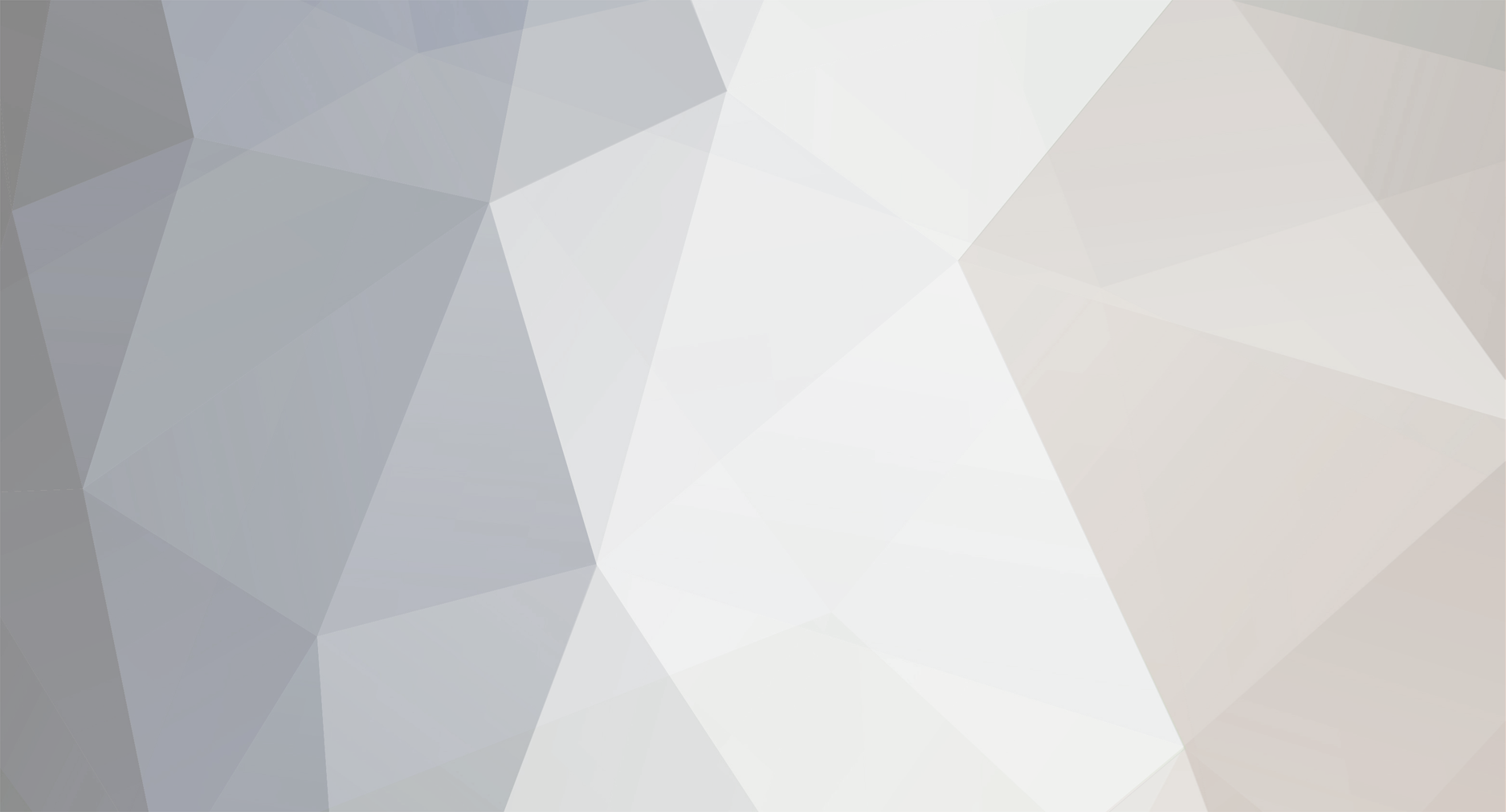
Asheeown
-
Posts
471 -
Joined
-
Last visited
Posts posted by Asheeown
-
-
Welcome to PHP, first off.
Your approach is knowledgeable, however there are better ways of going forth with it.
First lets take a look at
$sql2 = "SELECT userName FROM Users WHERE userName='$_POST[username]' AND passWord='$_POST[password]'";
$sql3 = "SELECT passWord FROM Users WHERE userName='$_POST[username]' AND passWord='$_POST[password]'";
Very good to match the username and password, however you can minimize it to one query and just have it select a count, like so:
$sql = "SELECT count(*) FROM Users WHERE userName='$_POST[username]' AND passWord='$_POST[password]'"; $result = mysql_query($sql); $Count = mysql_result($result, 0); if($Count > 0) { echo "User information is correct"; // You can continue with the login process after this } else { echo "User information is incorrect"; }
Also, when you say you are going to store the username and password in a session variable, it's not really a good idea to do this. You can however store a "logged in" flag in a session variable to tell you that user is logged in, then you can have others, such as username, first name, etc.
-
Make sure you give root a nice bulky password too
-
function tile(){
$tile = "grass";
return $grass;
}
$t = tile();
Why are you returning $grass, that variable doesn't even exist $tile is the actual variable
function tile(){ $tile = "grass"; return $tile; } $t = tile();
-
<?php function update() { $tile = "grass"; return $tile; } echo update(); ?>
-
echo constant("Lang" . $counter);
give that a go
-
Take away the period, I've done it with defining dynamic variables without the period:
echo Lang$counter;
-
Use a div then, I knew span would cause problems
echo "<div style=\"text-align:right\">$Count</div>";
-
<?php $result = mysql_query("select count(*) as rowcount from notes"); $Count = mysql_result($result, 0); echo "<span style=\"text-align:right\">$Count</span>"; ?>
Used your variable before, didn't think you stuck with my way of doing it.
-
To my knowledge I don't think that's possible, to make the link a .png, that's just me of course.
You can save the image but with multiple people using it, it may add up to a lot of images.
-
<?php //get data $button = (isset($_GET['submit'])) ? $_GET['submit'] : "default_value"; $search = (isset($_GET['search'])) ? $_GET['search'] : "default_value"; if (!$button) { echo "You didn't submit a keyword."; } else { if (strlen($search)<=2) { echo "Search term too short."; } else { echo "You searched for <b>$search</b><hr size='1'>"; //connect to our database mysql_connect("localhost","root",""); mysql_select_db("jobjar"); $button = mysql_real_escape_string($button); $search = mysql_real_escape_string($search); //explode our search term $search_exploded = explode(" ",$search); foreach($search_exploded as $search_each) { //construct query $x = 0; $construct = ""; $x++; if ($x==1) { $construct .= " Keywords LIKE '%$search_each%'"; } else { $construct .= " OR Keywords LIKE '%$search_each%'"; } } //echo out construct $construct = "SELECT * FROM jobs WHERE $construct"; $run = mysql_query($construct); $foundnum = mysql_num_rows($run); if ($foundnum==0) { echo "No jobs found."; } else { echo "$foundnum found!<p><br>"; while ($runrows = mysql_fetch_assoc($run)) { //get data $Title = $runrows['Title']; $Location = $runrows['Location']; $Salary = $runrows['Salary']; $Sector = $runrows['Sector']; $JobType = $runrows['Job Type']; $Duration = $runrows['Duration']; $JobRef = $runrows['Job Ref']; $Description = $runrows['Description']; echo "<b>Title:</b> $Title<br> <b>Location:</b> $Location<br> <b>Salary:</b> $Salary<br> <b>Sector:</b> $Sector<br> <b>Job Type:</b> $JobType<br> <b>Duration:</b> $Duration<br> <b>Job Ref:</b> $JobRef<br> <b>Description:</b> $Description<p><br>"; } } } } ?>
As far as I see by glancing at it, only $button and $search are from GET so I did it right under the mysql connect.
-
<?php if(empty($_GET["name"]) || !Isset($_GET["name"])) { echo "Please enter a username."; } else { $username = $_GET["name"]; switch ($_GET[sig]) { case '1': header("Content-type: image/png"); $image = imagecreatefrompng("sig1.png"); //imagecolorallocate($image, R, G, B) in HEX values $font_black = imagecolorallocate($image, 2, 1, ; $font_blue = imagecolorallocate($image, 10, 0, 255); $font_yellow = imagecolorallocate($image, 0xFF, 0xFF, 00); $font_grey = imagecolorallocate($image, 0x5A, 0x5A, 0x5A); $font_red = imagecolorallocate($image, 0xA7, 00, 00); imagestring($image, 5, 200, 10, $username, $font_red); imagepng($image); imagedestroy($image); break; case '2': header("Content-type: image/png"); $image = imagecreatefrompng("sig2.png"); //imagecolorallocate($image, R, G, B) in HEX values $font_black = imagecolorallocate($image, 2, 1, ; $font_blue = imagecolorallocate($image, 10, 0, ; $font_red = imagecolorallocate($image, 0xA7, 00, 00); $font_white = imagecolorallocate($image, 255, 255, 255); imagestring($image, 4, 50, 10, $username, $font_white); imagestring($image, 4, 287, 108, "test", $font_white); imagepng($image); imagedestroy($image); break; default: header("Content-type: image/png"); $image = imagecreatefrompng("sig.png"); //imagecolorallocate($image, R, G, B) in HEX values $font_black = imagecolorallocate($image, 2, 1, ; $font_blue = imagecolorallocate($image, 10, 0, ; $font_red = imagecolorallocate($image, 0xA7, 00, 00); imagestring($image, 4, 50, 10, $username, $font_white); imagestring($image, 4, 287, 108, "test", $font_white); imagepng($image); imagedestroy($image); break; } } ?>
That works for me, you MUST have the images sig.png sig1.png and sig2.png, depending on which one you go for.
sigmaker.php?name=Test - Uses sig.png
sigmaker.php?name=Test&sig=1 - Uses sig1.png
sigmaker.php?name=Test&sig=2 - Uses sig2.png
EDIT: forgot the username definition for the default sig
-
Didn't see you set rowcount, thought you needed a new way to get the count:
echo "<span style=\"text-align:right\">" . $row['rowcount'] . "</span>";
-
First of all make a designated mysql user for that project/database you are using. I am assuming though for now you're using root for development purposes.
Use "mysql_real_escape_string($Variable);" for all the variables that come from user input.
http://us2.php.net/manual/en/function.mysql-real-escape-string.php
-
<?php $result = mysql_query("select count(*) as rowcount from notes"); $Count = mysql_result($result, 0); echo "Number of Members: $Count"; ?>
-
That's exactly what I just said.......
ALL information is being pulled there and without it the script cannot function. That is, where it pulls from the high score list also.
As I said if there is no other place to get the user's information you cannot use this script.
-
$urldata = get_page("http://hiscore.runescape.com/index_lite.ws?player=".$username);
All data for the user is being pulled from here. Obviously the high score list...
If the user doesn't exist on the list and there isn't another page in which the data can be pulled from this cannot be accomplished.
-
Create a thumbnail on upload. Just create it in the sizes you may use. They are thumbnails after all they won't take up that much space.
If one image is only going to be used in one size just make an option to choose which size to create the thumbnail on upload.
Creating thumbnails on the fly creates unnecessary load time, creating thumbnails on upload uses unnecessary space. For me, it'd be space.
-
Have you checked the source?
What's in the desired output is in the file. It's excluding everything wrapped in < >
-
Still excludes all directives inside.
Output:
# Created on Aug 20, 2009ServerAdmin webmaster@test.com
DocumentRoot /home/Test/htdocs
ServerName www.test.com
ServerAlias test.com
AddHandler server-parsed htm html shtml
AccessFileName .htaccess
CustomLog /home/Test/Logs/access_log combined
AllowOverride All
Options Indexes
Options +Includes
ScriptAlias /cgi-bin/ /home/Test/cgi-bin/
Desired Output:
# Created on Aug 20, 2009ServerAdmin webmaster@test.com
DocumentRoot /home/Test/htdocs
ServerName www.test.com
ServerAlias test.com
AddHandler server-parsed htm html shtml
AccessFileName .htaccess
CustomLog /home/Test/Logs/access_log combined
<Directory />
AllowOverride All
</Directory>
<Directory /home/Test/htdocs>
Options Indexes
Options +Includes
</Directory>
ScriptAlias /cgi-bin/ /home/Test/cgi-bin/
-
That works great. Now for my VirtualHosts one, I made one, and like I said, I'm horrible at this.
<?php preg_match_all('/\<VirtualHost [^\*].*?\>.*\<\/VirtualHost\>/s', $Contents, $vhost_matches); ?>
Only problem is it takes away every directive, starting with <VirtualHost> they just don't show up in the output. There are other directives inside <VirtualHost> such as:
<Directory>
<Location>
And things of that nature, any idea of why that is happening?
-
I started working off what you gave me and I got it:
<?php preg_match_all('/NameVirtualHost [^\*].*:.*\r/', $Contents, $vhost_matches); ?>
Produces just what I need.
Now I just have to figure out the virtualhosts
-
preg_match_all('#<NameVirtualHost (.*?)>#', $Contents, $vhost_matches);
Produces two empty arrays within $vhost_matches
If I take away the # symbols in the pattern I get three values $vhost_matches[0] $vhost_matches[1] $vhost_matches[2] but all they have in them is "NameVirtualHost"
-
So I am making a linux migration script and my third step is to transfer all the VirtualHost directives and the NameVirtualHost to the new machine.
For the NameVirtualHost assignment I need to get any and all lines that look like this:
NameVirtualHost 192.168.1.170:80And save them in an array so I can add them to the new server, there are usually only one of those entries but multiples can occur
For the VirtualHost directives I'll give an example below
<VirtualHost 192.168.1.170:80>
# Created on Aug 20, 2009
ServerAdmin webmaster@test.com
DocumentRoot /home/Test/htdocs
ServerName www.test.com
ServerAlias test.com
AddHandler server-parsed htm html shtml
AccessFileName .htaccess
CustomLog /home/Test/Logs/access_log combined
<Directory />
AllowOverride All
</Directory>
<Directory /home/Test/htdocs>
Options Indexes
Options +Includes
</Directory>
ScriptAlias /cgi-bin/ /home/Test/cgi-bin/
</VirtualHost>
I figured since the VirtualHost directive is always the same, but the IP may be different it shouldn't be too hard. However, I never got into regex and I can't figure out how to get these things and store them in variable for later use.
-
I haven't really gotten a successful execution of scp_recv, and it's because I don't really understand how to use it. It only allows for one ssh connection handler but I have two, one for each server, so which connection handler do I run it for the source or destination. Also, what would I do for the paths to get the file from the other server. Like if I used the source connection handler to run scp_recv how would I tell it where to put the file on the remote server?
Paypal Integration Question.
in PHP Coding Help
Posted
Check this site out. This guy made a complete php mysql paypal shopping cart. If you aren't interested in the shopping cart part just see how he setup the payment pages. Also, for your testing needs paypal has a sandbox website that you can setup to test any scripts you may make. http://sandbox.paypal.com
http://www.phpwebcommerce.com/