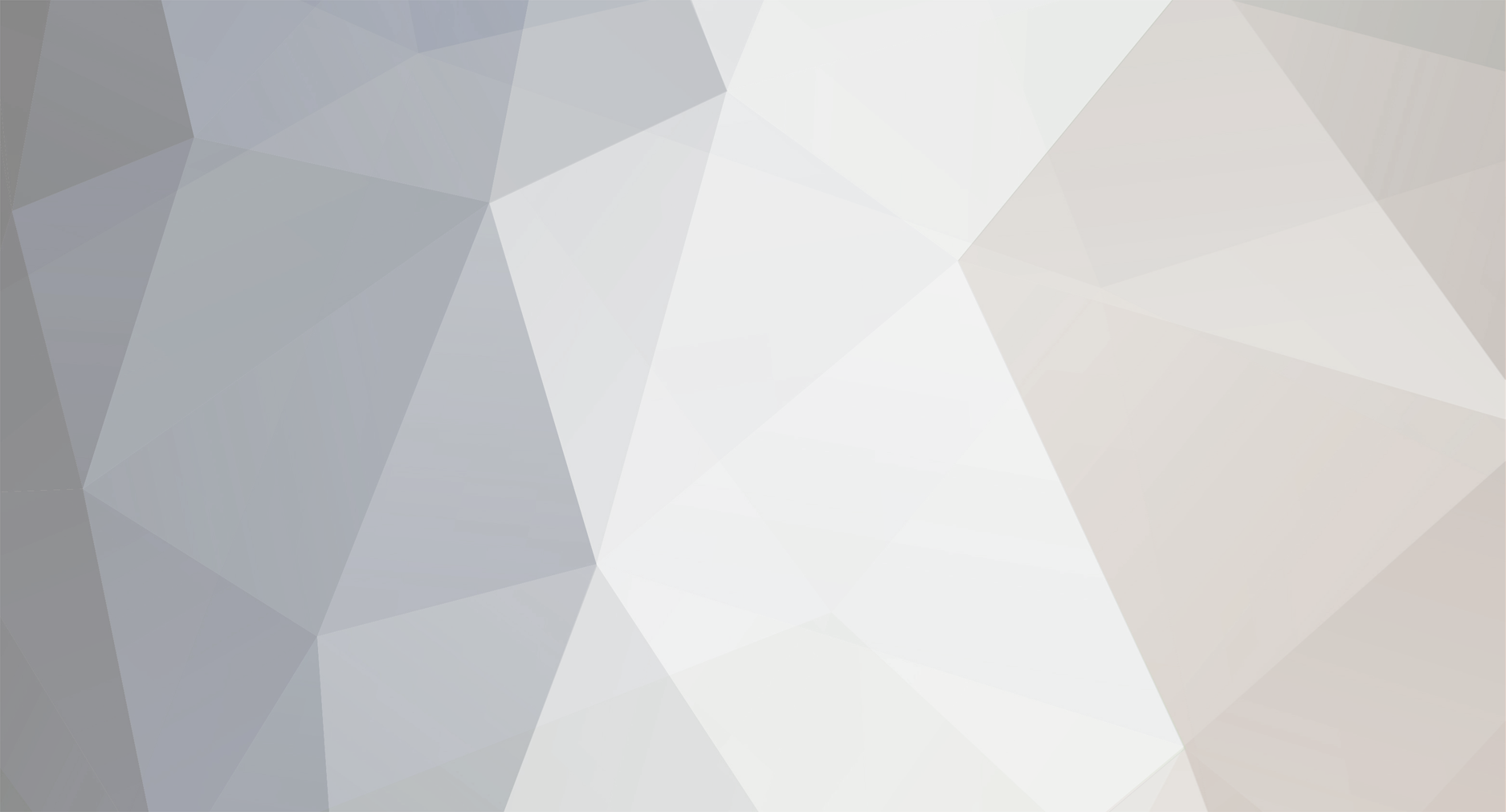
KevinM1
-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Posts posted by KevinM1
-
-
...okay.
1. JavaScript cannot invoke a PHP function unless you use AJAX. PHP runs first on the server, and is done running once the page is rendered. Even if you're using PHP to output your JavaScript.
2. You should never, ever, ever use 'global' in your PHP code.
3. When posting code on these forums, please use the code BBCode tags. You can do it manually, by placing
around your code, or by pressing the <> button in the editor.
4. We don't generally look at attachments here. So, if you could highlight just the parts that aren't working (your JS function, where you're invoking it, your PHP), that would help. And, please keep it to just the relevant bits.
-
how to become a guru ?
Be active, be helpful, be respectful. Gurus are selected by the staff, so if you're a member of this community that contributes in a positive way, you'll likely get noticed and promoted.
-
The error is pretty clear. Your base class' method has a different signature than the child class' method. Specifically, their arguments (or lack thereof).
-
Yup. No matter how you do it, user data will be sent to the server. Code defensively, and you should be fine.
-
die() is a built-in PHP function that kills script execution. You stop your script from executing right there.
-
Oh, you actually wrote your code without indents? Yeah, that's a problem. Not with the code itself, but with our ability to read it and deduce the problem you're having. Generally speaking, { } denotes a block of code. Whatever is between them should be indented (usually one tab/4 spaces). So, it should be:
if (/* something */) { // code // code //code } else { // more code // more code // more code } while (/* something else */) { if (/* another thing */) { // code // code } else { // more code } } if (/* blah */) { if (/* foo */ ) { // code } } else { // more code }
See how it makes it easier to read? We'll need you to do that before going any further. It may seem trivial (and like a PITA), but since code is a visual medium, it makes a lot of difference.
-
Can you do us a favor? Can you repost your code within
[/nobbc] tags? You can create those tags either by clicking on the <> at the top of the text editor, or by manually writing them. So, when you have something written as:[nobbc]
// stuff
It'll actually look like:
// stuff
It'll likely help with indentation, as your code currently has none, making it difficult to see where conditionals and loops begin and end.
Thanks.
-
Hi guys!!!
I am from Panamá.
I would like to buy a tablet cause smartphones are too short ...I would like to read some developer and linux ebooks or tutorial; that's why I would like to get a tablet. Is there any tablet out there that you can install like at least maybe notepad++ or any good editor for javascript or php?????????
Thanks you!
I know that iPads have a PHP app available that (supposedly) provides a simple environment to write simple scripts. There may be a JS app, too. I haven't tried them myself. That said, iPads don't give file system access, so they're very limited.
Androids, out of the box, don't allow file system access either, but you can probably find some sort of jail broken firmware that will help along those lines.
The simplest would be a Windows 8 tablet. The RT versions are limited to just the Metro interface, but the Pros are full Windows 8 computers. Slap VirtualBox on it with whatever *nix distro you like, and you have a portable development machine.
-
@OP, you'll need to provide your affiliates a unique URL that you'll be able to verify their status with. I'm not entirely sure what the best method is - I've never had to deal with affiliates - but the idea is that your script will be on the lookout for a certain kind of request (likely something unique through GET to keep things easy for your affiliates) that it will be able to parse, then for the duration of that user's session they're tied to the affiliate.
-
Check $_SERVER['HTTP_REFERER']
$_SERVER['HTTP_REFERER'] is not guaranteed to be set, or set with the right data. Says so right in the specs. Plus it's easily spoofable.
-
Lets say I want to use "dependency injection" for my MySQLDatabase class... Do i do it like this?
public function __construct() { $database = new MySQLDatabase(); }
No, you need to pass in a database object as an argument to your constructor:
class MyObject { private $db; public function __construct($db) { $this->db = $db; } // other class stuff } $database = new MySQLDatabase(); $myObj = new MyObject($database);
Functions and methods have several parts to them:
1. Their name
2. Their argument list
3. Their return type(s)
Put all together, they represent that function's or method's signature. That signature is important, because it tells you how that function/method works at a glance. More importantly, it infers a kind of contract between it and the rest of your code. "I will only work if you call me by name, pass in these kinds of values. In return, I'll give you this other kind of value." Functions/methods are supposed to be black boxes to the code that invokes them.
'global' breaks that contract by introducing an implicit requirement, one that doesn't follow the normal rules of scoping. In order for a function/method with 'global' to work correctly, the code that invokes the function/method needs to know of that hidden requirement. That makes them not black boxes, and instead leads to hard-to-change spaghetti code. Since functions and, even more to the point, OOP are all about reusability and modularity, you're writing code that undoes the entire point of it all.
So, 'global' is bad no matter how you slice it. Any resource that uses 'global' in its code should be considered a bad resource.
---
With dependency injection, you're simply composing objects at construction time (usually). If an object requires another object to work, simply make that dependency a member of the object that uses it. You get all the benefits and none of the restrictions of inheritance or (even worse) singletons (which are a special form of globals, and should be avoided at all costs). Your code will be easier to maintain.
-
mac_gyver.
Thanks for the reply. I changed the code by adding the following method in my database class
public function affected_rows() { return $this->_connection->affected_rows; }
Then I changed the following on my other class that has the update methodpublic function affected_rows() { return $this->_connection->affected_rows; }
Does this looks correct?Also I don't know what you mean by "didn't you fix this by brining it into the classes as a call time parameter when you created the instance of your classes?"
Please explain. Thanks
He means that you need to remove 'global' and instead pass in the database as an argument in your constructor. More to the point, never, ever, EVER use 'global'. I know that Lynda and other tutorials use it, but it's bad practice, especially in an OOP context. You need to forget you ever saw it.
-
Not to mention that WordPress isn't exactly known for its security....
-
You should also look into PCI compliance.
-
Since $db is private in User, you can't access it directly (even if I was public, you'd need to use $bob->db->some_db(); ). Create a public method like:Hey Kevin thanks for that info. Def going to ditch the tutorials. I have trying using your code to access the database object but was having some troubles doing so. For instance I was trying to access the some_db() method through the user object. But it couldn't find the some_db() method...
I know the code may look silly, as I am getting the hang of how things work
class Database { public function some_db() { echo "This is some DB code"; }}class User{ private $db; public function __construct($db) { $this->db = $db; }}$myDB = new Database();$bob = new User($myDB);$bob->some_db();
public function echoDB() { $this->db->some_db(); }
-
Hey vinny42. I am sorry but can you please explain me what you mean by user handler or usermanager, and what do mean by "Don't extend database classes, use composition"? Sorry about all these questions I am kind of new to all this. Thanks!
"Composition" is a fancy way of saying that objects can contain other objects. Newbies tend to overuse inheritance because that's pretty much where tutorials begin and end when it comes to adding functionality to classes. The problem is that inheritance creates rigid hierarchies, and can lead to weird relationships.
Every class you make is a type. A type is both a kind of data and the actions that can be performed on that data. Integer is a type, which are whole numbers that can be acted on via arithmetic. String is a type, which are collections of characters that can be concatenated, counted, spliced, etc. Even though PHP is dynamically typed (a variable can hold anything), the idea of type is still important, especially in OO.
Inheritance creates what's known as is-a relationships. Meaning, a child object is also considered to be an instance of the parent class. That's why vinny and trq were questioning you having a User extend a Database - it doesn't make sense for a User to be a Database.
What you want to do is use a Database so a user can do what it needs to do. You do this by composing the User and Database, like so:
class Database { // db code } class User { private $db; public function __construct($db) { $this->db = $db; } } $myDB = new Database(); $bob = new User($myDB);
Boom, you now have a User that uses the Database.That kind of setup has a couple advantages:
1. You no longer have that weird, "A User is also a Database" relationship.
2. If written correctly, your User can use any kind of database.
3. It's cheap - objects are passed by reference by default in PHP 5+, so User doesn't have a full copy of the Database, but just a link to it.
4. Because of that, Singletons (which are bad anyway, as they're a form of global) are wholly unnecessary because you can create just one Database and then assign its reference to an infinite number of other objects.
AbraCadaver, I got this code from a tutorial I was following, but wanted to change it up a little bit. Just wanted to play around with it a little bit to get a feel of how things work.
Like I said in another thread, ditch the tutorials. Get the following books:
Tutorials on OOP generally suck. Yeah, books cost money, but these two are definitely worth the investment. There simply isn't a better way to get introduced to OOP or the ideas behind it than these two.
-
Global variables are especially bad in an OO setting where each object is supposed to represent a concrete entity with clearly defined boundaries. 'global' completely bypasses those boundaries, negating one of the fundamental tenets of OO. 'Static' fields and methods do the same, since they're called on a class rather than an object, and can therefore be invoked in any context/scope. There are certain scenarios where 'static' is appropriate, but usually people use it a lot like 'global' - a quick and lazy workaround for bad app design which has its own pitfalls. So, your code example has potentially two strikes against it.
You're trying to learn from Lynda.com, right? I never liked her/their tutorials. If you want to learn OOP the right way, get Zandstra's book, then read the Gang of Four's book. Those two resources will get you on the right track.
-
Vinny is correct - create your page with PHP. That will save you a bunch of headaches. Since PHP is executed first, you can detect cookies immediately and serve the correct content without JavaScript or redirects or the noscript tag.
Seriously, you're way overthinking this.
-
In short, never, ever, ever use 'global' for anything, regardless if you're writing OO or procedural code. And if you're using a resource (book, tutorial, video, etc.) that has 'global' in its code, consider that resource suspect.
'global' is a sign of doing it wrong.
-
What are you trying to do specifically?
-
Try putting session_start at the very top of the page that requires your functions. Also, never, ever, ever use 'global'. For anything. You're already using the function's argument list. Just pass $ss_con in through it like you do with the other arguments.
-
I would charge anything between 150 to 200 euros.
Wow, that's cheap.
To the OP, the best way to figure it out is to have an idea of how much your work is worth an hour. It doesn't matter what kind of work (e.g., PHP vs. HTML vs. creating a layout in an image editor). Just figure out how much you think is fair to yourself per hour. From there, estimate how long it will take you to complete the project. After you have a ballpark time estimate, add 50%-100% more time for debugging, installation, last minute tweaks the client wants, etc. Multiply your cost/hour * the number of hours (including that 50%-100% buffer I just described). That's the estimate you give your client.
The best part about doing it this way is that most of the time you'll likely finish the project under the amount of time you originally project (which is part of the reason why a large buffer works so well). If that happens, you have a choice - charge the client the full amount, or take off some/all of the unused buffer time from the bill, thereby making your client very happy.
I personally have a base fee of $500. Meaning, even if something is trivial, that's what I charge. Remember: if you're doing this as full-time employment, you need to be able to earn enough to not just cover hosting, domains, software, hardware, transportation to and from a client, phone, etc., but enough to live off of.
-
Good call on protected vs. public. The rest still stands. Remember: the OP wasn't asking about best practice, just whether something could work or not.
-
So I have an instance of UserXyzPersister which extends DBPersisterAbstract which extends DBPersisterBase.
There is a function in the DBPersisterBase class that is protected:
protected function getArray($arg){ ... }
I'm trying to access it via:$u = new UserXyzPersister(); $u->getArray($arg);
This is working. My question is, can only the children of DBPersisterBase access getArray() or can the parents as well?If something is declared public or protected, then only the children of that class gain access to it. Inheritance is a one-way street.
EDIT: and by children, I mean any class down the inheritance chain from the original. Try it yourself:
class A { protected function hello() { echo "Hello from class A!"; } } class B extends class A { protected function test() { echo "Hello from class B!"; } } class C extends class B {} $a = new A(); $c = new C(); $c->hello(); $a->test();
Site Structure
in PHP Coding Help
Posted · Edited by KevinM1
There's no real best way. There are conventions, but they're used mostly because they're convenient.
With MVC sites, you'll find views (page templates) split by controller. So, you'll have something like (with the filesystem):
So, you could have something like:
But, again, there's no right/correct/best answer aside from whatever makes the most sense to you, and is easy to use.