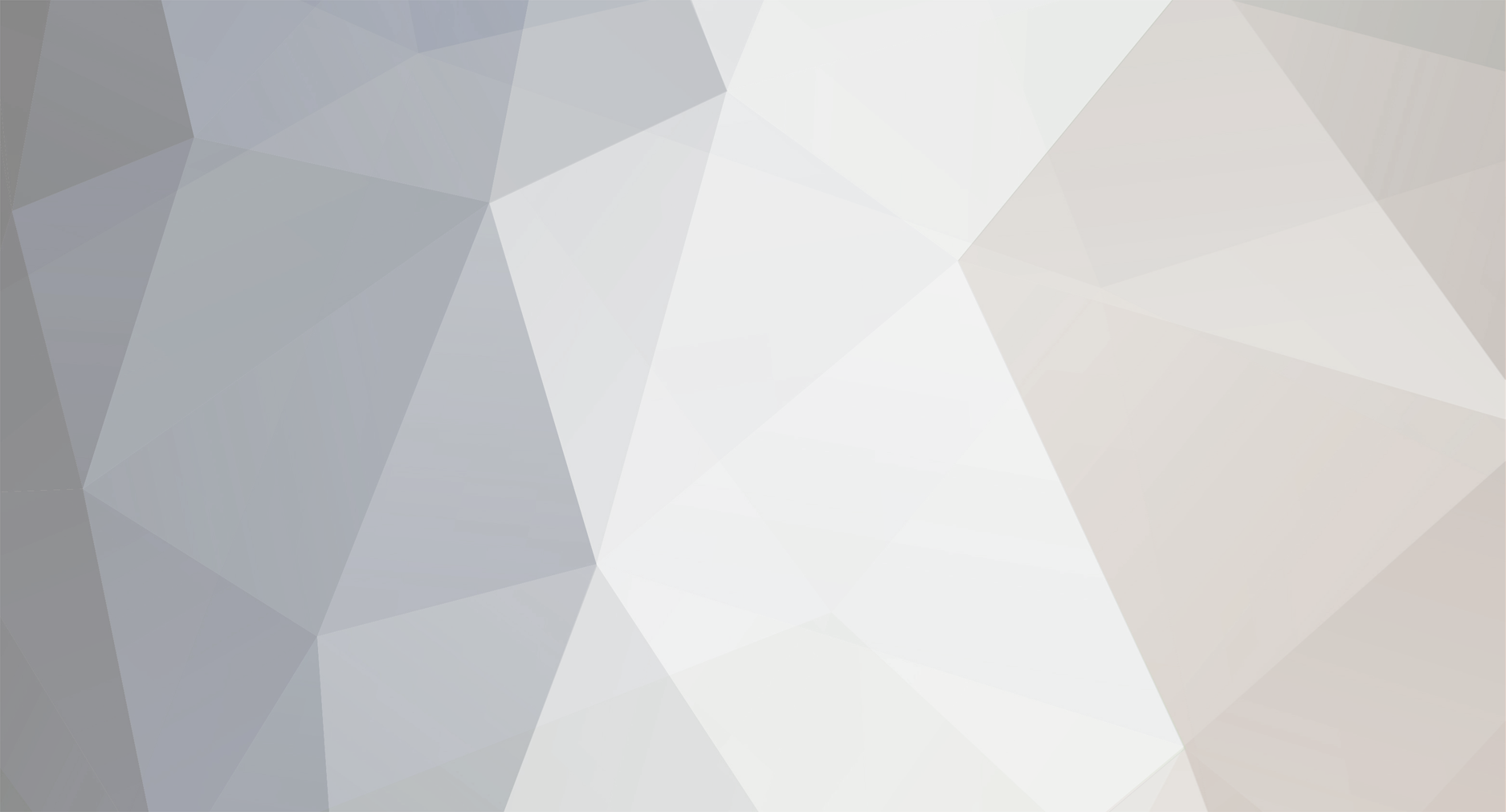
c_shelswell
Members-
Posts
275 -
Joined
-
Last visited
Never
Everything posted by c_shelswell
-
Great thanks very much - works a treat!
-
Hi still learning classes and I think there's a better way to do what i'm doing. I have a database class which logs on to db and does any queries I need. I'm also making a form class in a separate file when a user submits a registration it checks if the username is taken for this it needs the database class. I'm calling it like this: <?php class form { public function checkFields($postData) { /** check username available **/ $db = new db(); $data = array('fields'=>'username', 'table'=>'registered_users', 'where'=>'username="'.$postData['username'].'"'); $rtn = $db->selectFromDB($data); if ($rtn[0]->username > '') { $this->setError('username', '<br />This user name is already in use. Please choose another.'); } } ?> but can't I have something like <?php class form extends db{ public function checkFields($postData) { /** check username available **/ $data = array('fields'=>'username', 'table'=>'registered_users', 'where'=>'username="'.$postData['username'].'"'); $rtn = db::selectFromDB($data); } } ?> if i do it the 2nd way it seems to call the db class as I get a mysql access denied error so i guess it's not calling the database constructor? I don't think i'm fully grasping the extends concept I have been reading up on it but some help would be great. The start of my database class is below cheers: <?php class db { private $dbUser; private $dbName; private $dbPass; private $dbServer; private $connection; private $result; public function __construct($dbUser=DB_USER, $dbName=DB_NAME, $dbPass=DB_PASS, $dbServer=DB_SERVER) { $this->dbUser = $dbUser; $this->dbName = $dbName; $this->dbPass = $dbPass; $this->dbServer = $dbServer; $this->connect(); } private function connect() { $this->connection = mysql_pconnect($this->dbServer, $this->dbUser, $this->dbPass) or die (mysql_error()); mysql_select_db($this->dbName, $this->connection)or die(mysql_error()); } private function disconnect() { mysql_close($this->connection); } public function selectFromDB($data) { extract($data); $sql = 'SELECT '.$fields.' FROM '.$table; if(!empty($where)) { $sql .= ' WHERE '.$where; } if(!empty($groupBy)) { $sql .= ' GROUP BY '.$groupBy; } if(!empty($orderBy)) { $sql .= ' ORDER BY '.$orderBy; } if(!empty($limit)) { $sql .= ' LIMIT '.$limit; } $this->result = $this->query($sql); if($this->result) { $rows = $this->fetchRows(); } return $rows; } } ?>
-
Bit stuck with this database class - returning arrays
c_shelswell replied to c_shelswell's topic in PHP Coding Help
Just bumping this anyone got any ideas? Cheers -
Hi I'm still struggling to put together this database class that I found but am trying to learn how it works exactly. I'm basically grabbing some data from the db this all works great and i'm returned the array: Array ( [0] => stdClass Object ( [username] => cshelswell ) ) the class then sends that back to a function but that's when i'm getting: Warning: mysql_fetch_object(): supplied argument is not a valid MySQL result resource in C:\Program Files (x86)\Apache Software Foundation\Apache2.2\htdocs\a1\require\database_class.php on line 112 The function it's sending back to is: <?php public function selectFromDB($data) { /** have cut the above out the sql is good tho **/ $this->result = $this->query($sql); /** it's this bit it's getting stuck at **/ if($this->result) { $rows = $this->fetchRows(); } return $rows; } private function fetchRows() { $rows = array(); if($this->result) { while($row = mysql_fetch_object($this->result)) { $rows[] = $row; } } return $rows; } private function query($sql) { $this->result = mysql_query($sql) or die (mysql_error()); return $this->fetchRows(); } ?> I'm not to familiar with mysql_fetch_object i have looked it up and i think i get it but the class i have does as above so assume it did work for him. I guess i just need to separate the array so i only return: [username] => cshelswell Any help would be great cheers
-
[SOLVED] trying to make a database class but can't connect
c_shelswell replied to c_shelswell's topic in PHP Coding Help
Brilliant - thanks it's always the really simple things i've been looking at the page of code for ages and just couldn't see that!! Cheers -
[SOLVED] trying to make a database class but can't connect
c_shelswell replied to c_shelswell's topic in PHP Coding Help
Cheers PFM i'm getting Warning: mysql_query() [function.mysql-query]: Access denied for user 'ODBC'@'localhost' (using password: NO) in C:\Program Files (x86)\Apache Software Foundation\Apache2.2\htdocs\a1\require\database_class.php on line 121 Warning: mysql_query() [function.mysql-query]: A link to the server could not be established in C:\Program Files (x86)\Apache Software Foundation\Apache2.2\htdocs\a1\require\database_class.php on line 121 Access denied for user 'ODBC'@'localhost' (using password: NO) if i try and just connect from another page with the user and pass i'm providing the class it works fine. So I'm pretty sure it's something to do with my class. Cheers -
Hi I'm trying to learn a little about php classes and thought a database class might be a good idea as it's something most of my sites would use. I found a bit of code online and i'm trying to adapt it to work for me. Problem is I can't seem to connect to the database though I know my username and password is correct. I'm not too sure what i've done with the __construct bit does that automatically start? Also when i call the db class do i have to start with the connect function id $db->connect(); or can i just jump straight to $db->selectFromDB(); It would be good if i didn't have to connect every time, i'm trying to reduce the amount of code i have to write. My code's below if you could help that'd be great <?php include ("constants.php"); class db { private $dbUser; private $dbName; private $dbPass; private $dbServer; private $connection; private $result; public function __contsruct($dbUser=DB_USER, $dbName=DB_NAME, $dbPass=DB_PASS, $dbServer=DB_SERVER) { $this->dbUser = $dbUser; $this->dbName = $dbName; $this->dbPass = $dbPass; $this->dbServer = $dbServer; $this->connect(); } private function connect() { $this->connection = mysql_pconnect($this->dbServer, $this->dbUser, $this->dbPass) or die (mysql_error()); mysql_select_db($this->dbName, $this->connection)or die(mysql_error()); } private function disconnect() { mysql_close($this->connection); } public function selectFromDB($data) { extract($data); $sql = 'SELECT '.$fields.' FROM '.$table; if(!empty($where)) { $sql .= ' WHERE '.$where; } if(!empty($groupBy)) { $sql .= ' GROUP BY '.$group_by; } if(!empty($orderBy)) { $sql .= ' ORDER BY '.$order_by; } if(!empty($limit)) { $sql .= ' LIMIT '.$limit; } $this->result = $this->query($sql); if($this->result) { $rows = $this->fetchRows(); } return $rows; } private function fetchRows() { $rows = array(); if($this->result) { while($row = @mysql_fetch_object($this->result)) { $rows[] = $row; } } return $rows; } private function query($sql) { $this->result = mysql_query($sql) or die (mysql_error()); return $this->fetchRows(); } } ?> I'm calling it like: $db = new db(); $data = array('fields'=>'username', 'table'=>'registered_users', 'where'=>'username="'.$_POST['username'].'"'); $db->selectFromDB($data); Many thanks
-
[SOLVED] Generating delimited csv data with php
c_shelswell replied to c_shelswell's topic in PHP Coding Help
Cheers have eventually got it working! Thanks for all your help -
[SOLVED] Generating delimited csv data with php
c_shelswell replied to c_shelswell's topic in PHP Coding Help
Got the " on the data cheers PFMaBiSAd but I can't see where you mean the leading " on the header line? Cheers -
[SOLVED] Generating delimited csv data with php
c_shelswell replied to c_shelswell's topic in PHP Coding Help
Premiso -that's a great idea i now feel like an idiot for not even thinking of that!! Cheers. Seems they add double quotes round the data and if there's already a double quote in the data they quote that too ie "" so i think i'll use a str_replace on it. Cheers -
[SOLVED] Generating delimited csv data with php
c_shelswell replied to c_shelswell's topic in PHP Coding Help
no worries this is just one line from the database: (4, '[pro]To remove an item just click the ''REMOVE'' button. <br />This will remove one item only.', '[amt]Pour supprimer un article, cliquez sur le "Supprimer" bouton.<br /> Cela permettra d''éliminer un point seulement.', '[amt]So entfernen Sie einen Artikel klicken Sie einfach auf den "Entfernen"-Taste. <br />Dadurch wird nur ein Element.', '', '', '', '', '', '', '', '', '', '', '', '', '', '', '', '', '', 'show_cart.php', 'no'), You can see the 3rd record has a comma in the field. Each $row is one of these fields. Thanks again -
[SOLVED] Generating delimited csv data with php
c_shelswell replied to c_shelswell's topic in PHP Coding Help
Cheers but that still shifts data to the next column the first time it encounters a comma in the field. Surely I don't have to str_replace all commas in the data do i? Cheers -
Hi i'm having problem that i'm sure is very easy but i don't know the answer. I'm trying to create a csv file with php but some of the fields i'm creating contain commas already. I thought i had to add "$data", like that with the speech marks round it. But whatever i do it's not opening great in excel. My code is below: $q = "select * from main_site_languages limit 1"; $r = mysql_query($q) or die (mysql_error()); $headings = mysql_fetch_assoc($r); $res = mysql_query("select * from main_site_languages"); $csv = ""; foreach ($headings as $col => $v) { $csv .= strtoupper($col).","; $h[] = $col; } $csv = substr_replace($csv,"", -1); $csv .= "\n"; while ($row = mysql_fetch_array($res, MYSQL_ASSOC)) { $csv .= '"'.$row[$h[0]].'","'.$row[$h[1]].'","'.$row[$h[2]].'","'.$row[$h[3]].'","'.$row[$h[4]].'","'.$row[$h[5]].'","'.$row[$h[6]].'","' .$row[$h[7]].'","'.$row[$h[8]].'","'.$row[$h[9]].'","'.$row[$h[10]].'","'.$row[$h[11]].'","'.$row[$h[12]].'","'.$row[$h[13]].'","' .$row[$h[14]].'","'.$row[$h[15]].'","'.$row[$h[16]].'","'.$row[$h[17]].'","'.$row[$h[18]].'","'.$row[$h[19]].'","'.$row[$h[20]].'","' .$row[$h[21]].'","'.$row[$h[22]]."\n"; } header('Content-type: application/csv'); header('Content-Disposition: attachment; filename="main_site_languages_' . date("Y-m-d") . '.csv"'); print $csv; exit; any help would be great I'm racking my brains!!
-
[SOLVED] jquery - Stuck on a .toggle can't close the item again
c_shelswell replied to c_shelswell's topic in Javascript Help
Solved it - feel like a right idiot was looking at this for ages too! All i had to do was add the same class to the close button in the popup as the one that opened it. -
Hi I've got a page that's a calendar. Users can add events to each date. If there's an event then a marker shows on that date that a user can click on to see a jquery popup of the event. This all works great for multiple events on one page. The problem i'm having is closing the popup after it's open. I don't know if it's because i want to open it with one button but close it with a generic one on the popup. Any help would be great. My code is below thanks. <script type="text/javascript"> $(document).ready(function() { $('[class^=toggle-item]').hide(); $('[class^=link]').click(function() { var $this = $(this); var x = $this.attr("className"); $('.toggle-item-' + x).toggle(400); return false; }); $('.closeEvent').click(function() { var $this = $(this); var x = $this.attr("className"); $('.toggle-item-' + x).toggle(400); }); </script> <table border='0' width='455px' cellpadding='2' cellspacing='2' class='calendarTable'> <tr > <td class='calCellTop'>SUN</td> <td class='calCellTop'>MON</td> <td class='calCellTop'>TUE</td> <td class='calCellTop'>WED</td> <td class='calCellTop'>THU</td> <td class='calCellTop'>FRI</td> <td class='calCellTop'>SAT</td> </tr> <tr> <td class='calCell' valign='middle'><div class='eventMarker'><a href='#' class='link2'>x</a></div><div class='hiddenEvent toggle-item-link2'><a href='#' class='closeEvent'>close X</a><br />Start Time: 1233485100<br />End Time: 1233495900<br />Event: The event<br />Venue: the venue<br />Start Time: the details</div><a href='#' class='calLink addEvent'><span class='link'>1</span></a></td> <td class='calCell' valign='middle'><a href='#' class='calLink addEvent'><span class='link'>2</span></a></td> <td class='calCell' valign='middle'><a href='#' class='calLink addEvent'><span class='link'>3</span></a></td> <td class='calCell' valign='middle'><a href='#' class='calLink addEvent'><span class='link'>4</span></a></td> <td class='calCell' valign='middle'><a href='#' class='calLink addEvent'><span class='link'>5</span></a></td> <td class='calCell' valign='middle'><a href='#' class='calLink addEvent'><span class='link'>6</span></a></td> <td class='calCell' valign='middle'><a href='#' class='calLink addEvent'><span class='link'>7</span></a></td> </tr> </table> The line in the table with the 'closeEvent' tag is the one i'm hoping to close the div with. Hope this makes sense if not I could upload the site. Cheers
-
[SOLVED] checkdnsrr not working keeps failing domain
c_shelswell replied to c_shelswell's topic in PHP Coding Help
sorry just realised what i was doing should really put a .com at the end! -
Hi for some reason the below code keeps returning false not quite sure what i'm doing wrong. Any ideas would be great thanks. $mailDomain = 'hotmail'; if (checkdnsrr($mailDomain, "MX")) { return true; } else { return false; } unless there's a better way to test for badly typed domains. i.e. yaho rather than yahoo? Cheers
-
I need to display the data in one big table by month it's for an accounting area. So if the user selects "Show last 2 years" it will show each month at a time on one big table. Cheers
-
Hi I'm getting a bit stuck here and would really appreciate some help. I had made a bit of code to find the first and end date of each month over a range i.e. if a user selected to view 3 months of data it would find and create an array like 2008-01-01, 2008-01-31, 2008-02-01, 2008-02-29, 2008-03-01, 2008-03-31 etc I could then use the first and last date in my mysql query to cycle thru each month range and get the relevant data. This was all working fine till I started getting over a years worth of data. This is where i'm struggling I've got the first date i.e 2007-02-14 and I have the last date which will always be today. Is there an easy way to get all the first and end dates of each month in that range? My code is below but i think it might be pretty useless: if ($startMonth > $endMonth) { for ($i = $startMonth; $i <= 12; $i++) { $startDatesArray[] = strtotime(($currYear - 1) . "-" . $i . "-01"); } $x = count($startDatesArray); for ($i = 01; $i <= $endMonth; $i++) { $startDatesArray[$x] = strtotime(($currYear) . "-" . $i . "-01"); $x++; } } /*** if it's not a new year and the previous month is less than the end month do below ********/ else { for ($fm = $startMonth; $fm < $endMonth + 1; $fm++) { $monthArray[$fm] = $fm; $days_in_month[$fm] = cal_days_in_month(CAL_GREGORIAN, $monthArray[$fm], $currYear); $startDatesArray[$fm] = strtotime($startYear . "-" . $monthArray[$fm] . "-01"); } } many thanks
-
Hi I'm having a real problem with creating a sortable list with scriptaculous. I've got it working just fine in Vista but for some reason it wont work at all in xp on firefox or ie6. I just keep getting the error: Sortable is not defined. My code is below. If any one has any ideas why xp might not work that'd be great. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd "> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> <script src="http://localhost/frameworks/prototype.js" type="text/javascript"></script> <script src="http://localhost/frameworks/scriptaculous/src/scriptaculous.js"></script> <title>test</title> </head> <body> <ul id="categories" class="sortableList"> <li id="item_1">Galleries</li> <li id="item_2">Art</li> <li id="item_3">Roadtrip</li> <li id="item_4">Yosemite</li> <li id="item_5">Animals</li> <li id="item_6">Australia</li> <li id="item_7">Wesleyan</li> <li id="item_8">California</li> </ul> <script language="JavaScript" type="text/javascript"> Sortable.create("categories", { onUpdate: function() { alert('here'); } }); </script> </body> </html> cheers
-
I thought it ordered by ASC as default? I followed your advice and added it in and it worked fine though so thanks very much.
-
Hi, I'm having a bit of struggle with an order by mysql statement. My query is: select * from news_tours where area='news' order by timestamp limit 0, 3 This works fine and sorts everything if i don't have the limit on there but doesn't sort as expected when it's there. I'm sure this is very simple but if anyone could help that'd be great. Cheers
-
Hi I've just downloaded and started having a play with open rico drag and drop. I'm really struggling to find much information on it though. I was hoping that i could drag a div and using ajax fill mysql depending on where a user had dropped something. I just have no idea how to make this happen and after ages of trawling the net i've not come up with much. Could anyone point me in the right direction? Many thanks
-
Hi I'm trying to create a text image with a transparent background. I can create the image no problem but seem to get stuck with the transparent background it just keeps making it a solid colour. Here's my code: function createHeadings($string, $bckCol, $txtCol, $path, $name, $dlcLocation, $fontSize) { $font = './fonts/arialbd.ttf'; //(FONT_SIZE, ANGLE, FONT, $STRING) $bb = imagettfbbox($fontSize, 0, $font, $string); $w = abs($bb[4] - $bb[0]); $h = abs($bb[5] - $bb[1]); $h = 11; $im = imagecreatetruecolor($w + 5, $h+; $textColour = imagecolorallocate($im, $txtCol[0], $txtCol[1], $txtCol[2]); $backColour = imagecolorallocatealpha($im, $bckCol[0], $bckCol[1], $bckCol[2], 0); imagefilledrectangle($im, 0, 0, $w+5, 29, $backColour); // (IMAGE, FONT_SIZE, ANGLE, X-START, Y-START, COLOUR, FONT, TEXT imagettftext($im, $fontSize, 0, 01, $h+2, $textColour, $font, $string); $finalPath = "../$dlcLocation/$path/$name"; imagegif($im, $finalPath, 100); echo "<img src='$finalPath'><p />"; imagedestroy($im); } from what i could see in the manual I could just use imagecolorallocatealpha? Thanks for any help
-
I'd been hoping that I could use a class for all my form submissions and mysql inputs. Basically a user would submit a form to process.php that would then call the appropriate function from my siteSession class I presume this is not good practice then?