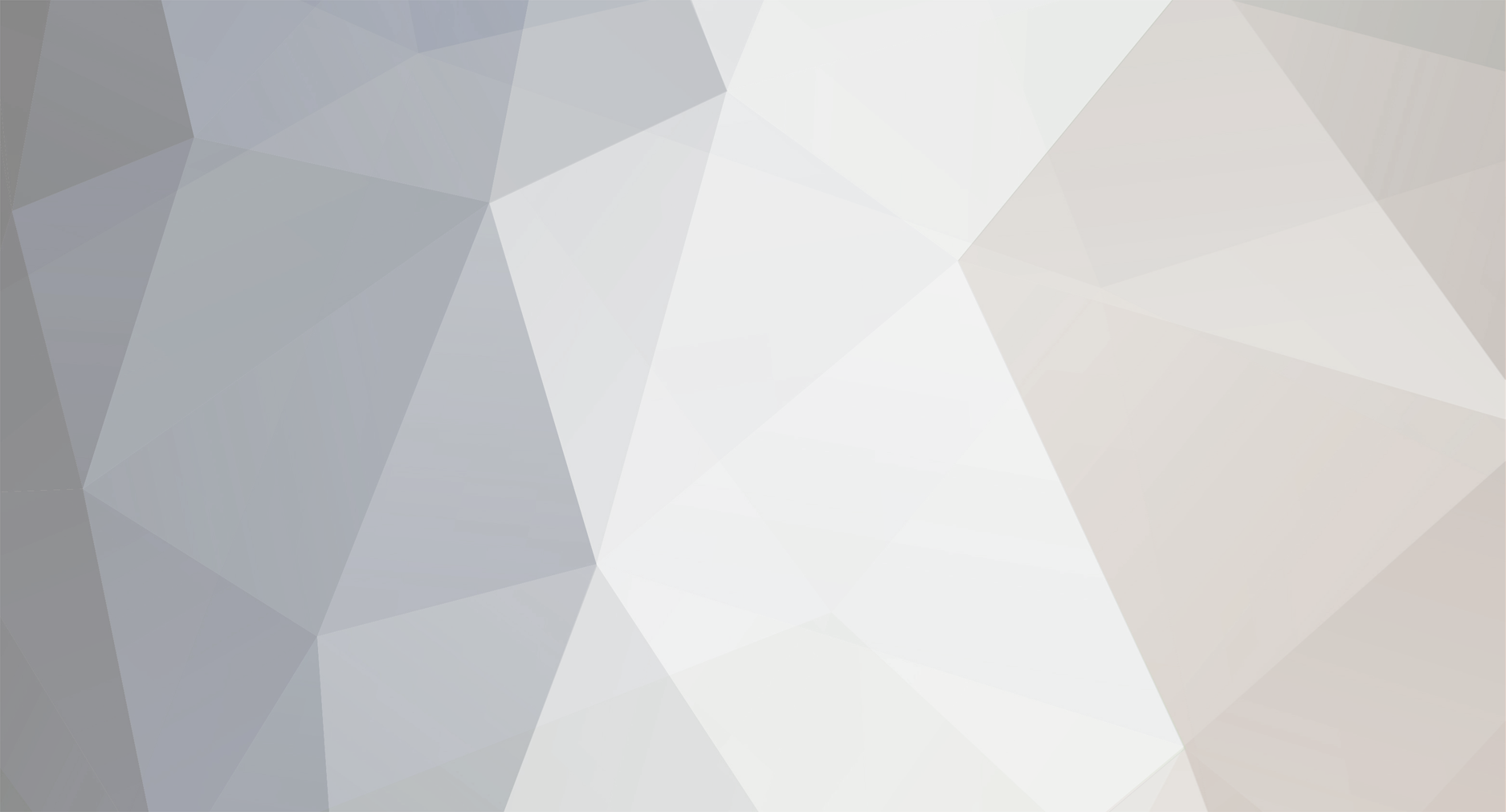
rcorlew
Members-
Posts
307 -
Joined
-
Last visited
Never
Everything posted by rcorlew
-
[SOLVED] How do I create a link to delete an image?
rcorlew replied to sketchi's topic in PHP Coding Help
You would use unlink($filename) in the area where you are checking to see if you are trying to delete it like this. if (isset($_GET['delete']) && $_GET['delete'] == "Yes") { unlink(mypic.jpg); } That should work. IF unlink() by itself does not work on your setup, use fopen() first, then unlink(). There is no native delete function in php, unlink does that. -
First put in a <div> like this: <div style='width: 350px; overflow : auto; padding: 5px;'>Then put user's table here</div> Just change the width of the div to whatever you need, it will scroll automatically and not get too big.
-
You probably caught your host in some maintanence. It is hard to ensure that your scripts don't get messed up because of your host, I had a sql crash a few months ago and lost some stuff. I may suggest trying to contact your host and asking when they do maintennce, and set the jobs to run accordingly.
-
Check the php.ini for the max post size. Most of the time it is between 8-16 mb. you can change that at the top of your script by using ini_set like this: ini_set('max_upload_size', '300000'); ini_set('max_post_size', '300000'); You can try different size limits to get different results. They should come right after you opening tag before anyting is sent to the page.
-
[SOLVED] wont insert anything into my mysql database
rcorlew replied to laron's topic in PHP Coding Help
use this as your query: <?$sql = "INSERT INTO users (username,password,email,firstname etc....... ?> You only have to use the backtics if you are using a reserved sql function as a row/cell like date that is other than the sql native funtion. -
Make sure that it is spelled correctly and is not capitalized or camel capped(eMail), you have a typo somewhere, the script ran fine when I copy and pasted it.
-
You have a comma before $userid, that is indicating 3 columns instead of the two you have declared.
-
Try changing this ('', '$userid', '$username')")or die(mysql_error()); to this ('' '$userid', '$username' ")or die(mysql_error());
-
change $sql="INSERT INTO user (username,password,email,confirmemail,Address,Phoneno) to $sql="INSERT INTO user (Username,password,email,Confirmemail,Address,Phoneno) names are case sensitive
-
[SOLVED] wont insert anything into my mysql database
rcorlew replied to laron's topic in PHP Coding Help
Try changing your variables set in your sql query to this {'$variable'} instead of '".$username."' -
Check the column names are correct, it worked on my server.
-
This should get you going: if(preg_match("[href|</A>|</a>|<script]",$g_name,$nm,PREG_OFFSET_CAPTURE) > 0) { echo"No spam allowed"; exit(); } Much luck.
-
I have just dealt with the same problem but I am using a sql db. The problem was the same, delete only certain text from a text source then rewriting it. I do believe that it could be of use to you since the text is stored as a string then exploded into an array then deleted and then rewritten. Here is a link to that post, I hope this could help you, if you would like help adapting to your scenerio, pm and I will work it out for you. http://www.phpfreaks.com/forums/index.php/topic,137307.0.html Richard
-
The only way to look up records or contents in a file is by using fseek(); . You would have to know where the information you are looking for is and then do the rewrite. Might I suggest converting the contents of the file into a string then do your manipulations and afterwords rewite it back to the file.
-
[SOLVED] How to delete only certain text in a mysql row?
rcorlew replied to rcorlew's topic in PHP Coding Help
Ok, if anyone would like to know how I soved this problem, I will post my code since I could not find any sort of method to delete only certain text. <?php $addto = $_GET["addto"]; if(isset($addto)) { $result = mysql_query("UPDATE users SET friends = CONCAT(COALESCE(friends, ''),',$addto') WHERE userid='$_SESSION[userid]'",$con); } function array_remval($val,&$arr){ $i=array_search($val,$arr); if($i===false)return false; $arr=array_merge(array_slice($arr, 0,$i), array_slice($arr, $i+1)); return true; } $remove = $_GET["remove"]; echo "<table id='myForum'> <tr> <th align='center'>Viewing My Friends</th> </tr>"; // echo "$user"; $con = mysql_connect($HOST, $USER, $PASS); if (!$con) { // Since the entire script depends on connection, die if connection fails die("Error connecting to MySQL database!"); } mysql_select_db($NAME, $con); $query = ("SELECT friends FROM users WHERE userid = '$_SESSION[userid]'"); $result = mysql_query($query) or die; echo"<tr> <td valign='top'><br />"; while($row = mysql_fetch_array($result)){ $users = $row[friends]; $eu = explode(",", $users); $ecount = count($eu); $newcount = $ecount - 1; $x = 0; $y = $newcount; for( $i = 0; $i <= $y; $i++) { if(!isset($remove)) { if($eu[$i] == null) { $eu[$i] = $_SESSION['userid']; } echo "<a href='forum.php?function=viewuser&user=$eu[$i]'>$eu[$i]</a> Send <a href='forum.php?function=sendmessage&user=$eu[$i]'>message</a> <a href='forum.php?function=friends&remove=1&text=$eu[$i]'>Remove</a> from friends<br /><br />"; } if(isset($remove)) { $text = $_GET["text"]; array_remval($text, $eu); } } if(isset($remove)) { $nu = implode(",", $eu); $query = ("UPDATE f_users SET friends ='$nu' WHERE userid = '$_SESSION[userid]'"); $result = mysql_query($query) or die; echo "$nu"; ?> The only weird side effect by using this so far is that by concating the friends field with a comma delimeter, you end up with array[0] being null which I cam up with a very nice solution by making it say the user's name there. Until I can come up with something even fancier to repalce the concate, maybe some sort of is null or if null then do the concate otherwise just enter the first field entry. But, if you would not like to have yourself as a friend, then who would like to be friends with you, lol. -
You would want to use the following query to add (append) data: $con = mysql_connect($HOST, $USER, $PASS); if (!$con) { // Since the entire script depends on connection, die if connection fails die("Error connecting to MySQL database!"); } mysql_select_db($NAME, $con); $result = mysql_query("UPDATE table_name SET column_name = CONCAT(COALESCE(column_name, ''),',$variable') WHERE row_entry='$variable_row_name'",$con); That should get you going in the right direction, if you do not want a delimiter between cell entries, just remove the comma before the $variable and replace it with a space.
-
[SOLVED] How to delete only certain text in a mysql row?
rcorlew replied to rcorlew's topic in PHP Coding Help
OK I have worked up a little code to basically remove the item out of the array that has been created by exploding the text. I used a sutom function that will remove the item from the array and then implode it back into a string and then it can be reinserted. I do not know if this is the most efficient way of doing this, but is the only think I could think of, correct me if I am doing something wrong. <?php function array_remval($val,&$arr){ $i=array_search($val,$arr); if($i===false)return false; $arr=array_merge(array_slice($arr, 0,$i), array_slice($arr, $i+1)); return true; } ?> <?php $remove = $_GET["remove"]; $users = ("thomas,frank,irene,john,luke,jenny"); $eu = explode(",", $users); $ecount = count($eu); $newcount = $ecount - 1; $x = 0; $y = $newcount; for( $i = 0; $i <= $y; $i++) { if(!isset($remove)) { echo "<a href='project2.php?remove=1&text=$eu[$i]'>$eu[$i]</a><br />"; } if(isset($remove)) { $text = $_GET["text"]; array_remval($text, $eu); } } if(isset($remove)) { $nu = implode(",", $eu); echo "$nu"; } echo "<br />"; echo "<br /><a href='project2.php'>Home</a>"; ?> -
I have a table setup that has a column setup as friends and users can add friends to this column no problem. What I would like to know is how to delete only certain parts of the text of the row/cell where their friends are stored. The friends text is seperated by the following : " , " That is a space on either side and comma in the middle of the space. I know how to explode the results and put into an array to display the reuslts, but is there a way to delete only one of the friends if the text is as follows. , thomas , frank , irene , I think you can get the idea. Is it possible to do this or should I just update the whole cell without the array[$i] result being the friend?
-
I was wondering what kind of methods people employ to help to limit the size of their scripts. What is the highest number of lines they would let be in one page before splitting them off into different pages for different functions? I know that some people perfer to try to keep it all in one page, and others perfer many different pages. I guess that it is personal prefernece and related to speed of functions and sript maintainability. I am just curious as to the methods that others employ.
-
$queryString = $_SERVER['QUERY_STRING']; Will set everything after the question mark. You can display it like this: http://grouper.com/video/MediaDetails.aspx?$querystring I don't really understand what you are trying to do, but that is the path you need to be headed down.
-
I started writing code for php3, that was some time ago. I think that most hosting companies are only using "Prebuilt" server software. Mostly they buy a server like we buy a pc, preconfigured or worse, they rent servers from whatever sompany and presto they are a webhost. I think that time presses on and site admins are tired of getting hacked because of session spoofing and sql injection that are easily fixed with functions like sanitize() and so on. My host uses only 4.3 or so, I test on 5, so all my code will just drop into place without any of the added functions. Maybe the smaller hosts will catch up, even some of the big ones are still running 4, but that maybe that demand for it is not that high.
-
$query2 = "SELECT * FROM table WHERE variable = '$variable' ORDER BY filename"; //Would put it alphabetically
-
YOu would have to do a num_rows query similar to this since this is the code I have used on differnet projects and it has not failed me yet, you can use it if you like or change it to suit your needs. <?php if(isset($submit)) { $page = $_GET["page"]; $rowmax = 10; //Set this to the number of items you want returned per page if(!isset($page)) { $page = 1; } if($page == 1) { $pagein = 0; } if($page > 1) { $pagein = $page * $rowmax - $rowmax; } //Below is where the total number of rows will be calculated $query = mysql_query("SELECT * FROM tablename WHERE text LIKE '%$searchterm%'"); $num_rows = mysql_num_rows($query); //Below is where you would do the selected query to draw only paginated results. $query1 = "SELECT * FROM tablename WHERE text LIKE '%$searchterm%' LIMIT $pagein, $rowmax"; $result1 = mysql_query($query1); while($row = mysql_fetch_array($result1)){ //This is the area where the paginated ruslts will be displayed by row results } //Below will only do calutaions if query was successful if($result1 != null) { $pagecount = ceil($num_rows / $rowmax); $resultinput = $pagein + 1; $resultsoutput = $pagein + $rowmax; if($resultsoutput > $num_rows) {$resultsoutput = $num_rows;}//Change $resultsoutput to $num_rows on last page here //Only displays pagination links if necessary below here if ($pagecount > 1) { echo "Page "; $x = 1; $y = $pagecount; for( $i = $x; $i <= $y; $i++ ) { print "<a href='index.php?page=$i'><b>[$i]</b></a> "; } } echo "<p align='center'>There were $num_rows results.<br />//Shows the total number of resutls on this line You are viewing results $resultinput through $resultsoutput.</p>";//shows which results you are viewing here } } ?> I hope this will give you a solid foundation to start from.
-
Try using and serializng sessions to store the selected items. That would probably be the easiest way to store selections across multiple pages then when the script is ended just pull the selected items back out of the sessions array by unserializing them.
-
you should use css and two different td id types like so: In your head do this with a text/css style layout <style type="text/css"> <?php if ($color == "yes") { echo " #yesColor td\{ background-color\: \#eeeeee\; \} "; } ?> </style> And then the table should layout nicely, notice that if no color is chosen the table will have no color at all <tr> <td id ="noColor">10/30/06</td> <td id="yesColor">cy52860231015</td> <tdid="noColor">Deposit</td> <td id="yesColor"> </td> <td id="noColor">$500.00</td> <td id="yesColor"><b>$1,677.65</b></td> </tr>