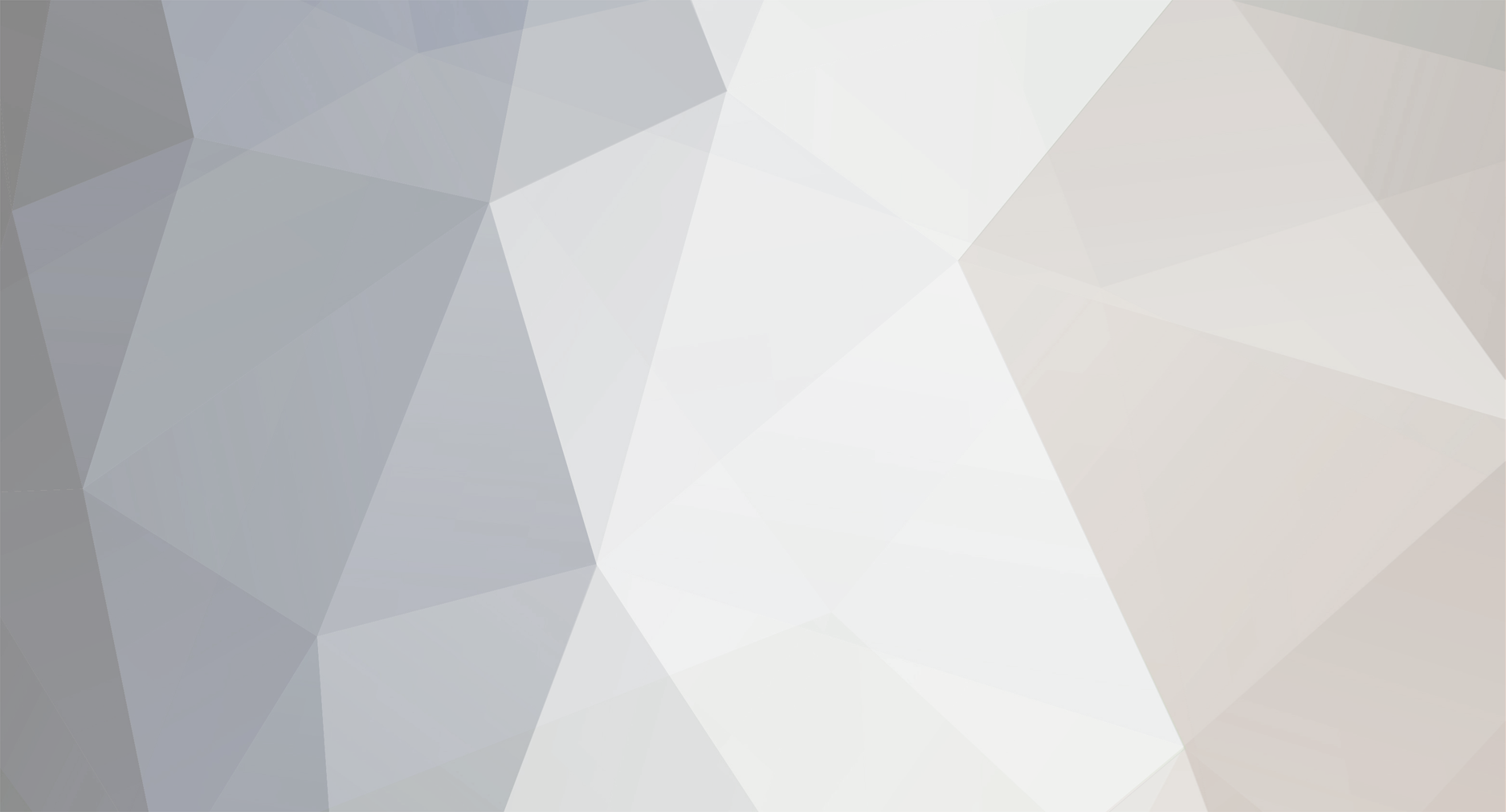
only one
-
Posts
437 -
Joined
-
Last visited
Never
Posts posted by only one
-
-
first page:
<html> <head> <title>PHP Test</title> </head> <body> <?php echo '<p>Hello World</p>'; ?> <form action="/processForm.php" method="post"> Enter youre name <input type="text" name="userName"> <input type="submit" name="submit"> </form></body> </html>
second page:
<html> <head> <title>PHP Test</title> </head> <body> <?php $userName = $_GET['userName']; print "hi $userName"; ?></body> </html>
or
firstpage:
<?php session_start(); ?> <html> <head> <title>PHP Test</title> </head> <body> <?php echo '<p>Hello World</p>'; ?> <form action="/processForm.php" method="get"> Enter youre name <input type="text" name="userName"> <input type="submit" name="submit"> </form></body> </html>
second page:
<?php session_start(); ?> <html> <head> <title>PHP Test</title> </head> <body> <?php $userName = $_GET['userName']; print "hi $userName"; ?></body> </html>
-
http://www.tutorio.com/tutorial/simple-and-complex-bbcode-with-php
check that, should explain quite a bit i hope
-
thats the problem with this, it is nearly impossible unless you make all the ways its possible which would take you forever :-\
-
<?php function emoticon($post) { $emoticonarray = array( '' => 'smile.gif', '' => 'sad.gif', '' => 'wink.gif', '' => 'tongue.gif', '' => 'cheese.gif' ); foreach($emoticonarray as $emoticon => $img) { $search[] = $emoticon; $replace[] = '<img src="http://lordoftheabyss.com/images/emotions/' . $img . '" alt="' . $emoticon . '" />'; } $post = str_replace($search, $replace, $post); return $post; } function bbcode_format($post) { $post = htmlentities($post); $simple_search = array('/(\[b\])(.*)(\[\/b\])(\[u\])(.*)(\[\/u\])(\[i\])(.*)(\[\/i\])/' ); $simple_replace = array('<strong>${2}</strong><u>${3}</u><i>${4}</i>'); // Do simple BBCode's $post = preg_replace ($simple_search, $simple_replace, $post); return $post; } ?>
this will only work for the [ b ][/b ][ u ][/u ][ i ][/i ] order
-
try using an echo "$userName";
or print ("$userName");
o yea, the form action should probobally be post, or you could put session_start(); at the top of the page (in php tages)
-
example
$bbcode = array('/(\[url=)(.*)(\])(.*)(\[\/url\])/'); $html = array( '<a href="http://${2}" target=_blank>${4}</a>'); $output = preg_replace($bbcode, $html, $output);
if you want it to red you [ b ]text[/b ][ u ]text[/u ] [ i ]text[/i ]
then you have to do: $bbcode array('/(\[b\])(.*)(\[\/b\])(\[u\])(.*)(\[\/u\])(\[i\])(.*)(\[\/i\])/'); $html = array('<strong>${2}</strong><u>${3}</u><i>${4}');
hope that makes sense
-
hey thx, id never have known
-
<table> <tr> <td style= align="center" width="250" height="20"> <font face=arial color=#000000 size=1><div id="message_html" class="messagehtml" }>Please enable javascript</div></font> </td> </tr> </table> <script language='Javascript'> setup=2; function countdown() { if ((0 <= 100) || (0 > 0)) { xsetup = setup--; if(xsetup == 0) { if (0) document.getElementById("messagehtml").innerHTML = 'blah'; if (0) document.getElementById("message_html").innerHTML = '<?php update here ?>'; countdown(); } if(xsetup > 0) { document.getElementById("message_html").innerHTML = '+xsetup+''; setTimeout('countdown()',1000); } } } countdown(); function SetCookie(cookieName,cookieValue,nHours) { var today = new Date(); var expire = new Date(); if (Hours==null || nHours==0) Hours=1; expire.setTime(today.getTime() + 3600000*Hours); document.cookie = cookieName+"="+escape(cookieValue) + ";expires="+expire.toGMTString(); } </script>
this hasnt been tested!
-
-
lol simple html, you need to give your iframe a name...
-
id advise using cookies if your going to send it too a different window
or you could have the popup url have the variables as example.php?valriable=examlpe....
-
here
$display=555-555-5555;
$bbcode = array('.', '-'); $html = array( '', '' ); $display = preg_replace($bbcode, $html, $display);
now $display will echo 5555555555
-
explain in more detail?
if your serves forward an hour just minus 1 from the hour...
-
try this, youl have to make a folder called uploads, in the folder where this page is saved
<?php $path = "uploads/"; if (!isset($HTTP_POST_FILES['userfile'])){ echo " <FORM ENCTYPE=multipart/form-data ACTION='' METHOD=POST> <p>The file:<br> <INPUT TYPE=file NAME=userfile> </p> <p> <INPUT TYPE=submit VALUE=Upload> </p> </FORM> "; }else{ if (is_uploaded_file($HTTP_POST_FILES['userfile']['tmp_name'])) { if (($HTTP_POST_FILES['userfile']['type']=="audio/mp3")")) { if (file_exists($path . $HTTP_POST_FILES['userfile']['name'])) { echo "<font color=red>Error</font>! The file already exists, try calling your file a different name.<br><FORM ENCTYPE=multipart/form-data ACTION='' METHOD=POST> <p>The file:<br> <INPUT TYPE=file NAME=userfile style='border: 1px solid #222222; background-color: #111111; color: #888888'> </p> <p> <INPUT TYPE=submit VALUE=Upload style='border: 1px solid #222222; background-color: #111111; color: #888888'> </p> </FORM>"; }else{ $res = copy($HTTP_POST_FILES['userfile']['tmp_name'], $path . $HTTP_POST_FILES['userfile']['name']); if (!$res) { echo "upload failed!<br>"; exit; } else { echo "upload sucessful<br><br>"; } echo "File Name: ".$HTTP_POST_FILES['userfile']['name']."<br>"; echo "File Path: <a href=uploads/".$HTTP_POST_FILES['userfile']['name']." TARGET=_BLANK>uploads/".$HTTP_POST_FILES['userfile']['name']."</a><br>"; echo "File Size: ".$HTTP_POST_FILES['userfile']['size']." bytes<br>"; echo "File Type: ".$HTTP_POST_FILES['userfile']['type']."<br>"; echo "<img src=uploads/".$HTTP_POST_FILES['userfile']['name']."><br>"; }}}} ?>
-
if that doesnt work just use an if under your select function
if(parent_id = $forums){
you knwo your variables better than me :-\
-
try:
$query2 = "SELECT title,tid,start_date,last_post FROM ibf_topics WHERE (forum_id = '$forums') AND (parent_id = '$forums') AND (start_date > '$beginstamped') AND (start_date < '$endstamped') ORDER BY start_date DESC";
?? you dont make much sense
-
check your webhost, does it say it allows the mail() function?
if its freehostia or awardspace your mail() functions wont work
-
it depends on the server time, if your servers set to the same time as GMT the time on your webstie will be aswell,
visit
this will have all your date and time functions
hope that solves your problem
-
check phpinfo(); to see if you have the mail(); function enabled
-
hi im seting up a fishing function for my rpg, this is my first javascript porject ive ever done, ive learned the basics (i think)
the only problem im having is the reelback(); function,
everytime i click to go to it, it has an error and doesnt work.
anyone have any ideas?
<table> <tr> <td style= align="center" width="250" height="20"> <font face=arial color=#000000 size=1><div id="message_html" class="messagehtml" }>Please enable javascript</div></font> </td> </tr> </table> <script language='Javascript'> var setup=10; var casting=5; var reelback=10; var waiting=20; function countdown() { if ((0 <= 100) || (0 > 0)) { xsetup = setup--; if(xsetup == 0) { if (0) document.getElementById("messagehtml").innerHTML = 'you set up your line'; if (0) document.getElementById("message_html").innerHTML = 'you set up your line'; cast(); } if(xsetup > 0) { document.getElementById("message_html").innerHTML = 'seting up line '+xsetup+''; setTimeout('countdown()',1000); } } } countdown(); function cast() { if ((0 <= 100) || (0 > 0)) { xcasting = casting--; if(xcasting == 0) { if (0) document.getElementById("messagehtml").innerHTML = 'you set up your line'; if (0) document.getElementById("message_html").innerHTML = 'you cast out your line'; document.getElementById("message_html").innerHTML = 'wating for bite<br><br><a class="messagehtml" onclick="reelback();"><u>check bait</u></font></a>'; } if(xcasting > 0) { document.getElementById("message_html").innerHTML = 'casting out line '+xcasting+''; setTimeout('cast()',1000); } } } function reelback() { if ((0 <= 100) || (0 > 0)) { xreelback = reelback--; if(xreelback == 0) { if (0) document.getElementById("messagehtml").innerHTML = 'you set up your line'; if (0) document.getElementById("message_html").innerHTML = 'you cast out your line'; document.getElementById("message_html").innerHTML = '<a class="messagehtml" onclick="countdown();">Replace bait</font></a>'; } if(xreelback > 0) { document.getElementById("message_html").innerHTML = 'reeling in line '+xreelback+''; setTimeout('reelback()',1000); } } } function wait() { if ((0 <= 100) || (0 > 0)) { xwait = wait--; if(xwait == 0) { if (0) document.getElementById("messagehtml").innerHTML = 'you set up your line'; if (0) document.getElementById("message_html").innerHTML = 'you cast out your line'; document.getElementById("message_html").innerHTML = 'You caught one!!<br><br><a class="messagehtml" onclick="countdown();"><u>Try again</u></font></a>'; } if(xwait > 0) { document.getElementById("message_html").innerHTML = '<br>'; setTimeout('wait()',1000); } } } function SetCookie(cookieName,cookieValue,nHours) { var today = new Date(); var expire = new Date(); if (Hours==null || nHours==0) Hours=1; expire.setTime(today.getTime() + 3600000*Hours); document.cookie = cookieName+"="+escape(cookieValue) + ";expires="+expire.toGMTString(); } </script>
-
no ones ever on that board...
-
im pretty new to javascript, im creating a fishing function, but theres a problem with my second countdown
<table> <tr> <td style= align="center" width="250" height="23"> <div id="message_html" class="messagehtml" style="font: Arial; font-weight: normal; font-size: 13px; color: black; }">Please enable javascript</div> </td> </tr> </table> <script language='Javascript'> setup=10; function countdown() { if ((0 <= 100) || (0 > 0)) { setup--; if(setup == 0) { if (0) document.getElementById("messagehtml").innerHTML = 'you set up your line'; if (0) document.getElementById("message_html").innerHTML = 'you set up your line'; document.getElementById("message_html").innerHTML = '<a class="messagehtml" onclick="cast();">Cast you line</font></a>'; } if(setup > 0) { document.getElementById("message_html").innerHTML = 'seting up line '+setup+''; setTimeout('countdown()',1000); } } } countdown(); function cast() { document.getElementById("message_html").innerHTML = 'casting out line'; casting=5; function countdown2() { if ((0 <= 100) || (0 > 0)) { casting--; if(casting == 0) { if (0) document.getElementById("messagehtml").innerHTML = 'you cast out the line'; if (0) document.getElementById("message_html").innerHTML = 'you cast out your line'; document.getElementById("message_html").innerHTML = '<a class="messagehtml" onclick="check();">check bait</font></a>'; } if(casting > 0) { document.getElementById("message_html").innerHTML = 'casting out line '+casting+''; setTimeout('countdown2()',1000); } } } countdown(); } function SetCookie(cookieName,cookieValue,nHours) { var today = new Date(); var expire = new Date(); if (nHours==null || nHours==0) nHours=1; expire.setTime(today.getTime() + 3600000*nHours); document.cookie = cookieName+"="+escape(cookieValue) + ";expires="+expire.toGMTString(); } </script>
my countdown2 function isnt countingdown :-\
-
google helps if you go to the 300th page, somethimes
-
i think ill explain in more detail....
say your fishing levels 1
then it randomises between 10-15 seconds to set up your line etc...
then i use javascript to countdown the random number between 10-15
now i want it to echo the next bit like echo you cast out your line, then they wait more to catch a fish depending on the level,
i want to do this without refreshing the page, or going to a different one...
php n00b needs help.
in PHP Coding Help
Posted
sofware: id advise textpad, its very easy to wrok with
check http://www.pixel2life.com/tutorials/php_coding/ there should be lots of beginner tutorials