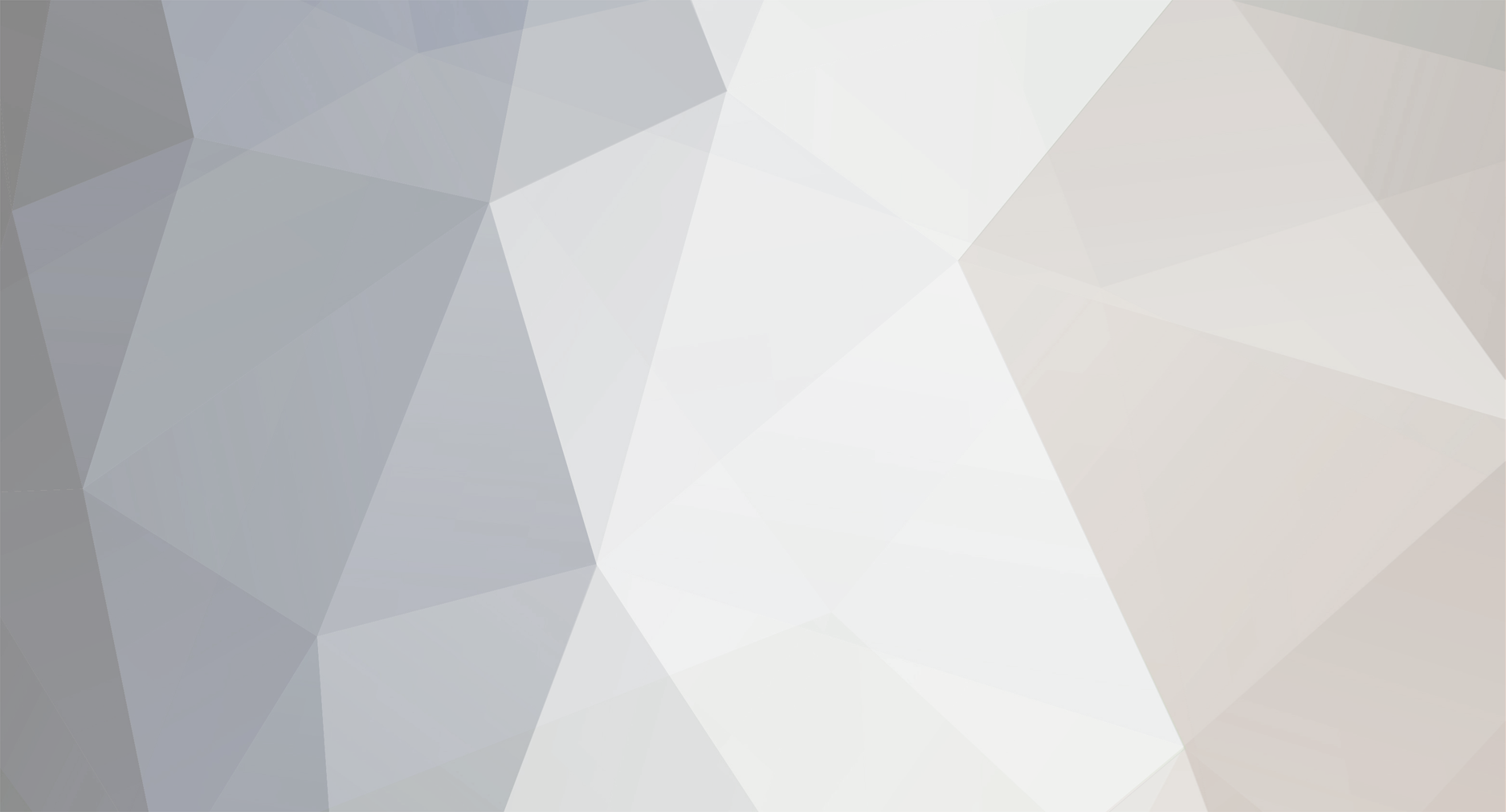
Asheeown
-
Posts
471 -
Joined
-
Last visited
Posts posted by Asheeown
-
-
I tried that, when my news post comes up it doesn't fall down to the next line when it hits the end of the box it's in because the whole string is one line "word1 word2 word3 word4" etc.
Any other ideas?
-
I have text that gets echoed out as news posts, and some of them have lists like this:
- Category- Option 1
- Option 2
But it comes out like this
- Category- Option 1
- Option 2
Any suggestions on how I can get it to actually space it right?
-
Ah, damn, I was trying to avoid rewriting all of them or have to add a line to every option.
Looks like I'm making a big array
thanks for your help
-
Only going to post a few of the select options as to not load the screen up, here are the two, summarized.
<select name="Make" style="width: 90px"> <option value="542">Abarth</option> <option value="457">AC</option> <option value="58">Acura</option> <option value="16">Alfa Romeo</option> <option value="456">Allard</option> <option value="609">Allstate</option> <option value="486">Alpine</option> <option value="501">Alvis</option> <option value="66">American Motors</option> <option value="44">AM General</option> <option value="550">Amphicar</option> </select> <select name="CarModel" style="width: 90px"> <option value="973">100 (Car)</option> <option value="2143">100 (Truck)</option> <option value="4719">1000 (Car)</option> <option value="6294">1000 (Medium Duty)</option> <option value="6264">1000A</option> <option value="2144">1000B</option> <option value="2145">1000C</option> <option value="2146">1000D</option> </select>
EDIT: Rhodesa, they are all static :\ wasn't my choice
-
I am doing error reporting on a page, and it's a submit page for information. There are two select drop down boxes, with hundreds of options in each.
Now if they choose one of those correctly but do not fill out a required field it will redirect them back and tell them "One of the required fields is not correctly filled in"
I don't want the user to have to search back through those boxes again, is their a more simple way of selecting the one that the user selected before using the value in the $_POST variable?
-
Remove the
<base target="I1" />
from your HTML since you're not using frames anymore. Also remove any other target attribute you may have.
Amazing, that's exactly it, and fixed, thanks very much. Was bugging the hell outta me.
-
No it's not supposed to...firefox doesn't open a new page, nothing is forcing it to open in a new page.
-
Alright, I have a page that in plain HTML works fine in both firefox and internet explorer. The HTML version uses IFrames.
Now, in PHP I took it and made it to just a plain "include()" the basic pages. It works perfectly in firefox all the links, login system. However, in IE6 and IE7, when you click ANY link it opens it in a new page, now it only opens one new page. All the rest of the links clicked are opened in that first new page and any links clicked INSIDE the new page opens up fine.
Check it out for yourself
http://pointcomfort.lhhost.com
Check it in firefox and then IE
-
No, that's completely different.
Alright, how do you get the last (highest) record of an ID column if they're all intergers.
-
Okay, so I am making a script for an emulated gaming server which uses a standard MySQL server for all it's data. Now this is a mailer system and this is going to create and send items.
What it does:
1. Gathers a comprehensive list of everyone in the game it's going to send to (DONE)
2. Inserts a new item into the database (DONE)
3. Inserts a record into the mail table with the item ID added in step 2 and the UserID from step 1 (Partially Done)
Now all the coding is done except for ONE thing on step 3
The ID field in the mail table for step 3 is not auto_increment and the structure cannot be changed, what I need it to do is pull the absolutely last (biggest) ID number in the mail table and increase it by one and if no records exist make the ID 0
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Dynasty Mailer</title> </head> <body> <?php $Host = "localhost"; $User = "xxxxxx"; $Password = "xxxxxx"; $conn = mysql_connect('localhost', $User, $Password); $CharacterDB = "fun_characters"; $ItemID = "100101"; $Subject = "A Gift From Dynasty!"; $Body = "This is the message sent for the user."; mysql_select_db($CharacterDB); ?> <?php $CharacterQuery = mysql_query("SELECT * FROM characters"); while($Characters = mysql_fetch_assoc($CharacterQuery)) { extract($Characters); // Create Item $Insert = mysql_query("INSERT INTO playeritems (ownerguid, entry, randomsuffix, enchantments) VALUES('0', '$ItemID', '', '')"); $Item = mysql_insert_id(); // Mail Item if(!$message_id) { $MailCount = mysql_query("SELECT message_id FROM mailbox ORDER BY message_id desc"); if(mysql_num_rows($MailCount) == 0) { $message_id=0; } else { $MailCount = mysql_fetch_assoc($MailCount); extract($MailCount); echo("<center><strong>Item: $ItemID mailed to $guid.</strong></center>"); $message_id = $message_id+1; } } else { $message_id++; echo("<center><strong>Item: $ItemID mailed to $guid.</strong></center>"); } $Mail = mysql_query("INSERT INTO mailbox (message_id, player_guid, sender_guid, subject, body, attached_item_guids) VALUES ('$message_id', '$guid', '1', '$Subject', '$Body', '$Item')") or die(mysql_error()) ; } // End of Characters ?> </body> </html>
Okay right now under the Mail Item comment I have no idea where I was going with this, everything else is fine just getting $message_id from what is currently in the database "message_id" is the column in which the ID is put
$guid = User ID
$Item = Item ID
-
</form>'; } else echo 'testing';
see that, even though it's an else it has to be
</form>'; } else { echo 'testing'; }
-
The code would look like
<?php $sec="5"; echo $_POST[message]."<br><br><b>You will be redirected to $_POST[redirecturl] in 5 seconds</b>"; echo '<meta http-equiv="refresh" content="'.$sec.';url='.$_POST[redirecturl].'"/>'; ?>
What this means is that it takes the message posted in the form $_POST[message] and your redirect url "$_POST[redirecturl]" and makes a text display...for example
This is the users message that will be posted hereYou will be redirected to www.domain.com/somefile.php in 5 seconds
Then it redirects them in 5 seconds
-
He probably wants it to be public
Dysan that is all DNS, virtual hosts can be setup for local use just like MadTechie shows, however for it to be public and everyone to see a DNS record for say subdomain.mydomain.com must be pointed in the dns records of your host to your server.
-
Well it's pretty simple just do one after the other, once one is finished, send the other one, here's an example
<?php // Contact Subject $Subject = "This is your subject to your user"; // Contact Message $Message = "This is your email message to your user, include the name, username, and even password"; // Email that you are sending from $From = "no-reply@mydomain.coml"; // From $Header="from: $From"; // Your users email address $To = $UserEmail; $MailUser=mail($To,$Subject,$Message,$Header); // Check to see if that email was successful if($MailUser) { // Success now start our personal mail to notify us // Subject to yourself $Subject = "New User Registered"; // Message to yourself $Message = "A new user has been registered under the name of: $Name...blah blah blah blah blah"; // Email that you are sending from $From = "no-reply@mydomain.coml"; $Header="from: $From"; // Your email address $To = "new-registrations@mydomain.com"; $Mail=mail($To,$Subject,$Message,$Header); // Check to see if THIS mail was successful if($Mail) { echo "Mail sent successfully"; } else { echo "Something went wrong in the mailer"; } } else { echo "Something went wrong in the mailer"; } ?>
I didn't test this code, I just wrote it, so test it and get back to me
-
Everything seems to be okay, what is the problem with it?
Plus, for user confidentiality I wouldn't send a copy of the email with their private information in it to your email. Send two emails, one to the user containing his/her information and another to yourself at a private account notifying you of a new user.
-
I'm trying to write a dynamic content script for a web server (not mine) and it works on mine, my machine is a Fedora Core 8 system Apache MySQL/PHP. The system I am doing the script for is a shitty Windows 2000 IIS w/ Front Page extensions.
What measures need to be taken to prep. the Windows 2000 server to accept AJAX?
-
It was the most simple thing, I'm sorry it was sitting in front of me the whole time, just every guide helping me set it up was skipping ahead and assuming I had already installed it and I couldn't find the core for PEAR. But I finally found a guide to do it and it worked.
-
Warning: id3_get_tag() [function.id3-get-tag]: ID3v2 tag contains invalid padding - tag considered invalid in /home2/Music/htdocs/test.php on line 2
WTFFFFF, I have no idea what it even means, I haven't seen the error before, I've used so many variations of this string as possible but it just doesn't go away, any hints?
$tag = id3_get_tag( "Music/song_03.mp3", ID3_BEST ); print_r($tag);
Above is one of the last ones I tried ID3_BEST was suggested after throwing an invalid version ID into the mix. Below is the first I have tried:
$tag = id3_get_tag( "Music/song_03.mp3", ID3_V2_3 ); print_r($tag);
EDIT: It does still execute the command, just shows that error,
-
I need help installing PEAR with PECL support on my linux server, merged in with my current version of php, 5.2.4.
-
If so many programs can extract that one certain part of the song details why can't a web based language do it? Anyone have any ideas at all?
-
I am building a media player and when I upload the songs is their anyway to read the deep information of the file like album name, artist, or duration. I mostly need it for track numbers though, so if it even was for that, it would be great.
-
<?php function csv_array($file,$delim) { $fh=fopen($file,"r"); while(!feof($fh)) { $line=fgets($fh); $row=explode($delim,$line); foreach($row as $key => $val) { if(($ch=substr($val,0,1))=='"' || $ch=="'") { // we got a string delimeter $skey=$key+1; do { $sl=strlen($val); if(substr($val,$sl-1,1)==$ch) break; // we got a matching delimeter $val .= $row[$skey]; unset($row[$skey++]); } while(true); $sl=strlen($val); $row[$key]=substr($val,1,$sl-2); // remove the quote delimeter } } $newrow[]=$values($row); // Rebuild our array removing empty sets (ones we removed with unset); LINE 23 } fclose($fh); return $newrow; } $Array = csv_array("Spells.dbc.csv",","); print_r($Array); ?>
Gives me:
Fatal error: Function name must be a string in /home/Development/htdocs/Php/SpellMaker/test.php on line 23I specified what line 23 is in the code.
If I change "$newrow[]=$values($row);" to "$newrow[]=$values[$row];" or "$newrow[]=$val[$row];" it still gives me other errors or doesnt set the array with any values, 11 fields show in the array though, that is how many are in the csv file, just nothing set to them
-
This wouldn't work to begin with, when I do the command fgetcsv I use the delimiter "," and it has to be that way and that's when the columns get added.
-
I'll try it out, I don't know if the text is wrapped in quotes it's a normal csv file.
Socket Connection Help
in PHP Coding Help
Posted
Okay let me start off by saying what I am trying to accomplish here.
I am building a website to monitor a program that offers a socket connection and a list of commands to operate the program remotely.
The program offers many informational commands that tells the status of what's going on. It also offers a log command which is what brings me to this.
In my program I start up my connection to the program and enter in my password...if all is well, continue.
I enter in a status command and extract the information from it:
Everything is PERFECT, the status of the program is posted in front of the user...now here is the tricky part
The command to log all information, which I would like to use, is "/log all". Now when that command is initiated all logged information is posted in the socket connection window (I tried this with a windows telnet client and saw all of it).
The goal I am trying to accomplish is to get that information asynchronously as to not kill the socket connection.
Any ideas? If anymore explanation is required just let me know.