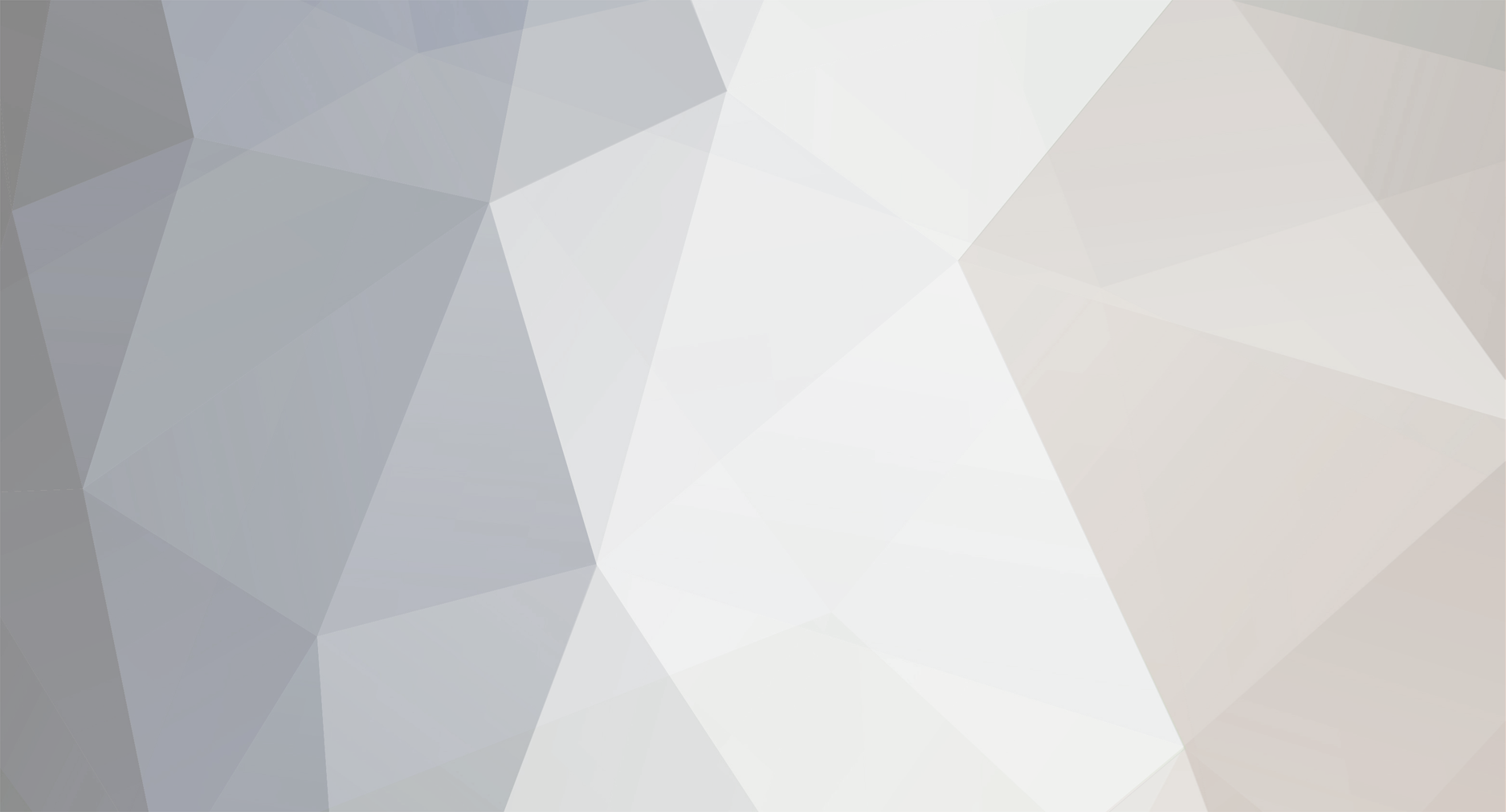
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
need foreach (in php terms) applied to this script
KevinM1 replied to sKunKbad's topic in Javascript Help
The problem is, there are many others in the actual implementation of the code, and I was hoping to make the code as small as possible. I'm not super good at javascript (obviously), so I wasn't aware that eval was evil. You can still loop through them all without hard coding the radio button names. Use document.getElementsByTagName('input') to get an array of all form inputs. Your idea of storing a white list of radio button names is a good idea. You can do something like: var allowed = new Array(); // populate it with the names of your radio buttons var radios = new Array(); // this is where all the valid buttons go for(var i = 0; i < inputs.length; ++i) { for(var j = 0; j < allowed.length; ++j) { if (inputs[i].name == allowed[j]) { radios.push(inputs[i]); } } } From there a simple for-loop over the radios array should do the trick. As a general tip, don't be afraid to use multiple loops in succession to filter and process elements. It may be more typing, but it's more efficient than nested loops, and has the benefit of clarity and readablity. -
need foreach (in php terms) applied to this script
KevinM1 replied to sKunKbad's topic in Javascript Help
You're on the right track, but your use of eval() should be a warning sign. As a rule of thumb, if you use eval(), you're doing it wrong. Basically, you need to loop through each set of radio buttons, and figure out which one is checked. A simple for-loop is all you need. var total = 0; var oAnother = document.forms['the_form'].elements['l_another']; for(var i = 0; i < oAnother.length; ++i) { if (oAnother[i].checked) { total += parseInt(oAnother[i].value); } } Repeat for the others. -
Here you go: <?php function clean($value) { if (is_array($value)) { foreach($value as $k => $v) { $value[$k] = clean($v); } } else { if(get_magic_quotes_gpc() == 1) { $value = stripslashes($value); } $value = trim(htmlspecialchars($value, ENT_QUOTES, "utf-8")); //convert input into friendly characters to stop XSS $value = mysql_real_escape_string($value); } return $value; } ?>
-
Ok, Arrays are starting to make my head spin now...
KevinM1 replied to soma56's topic in PHP Coding Help
The easiest way to do this would be with objects. Something like: class Lady { private $name; private $shoeSize; private $shoes = array(); public function __construct($name, $shoeSize) { $this->name = $name; $this->shoeSize = $shoeSize; } public function getName() { return $this->name; } public function getShoeSize() { return $this->shoeSize; } public function buyShoe(Shoe $shoe) { $this->shoes[] = $shoe; } } class Shoe { private $size; public function __ construct($size) { $this->size = $size; } public function getSize() { return $this->size; } } class Mall { private $shoes = array(); public function addShoe(Shoe $shoe) { $this->shoes[] = $shoe; } public function visit(Lady $lady) { foreach ($this->shoes as $shoe) { if ($shoe->getSize() == $lady->getShoeSize()) { $lady->buyShoe($shoe); } } } } $Dorothy = new Lady("Dorothy", ; // build our lady $mall = new Mall(); // generic mall for ($i = 0; $i < 20; ++$i) { $shoe = new Shoe(/* random size */); // build a random shoe $mall->addShoe($shoe); // add it to our mall } $mall->visit($Dorothy); // the mall is visited by Dorothy, who buys all shoes in her size Obviously, it's just a rough sketch, but this is how I'd approach it. -
OOP should be a long term goal. And, you're not going to learn OOP by merely reading syntax. Stuffing a bunch of similar functions in a class is not OOP. There's an art to writing good functions. Unfortunately, it can only come with practice. Just remember two things: functions are used to both reduce repeated code and to abstract code. You should strive to keep your main code as readable as possible. It should be free from as many bits of detail not directly related to whatever the main code should be doing as possible. Look at PHP's built-in functions - you probably don't care, and wouldn't want to see, what something like mysql_query or include looks like under the hood, or exactly how they do what they do. The same approach should be taken with custom functions. So, some key thoughts when writing a function: 1. What should it do? 2. What kind of information will it need to complete its task (argument list - NEVER use the 'global' keyword)? 3. What should it return once it finishes doing its job ('nothing' is a valid answer)? 4. How can I make it as abstract as possible, being useful in as many situations as possible?
-
formitem isn't a legit HTML element. Why not use a div to hold these? Also, what is 'elem' in your code? Where does it come from? For your function, why wouldn't: function takeOut(id) { var parent = document.getElementById('formitem' + id); // etc } Work?
-
Also, you seem to have some confusion regarding what a function's argument list actually is. The variables listed in an argument list are passed into the function. Your various result variables (e.g., $aboutResult) don't exist outside of the function, but are rather created within the function. Since they're not being passed into the function, they shouldn't be listed as an argument (and you'll probably get an error about passing an undefined variable into the function if you don't fix it). You should have functions that look like: function aboutMenu($about) { $aboutResults = mysql_query("SELECT * FROM $about"); $numAbout = mysql_num_fields($aboutResults); // continue processing, or return a value }
-
Discussion: Can truly professional brower apps be made with a JS library?
KevinM1 replied to haku's topic in Javascript Help
When used properly, frameworks enhance creativity. They free us from having to worry about the low-level stuff in order to concentrate on the code that actually does stuff. Of course, frameworks can be used as a crutch, but the same can be said of any programming aide. So long as you have a general idea of how the language works and what the framework is doing under the hood, I don't see a danger. jQuery in particular is nice in that in many cases one still needs to write some vanilla JS along side it. Regarding whether or not using 3rd party code is professional or 'good/right', I think every coder asks themselves whether or not using someone else's code somehow diminishes their work. There's an implicit question of trust buried there, too - can I trust this code that I did not write? This is actually a bit of psychology that has a name: Not Invented Here. The answer is that it's perfectly professional to use these tools. They're proven and increase productivity. It'd be unprofessional NOT to take advantage of things that can make your job easier. Also, do you play games? Ever notice how many use the Unreal Engine? Or the PhysX physics library? Same thing on a more complex scale. -
I understand. Clients/bosses can have tunnel vision. Have you scoped out your competition? See how their sites are organized? It might help drive home whatever changes you suggest to your boss(es).
-
The home page suffers from a lack of focus. The site name refers to signs, but the first two sections are the store and website design. The flash animation doesn't lend any clarity to it, either. Your flash animation would be better served showing examples of the signage, both for the big companies and smaller, in-stock signs. Without seeing any examples up front, why would I want to buy anything? Remember: users, above all else, are lazy and skeptical. They don't want a bunch of marketing spiel with nothing to back it up, and they certainly don't want to dig through your site to find examples of your work (btw: the gallery loads very slowly). You claim to use state of the art technology? So doesn't everyone else. Where's the proof? Some other small things: Embrodiaworld - what is it, why is it the first link on the site, why should I care? The contact link looks out of place to me. It shouldn't look like a navigation heading (non-clickable). What's with the red band across the top? Odd to see the content of a site moved down 10px or so for no good reason. There's a real solid base here, but it doesn't come together. You need to think about your own branding (who you are, what you do, what you sell), and realize that if your home page doesn't entice users, they're not going to bother staying.
-
That's actually defining a variable. Declaring a variable: simply writing a variable name to be used later ($var;). Defining a variable: declaring a variable and assigning a value/initializing it in one step ($var = "value"; ). Variables cannot be used unless they contain a value.
-
<? } ?> Should be <?php } ?>
-
After digging through the fine print, there is no mail functionality with my flavor of shared hosting. D'oh!
-
Have you tested to see if $search_words is being set correctly? That you're successfully retrieving the $_GET data?
-
I don't have access to the master, so I'm not sure.
-
I have the following quick and dirty contact form script: <?php ini_set('display_errors', 1); error_reporting(E_ALL); if (isset($_POST['submit'])) { if (isset($_POST['name']) && !empty($_POST['name'])) { if (preg_match("/^[A-Z\-' ]+$/i", $_POST['name'])) { $name = $_POST['name']; } else { $name = false; } } else { $name = false; } if (isset($_POST['email']) && !empty($_POST['email'])) { if (preg_match("/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i", $_POST['email'])) { $email = $_POST['email']; } else { $email = false; } } else { $email = false; } $message = htmlentities(trim($_POST['message']), ENT_QUOTES, "utf-8"); $message = "Email Address of user: $email<br /><br />Message:<br />$message"; $headers = 'From: mail@majorproductionsnh.com' . "\r\n" . 'Reply-To: mail@majorproductionsnh.com' . "\r\n"; if ($name && $email) { if (mail("*******", "Major Productions Inquery From $email", $message, $headers)) { header("Location: success.html"); exit; } else { header("Location: failure.html"); exit; } } else { header("Location: failure.html"); exit; } } else { header("Location: home.html"); exit; } ?> For some reason, mail is failing. Error reporting shows nothing when I remove the header redirects. Anything jump out as being wrong? The headers, perhaps? I'm thinking it's something with the shared hosting I'm on, but want to verify I didn't do something dumb before submitting a ticket to them.
-
Your problem is that you're confusing GET with POST. Looking at jQuery's ajax documentation, it's clear that the 'data' attribute is only supposed to be used with a GET request. This makes sense, as it appends the 'data' to the end of the destination script's URL as part of a query string. POST is done behind the scenes - no URL is used to pass data along. In short, you can't use 'data' with a POST because POST doesn't work the way you think it does. You'll need to rethink your design.
-
Not an entirely different db, but a neighborhoods table, yes. You'd need something like: City Table: id name 1 Someplace 2 Someplace Else . . . Neighborhood Table: id city_id name 1 4 Some Neighborhood in City 4 2 4 Another Neighborhood in City 4 3 11 Some Neighborhood in City 11 4 2 Some Neighborhood in City 2 . . . There's a one-to-many relationship here: one city contains many neighborhoods. In order to model that in the db, you need your neighborhoods to know which city they reside in. This is done by adding the proper city id to their row of information. The city id acts as a foreign key.
-
I just ran into issues using trim on a value within a conditional in another project. Try removing it.
-
The bare bones simplest way to do it is with straight JavaScript and CSS manipulation. Build all of your selects, but set the neighborhoods with display: none. Put an event handler on the city select that will change its corresponding neighborhood select so it has display: block. The more elegant solution would be to us ajax to populate the neighborhood select based on the value of the city select. The structure of this would be similar to that of the first solution, but the devil is in the details.
-
Why should you not use Global $variable; ?
KevinM1 replied to TeddyKiller's topic in PHP Coding Help
Like I've stated many times to others, functions/methods have argument lists for a reason. ToonMariner got part of the global problem right - a global variable is a single point of failure in one's code. If the value stored in that variable is overwritten, that change leads to a cascade of errors, many of which may be difficult to find in a complex project. On the flip side of that is considering what a function/method signature does - it tells the person using it what data it needs to perform (its argument list) and what, if anything, it returns. Globals obfuscate this communication because they're never present in the argument list. If you're using someone else's code (like a framework), how would you know if a function/method needed a global variable to work? You wouldn't. So, not only is overwriting the value a possibility, never knowing that a certain value is required for a function/method to work is another. Like Mchl, a singleton may be the right way to go. A registry is essentially a specialized singleton. Do yourself a favor and buy the following book: PHP Objects, Patterns, and Practice 3rd Edition. It's essentially the tome of OOP for PHP. -
You're on the right track. What you need to do is normalize your tables before you go any further. This will aid in your design and help remove insert/delete anomalies. See: http://dev.mysql.com/tech-resources/articles/intro-to-normalization.html To skip ahead a bit, your questions and answers tables will need two id columns - one for themselves, which would be auto incremented like you originally thought, and one that contains the product id, which you'd obtain with mysql_insert_id. You may need an extra id column in either questions or answers to link each question with its appropriate answer, as well, but that's dependent on what your design needs are (might instead need to create a pivot table). In any event, this will link your questions and answers to a specific product.