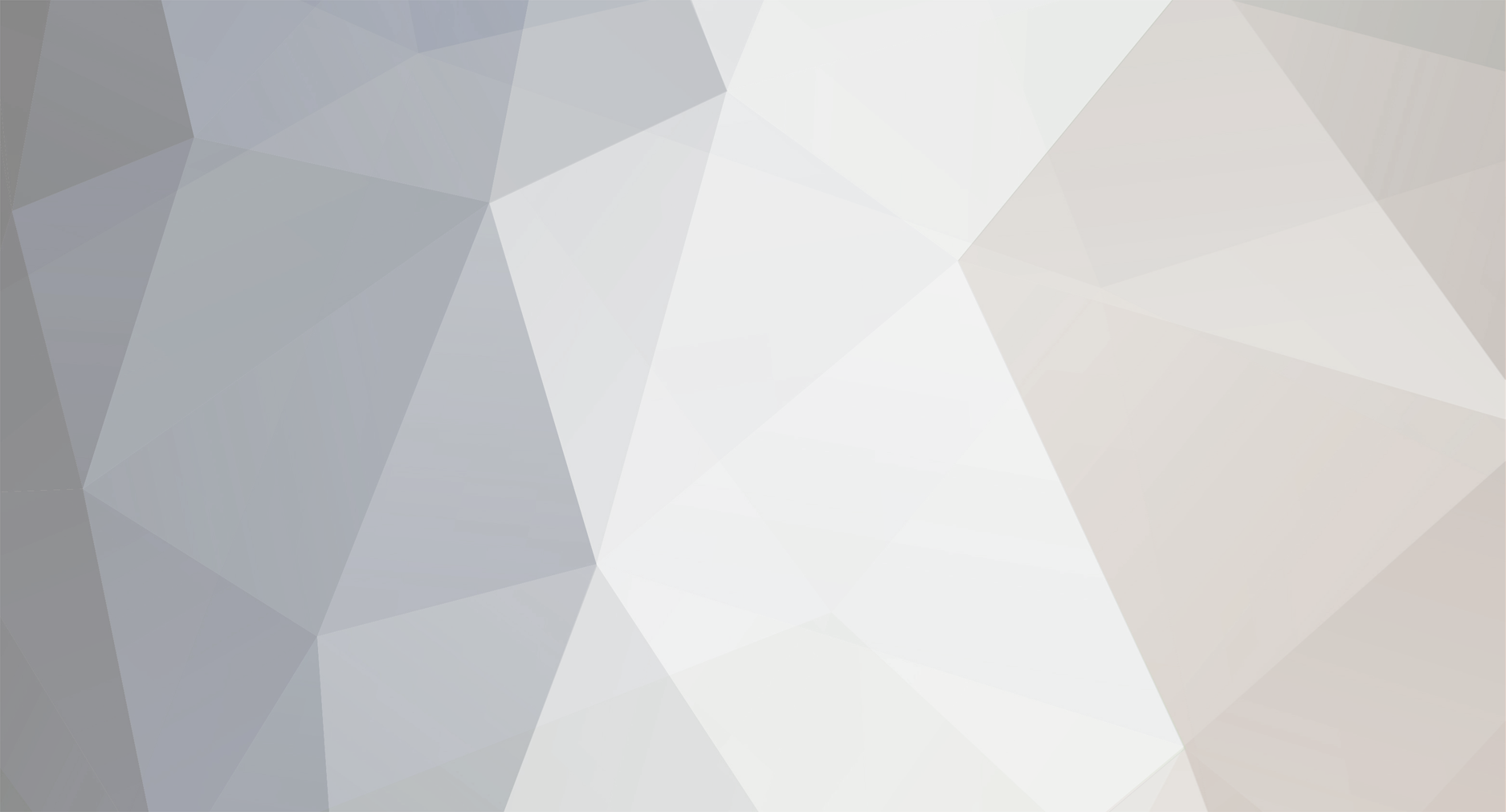
rcorlew
Members-
Posts
307 -
Joined
-
Last visited
Never
Everything posted by rcorlew
-
password protect with (or without) sessions?
rcorlew replied to HaLo2FrEeEk's topic in PHP Coding Help
Do you currently have a login page? If so using sessions is easy like so. <?php $username = $_POST["username"] $pass = $_POST["pass"]; if(isset($username) && ($pass)) { $hashedpass =(sha1($pass)); $link = mysql_connect('localhost', 'user', 'pass'); mysql_select_db(DBname) or die; $result = mysql_query("select count(userid) as Total from users where username='$username' and password='$hashedpass'",$link); $row = mysql_fetch_array($result); if ($row["Total"]=="0") { echo "<h3 style='color: red; text-align: center;'>WARNING: USERID/PASSWORD Failed!</h3> <p align='center'>Please attempt to login below</p>"; } if ($row["Total"]=="1"){ echo "<div align='center'> <h3>You are now logged in</h3> <b>Welcome Back $username</b><br /><br /> </div>"; $_SESSION['username'] = (md5($username)); mysql_close($link); } } } //Then here is how the session plays into it if(isset($_SESSION['username'])){ //Do whatever you want to here, they now have been logged in and have sessions set to use } ?> I do not know if you are incrytping or hashing their passwords, but change the sha1 to whatever you need -
This might help you get a good start: <?php // Connect to MySQL database $con = mysql_connect($HOST, $USER, $PASS); if (!$con) { // Since the entire script depends on connection, die if connection fails die("Error connecting to MySQL database!"); } mysql_select_db($NAME, $con); function getCareers($careers) { $days = array(); $sql = mysql_query("SELECT * COUNT(career_id) FROM career_table"); if (mysql_num_rows($sql) > 0) { while ($row = mysql_fetch_array($sql)) $careers[] = $row['careers']; } return $careers; } function drawCareerTable($careers) { // Start drawing calendar $out = "<table id=\"myCareer\">\n"; $out .= "<tr><th colspan=\"2\">Careers</th></tr>\n"; $out .= "<tr>\n"; foreach ($careers as $c) $out .= "<td class=\"isCareer\">$c</td>\n"; $i = 0; for ($e = (1 - $offset); $e <= $careers; $e++) { if ($i % 2 == 0) $out .= "<tr>\n"; // Start new row if ($e < 1) $out .= "<td class=\"nonCareer\"> </td>\n"; else { if (in_array($c, $careeres)) { $out .= "<td class=\"isCareer\">\n"; $out .= "$c\n"; $out .= "</td>\n"; } else $out .= "<td class=\"isCareer\">$c</td>\n"; } ++$i; // Increment position counter if ($i % 2 == 0) $out .= "</tr>\n"; // } // Round out last row if we don't have any more careers if ($i % 2 != 0) { for ($j = 0; $j < (2 - ($i % 7)); $j++) { $out .= "<td class=\"nonCareer\"> </td>\n"; } $out .= "</tr>\n"; } $out .= "</table>\n"; return $out; } echo drawCareerTable($careers); ?> It should work just adjust the tablename and stuff to suit your needs
-
That's pretty much where I am headed now. So I think that I will get a couple of Oreilly refrence manuals and just keep challenging myself. I am not talking about the nOOb create a website with php/mysql stuff, I mean php security and mysql ref. manuals. The php manual already resides on my laptop as chm and that is perfect for me. I guess that when it comes to this line of work people always want to see what you have done and what you can do.
-
I have been on the ol' learn as the need arises path and have found that to be very fitting. I was asking because some of the people in here are very talented and I was thinking they had to go to school for that.
-
echo date('M j, Y');
-
Thats what the LIMIT 4 is. As long as your id row is set to autoincremint that will work wonders. It will pull the last 4 rows of data which will be the newest 4 rows.
-
[SOLVED] Echoing if there are no results from the DB to display
rcorlew replied to HoTDaWg's topic in PHP Coding Help
You should use if($result == null){ echo "No results to display!"; }else{ // other query here I have found that using a num_rows on top of a while loop throws the num_rows off for some reason that I am unfamiliar with -
$sql = mysql_query("Select * FROM tablename ORDER BY id DESC LIMIT 4");
-
You should echo out the errors when debugging like so: $res=dbQuery("SELECT B.PD_THUMBNAIL,B.PD_NAME, B.PD_DESCRIPTION,B.PD_QTY,B.PD_PRICE FROM TBL_CATEGORY A, TBL_PRODUCT B WHERE A.cat_Parent_ID=$category AND A.CAT_ID=B.CAT_ID ORDER BY B.PD_PRICE") or die(mysql_error()); You may find something simple that you are not seeing like ' or " unescaped.
-
I am wondering if there are any college or online courses or classes that teach php. At my local colleges are I have seen is java and visual basic. I have not even seen any perl classes around me. I was also wondering if they would be better than just spending $200 on some good books and teaching myself? I feel that I am somewhere near the end of the beginner stage and can also work out some good code and have taught myself good coding techniques that make it easy to modify old scripts to maintain them.
-
It should be like this: echo "<td>$record[PD_NAME]</td>"; Not like you have as this: echo "<td>$record['PD_NAME']</td>"; You have a ' around your row($record) results.
-
You are not checking the used_ips in your table before you do an insert. You should do a query to check if the ip has been used and then something like this <?php $ip = $_SERVER['REMOTE_ADDR']; $query = mysql_query("SELECT * FROM table WHERE used_ips = '$ip'"); $num_rows = mysql_num_rows($query); if($num_rows == 0) { //run insert query here } if($num_rows > 0) { echo "You have already voted"; } ?>
-
Much nicer. I like the new layout style.
-
I tend to think of things through if statements. if($clerk_work == null){ unset($command) $newcommand="$command++"}
-
I am trying to sanitize $_POST and $_GET variables globally to stop things like sql injection. I have not really been able to get it to work. I have a nice way to replace the things I don't want to be posted but I am trying to do this globally and not one value at a time. Here is the code that I have so far: <?php $nonhack = "$string1"; $hackcheck = strtolower($nonhack); $patterns = array( '`\*`is', '`drop`is', '`\'`is', '`delete`is', '`select`is', '`\(`is', '`\)`is', ); $replaces = array( '\/*\/', 'd/odRo\/', 'nm', 'donot', 'forget', 'mlknxc', 'mlknxc', ); $hackcheck = preg_replace($patterns, $replaces , $hackcheck); //echo "$hackcheck"; ?> Could I just change the $string1 to $_POST or $_GET and reset the data like $_POST = "$hackcheck"; That may sound kind of weird but I am at a loss here.
-
You are setting as 0 here if ($_GET["page"]==$single_page) { $min_num = ($single_page - 1) * 25; $single_page is set to 1 on the initial call and then you subtract that 1 to make it 0 I do not see how you are passing the variable from page to page to make the $single_page increase it's value to the next page.
-
Anywhere from $15-$30 hr and about a day or so worth of work. I really don't do freelance so I really don't know. I have something similar that I have made for one site and it took me about two days to set all the stuff up right. It has all the username, password, forgotten password, email conformation, userlevel things but no avatars and all the personal stuff. No comments from users but that is not hard to add, just like making a blog script.
-
You can either hire someone in the freelancing area or go to www.hotscripts.com Hotscripts should have what you need if you don't mind the linkbacks and stuff.
-
$single_page is set up as being a 0, and 0*25 =0 You should try setting it as 1 and see what happens.
-
[SOLVED] Could you all test my forum "lite" for any flaws?
rcorlew replied to rcorlew's topic in Beta Test Your Stuff!
Thank you for the compliment. I did a lot of homework on what makes a site like that work. I have also gotten lots of ideas/info from all you folks and really do appreciate it. -
I have this script that when it is executed will count the rows in 3 columns with 3 different queries. Then depending on the final query it will run a 4th to fetch array. Here is the code and any suggestions would help if there is anything wrong with doing this this way. At the bottom of the page I do a mysql_free_result for the queries. <?php if(isset($var) { echo "<table id='myForum'> <tr> <th align='center'>Viewing the Forum Info</th> </tr>"; $con = mysql_connect($HOST, $USER, $PASS); if (!$con) { // Since the entire script depends on connection, die if connection fails die("Error connecting to MySQL database!"); } mysql_select_db($NAME, $con); $result = mysql_query("SELECT * FROM users",$con); $usercount = mysql_num_rows($result) or die; $result2 = mysql_query("SELECT * FROM threads",$con) or die; $thread_count = mysql_num_rows($result2) or die; $result3 = mysql_query("SELECT * FROM f_topics",$con) or die; $cat_count = mysql_num_rows($result3) or die; $result4 = mysql_query("SELECT * FROM threads ORDER BY id DESC LIMIT 1",$con) or die; while($row4 = mysql_fetch_array($result4)) { $lastpostby = "$row4[poster]"; $lastthreadposted = "$row4[id]"; $lastreply = "$row4[ref]"; $lastpostdate = "$row4[date]"; $lastposttime = "$row4[time]"; } if($lastreply == 0){ $lastthread = $lastthreadposted; } if($lastreply != 0){ $lastthread = $lastreply; } echo"<tr> <td valign='top'>There are $usercount users registered for this <br /> There are $thread_count posts in $cat_count Topics.<br /> The last post was on $lastpostdate at $lastposttime by $lastpostby<br /> You can view it <a href='forum.php?function=viewtr&thread=$lastthread'>here</a><br /> </td> </tr> "; echo "</table>"; } } ?>
-
Try this, I use it all the time. <?php if($num_rows > 0) { //Put your stuff here } if($num_rows == 0) { echo "We are sorry there were no results found"; } ?>
-
You should try this if your month is the second number in your in your date format: <?php $date = date("j-n-Y") $sql = "Select * FROM table WHERE formdate LIKE '%$date%'" ?>
-
If they keep hitting refresh the browser has the $var, if they are trying to post again and getting old $var the server has the $var. I have not heard of a way of remving a variable on the browser without it being a cookie.
-
After you use a vriable and before you prepare it for reuse you should use "uset($var);" to clean the old value then you can reset the new value and post again.