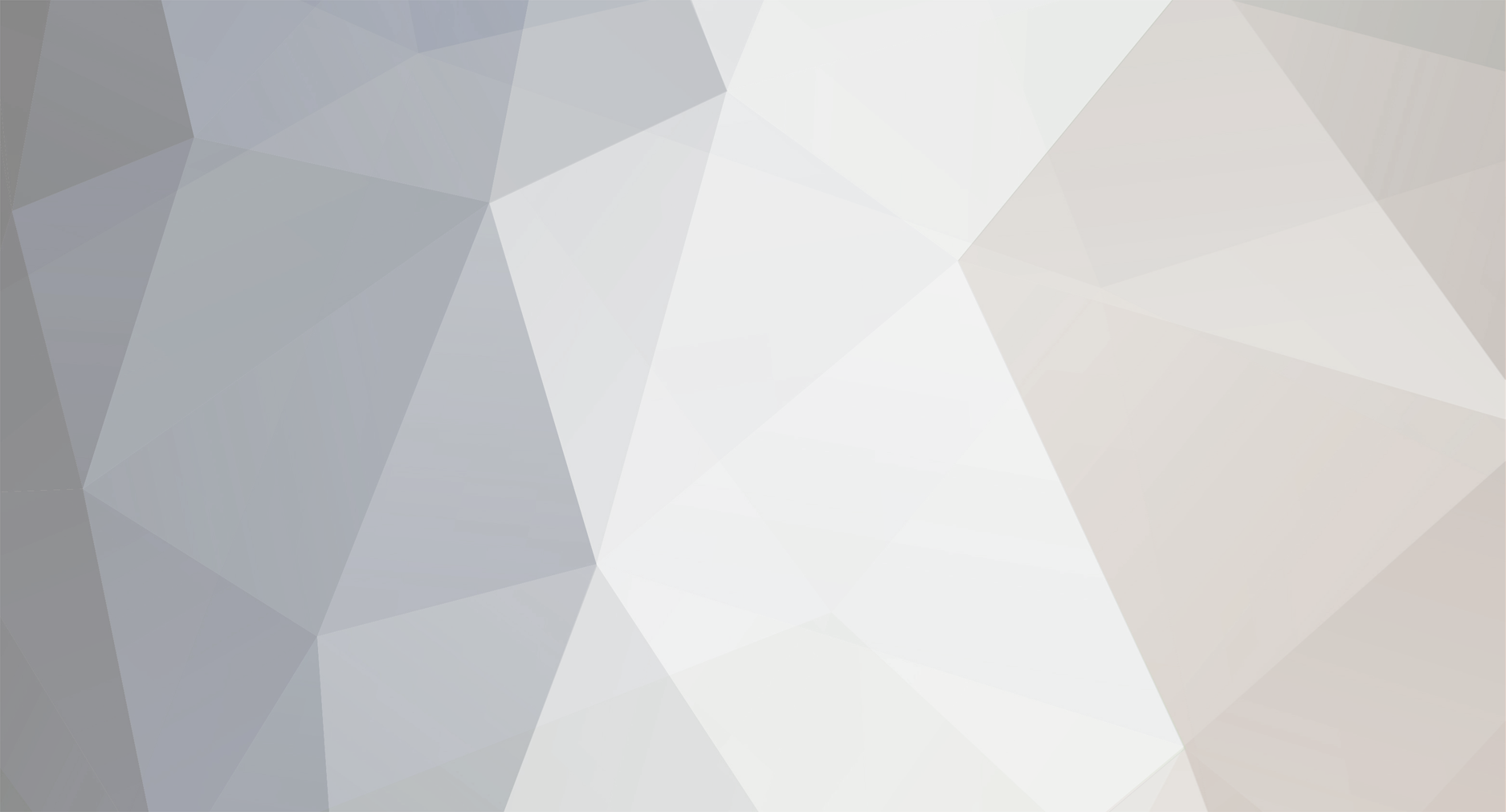
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Understanding get, set and property overloading
KevinM1 replied to Drongo_III's topic in PHP Coding Help
One of the weaknesses of OOP in PHP is that it allows you to make public fields on the fly. Good OOP is all about encapsulation, which means having private or protected fields. That said, sometimes it's easier/more readable to reference a field as though it was public, in order to remove otherwise necessary getField()/setField() methods that pollute classes. So, if your class isn't going to be doing anything aside from setting or returning values, using __get and __set is an easier way to go. Note, however, that you don't really use __get and __set properly in your code. Again, those two magic methods are used to give the appearance of public fields. Internally, they should still be private or protected: class Example { private $allowedProps = array('prop1', 'prop2', 'prop3'); private $data = array(); public function __set($propName, $propValue) { if (in_array($propName, $this->allowedProps)) { // set property } else { // error } } public function __get($propName) { if (array_key_exists($propName, $this->data)) { // get property } else { // error } } } In the example above, there are two private arrays. The first contains the names of all allowed fields, so that not just any field can be created/used. Ideally, you'd have some idea of what an object should contain. The second array is the actual data. __get and __set check to see that: 1. The field that's trying to be accessed should be accessed 2. That data exists, or should be created -
Power comes from having a contract. Yeah, it sucks to have to play games in the legal system, but that's the only way to do it. Next to the supplies you need to have in order to create sites (computer, software, etc.), a lawyer is the best investment you can make. And, if you want to get paid, there's nothing wrong with writing a contract specifying that you will get a portion of the agreed upon fee at certain milestones in the project. In fact, that's probably the best way to do it, as it forces the client to honor the contract early, and you won't spend extra time working on stuff that hasn't been paid for. Finally, why wouldn't you work remotely? You should never do work directly on the server. Get yourself VirtualBox, and install whatever flavor of linux you like on it. Install PHP, whatever database the client wants, and some sort of version control software (Git, Subversion), so you can track your changes without overwriting old copies. Do your development there, and push whatever you need to the server when you need to do it.
-
I have to disagree with sunfighter. To me, it's way too busy. The graphical element that comprises the footer makes it hard to determine if there's more content below it. You have a rather tall header, followed by a surprisingly spartan navigation menu, followed by a social media menu. The content is buried (literally, given the footer) under all of this. To make matters worse, the turquoise background and gray text is somewhat hard to read. The site completely fails the "at-a-glance" test. What's really bad is that each page obviously, and distractingly, displays all of the images used for the header animations before hiding them. Each page takes about two seconds to 'collapse' into the right shape. My suggestions: 1. Don't absolutely position the footer. 2. Move the social media menu - either consolidate it with the header, or merge it with the other social media box. 3. Improve the header. Two white boxes with rotating still images looks amateurish. You'd be better off with a bigger image cycle somewhere in your content area. 4. If you need to hard code the cycle images, give them display: none. 5. Use a color for your font that has better contrast.
-
Like the error says, no, it doesn't make sense to pass a query string to an include function. It's looking for a file at the path sent into the function. Unless you have a file named 'file.php?id=1', you're not going to get a match. Really, the error message is pretty explanatory about what include is trying to do and why it's failing. And, no switching to one of the others, like require, wouldn't work either as they all work the same way. What you can do is simply have file.php include the file you want. Your included code will be pasted, line by line, in memory at that spot, so you should still have access to $_GET without any extra work.
-
...so, this is where bad analogies go to die. Good to know.
-
I agree that you need some CSS hover effects for the main navigation. I'd also do away with its drop shadow entirely. Shadows should follow the shape of the elements they're supposed to be stemming from. It just looks sloppy if they don't. If you're going to use a drop shadow, you should emulate what you did on the "Find Bounties" bar. That said, I think the gradient can stand by itself without any shadow at all. In the "Find Bounties" bar, the "Bounty Amount" is too short, with the drop down arrow covering the 't' in 'Amount'. The same goes for the last 's' in "# of Responses."
-
If you're using classes simply to group functions by theme, you're not doing OOP. You're only adding unneeded complexity to what could easily be solved simply by having pure function library files. OOP is something that should not be attempted until you have a firm grasp of the fundamentals. Otherwise you'll just end up confusing yourself. If you insist on using spl_autoload_register, here's how you'd do it: function myLoader($className) { include_once("classes/" . $className . ".php"); } spl_autoload_register("myLoader");
-
Why do you want to learn any scripting language? Like just about everything else in life, what you get out of it is directionally proportional to the amount of effort you put into understanding it. So, instead of vague questions, why not just be honest with us and tell us what's on your mind?
-
The two are hardly comparable as they're generally (with the exception of Node.js) used for two entirely different things and are executed in two entirely different environments.
-
What are your (both xyph and Dan) thoughts about using a timestamp as a salt? It has the following benefits: Always unique per user. Fairly randomized on its own. Fairly long. Difficult to guess as it can look like benign user profile data (it doesn't need to be labeled as a dedicated salt column, although obfuscation is admittedly a weak benefit).
-
Look into using trim
-
1. Do not create a new thread for the same problem. 2. Have you tried checking to see if $m_tag actually contains a value, as I suggested here: http://www.phpfreaks.com/forums/index.php?topic=347751.msg1641373#msg1641373 ? EDIT: Topics merged. Let's not do that again, eh?
-
I 'unsolved' this thread due to more questions being asked.
-
There's nothing similar that I've found in my searches for something similar (which sucks). I generally ask questions at Stack Overflow and the official MSDN forums.
-
This topic has been moved to Other Programming Languages. http://www.phpfreaks.com/forums/index.php?topic=347880.0
-
There's a difference between defining a function and invoking a function. A function definition looks like: function someFunction(/* argument list */) { // function body } Function definitions should be written first, before any other code in order to ensure that they're available when you want to use them. Funciton invocations look simply like: someFunction(/* arguments */); // or, if the function returns a value $var = someFunction(/* arguments */); In order to have a recursive function (that is, a function that calls itself), you need to do something along the lines of: function someFunction(/* argument list */) { someFunction(/* args */); // escape clause } Note the escape clause. Without that, the function would loop for an infinite amount of time. An escape clause is the part of your code that says "Stop calling yourself." An example: function factorial($x) { if ($x == 0) // escape clause return 1; else return $x * factorial($x - 1); } echo factorial(5);
-
Initial thoughts: Main navigation text and "Fresh from the Oven"/"Connect with us" text is blurry. The bulleted list needs more contrast, as its green-blue colors blends with the blue of the background. Kinda disappointing that you don't use a fluid layout. Why does the shop page/site look so different? I wouldn't launch what you have until the shop site fits in with the rest of the redesign. Other than that, nice job. Do you have a mobile-friendly site? Nothing would rock more than being able to order a pie from your phone. Perfect for that last minute, "I'm stuck in line for X-mas shopping and just had a flash of inspiration" gift idea.
-
You need a footer, desperately.
-
It's a broken link for me.
-
This is a great problem to force you (and others) to start thinking like a programmer. Like with other problems, what you need to do is start jotting down what you perceive to be the right way to go re: table columns, and then normalize. Right now, it's hard to tell if you want 1:* relationships, which would necessitate a foreign key in one of your tables, or *:* relationships, which would necessitate pivot tables, or a mix between the two. So questions to answer: How many user levels are you anticipating? What rights should each user level have? How many moderators can an individual sub-forum have? The answers to these questions should inform your design. Remember: good programmers tend to use paper or a whiteboard before writing code. Don't be in a rush to sit down and code immediately.
-
So... where's you're mysql_query() call, then? And are you expecting to have many IDs? You need to do something along the lines of: $checker = "SELECT ID FROM edible_uses"; $result = mysql_query($result); while($row = mysql_fetch_array($result)) { // do something with every $row['ID'] you get }
-
This topic has been moved to Miscellaneous. http://www.phpfreaks.com/forums/index.php?topic=347856.0
-
You need to try a SELECT query on the date/time/whatever it is that would indicate a conflict before attempting to INSERT a new reservation. Something like (pseudo-code, adjust to fit your needs): $query = "SELECT id FROM reservations WHERE day = $day AND start_time >= $start_time AND end_time <= $end_time"; When you run the query, count the number of rows it returns. If it's >= 1, you have reservation(s) booked already: $result = mysql_query($query); if (mysql_num_rows($result) >= 1) { // error: something has already been reserved } else { // insert new reservation }
-
Comment out your query, i.e.: /*$sql = mysql_query("INSERT INTO products (product_name, price, details, category, subcategory, m_tag, date_added) VALUES('$product_name','$price','$details','$category','$subcategory','$m_tag',now())") or die (mysql_error()); $pid = mysql_insert_id(); // Place image in the folder $newname = "$pid.jpg"; move_uploaded_file( $_FILES['fileField']['tmp_name'], "../inventory_images/$newname"); header("location: inventory_list.php"); */ And literally echo out $m_tag to the screen: echo "m_tag is: $m_tag"; Do you see a value?