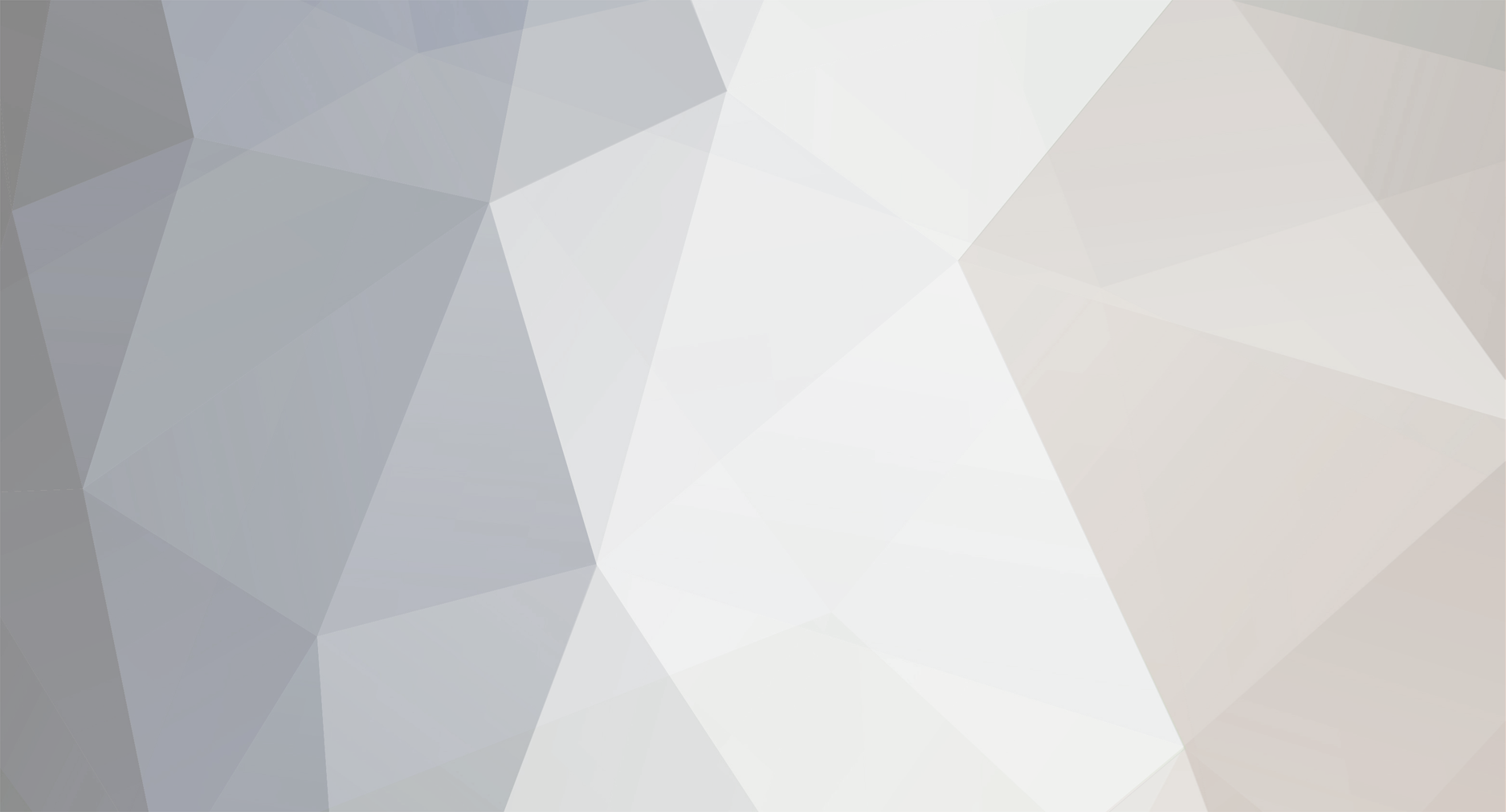
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
Can you show me an example of how you can do this? Where you can tell the difference between a mismatched password and a user not existing, and only do one query?
select (select count(*) from users where username = 'dog' and password = md5('xxxxxx')) as user_found, (select count(*) from users where username = 'dog') as use_exists;
You realize that's still two queries?
Which is fine, it's really not that big a deal. I was mostly being hypothetical on not doing 2 queries.
No, it is one query with two selects.
-
Can you show me an example of how you can do this? Where you can tell the difference between a mismatched password and a user not existing, and only do one query?
select (select count(*) from users where username = 'dog' and password = md5('xxxxxx')) as user_found, (select count(*) from users where username = 'dog') as user_exists;
-
I don't get it....
-
I may be wrong, but I think you need to sum up TotalSales and multiply that by the price.
SELECT (Cars.Price * SUM(MonthlySales.TotalSales)) as revenue FROM Cars JOIN MonthlySales ON ( Cars.CarCode = MonthlySales.ProductCode ) GROUP BY Cars.CarCode
-
I only need to to update, for example, an employe could be ABSENT on 2011-12-01 But he was taken as PRESENT, now I need to fix, so I need to update
my "ON DUPLICATE KEY UPDATE" will just that
-
the easiest way is through the command line, this can import a file about 100 times faster than phpmyadmin.
cd C:\wamp\bin\mysql\mysql5.1.xx\bin mysql -h localhost --password=xxxxxx -u username databasename < C:\my_sql_file.sql
replace xxxxxx with your password, databasename with the name of your database and my_sql_file.sql with the name of your sql file
-
I don't know how your page is laid out but assuming that you are using two radio button for each employee (one for present one for absent)
<input type="radio" name="ap['a']" value="123" /> <input type="radio" name="ap['p']" value="123" /> <input type="radio" name="ap['a']" value="456" /> <input type="radio" name="ap['p']" value="456" /> <input type="radio" name="ap['a']" value="789" /> <input type="radio" name="ap['p']" value="789" />
now that builds us two arrays one for present employees one for absent employees.
Next we want to update our rows.
The follow uses a unique key on date and employee_id (for an on duplicate key update)
// Process the absent employees $absent = implode(",", $_POST['ap']['a']); $abs = array(); foreach($absent as $a){ $abs[] = "(currdate(), $a, 'a')"; } $av = implode(",", $abs); mysql_query("INSERT INTO my_table (date, employee_id, status) values $av ON DUPLICATE KEY UPDATE status = values(status)"); // Process the present employees $present = implode(",", $_POST['ap']['p']); $pre = array(); foreach($present as $p){ $pre[] = "(currdate(), $p, 'p')"; } $pv = implode(",", $pre); mysql_query("INSERT into my_table (date, employee_id, status) values $pv ON DUPLICATE KEY UPDATE status = values(status)");
-
First do an explain on the select portion of your query:
EXPLAIN SELECT table1.id FROM table1 JOIN table2 ON table1.id = table2.id WHERE … ORDER BY table1.date DESC LIMIT 400
is it using any indexes?
No? Add some. (probably on columns table1.id and table2.id)
-
Use mysql in()
$ids = array(23,234,242,12,234,1234); $idstr = implode(",", $ids); mysql_query("UPDATE account SET found = 1 WHERE id in($idstr)");
-
you can use unset
// unset 1 item unset($_SESSION['val1']); // unset multiple items unset($_SESSION['val1'], $_SESSION['val2'], $_SESSION['val3']); // unset all session data unset($_SESSION);
-
use sessions session_start
page2.php
session_start(); $_SESSION['val1'] = $_POST['val1']; header("location: page3.php"); exit;
page3.php
session_start(); echo $_SESSION['val1'];
-
function __autoload($class) { require strtolower($class) . '.php'; }
Might want to use require_once, require will attempt to load it again. if it already has been loaded you will get an error, since you would then be trying to redefine a class.
-
To use autoloaders, it is best to have strict naming standards. For example, all files lowercase and all classes start with uppercase. You'll also have to use only one class per file, because with an autoloader you load the class based on the file name.
I always name my class files with an uppercase first letter such as MyClass.php then when I do an Autoload, I do something like this:
function __autoload($class){ $file = "path/to/files/$class.php"; if(is_file($file)) require_once $file; }
-
It is common practice to have 1 class in 1 file.
-
This is just a rough draft, but it does work (please read the comments):
<?php $salt = "oasd83or34509ig6DF"; echo $message = "Hello, what are you doing tonight?"; echo "<br><br><br>"; // Encode it for the database: echo $val = base64_encode(base64_encode($salt).base64_encode($message)); echo "<br><br><br>"; // An attempt to decode it if you DON'T know how it was hashed: // It will return something like this: "b2FzZDgzb3IzNDUwOWlnNkRGSGVsbG8sIHdoYXQgYXJlIHlvdSBkb2luZyB0b25pZ2h0Pw==" // You need the salt to get past, and the algorithm echo $return = base64_decode($val); echo "<br><br><br>"; // An attempt to decode it if you DO know how it was hashed: echo $return = base64_decode(base64_encode($salt).base64_decode($val)); echo "<br><br><br>"; // Remove the salt (2 salts were added for some reason) echo preg_replace("/^$salt$salt/i", "", $return);
-
you could store them as a base64 string using a salt
-
usually it is in a "Logs" directory where php is installed.
One thing I see is your trying to make a class but have an invalid structure.
class MyClass{ public $error = array(); public function validateFirstname($str){ if (preg_match('/^[a-zA-Z0-9]{2,}$/',$str)) { return preg_match('/^[a-zA-Z0-9]{2,}$/',$str); } else { $this->error[] = 'Please enter a valid first name!'; } } }
-
look at your error logs.
-
I would do something like this:
$arr = array(); $getRecords= mysql_query("select student_info.id, student_name, student_subject FROM student_info,student_subject WHERE student_info.id = student_subject.student_id"); while($getresult=mysql_fetch_assoc($getRecords)){ $arr[$getresult['id']]["name"]= $getresult['student_name']; $arr[$getresult['id']]["subjects"][] = $getresult['subject_name']; } print_r($arr);
-
Why is it I always figure it out almost instantly after I post?
imagecopy not imagecopymerge
-
I have 2 GD images, one is a JPG, the other is a gd created image.
<?php header("content-type: image/png"); $img = imagecreatefromjpeg("./real/soldier.jpg"); $img2 = imagecreatetruecolor(300, 300); imagesavealpha($img2, true); $color = imagecolorallocatealpha($img2, 255, 0, 0, 75); imagefill($img2, 0, 0, $color); $final = imagecreatetruecolor(300, 300); imagecopymerge($final, $img, 0, 0, 0, 0, 300, 300, 100); imagecopymerge($final, $img2, 0, 0, 0, 0, 300, 300, 100); imagepng($final, null, 9);
I am trying to place the gd created image (Red image) on top of the other (soldier.jpg), but for some reason I can not figure out why the red is a solid red when I told it to have transparency to it (75).
So basically how do I create images like this with saved alpha?
-
I am making a PHP GD Library, which in the end Will have lots of features (Hopefully). I don't currently have a download for it but I do have documentation/tutorials. I was hoping I could some feed back on things to add/change to the class and/or documentation.
Here is a link (its mostly pictures
)
-
OR:
ALTER table test3.users ADD primary key (uname);
-
I think something like this is what you want:
SELECT SQL_CALC_FOUND_ROWS, * FROM ( ( SELECT * FROM `users` JOIN `u_data` ON `users`.`ID`=`u_data`.`ID` WHERE `users`.`ID` in (4,5,6,7) AND `u_data`.ID NOT IN (1,2,3) ORDER BY FIELD(`u_data`.ID, 4,5,6,7) ) UNION ( SELECT * FROM `users` JOIN `u_photographers` ON `users`.`ID`=`u_photographers`.`ID` WHERE `users`.`Level_access`=2 AND `users`.`active`=1 AND `users`.`ID` NOT IN (1,2,3) ORDER BY ((`u_data`.`referral_points` + 60) * RAND(5330)) DESC ) ) as myTable LIMIT 10, 10;
PHP Login Not working?
in PHP Coding Help
Posted
database access!
What do I win?