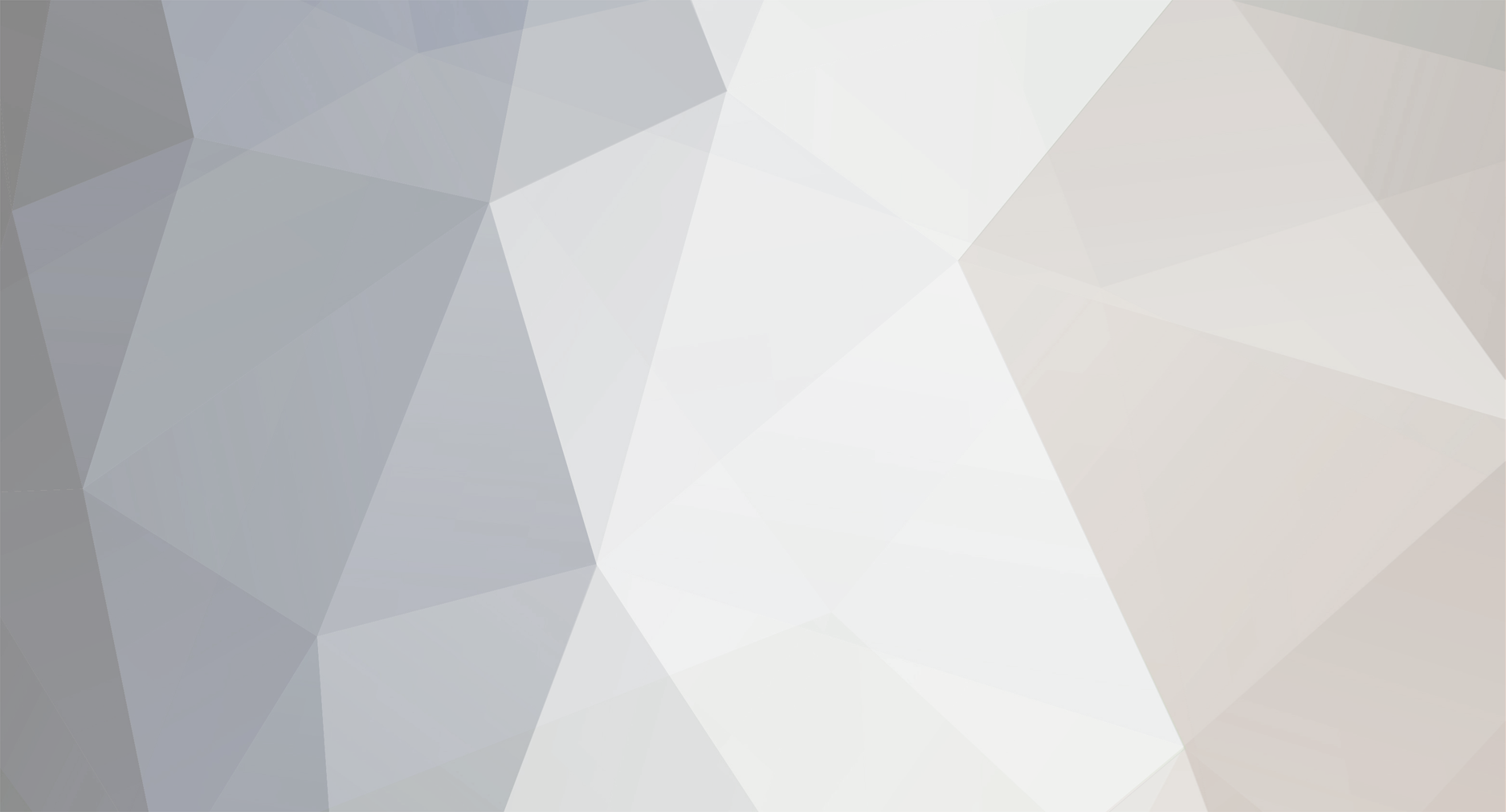
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
Is there any why to test if a given date (YYYY-MM-DD) is a valid date? I would like to check via javascript before I check via php.
Anyone know of a good way to test?
-
But it is my 3 most viewed file....
-
JavaScript that executes what it is supposed to do, for example:
<script> alert("hello"); </script>
will actually display an alert. I have ads that use JavaScript, I would like to add them to my RSS feed.
-
I have never tried this, and have been attempting... Can I put working javascript in an XML file? If so how?
-
That is the affect of the SUM function. If you want each one to show, you need to use a group, but then your sum will probably not have the same number.
-
This will completely remove all \r and/or \n
// Gets rid of \r\n completely: $source = preg_replace("/\r|\n/", "", $source);
-
3) Get hosting outside the UK
-
Objects have procedural code within them. In the end, object are non-existent.
-
To do this, you could do something like this(untested):
<?php require_once "db_connection.php"; require_once "MysqlClass.php"; $database_name = "test"; $new_database_name = "test2"; $create_table = array(); $tables = array(); // User, password, host, database $db = new Mysql("user", "password", "localhost", "test"); $db->query("show tables"); while($row = $db->row()){ $tables[] = $row["Tables_in_".$database_name]; } foreach($tables as $table){ $db->query("show create table $table"); $row = $db->row(); $create_table[$table] = $row["Create Table"]; } // Create the new database and tables $db->query("create database $new_database_name"); $db->query("use $new_database_name"); foreach($create_table as $table => $create){ $db->query($create); $db->query("alter table $table auto_increment = 0"); } echo "\n\nNew Database Created!\n\n"; ?>
-
Only the InnoDB supports "Real" Foreign Keys:
http://dev.mysql.com/doc/refman/5.5/en/innodb-foreign-key-constraints.html
But you can have Foreign Keys in any other engine, but they won't be constant. With InnoDB, if you have a Primary Key and 10 tables that have a Foreign Key that points to the parent tables Primary Key, you can then update the primary key and all your foreign keys update along with it. In any other table you would have to update all the foreign keys manually.
That is as much as I have read/heard about Foreign Keys.
-
I heard that PHP 6 was going to be compilable so it would run faster
-
The only thing I have really made was something that did some bench marking.
$d = new Debug(); // pass false to disable // Do some code $d->time(); // Do some code $d->time(); // Do some code $d->time(); // Do some code $d->time(); // Do some code $d->time();
every time you called the time() method it would echo something like this:
0.0282020983 seconds at line 2 in file /home/www/index.php 0.0282020983 seconds at line 10 in file /home/www/index.php 0.1282020983 seconds at line 81 in file /home/www/index.php 4.0282020983 seconds at line 120 in file /home/www/index.php 4.0482020983 seconds at line 578 in file /home/www/index.php
As you can see on the 4th call it jumps up to 4 seconds and is something you might want to take a look at. If you were to place the $d->time() in an included file, it would say that file's name and the line in that file. I don't know if there is a better way to do this, but It has allowed me to pin point slow sections with in code after loops, MySQL queries, or any other constructs/functions/methods.
-
We use git.
The live site doesn't have git on it yet, but our beta one does. We are building a new phpsnips, and we have our beta on beta.phpsnips.com which has git, then we also have a personal copy of the website so we can run it on our personal computers too, then when we like what we have done we commit our changes to the beta. Once the beta is all complete, we will add git to the live site, and download the repository to the live site. This process will take less than 30 seconds. Once the live is the same as the beta we can work on our beta and push to the remote repository hundreds of times, then once there is a new feature ready to go live we will pull from the remote again on the live site and the new stuff will be live almost instantly!
git is a fun and useful tool you should look into if you don't use it.
-
Is there anything that uses Nginx? I like that better than Apache.
-
I prefer WAMP, to me it seems easier to customize.
-
I feel that this image has a very good message, and I figure I should share it with you!
-
no, that is the time the file was modified on the server, I want something that gives me when the file was last downloaded or something like that.
I have this:
header("Cache-Control: private, max-age=10800, pre-check=10800"); header("Pragma: private"); // Set to expire in 2 days header("Expires: " . date(DATE_RFC822,strtotime(" 2 day"))); if(isset($_SERVER['HTTP_IF_MODIFIED_SINCE'])){ // if the browser has a cached version of this image, send 304 header('Last-Modified: '.$_SERVER['HTTP_IF_MODIFIED_SINCE'],true,304); exit; }
But it only seems to work on Apache.
-
I need to know when a file was last modified to see if the browser has an updated cached version, if the browser does use its cache otherwise get the most current version.
How can I do this?
-
perfect!
Thanks for the help!
-
I am trying to get the top 10 most popular searches from my database, I have the following:
select *, count(*) c FROM searches group by search_query order by c desc
This will get the top ten, but it will also get values for people that searched for the same thing 2+ times. So if 2 people search for "Monkey", and one person searches for it 5 times and the other searches for that 3 times, it should show it was searched for 2 times.
I am not sure how to format that query....
mysql> explain searches; +--------------+------------------+------+-----+-------------------+----------------+ | Field | Type | Null | Key | Default | Extra | +--------------+------------------+------+-----+-------------------+----------------+ | search_id | int(10) unsigned | NO | PRI | NULL | auto_increment | | ip | int(10) unsigned | YES | | NULL | | | search_query | varchar(100) | YES | | NULL | | | search_date | timestamp | YES | | CURRENT_TIMESTAMP | | +--------------+------------------+------+-----+-------------------+----------------+ 4 rows in set (0.00 sec)
-
login to your web server via putty (Windows) or terminal (Linux/Mac).
in your home dir
cd ~
Download the file
wget http://wordpress.org/latest.zip
next unzip the files to your webserver
unzip latest.zip -d ~/www/mysite.com
-
make 2 tables
table 1: dictionary
- dictionary_id
- word
table 2: definition
- definition_id
- dictionary_id
- definition
so in table 1 dictionary_id would be an auto_increment filed, but not it table 2 and you would match on those two columns, it would look like so:
select * from dictionary dic left join definition def using(dictionary_id) where dic.word = "bat";
you would then get this:
+---------------+------+---------------+---------------+----------------------------------------+ | dictionary_id | word | definition_id | dictionary_id | definition | +---------------+------+---------------+---------------+----------------------------------------+ | 1 | bat | 1 | 1 | an animal with wings | | 1 | bat | 2 | 1 | an instrument used by baseball players | +---------------+------+---------------+---------------+----------------------------------------+
-
... whereas a single database for several clients, each with different requirements, is a dream?
if it is built properly.
-
Full text has stop words (words that are ignored in a fulltext search), and if you are using shared hosting, you probably cannot change them.
in your example the only words that would have been matched are "Love" and "Coffee"
http://dev.mysql.com/doc/refman/5.1/en/fulltext-stopwords.html
date validation
in Javascript Help
Posted
Here is what I came up with, but I don't know if it is good or not: