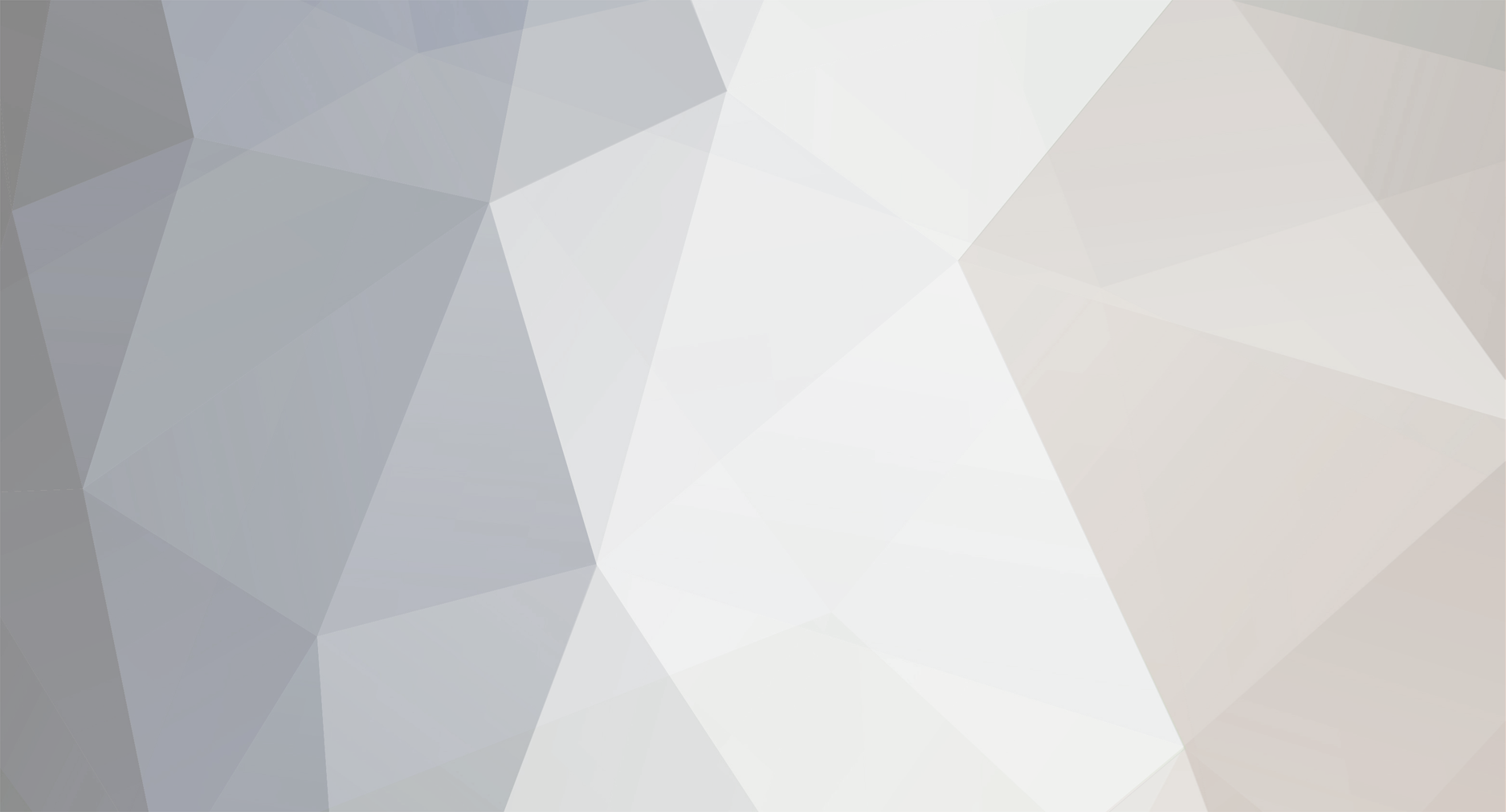
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
using db connection in oop, guidence needed
KevinM1 replied to The14thGOD's topic in PHP Coding Help
Look up dependency injection/inversion of control. Essentially, you have a class called a dependency injection container which takes an object (in your case, your User object) and the dependency it relies on (the db connection), and does the dirty work of injecting that dependency into the object for you. I believe Symfony has a DI container you can plug into your system with minimal fuss. -
I don't see why the title and user names should be placed in visible inputs at all. If a user sees a textbox, they're going to think the value inside is editable. Having them not be editable will only lead to frustration for the end user. Your best bet is to use form inputs only for what you want the user to submit their own data with. Data that wires up your db tables - like article id and user id (please tell me you're not trying to submit a comment based on their name) - is better sent along in hidden inputs. Finally, setting a form input to 'disabled' has no security benefit whatsoever. Your HTML source will always be visible to the curious, and you can safely assume that anyone trying to harm your system is capable of making their own form with the names of your inputs, with its action attribute set as your form handler script.
-
Function that returns several values, how to OOP call it ?
KevinM1 replied to Dzherzinsky's topic in PHP Coding Help
The problem is that you're not passing an array into your object. In your script, you need: $v = array(50, 20, 600); $suzuki = new Motorbike(); $speeds = $suzuki->speed($v); echo "Speeds are: "; foreach($speeds as $speed) { echo $speed . "<br />"; } Remember: a class is merely a blueprint, much like a function definition. It doesn't become real until you instantiate it (create an object of your class' type) by calling "new classname();" Just because you define a method (class function) to take an array as a parameter, that doesn't mean it magically happens. You still need to construct the array and pass it into the method in the script yourself. -
Function that returns several values, how to OOP call it ?
KevinM1 replied to Dzherzinsky's topic in PHP Coding Help
Can I see your code that's trying it with the array? -
This topic has been moved to Miscellaneous. http://www.phpfreaks.com/forums/index.php?topic=342919.0
-
I don't know enough about your system architecture to say.
-
mysql_real_escape_string() is what you want to use before utilizing variables to interacte with a database: http://php.net/manual/en/function.mysql-real-escape-string.php That and validating the data. that only helps if he uses mysql as his database. If it's not, then he should use the correct escaping method used by his database. Or, parameterized queries. addslashes does nothing for security.
-
Function that returns several values, how to OOP call it ?
KevinM1 replied to Dzherzinsky's topic in PHP Coding Help
You're confused about class variables, arrays, and how they work. Try: class Motorbike extends Vehicle { protected $bikes_speed = array(); public function speed ($d1, $d2, $d3) { // the 'this' keyword denotes that the variable $bikes_speed belongs to the current object $this->bikes_speed[] = $d1/10; $this->bikes_speed[] = $d2/10; $this->bikes_speed[] = $d3/10; return $this->bikes_speed; } } In your script: $v1 = 50; $v2 = 20; $v3 = 600; $suzuki = new Motorbike(); $speeds = $suzuki->speed($v1, $v2, $v3); echo "Speeds are: "; foreach($speeds as $speed) { echo $speed . "<br />"; } That said, you could simplify your speed method by accepting an array: public function speed($speeds) { foreach($speeds as $speed) { $this->bikes_speed[] = $speed/10; } return $this->bikes_speed; } But, really, before you even attempt to play with OOP, you need to get the basics of PHP down first. If you can't handle 1-dimensional arrays, foreach loops, and how to echo values correctly, you won't have a chance with objects. -
I haven't seen your code, aside from the snippet you have in the first post in this thread.
-
Are you completely married to step 3? Most sites have a text area directly below their content to allow users to add comments. It's easier to implement that way (although certainly not impossible to do it your way). Step 1: The "Login or Register" portion should be based on a conditional. If the person is not logged in, then it should show the login/register buttons. If they are logged in, it should show an "Add Comment" (or something similar) button. The easiest way to do this would be to use sessions. Step 2: When you go to the login screen, it will need to know what page you were on when you left to log in. There are a couple ways to do this - sessions (again), or query string values. I prefer query string values here. The values should likely be content/page type (in this case, articles), and its particular id number. So, something like site.com/login.php?page=articles&id=334 Step 3: The same thing, really. Use a header redirect with the same query string values appended to the end. Step 4: Easiest step. Just redirect back to the article. Not sure what else you'd need without seeing actual code, but that's how I'd approach it.
-
To be honest, I'm wondering why your bootstrap is even trying to handle the header and footer. That should be the responsibility of your view logic.
-
Correct. Understood, but I also don't want to overdo it and check more than needed. Unfortunately, there's no hard, fast rule which specifies what would be needed. That's where thorough testing comes into play. I think part of your problem is your design. From what little code I've seen from you, it looks like you're doing things in just about the hardest way possible. A login system and a comment system each has only a couple moving parts - is the person a registered user? are they logged in? can you tie their comment to a particular article? The way you have things structured, and how you try to jump around from piece to piece, would leave many confused. Design is one of the harder things to learn, and is usually only learned after hours of sweat and tears.
-
Thoughts: Serif fonts are generally unfriendly for the web. Look for a sans-serif alternative. Along the same lines, I also dislike the small indent you have with the body of your text. It's so minute that all it does is make your site appear that it has alignment problems. If this is your site to advertise yourself, then why is your blog the home page? Look at other, professional freelance sites. The ones that know what they're doing have their advertising spiel and a couple examples of their best work front and center. A blog is not a selling point. Examples of your work are. Don't make your entire portfolio your home page. Just the one or two projects you feel best highlight what you can do. Speaking of your portfolio, you need links to your actual projects, and better large images. A small corner of a site here, a header graphic there doesn't say much of anything. In fact, it creates the impression that you're not proud of your work. Else, why show so little? Do you have a logo for your own business? Even if you're not an official business entity, you still need to look the part online, on your invoices, business cards, etc. Make a logo or icon, even if it's simple.
-
If a row was actually returned, then yes, you can assume that id and first_name are present. But, like voip was trying to point out, you need to ensure that you actually have a db row returned from your query before you can start working on the data you expect it to contain. Technically, no. I tend to declare variables before using them because it makes code more readable. I hate seeing magic variables just pop up. It's also required in other languages, which are tighter about type. Finally, as a tip, you don't need to initialize a variable to an empty string unless you're going to add to the string later. Something like: $message = ''; foreach($something as $value) { $message .= " something else "; } Doing it just because, especially if you're going to be putting non-string data in a variable (like your memberID), is pointless. A simple: $id, $name; Will suffice, as it declares them before use. Again, you need to check that your query returned results. Depends on the context. If you're certain that the values you're storing in sessions exist, then no. But that depends on what happened further up the script. Generally, you'll have your success condition code inside a if-else anyway, so for the success condition to fire, everything would need to be correct up to that point. If you're bringing these values into your script via sessions, then yes, you'll have to check them before using them. Isn't that the point of error checking? To stop the entire operation and alert others when something bad happens? Error checking is tedious. It's also necessary. An overabundance of caution is preferable to the alternative.
-
So long as your PHP is expecting values sent via $_GET, yes. You may need to do more tweaking, depending on your needs (what URL your PHP function is at, etc.). http://api.jquery.com/jQuery.get/ If you're using Zend Framework, it may already be done for you (don't know...never used that particular framework). That said, .htaccess is outside the scope of this discussion.
-
Function that returns several values, how to OOP call it ?
KevinM1 replied to Dzherzinsky's topic in PHP Coding Help
Objects can contain other objects, which is known as composition, and is generally a better way to go in most cases than inheritance. Objects can be passed as parameters in methods. Objects can invoke another object's methods if they're public and in scope. Static methods exist, which are class-based rather than instance-based. -
Function that returns several values, how to OOP call it ?
KevinM1 replied to Dzherzinsky's topic in PHP Coding Help
Whoa, timeout. 'global' should never be used in any context, and it's especially egregious in OOP. It ties your object/method/function/whatever to the context in which it's used, which is exactly what you don't want to do. The whole idea behind OOP is to make modular code. Code cannot be modular if it's bound to a particular context. Second, in most cases, you don't want your fields to be public. Methods? Sure. But your fields should be protected or private, depending on whether or not you expect to extend your class. There are exceptions, but private/protected fields should be your default setting. Third, custom constructors, like SnapGravy said, aren't mandatory. A constructor is always invoked when an object is instantiated, as PHP (and, really, most/all languages with objects) have a default constructor which handles instantiation. When you write a custom constructor, your code is executed in addition to the default constructor. So, with all that said, can you actually show the class code you're having trouble with in its entirety? I can't tell what specific problem you're having with your code as your examples seem canned and/or haphazard. Seeing the complete picture of what you're trying to do will help me help you. -
Where did he say anything about a form? He's using an ajax call to retrieve data from his PHP script. It's not necessary to use a form to do it. I will say, @cerberus478, if all you're doing is retrieving info from the back end, use GET instead. That way, if, for some reason, JavaScript isn't available to your user, they can still access the data via query string. That, and it's semantically correct. GET = retrieving data. POST = updating data. No it doesn't. MVC frameworks are all about clean URLs. It's a simple matter of a .htaccess. Like he said, he's using Zend.
-
Not necessary if there's only one statement in a clause, as is the case here. He did forget to quote his output in the first clause. That said there's no telling exactly what he wants solved as his question sucked.
-
Reread what I wrote.
-
Howabout giving us your definition of 'working' along with more than a handful of lines of code that is bereft of context?
-
Function that returns several values, how to OOP call it ?
KevinM1 replied to Dzherzinsky's topic in PHP Coding Help
You should be able to both pass in and return an array to a method. How were you attempting to do it? -
Or: if ($weblink !== false){ // do stuff }
-
@rosslad2011, mjdamato was right on in his assessment of your design. Your back end code should be complete without having to rely on JavaScript to do something. JavaScript should be used as a skin on top of your system to add user interaction elements. How you really should be approaching it, if you absolutely need to include/not include something based on JavaScript availability, is to handle the non-JavaScript state as the default, then handle the JavaScript exists state as the part to code around, which is exactly the opposite direction you're currently going in. All that said, your question raises red flags about your overall design. It's hard to say for sure without seeing code, but my inkling is that you're doing it wrong.
-
It looks like a scope issue to me. Just because you invoke the bootstrap's header method in your league controller doesn't mean that the bootstrap will automatically have access to the controller's members. You need to pass in the view to the method, just like you would with a function in a procedural context. Remember: in PHP methods are functions. Treat them accordingly. Scope still applies.