. You want whatever name the end user selects to be sent to the ncmr table I'm unsure of the rest. Can you describe it in terms of what the end user should do/see?
-
What I mean by separating PHP and XHTML in separate sections I mean one page two distinct areas. I use to do that, and then was told to not because it is better to wrap PHP around everything than to go in and out of PHP and XHTML... Ah. That's an odd suggestion, especially considering how larger PHP apps do things. Mostly HTML templates/views are the way to go. Like I said before, they should have just have the bare minimum amount of PHP to display the results of some other process. ~99% of them should be pure markup. Now you see why I probably get confused about how to do things a lot, being told and taught to do it one way, instead of another sort of bites one in the ass quite a bit. I understand completely. All of our members have different experience levels. While the badged members have years of professional experience, the non-badged have a variety of different backgrounds. Not to imply that the non-badged members give bad advice as a rule (many give great advice), just that it's good to think things through and verify when you can. If something smells funny to you, bring it up with your reasons why. Just remember to be as clear/explicit as possible, so everyone isn't assuming at each other.
-
What I mean by separating PHP and XHTML in separate sections I mean one page two distinct areas. I use to do that, and then was told to not because it is better to wrap PHP around everything than to go in and out of PHP and XHTML... Ah. That's an odd suggestion, especially considering how larger PHP apps do things. Mostly HTML templates/views are the way to go. Like I said before, they should have just have the bare minimum amount of PHP to display the results of some other process. ~99% of them should be pure markup.
-
You don't necessarily have to have separate pages, as in, separate files. For pages that post to themselves, having everything in one file tends to be simpler as you can display errors right on the form without having to play hidden input/session shuffle. What's important is maintaining internal discipline and keeping clear boundaries between different kinds of code. EDIT: How code is structured, after everything has been include-ed or whatnot, is what's important. The physical structure (files, folders) should be whatever makes the most sense to your particular workflow and comfort.
-
I'm more asking where to put this code... prior the submit button or during the post? It should be somewhere in your post handling code. It's probably too late to change now, but if you want to save some headaches in the future, you should separate as much PHP from your HTML as possible. Putting the vast majority of your PHP first will let you separate logic from presentation, and make everything a lot easier. Well-formed PHP scripts tend to look like: <?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { // check to see if we're accessing the page via POST // all form handling code // this allows you to store results in variables and redirect the user (if necessary) without getting those // pesky 'Headers already sent' errors // this is also where error handling code goes } ?> <!-- HTML goes here. If written correctly, the only PHP you need here are statements echoing results, conditionals, and/or loops. Simple code with minimal logic to display errors or results. If you find yourself doing any processing, accessing the db, etc., you're doing it wrong. --> You should always strive to separate your concerns as much as possible. HTML has a different 'job' than PHP. HTML is only concerned with presentation. PHP is concerned with back end logic. The only PHP that should be in HTML is what is necessary to complete the presentation. Similarly, HTML and CSS should be separated, as HTML deals with layout and CSS with formatting. And JavaScript should be written unobtrusively (meaning no inline JS in your HTML) as JavaScript manipulates the document, but is not part of it. If you can think of code in terms of layered components, you'll have a much easier time. HTML is really just a skin on top of your app.
-
This. MySQL is a relational database. If you have data that's separated but related to other data, then normalize their relationship. Using a RDB like a giant spreadsheet means you're likely doing it wrong.
-
Wow, I sense a bit of bitter impatience there. Considering I didn't even get the code I requested until nearly 7 hours after the fact around 8pm my time and when I'm trying to spend time with my family... Anyways, since I'm getting the gloomy feeling that our help is more expected rather than appreciated, good luck with your script, I hope you fix the issue. Actually I'm not the impatient one, I am trying to get this working for a company I work for, they are the ones hounding me. I am feeling the pressure on my end to do something I've really never done before. So sorry you feel that way, but I like my job, and I really don't want to loose it over something as "simple" as people here is making it out to be. When to me it's not. Honestly I learned how to code XHTML with PHP and SQL in a little over 2.5 months, what you see here is a culmination of everything I've learned from two books, no classes, and a lot of pressure on me to do so because this economy has made it where it is the employer is always right, and if you can't do it they will hire someone else who can, and my skillset has a flood of people out there that are unemployed, so yes I am in a way impatient because I don't want to loose my job... can you blame me? I am a graphic designer and a computer tech by trade, I never made a website or cracked open a coding book till two months ago, and now I am stuck on this, asking for help, and people here are telling me that x part is wrong, and I'm not sanitizing anything, etc... I've said in the past that I am new at this, this is the first site I registered to, to get help, now I am being pressured at work to do something I have a fleeting grasp of doing, and people here are telling me that I should know what I am doing, and that instead of telling me what is wrong by showing me, they are saying you aren't doing X... when I have no clue what they are saying... and I keep on telling people that as well... but people here don't seem to understand that when I say I don't understand and I keep asking over and over again the same thing, it means I am not understanding what you are saying. Matt, we're not here to be your tutors. We are here to help, but trying to teach any member PHP from nothing is beyond the scope of what we do. Part of the problem is that you don't read. There's a reason why people keep repeating themselves to you (your words). There's a reason why this thread is now on page 4, and the sanitizing thread went 6 pages. There are only so many ways for us to explain things, and often you have the gall to imply that we're wrong (see: sanitizing thread), or that you did things right (see: your HTML header/footer issue, where your HTML is a bonafide mess). I don't know if you know this, but we're all volunteers. Even the people with titles and badges. None of us see one red cent from being here. We try to help the best we can because we were all newbies once and we want to pay the community back. That said, we're doing this while also dealing with work, family, health issues, etc. What this means is that while we may be sympathetic to your plight, you're not entitled to specialized treatment beyond what other members get. Getting frustrated and pissy at the people offering you free professional help is only going to decrease the chances of anyone answering one of your questions in the future. So, here's what you do: Leave your preconceptions at the door. You're not at the point where you can even consider how things ought to work, so what's the point of being frustrated with how it does work? Leave your ego at the door, too. 20+ years IT, several years as a cook... who gives a crap? Programming is significantly different than both. Leave that all behind and embrace the differences. Finally, take the time to read and decipher what we're saying. We're not going to stop and explain every piece of basic jargon we say. That would be asinine, especially given how rudimentary and universal it is (even in Objective-C and Java, which I thought you were leaving us for...). Reading comprehension and the ability to follow instructions are probably the two most critical skills a programmer can have.
-
Actually, the way he has it makes sense. The page is obviously first accessed via URL, which means GET. Since it can post to itself, the rest is handled by POST. It's a bit sloppy in execution, but the idea is semantically correct and RESTful. It's a fairly common pattern in MVC. An action method accessed via GET to show a page, and an action method with the same name to handle the postback.
-
Access different classes from another class
KevinM1 replied to Twister1004's topic in PHP Coding Help
This is pretty much the antithesis to good OOP. The whole idea behind OOP is that objects are separate and don't know the internals of another object. Having child objects work back up the chain to call a superobject's (whose very name should be a warning sign) method is completely ass backwards. What you need to do is rename your OOP class to something better, and treat it as a Facade (it's a design pattern, google it)*. Then, make the Login and Report classes not inherit from it. The Facade's login method would delegate to the Login object and Report object as necessary. *You likely don't even need a Facade, but it depends on the complexity of your system.