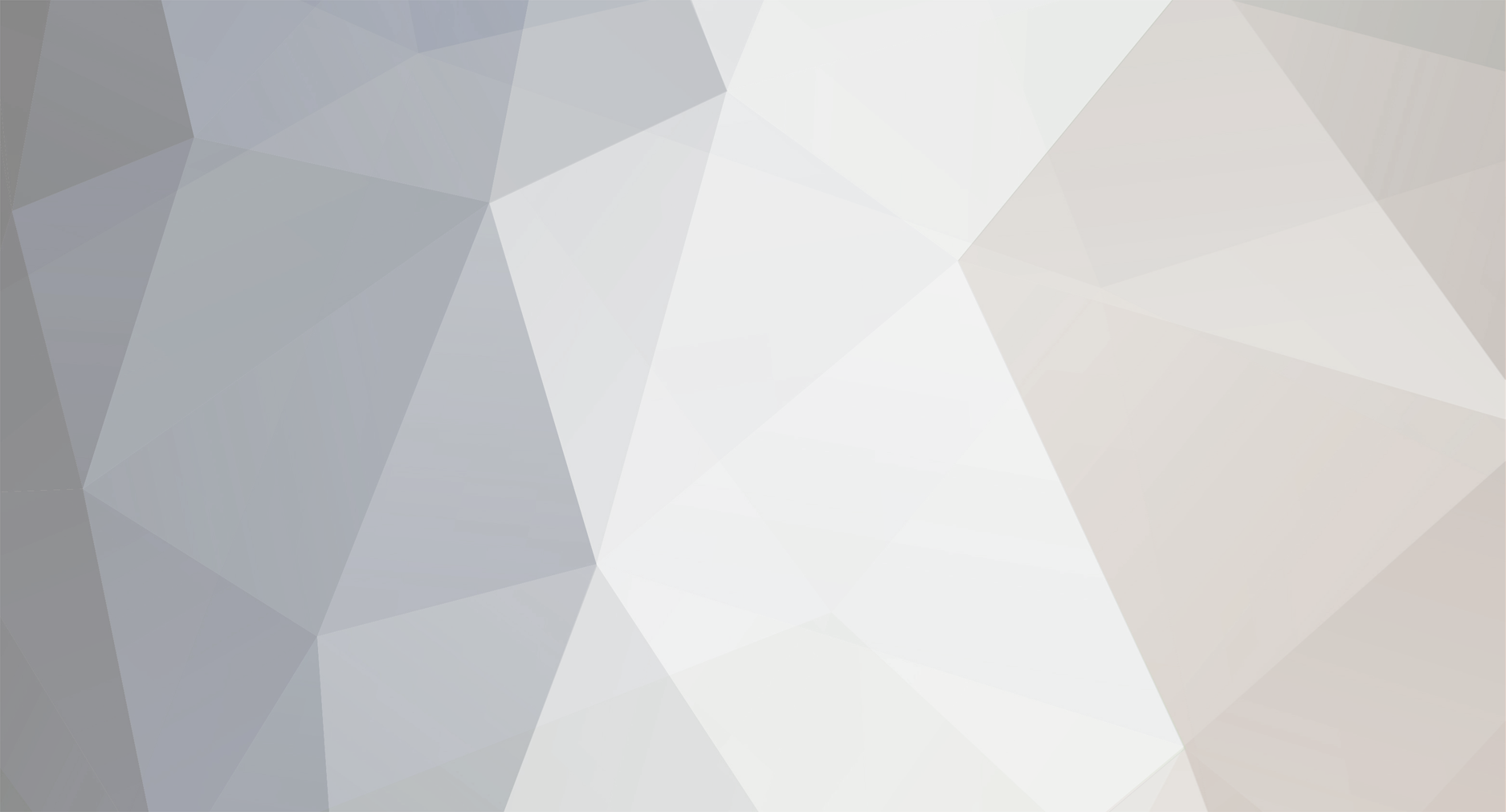
aleX_hill
Members-
Posts
143 -
Joined
-
Last visited
Never
Everything posted by aleX_hill
-
Displaying image based on filename stored in MYSQL DB
aleX_hill replied to ReeceSayer's topic in PHP Coding Help
Take a look at these two lines: $query2 = "UPDATE users SET avatar = '$id.jpg' WHERE username = '{$_SESSION['username']}'"; echo "<img src='../avatars/'" . $_SESSION['username'] . "'.jpeg' width='526' height='45' />"; Notice something different with the extensions? I see a mystery "e" appearing in there. Check your file system to make sure the file actually exists, and whether it should have the .jpg or .jpeg extension -
The way I would do this (and it probably isnt the best way) is: When the user first adds something to the cart, set $_SESSION['mysession'] to a random string based on the time: $random_hash = md5(date('r', time())); $_SESSION['mysession'] = $random_hash; This $random_hash would also be inserted into the database holding the shopping cart. The database would probably look similar to: session - the $random_hash item - the id of the item the user has added to the cart other stuff here Then the view cart page would run a query to get the ids of all the items linked to the $_SESSION['mysession'] value in the database. You will need to run session_start(); on ALL pages where you use a $_SESSION variable, and this must be called before anything is sent to the browser. Make a habit of making it the first line in each file you need it in. A cronjob to periodically clear any items put in the database more then x time ago (ie 12 hours) would be a good idea to stop the db getting exponentially large
-
I have a mailing list which I am developing which allows users to sign up to any (or multiple) of three lists. The send email form looks like this: <h2>SEND EMAIL TO LIST</h2> <form action="/mailingList/submitMail/" method="post"> Which Lists:<br /> <input type="checkbox" id="list1" name="list1" value="1" /> List 1<br /> <input type="checkbox" id="list2" name="list2" value="1" /> List 2<br /> <input type="checkbox" id="list3" name="list3" value="1" /> List 3<br /> Enter Email:<br /> <textarea name="email" cols="50" rows="5"></textarea> <button type="submit"> Send Email </button> </form> Now My database is a very simple structure with the following fields: id - int Name - varchar Email - varchar list1 - bool list2 - bool list3 - bool What I am stuck on is the best way to develop my SQL query based on the $_POST data. I dont want to use a whole bunch of if/switch statements and write a different query for each, and I am sure there is an easier way to do it then that. Any suggestions?
-
That was easy... Thanks
-
I have the following structure: id: autoincrement title email When I submit a title/email pair I want to get the auto-generated id. What is the best way of doing this?
-
page.php has an iframe with source upload.php, which loads a flash object to handle uploads. this then calls uploadify.php with the $_FILES details. I could get the session vars into the iframe fine, but just not into uploadify.php . The same would have happened whether upload.php was in an iframe or standalone.
-
A couple of things. 1. is there a reason you mix mysqli_fetch_array and mysql_fetch_array in the last file? 2. Try making the query mysql_fetch_array($result, MYSQL_ASSOC) or use mysql_fetch_assoc 3. Does your testing code print out the correct values?
-
OK, I figured this one out. Because uploadify.php is called by flash, session and cookie data arent transferred. I found a way to transmit an encoded session id through to uploadify.php where it is decrypted and set as the active session.
-
To make it a little more clear, you are missing the ' ' around the value, so it should read 'jim.bob' (as read from the session var.
-
That may do the trick. I dont particularly want to just use encode and decode, because if someone sees the encoded part in the source, then chucks it into the decode function, they have the data. I am looking at somehow appending a random string of characters to my decoded string. But seeing as it is a filepath I want to encode, if someone decodes it and sees "insoknf3/home/my/file/path/knsokfn4334" it might be a little obvious.
-
Following my problem at http://www.phpfreaks.com/forums/index.php/topic,288871.0.html I am trying to take a different approach. Is there a function to encode some data with a key, which can then be shown in the source code, and then decode that in another php file, using the key to decode it? Obviously md5 wont allow the decode for this.
-
Is there a reason that you need to write to the page before you process the if statement. I would put the processing part of the file at the top, and put the displaying part at the bottom. You can use a header() function anywhere as long as you havent written anything to the page first. Doesnt need to be at the top if it is only php processing before it.
-
Personally I would do it this way from AddStuff.php if(isset($_POST['variable'])) //If there is a POST variable sent, process the data. Change variable to the id of your submit button { //Do your validation stuff here } else { //Nothing submitted, show the form ?> <html> <head> etc. <?php } //Close the else ?> To make it a bit more complex, put the drawing of the form in a function like so: function drawForm($errorMessage) { //HTML for form here } That way, if you get an error in validation in the PHP you can send through an error message to the form when you create it.
-
Grrrr, pulling my hair out here, nothing seems to want to put the session var into the script. Think I might need to take a break for a few hours and look with fresh eyes.
-
I agree, hence it being a bare bones solution. While uploading with a random filename is a viable solution, if it matters what your file is called (ie if your users download them and you dont want them downloading nj44knkln.swf ) then take a look at the file_exists() function, and if it does, stop the uploading.
-
Something like this: upload the video to videos/videoname.swf (lets say its a flash video for the moment) insert into the db an entry with the date, and "videoname.swf" like so: $date = date(); $filename = $_FILES['uploadField']['name']; mysql_query("INSERT INTO videos (date, file) VALUES ('$filename', '$date')"); Then to display, try something like: $result = mysql_query("SELECT file FROM files SORT BY date ASC"); while($row = mysql_fetch_assoc($result)) { echo $row['file']; } Obviously basics here, will probably want a more details mysql table, and convert the echo row to something a little more useful, but you get the idea.
-
If I understand correctly, then No, there is no way for PHP to do this. PHP is a server based language which parses a page as it is created. What you are trying to do is more client based (ie get the code that this website has given me, and stick it into this other website i am accessing). I doubt there would be a way in js either, but I am not a js expert.
-
I have to agree. I never store files in the mysql database, but instead store it in the filesystem and reference its location in the database.
-
Php View all Members but not Editing or Deleting?
aleX_hill replied to gkishorekumarg's topic in PHP Coding Help
Firstly, and a little off topic, look into using CSS to style your table cells rather then the way you are doing it now. Secondly, without seeing your editform.php and delete.php files, we cant help you. delete.php would look something like: include(connection.php); $query = "DELETE FROM star WHERE id='$_GET[id]'"; $result = mysql_query($query); if($result) echo "Deleted"; else echo "Not Deleted"; the editform.php would look similar to your add user form, but with some sql at the top and a few value="<?= $array['id'] ?>" in the form fields. -
Not really that setup. The site is a CMS which connects to a different db depending on the login, used by a couple of primary schools in my area. So when they hit the login page, they choose their school from the drop down, then enter their username/password. This way I know which db to connect to so that I can properly authenticate them. The session vars also get used to connect to the db each time I run a query. So same source code for the cms for all schools, except it is operating on a different db. If I need to add a school, my thoughts were just to duplicate the profiles/schoolname.php file and put in the new db details, but after this thread I will probably change the way I am doing things. Either way, I want to be able to read the session vars in the uploadify.php file rather then pass them through as a parameter to the iframe, so people cant just call the upload script, pass in a "user_id" or similar and connect to the db. (ie <iframe src="uploadScript.php?user_id=2"> ) I will keep working on it and see what I come up with.
-
Where To Start In PHP? I Think I Am Crazy
aleX_hill replied to gobbly2100's topic in PHP Coding Help
To me the "main" function of PHP is reading/writing to a database. In other words, use it to store some stuff, edit some stuff and delete some stuff (very elementary, but hey you are still learning, just like me). Take a look at: http://www.freewebmasterhelp.com/tutorials/php Then google for a simple user management system tutorial and try this. The main parts would be add user form, login form and check user code. Then extend it to allow you to delete and edit the users in the database. It might seem superfluous at the moment, but once you have what I mentioned above under your belt you can make MOST things in PHP (well the things I use anyway). It might not be pretty code, or the most efficient (ie using classes) but you can do it. Also, make sure you have a pretty good handle on HTML first. -
Are you missing some code here? There is an echo statement with nothing after it, and no </div> or anywhere to excecute the code. I am not sure as to whether it is possible or not.
-
I just get nothing in mysql. So if i use the session var, nothing is written to the db. If I use hard coded and run the query to put the $_SESSION var into the db, then I get a row added to the db, with the $title var going in fine, but the "file" item in the db row is blank. And the structure of setting the sesison vars is irrelevant at the moment. the main reason i did it this way was it was easier for me to troubleshoot at the beginning (there is only 3 profile pages). The login really asks for username, password and website (from select box). Each "website" has its own db, hence the different session vars. Like I said, i will change this in the future.
-
ohdang888: I will look into that in the future, still in a dev stage at the moment. The setup I use is: login form -> login.php which includes a file similar to "/profiles/$_POST['username'].php" - there are very few users so I dont have 500 profile files then header.php (on all site pages) calls sqlConnect.php which uses the $_SESSION vars to connect to the db. I might put a user_id into the session var then put a switch in the sqlConnect.php file. Either way, I will need to get the session var of the user id through to the uploadify.php file
-
That file is called in an iframe, so I havnet bothered putting <html> or <DOCTYPE> tags etc. The $_SESSION vars work well in the code I showed you and i get: 'folder' : 'my/path/to/downloads/', fine. But when I upload a file, this info is passed to uploadify.php (which I have put a cut down version below): <?php session_start(); $connection = mysql_connect($_SESSION['server'], $_SESSION['user'], $_SESSION['password']); mysql_select_db($_SESSION['database'], $connection); if (!empty($_FILES)) { $tempFile = $_FILES['Filedata']['tmp_name']; //$targetPath = $_SERVER['DOCUMENT_ROOT'] . $_REQUEST['folder'] . '/'; $targetPath = $_REQUEST['folder']; //$targetFile = str_replace('//','/',$targetPath) . $_FILES['Filedata']['name']; $targetFile = $targetPath . $_FILES['Filedata']['name']; // $fileTypes = str_replace('*.','',$_REQUEST['fileext']); // $fileTypes = str_replace(';','|',$fileTypes); // $typesArray = split('\|',$fileTypes); // $fileParts = pathinfo($_FILES['Filedata']['name']); // if (in_array($fileParts['extension'],$typesArray)) { // Uncomment the following line if you want to make the directory if it doesn't exist // mkdir(str_replace('//','/',$targetPath), 0755, true); //Check to see if I got my session vars through (I hard code values when using this bit) $title = $_FILES['Filedata']['name']; $file = $_FILES['Filedata']['name']; $query = "INSERT INTO photos (title, file) VALUES ('$title', '$_SESSION[db_server]')"; $result = mysql_query($query); move_uploaded_file($tempFile,$targetFile); echo $result; //nothing is ever echoed as it is called in the background // } else { // echo 'Invalid file type.'; // } } ?>