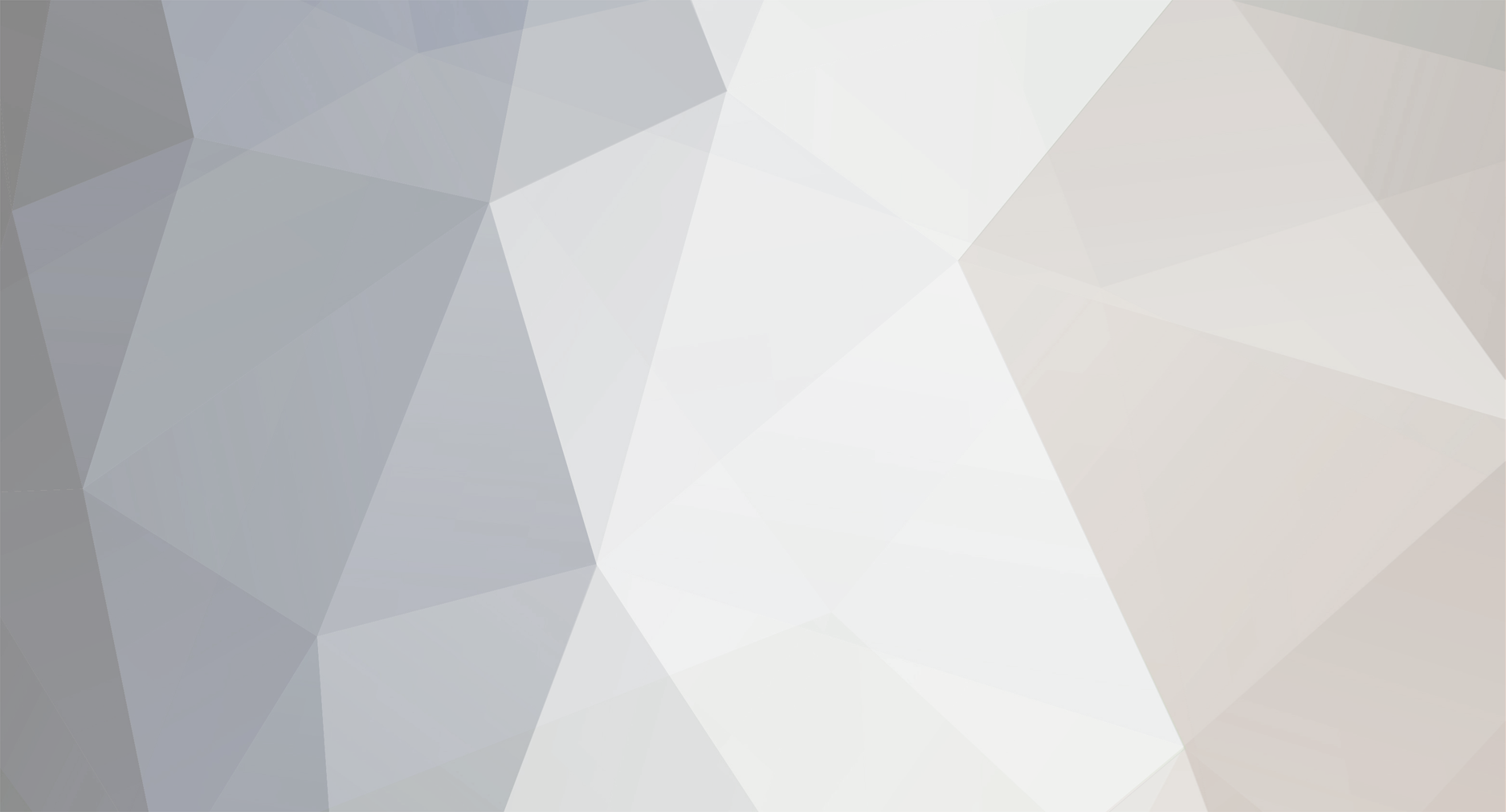
AP81
Members-
Posts
100 -
Joined
-
Last visited
Everything posted by AP81
-
Displaying 'X' results per page PHP and MSSQL
AP81 replied to mikemessiah's topic in PHP Coding Help
Lets say your table has 50 rows: SELECT * FROM table1 LIMIT 0,5 will output the first 5 SELECT * FROM table1 LIMIT 5,10 will output rows 5 to 10 SELECT * FROM table1 LIMIT 10,15 will output rows 10 to 15 Get it? So what you need to do is display a pager which passes a parameter to the page, i.e. Page (1) (2) (3)... Clicking 3 will pass '3' to the page, so you know you query will be limit 10,15 (15 = 3 x 5 and 10 is 15 - 5 result per page) So lets say your query as a whole contains 47 results, you know that you will have to display Page (1)..(10). 45 / 9 is 9.4, so will need 10 pages. You would do something like this (copy and paste this code so you get an idea of what is happening): $db_result_count = 47; // lets pretend there are 47 results $pages = ceil($db_result_count / 5); // round it up to the nearest whole number for ($i = 1; $i <= $pages; $i++) { echo "<a href=\"$PHP_SELF?page=$i\">$i</a> "; } Now when a link is clicked, the page will redirect to itself, and you can use $_GET["page"] to find what you have clicked, and do a query. You can beautify it by making a nice css style and apply it to the <a> tag, i.e. <a class="pager" ...> -
If it is a Linux machine you can: 1. configure a cron task (Google: Linux cron task) 2. Your cron task can then run the PHP file.
-
You don't need to use a .php file if you want to read static content. Have a look in the PHP file for fopen. Using fopen you can open the file, then use fread to read the file.
-
MAX_JOIN_SIZE & query takes 4.7 seconds with no other processes running
AP81 replied to kaosweaver's topic in MySQL Help
Firstly: Table jtMemberCategory has no primary key. If member_id and cat_id together make a unique key, the create a composite key on these columns. Secondly: Use the EXPLAIN MySQL function. Make this a habit! This will help you to optimise you joins and find bottlenecks Thirdly: I would change your query to this: SELECT c_name, tblmember.catid, tblmember.memberid, tblCategory.lft, tblCategory.rgt FROM (tblCategory left JOIN jtMemberCategory ON tblCategory.catid=jtMemberCategory.cat_id) left JOIN tblMember ON tblMember.member_id=jtMemberCategory.memberid WHERE tblCategory.cat_id=25 AND jtMemberCategory.member_id=5 ORDER BY c_name Because jtMemberCategory has 245,000 rows, specifying jtMemberCategory.member_id=5 cuts this table down a lot. Your joins in theory will speed up. I am guessing that you are using MySQL control center, as I can recall having a similar problem. Make sure you use the latest version will fix this problem. -
I use the Pear MDB2 database abstraction layer to connect to MSSQL. You can get it from here: http://pear.php.net/package/MDB2 I previously used ODBTP to connect to MSSQL which also works well. One last question: did you restart Apache after commenting out php_mssql.dll?
-
Use the MySQL concat_ws function. This will allow you to concatenate multiple fields with a separator of choice, which in your case is space. SELECT CONCAT_WS(' ', FIRST_NAME, MIDDLE_NAME, LAST_NAME) AS NAME FROM people
-
1) Yes, that looks nice. Nice and normalised to 3NF. 2) The only real way to adhere to good database design with this is to have separate tables for each category. For example, clothes would have a table cat_clothes. Exercise equipment would have cat_exercise. I know you probably don't want to go down this path, but there is too much variance between categories and what information needs to be stored. Storing these in a single table would mean that you are storing a lot of redundant data. Someone correct me here if you believe otherwise. 3) Use decimal(10,2) for fields that will contain monetary values. If you use a float it will round the values, while decimal won't. I don't see how or why you would want to use an Integer data type here. You need to store the amount accurately, you shouldn't have to manipulate/calculate prices.
-
Looks like you aren't joining the tables correctly. Take a look at your code, I can't see anywhere where you are joining the villas table to the resorts table. You'll need to edit your query to something like this: <?php $sql = "SELECT villas.*,resorts.resortName FROM villas, resorts WHERE villas.resortID = resorts.resortID; ?> This takes into account that resortID is the Key you will be joining on.
-
You can do something like this: <?php foreach ($_POST as $key => $value) { if (!empty($value)) { //do something } } ?> However I'd advise against it. It is much better to do individual validation for each posted item.
-
You need to dynamically build your query, based on what information you have. Take a look at this post which I gave some advice on something similar to what you are doing: http://www.phpfreaks.com/forums/index.php/topic,191974.msg862474.html#msg862474
-
Can you clarify what exactly you want to do here? Your question is vague.
-
Omitting gender from the query will make your results include both male and female: <?php $data = mysql_query("SELECT * FROM users WHERE registered_as='model' and ethnicity='$ethnicity' and btype='$btype'); ?>
-
I'm not quite sure why are taking this approach, it would seem more sensible to include a file on each of the restricted pages which does the authentication, i.e. In area.php you would include a file called authenticate.php <?php require_once('authenticate.php'); ?> <html> <head> ... authenticate.php would need to include the checkIsLoggedIn(); and checkSession(); functions. This way you don't have to hard code any page names.
-
Why do you post the page to itself? There are two ways to fix this: 1) Change the form action in to page2Form.php to page2Form.php <?php <form action="page2Form.php" method="post" > ?> In page2Form.php, you would do this: <?php $Username = $_POST['Username']; $Password = $_POST['Password']; $PasswordQuestion = $_POST['PasswordQuestion']; $PasswordAnswer = $_POST['PasswordAnswer']; mysql_query("INSERT INTO `PersonnelDetails` (Username, Password, PasswordQuestion, PasswordAnswer) VALUES ('$Username', '$Password', '$PasswordQuestion','$PasswordAnswer')"); ... ?> 2) you can call redirect the page using JavaScript <?php mysql_query("INSERT INTO `PersonnelDetails` (Username, Password, PasswordQuestion, PasswordAnswer) VALUES ('$Username', '$Password', '$PasswordQuestion','$PasswordAnswer')"); echo "<script>document.location.href = 'page2Form2.php'; </script>"; ?> IMO it is hack programming to submit a form, then redirect to another form... so I'm not sure why you want to do this exactly.
-
That will probably work, but you need to pass that as a FlashVar. i.e. ... <PARAM NAME=bgcolor VALUE=#FFFFFF> <EMBED src="flaMovie1.swf?imageFilename=$fruit1 ... In you flash program you will need to read in the variable imageFilename, then display the picture if that is valid.
-
You are missing a bracket. You need another bracket after the asterisks': SELECT CONCAT(LEFT(PHONE,9), ' ***')
-
You need to output the PDF before any HTML is outputted to the page, so you would have need to do something like this: <?php if isset($data) { createPDF($data); return; } ?> <html> <head> ...
-
You shouldn't run into any problems here. Just make sure you stop the SQL service and do a full backup prior to converting the table engine.
-
You can't pass images to Flash. All you can pass is variables.
-
You could also do it in MySQL like this: SELECT CONCAT(LEFT(PHONE,9), ' ***') FROM tablename
-
$sPhone = '09876 987 876' $sSecurePhone = substr($sPhone,0,9) . ' ***'
-
Good point. Yes, you are right, it would do an entire select. To get around it, you can do this: <?php $selectString .= BuildQuery("petName",$petName); $selectString .= BuildQuery("petSpecies",$petSpecies); $selectString .= BuildQuery("petBreed",$petBreed); $selectString .= BuildQuery("petAge",$petAge); $selectString .= BuildQuery("petWeight",$petWeight); if (strlen($selectString) == 0) { die('Please ensure one or more fields are filled'); } else { $selectString = "select * from tblPatientBK " . $selectString; // add the query parameters to the base query $resultPatient = mysql_query($selectString); } // do the same thing to the next query ... ?>
-
Try this: SELECT events_signup.event_id, events.* FROM events_signup, events WHERE events_signup.event_id = events.event_id AND user_id='$user_id' AND events.date > NOW()
-
That looks fine, but just remember that if you want Foreign Key support you need to have your table engine set to InnoDB, not MyISAM. MyISAM will accept the syntax for foerign key, but it won't actually enforce referential integrity. Someone please correct me if I am wrong.
-
The is most likely something in the PHP.ini file. Best to take a look at the PHP manual and take a look at it.