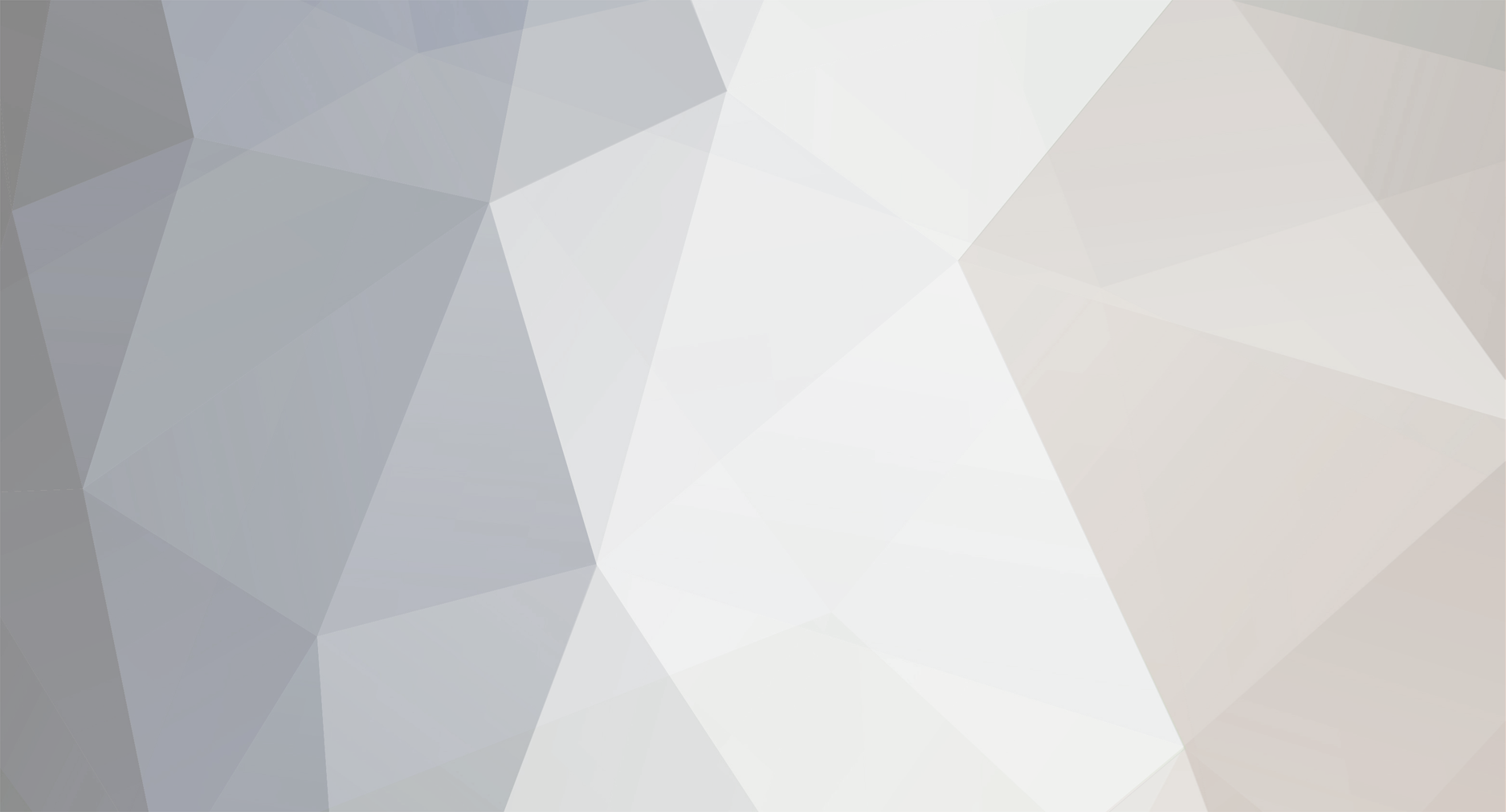
s0c0
Members-
Posts
422 -
Joined
-
Last visited
Never
Everything posted by s0c0
-
The traditional approach would have been to have the client continually poll the server looking for updates. There are new ways where the server can tell the client there has been an update such as http://www.ape-project.org/ and many many others.
-
ManiacDan is correct here. I'd just like to point out that your code is vulnerable to SQL injection, here is a better option for the authentication portion: <?php include "setup.php"; /* establish a connection with the database */ $server = mysql_connect("$db_host", "$db_username","$db_password"); if (!$server) die(mysql_error()); mysql_select_db("$database"); /* get the incoming ID and password hash */ $username= mysql_real_escape_string($_POST['username']); $password= mysql_real_escape_string($_POST['password']); $password=md5($password); // Encrypted Password /* SQL statement to query the database */ $query = "SELECT * FROM users WHERE Username = '$username' LIMIT 1"; /* query the database */ $result = mysql_query($query); $r = mysql_fetch_assoc($result); if($r['Password'] == $password){ echo "Access Granted: Welcome, $username!"; } else{ echo "Access Denied: Invalid Credentials."; } ?> This is better because it escapes some bad characters using mysql_real_escape_string (still not completely secure). Also it forces them to match only the username from the database and then that username record must match the supplied password. Otherwise I could have hacked into the system sending the following inputs: username: ' OR 1='1 password: ' OR 1='1
-
I'm trying to create a trigger that updates a field each time another field in the same table is changed. I created a numerical hash of a varchar column for increasing speed on lookups and group by operations. However, I get the following error: It looks like MySQL is preventing the trigger from executing because it would create an infinite loop. Here is my trigger syntax: delimiter $$ CREATE TRIGGER pHash AFTER UPDATE ON products FOR EACH ROW BEGIN UPDATE products SET model_hash = crc32(products_model); END$$ delimiter ; Any idea on how I could accomplish this? Doing this in the code is not an option because we have a very old code base where updates occur all over the place, not very OOP at all so this must occur within the database engine.
-
It all has to do with PCI compliance and the above poster is correct that you probably don't want to deal with this. Instead you can use a service like authorize.net or merchant e-solutions and have them store the credit card for you. Alls you would need to store at that point is a token referencing the securely stored credit on their system. I designed a system like this before and to be even more secure I even encrypted the token using a two-way cypher: http://www.phpclasses.org/package/6264-PHP-Encrypt-and-decrypt-data-using-Rijndael-256-cypher.html. With that said I worked for an employer once and my boss didn't care much for the safety of the customers financial data. We stored the credit card information using a custom written two-way cypher and probably still do to this day. If I were the decision maker, its not a decision I would make. You wouldn't want your data to be stored in a non-secure non-PCI compliant manner, would you? https://www.pcisecuritystandards.org/
-
This is a parse error meaning you did not close a string properly or were missing a ";" from your code. You will want to write cleaner code so it is easier to debug this little things. I suggest using a full blow IDE to help you debug things like this. Alls I did was throw your code into netbeans and it told me where the errors were...I think reformatted your code to be easier on the eyes. echo '<form name="form3" method="post" action=""> <select name="menu2" onChange="MM_jumpMenu(\'parent\',this,0)" class="textbox"> <option value="">Please choose a town!</option>'; while($row1 = mysql_fetch_array($townresult)){ echo '<option value="pubs_norm1.asp?rsTown='.$row1['RSTOWN'].'"> Pubs and bars in '.$row1['RSTOWN'].$row1['RSTOWN'].'</option>'; } echo '</select></form>';
-
I program on my local box and my staging server is on shared hosting account. Will SVN up work with this type of setup?
-
What is the best way to get a list of files that have changed/committed since a certain point in subversion/svn? Here's what I am trying to accomplish. When I start a new project or release for a client I want to get a list of only what has been committed and then deploy that to the my staging server. What I've been doing is just rolling the entire PHP project via FTP which takes time. Maybe I start a branch/tag each time? Anyways, I'm sure someone on here does something similar to this or maybe even has a better idea.
-
I fixed this by creating a new virtual host and telling apache to listen on a new port. in /etc/apache2/sites-available/default: <VirtualHost *:8080> ServerAdmin webmaster@localhost ServerName 127.0.0.1 DocumentRoot /var/svn/client ErrorLog /var/log/apache2/error.log # Possible values include: debug, info, notice, warn, error, crit,alert, emerg. LogLevel warn CustomLog /var/log/apache2/access.log combined </VirtualHost> in /etc/apache2/ports.conf NameVirtualHost *:80 NameVirtualHost *:8080 Listen 80 Listen 8080
-
I will want to programatically input selected for a given option. Here's some quick example code: <? $monthArr = array(1,2,3,4,5,6,7,8,9,10,11,12); echo '<select name="month">' foreach($monthArr as $i){ echo '<option value="'.$i.'" '.(($_POST['month']==$i)?'selected':'').'>'.$i.'</option>'; } echo '</select>'; ?>
-
This might be better for the Apache forum, but I'll explain anyways. I'm switching up my local dev environment so I can use SVN. I have a directory for all my SVN stuff on my local machine now in /var/svn. When I call $_SERVER['DOCUMENT_ROOT'] its listing the document root as /var/www. For this alias I've setup in apache I want the document root to be /var/svn/myproject. Alot of my includes are failing because of this now.
-
Thats a pretty small query. I think you're fine. One practice I've taken to making my queries easier on the eyes is formatting them like so: SELECT table1.id, table1.review, table1.time, table2.author, table2.title FROM table1, table2 WHERE table1.id = table2.id AND table1.reviewer = '{$username}' ORDER BY table1.id
-
I believe the point of my post is being missed by those responding.
-
Agreed, however queries will still be doing look ups against the varchar value even with this change and not the primary_key. No time to change that much code and test it all at this point. Just wanting to know if this is worth the change.
-
mikosiko won't do the job because you are not storing the user id in the web_messages table. Just an FYI, in the messages table you should store users by the web_users.id rather than username. Searches on text take longer and you're having to store more data that way. Just sayin'. See if this works for you: SELECT web_users.*,web_messages.* FROMweb_users LEFT JOIN web_messages ON web_users.username = web_massages.userGROUP BY web_users.id ORDER BYweb_messages.time DESC else you might have to sub select: SELECT web_users.*, (SELECT web_messages.id FROM web_messages WHERE web_users.username = web_messages.user ORDER BY time DESC LIMIT 1) as web_massages_id FROMweb_users
-
We have a varchar(32) serving as a primary key. I know this is bad and we will eventually rewrite the code and redesign the database schema. Luckily the rows in the table never get more than about 1,000 since it just stores session data. For now I am wondering if I would see any performance increases by switching to an auto-increment int(10) primary key and switching the varchar to a unique index. Also wondering if MyISAM would store less meta data in the MYI file. So it would go from this: sessionkey | varchar(32) primary key unixtimestamp | int(11) unsigned data | text To this: session_id | int(10) primary key auto inc sessionkey | varchar(32) unique unixtimestamp | int(11) unsigned data | text Thoughts?
-
IMHO its very true. Always explore other options if you find yourself writing sub queries they are obviously slower and the Big O proves it and try to avoid LEFT JOINS because it analyzes more than you may need, but hey I'm not looking to get into a debate.
-
The sub select should be avoided at all cost as they are very slow. Left Join should be avoided as well. Please post the schema of your table.
-
Try SELECT * FROM events WHERE event_date > CURRENT_DATE() This is a very basic question, you should really go grab a book on PHP/MySQL if you're going to be programming a lot. *edit* I just noticed you're using a unixtimestamp. You'll need to look up how to convert the current_date into a unixtimestamp. There should be a mysql function that does this, you can use PHP as well. Beyond that the SQL I gave you will work, just figure it out.
-
You could attempt using a UNION. A union combines to querries and returns them in the same result set. However, the columns must be the same. You could fake this by creating aliases from the columns. You know like "column AS columnAlias. http://dev.mysql.com/doc/refman/5.0/en/union.html Your other option is programatically to merge the arrays into one with whatever language you're using.
-
Have you considered using an INT(10) or even a BIGINT(19). Also ensure your integer values are unsigned as this gives more positive integers to work with, but disallows negative integers. http://dev.mysql.com/doc/refman/5.0/en/numeric-types.html I'm not sure about recycling IDs. It depends on a lot of things, I wouldn't do it, but I'll allow someone else on here to comment on that.
-
How much dev rosources do you need to run two ALTER TABLE queries? Ok I understand that some testing needs to be done beforehand, but moving to InnoDB when you don't need full text searches (and it doesn't look like you do) is next to trivial, and you get rid of those crashes immediately and forever. We will move to InnoDB if complete drop/create of the tables does not fix the issue. InnoDb is known to be slower than MyISAM, but the nominal drop in performance is worth the uptime. That doesn't need dev time, when I think of InnoDB I think of using foreign keys and referential integrity which would cause significant resources to fix bad data. Obviously just running an alter statement can be done very quickly. We are also having a hardware diagnostic performed.
-
We have run repair on these tables multiple times in the past two weeks and they continue to periodically crash.
-
fenyway - Then we will just completely DROP and rebuild from the mysqldump file. ignace - Innodb is not an option for us. Our data structure is in bad condition and lots of dev resources would be needed.
-
Just include the names of the hidden fields in your cURL post parameters. I've never done this, but it should work. Do you not know how to see the names of the hidden post fields? If not the web developer plugin for firefox is great or just view source and search.