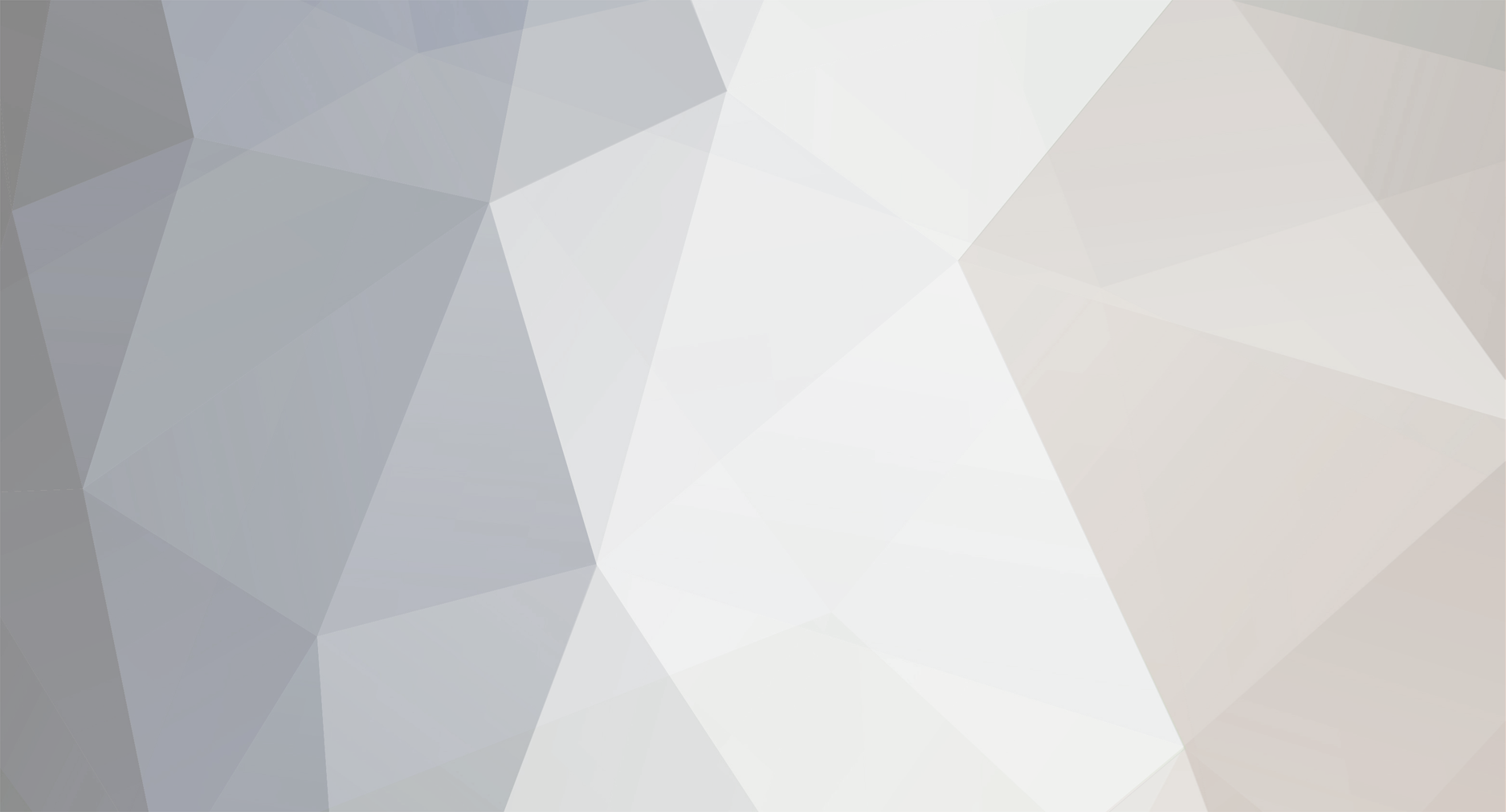
Cagecrawler
-
Posts
247 -
Joined
-
Last visited
Never
Posts posted by Cagecrawler
-
-
You don't, at least not in the normal way. Use fopen to turn the url into a stream, then read it's contents and write those into a file on your server.
-
My knowledge of flash is basically nonexistant but I believe you can make a call to a url and get the output? What you'd basically need to do is an AJAX call but using flash instead of javascript. Have your swf call the php script, every second maybe, and the script would output whatever you needed to know in a form you can then parse in flash.
You might want to look up something called AMFPHP - not sure what it does but I heard that it is good for working with Flash and PHP.
The tutorial below is for a Flash-based email form, but it gives you the basics:
http://www.kirupa.com/developer/actionscript/flash_php_email.htm
-
You can read about superglobals here:
http://uk3.php.net/manual/en/language.variables.predefined.php
-
That'll hit errors (you've used commas instead of periods). Try this:
while($row2=mysql_fetch_array($exec2,MYSQL_BOTH)) { echo'<tr>'; echo"<td width=100 align=center>".substr('$row2[datePosted]',0,10)."</td>"; }
-
In the UPDATE, $player_income should be $players_new_income, no?
-
mysql_query("SELECT columns FROM table WHERE column1 NOT IN (forum1,forum2,forum3)");
The section in the brackets can be passed as an array, just do this:
mysql_query("SELECT columns FROM table WHERE column1 NOT IN (".implode(",",$unwanted_forum_array).")");
-
The $_GET superglobal is used to pass variables across pages using the url. For example, if you had "index.php?foo=bar", then you could access that as "$_GET['foo'] = bar". $_POST is the superglobal mainly used for forms and $_REQUEST is sort of in between. I've never needed to use $_REQUEST.
-
$PercentageAddOn = $PercentageResults[$PercentageKey];
should be:
$PercentageResults = $PercentageAddOn[$PercentageKey];
Now, $PercentageResults would be equal to 1.32
-
You can do it 2 ways. Either limit the sql query to 20 results and have multiple queries, or have some sort of counter that increases each time the loop is executed. When it gets to 20 (or a multiple thereof), include the headings.
<?php $i = 0; while($array = mysql_fetch_array($query)) { if(fmod($i,20) == 0) { //echo heading } //echo current line } ?>
-
Simply encrypt the input at login and compare the hashes. It's probably easier to use a hash function such as md5 or sha1 for this. Simply execute the function, then compare.
<?php $password = sha1($_POST['password']); $id = mysql_real_escape_string($_POST['id']); $sql = "SELECT * FROM user WHERE id= '$id' and password = '$password'"; ?>
-
When dealing with directories on the server, you need to use \ instead of /.
$cwd = $cwd."/".$filedir."/";
should be
$cwd = $cwd."\\".$filedir."\\";
Also,
<form action="<?=$PHP_SELF?>" method="post">
should be (unless your server supports short tags):
<form action="<?php echo $_SERVER['PHP_SELF'];?>" method="post">
EDIT:
Your filedir isn't being passed to the second page. You can pass it via a hidden input in your form:
<input type="hidden" name="filedir" value="<?php echo $filedir;?>">
or add it as a GET:
<form action="<?php echo $_SERVER['PHP_SELF']."?value=$filedir";?>" method="post">
-
You need to create a table called buddies. Use the following SQL or use phpMyAdmin:
CREATE TABLE `dowmodsc_pico`.`buddies` ( `ID` INT NOT NULL AUTO_INCREMENT PRIMARY KEY , `userID` INT NOT NULL , `buddyID` INT NOT NULL ) ENGINE = InnoDB
You can then insert buddies using the following SQL query:
INSERT INTO buddies (userID,buddyID) VALUES ('1','2');
Where userID and buddyID relate to the relevant userid from the users table.
-
Where is $cwd being set?
-
WAMP doesn't set doc_root out of the box. You need to go into the php.ini file (Config files>php.ini on the WAMP menu) and search for doc_root (line 433). Set it to the doc root, usually "C:\wamp\www".
-
That doesn't have the function or the include in anywhere...
-
You can live without them, I was referring to the fact that the function is returning a value but you're not doing anything with it. You either need to echo it or assign it to a variable.
-
RemoveXSS() needs to be assigned to a variable.
ie.
$input = RemoveXSS($_REQUEST['input']);
-
-
<?php function loveLogged($admin) { $query = mysql_query("SELECT * FROM ". MEMBER_TBL ." WHERE `username` = '".$_SESSION['username']."' AND `password` ='".$_SESSION['password']."'")); if(mysql_num_rows($query > 0) { //User is logged in. if($admin == true) { //Test for admin $user = mysql_fetch_array($query); if($user['user_level'] = ADMIN) { //User is an admin return true; } else { //User isn't an admin. return false; } } else { //User doesn't need to be admin. return true; } } else { //User isn't logged in. return false; } } ?>
-
You want something like this:
<?php function adminCheck() { $query = mysql_query("SELECT * FROM ". MEMBER_TBL ." WHERE `username` = '".$_SESSION['username']."' AND `password` ='".$_SESSION['password']."'")); if(mysql_num_rows($query > 0)) { //User is logged in correctly $user = mysql_fetch_array($query); if($user['user_level'] = ADMIN) { //User is an admin. return true; } else { //User isn't admin. return false; } } else { //User isn't logged in correctly. return false; } } ?>
-
Yes, but that loads the whole file into the array to start off with, which is what the OP is currently doing and wants to avoid.
-
$password = $_SESSION['XXXXX]; // set the session password to a variable
User page: You're missing a ' here. Should be:
$password = $_SESSION['XXXXX']; // set the session password to a variable
-
Can't you use fgets()?
http://www.php.net/manual/en/function.fgets.php
<?php $handle = fopen("file.txt", "r"); $firstline = fgets($handle); echo $firstline; ?>
-
You're declaring session_start() more than once. This can happen if you have it in multiple files and then include them together in the same file. Either put it in a completely separate file and use require_once() to ensure it doesn't get repeated or only use it in the final file (ie. the one the user views).
[SOLVED] Upload from a Link?
in PHP Coding Help
Posted
You need to have allow_url_fopen set to true in your config. fopen and fread work with binary as well as text data, so the filetype doesn't matter.