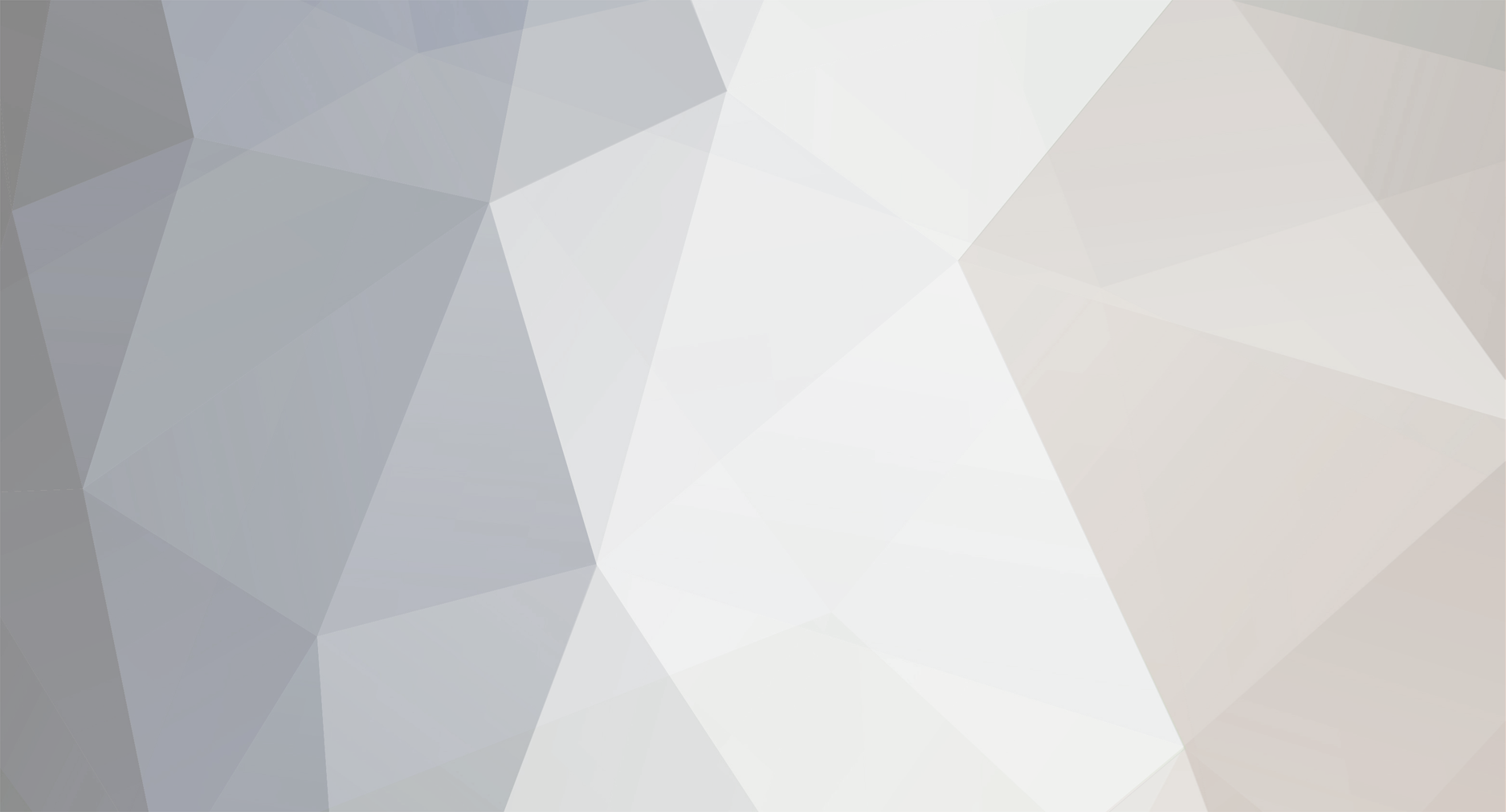
Cagecrawler
-
Posts
247 -
Joined
-
Last visited
Never
Posts posted by Cagecrawler
-
-
Try naming the class Default_Form_Login, keeping filename and place the same. If this doesn't work, you'll have to set up a specific autoloader.
If you're using Zend_Application_Module_Autoloader (as they do in the quickstart guide) then the example they provide should work (I haven't tested). Insert the following function into your bootstrap.
<?php protected function _initAutoload(){ $autoloader = new Zend_Application_Module_Autoloader(array( 'namespace' => 'Default_', 'basePath' => dirname(__FILE__), )); return $autoloader; }
I'm actually using Zend_Loader_Autoloader (for more complex things) - below is how I solve it.
<?php protected function _initAutoload(){ $resourceLoader = new Zend_Loader_Autoloader_Resource(array( 'basePath' => APPLICATION_PATH, //APPLICATION_PATH is is folder Bootstrap.php is in 'namespace' => 'Default', )); $resourceLoader->addResourceType('form','forms/','Form'); }
-
You can use output buffering to stop the html being sent before the header.
-
It's probably down to $maxnewsitems not returning a integer. Could be another problem with variable scope.
-
If they are limited to two teams, you could have 'team1_id' and 'team2_id'. Then use SQL something like this:
SELECT * FROM user WHERE team1_id = 10 AND team2_id = 15
Of course, only using two tables means adding an extra column if you want to allow them to be in another team, which should be avoided.
-
Use the html <meta> tag:
-
I'd use three tables in that case. A 'user' table for each player (could easily double up as storage for login info etc), a 'teams' table listing each team and it's info and a 'user_team' (or something to that effect) table which stores each user-team relationship.
Altering deadonarrival's table structure, remove team_id from the users table. Then create a third table ('user_team') with three columns - id, user_id and team_id. If a player is in more than one team, they have more than one entry in the table.
-
header("Location: " . $_SERVER['PHP_SELF']);
The header("Location:.... function instantly redirects the script to the target location - anything after this point is ignored by the script and so will not be printed. If you want to show the text for a little while and then redirect, you should use the html <meta> tag.
<?php echo "<META HTTP-EQUIV=\"Refresh\" CONTENT=\"5; URL=".$_SERVER['PHP_SELF']."\">"; ?>
You need to put this into the <head> tag of your page though, so it might not be appropriate.
-
Create a download page which calls the file you want and does a force download (ie. download.php?file=example.php). Make sure to include relevent checks to stop XSS. To force a download, I think its "Content-Disposition: attachment" is what's needed.
Quick google example: http://www.phpit.net/code/force-download/
-
explode('|',$array[0])
-
<?php $newvar = ($_POST['newvar'] == '')? NULL : $_POST['newvar']; ?>
-
It works fine for me...
-
Oops, I noticed that because some of the codes overlap (ari/rin/ri), some of the letters get mistaken. I've reversed the loop and all works fine now:
<?php function decode($message) { $letters = array('ka','tu','mi','te','ku','lu','ji','ri','ki','zu','me','ta','rin','to','mo','no','ke','shi','ari','chi','do','ru','mei','na','fu','zi',' '); $alphabet = array('a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z',' '); for($i = count($letters) -1;$i >= 0;$i--) { $message = eregi_replace($letters[$i],$i.".",$message); } $tmp = ""; foreach(explode('.',$message) as $t) { $tmp .= $alphabet[$t]; } return $tmp; } function encode($message) { $letters = array('ka','tu','mi','te','ku','lu','ji','ri','ki','zu','me','ta','rin','to','mo','no','ke','shi','ari','chi','do','ru','mei','na','fu','zi',' '); $alphabet = array('a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z',' '); for($i = count($letters) -1;$i >= 0;$i--) { $message = eregi_replace($alphabet[$i],$i.".",$message); } $tmp = ""; foreach(explode('.',$message) as $t) { $tmp .= $letters[$t]; } return $tmp; } ?>
-
I've used numbers as a transition phase to stop the decoder from altering parts that have already been decoded:
<?php function decode($message) { $letters = array('ka','tu','mi','te','ku','lu','ji','ri','ki','zu','me','ta','rin','to','mo','no','ke','shi','ari','chi','do','ru','mei','na','fu','zi',' '); $alphabet = array('a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u,','v','w','x','y','z',' '); for($i=0;$i < count($letters);$i++) { $message = eregi_replace($letters[$i],$i.'.',$message); } $tmp = ''; foreach(explode('.',$message) as $t) { $tmp .= $alphabet[$t]; } return $tmp; } function encode($message) { $letters = array('ka','tu','mi','te','ku','lu','ji','ri','ki','zu','me','ta','rin','to','mo','no','ke','shi','ari','chi','do','ru','mei','na','fu','zi',' '); $alphabet = array('a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u,','v','w','x','y','z',' '); for($i=0;$i < count($alphabet);$i++) { $message = eregi_replace($alphabet[$i],$i.'.',$message); } $tmp = ''; foreach(explode('.',$message) as $t) { $tmp .= $letters[$t]; } return $tmp; }
-
Use the MYSQL function NOW() to insert the current time.
INSERT INTO table('foo','bar','time') VALUES('foo','bar',NOW())
-
He's used a ternary operator to choose whether to use the SESSION array or create a new one. Using If else:
<?php if(isset($_SESSION['somename']) && is_array($_SESSION['somename'])) { $array = $_SESSION['somename']; } else { $array = array(); } ?>
-
UPDATE users SET activated = 1 WHERE activation_code=...
-
Are you using an unsigned integer for the points column in your db?
-
is the html code for a non-breaking space.
Normally HTML will truncate spaces in your text. If you write 10 spaces in your text HTML will remove 9 of them. To add spaces to your text' date=' use the character entity.[/quote'] -
html automatically shrinks a line of whitespace down to only one space. You need to do this:
<?php Echo' ';Echo$FuelLeft; ?>
-
In the error-handling code:
mysql__error()
should be:
mysql_error()
-
I use phpDesigner 2007 for projects and SciTE for quick edits/code.
-
phpDesigner 2007 has the option to print with line numbers. There is a free version for non-commercial use and the professional version costs €39/$55.
-
If you mean the bit between the body tags, then a straight include should work:
<body> <?php if($logged_in) { include("bodycontents.php"); } else { include("login.php"); } ?> </body>
-
PHP is a program like any other. When you request a php webpage, your webserver tells the PHP interpreter (can be called the runtime, ie. php.exe) to take the interpret the file you asked for and then gives the output to the client. When you run a cron, you tell the computer to start the php interpreter and bypass the browser completely.
There are three different interpreters, php (the main one), php-cgi and php-win (for windows machines). You can use php or php-win, I don't think it matters.
writing an IF statement for a form (if certain option from option box is chosen)
in PHP Coding Help
Posted
Try something like this:
The sql now only updates the fields where the data has been entered.