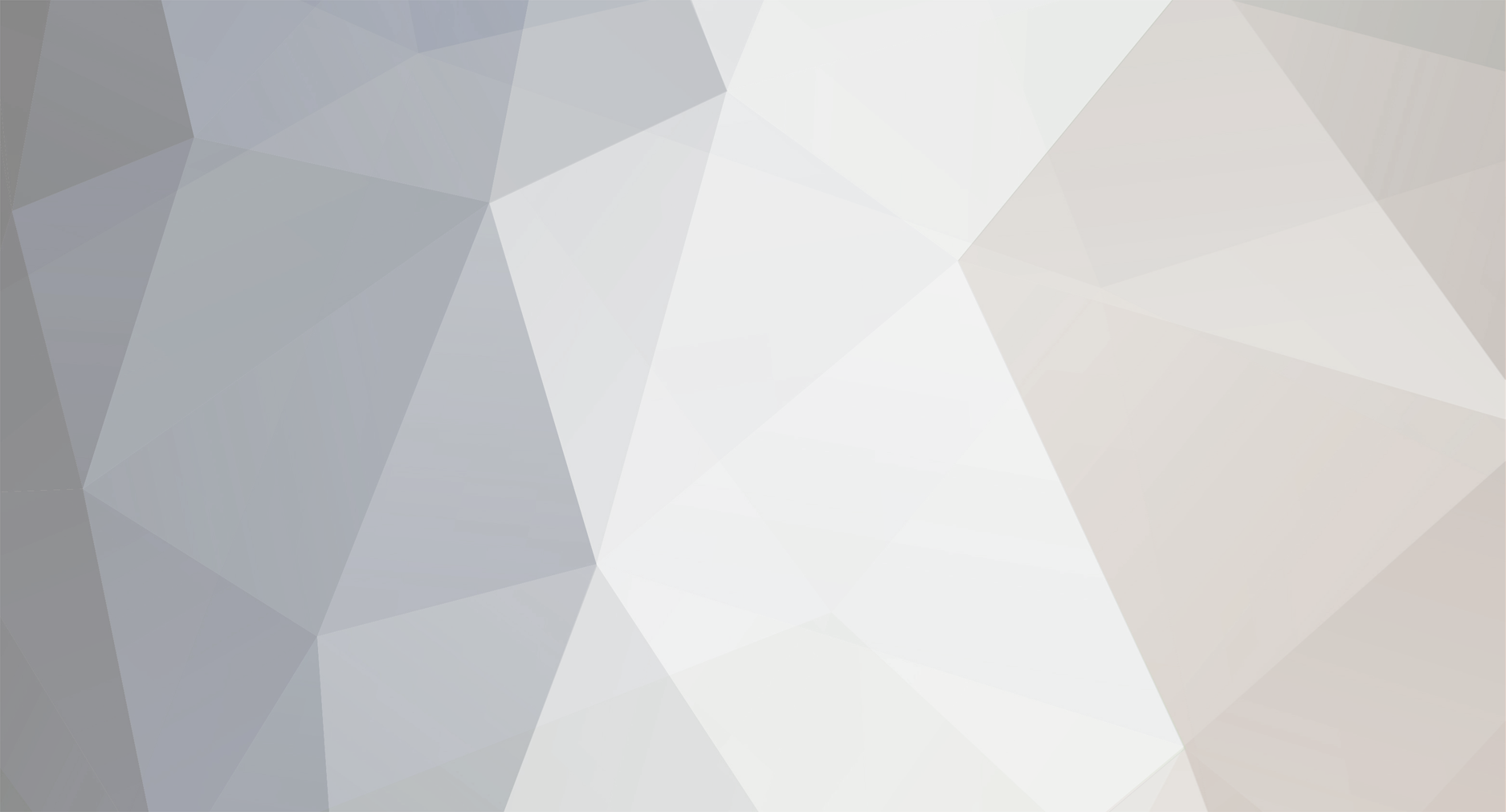
only one
-
Posts
437 -
Joined
-
Last visited
Never
Posts posted by only one
-
-
You forgot to add a quotation mark
<?php echo ('<?xml version="1.0" encoding="iso-8859-1"?>') ?>
-
I would do it using sessions. Something like.
<?php session_start(); $num = rand(1,100); $questionsAskedArray = explode("|", $_SESSION['questionsAsked'], -1); $question = array(); //array of all your questions.. if( !in_array($questionsAskedArray) ) { echo $question[$num]; $_SESSION['questionsAsked'] .= "|". $num; } ?>
You might want to use a loop in it to keep trying until it actually works.
-
Erm, this has nothing to do with PHP.
-
Copying youtubes template isn't a good idea.
-
Try something like.
<?php include("sharons_dbinfo.inc.php"); mysql_connect(mysql, $username, $password); mysql_select_db($database) or die("Unable to select database"); $query = "SELECT * FROM `login` WHERE `email` = '$email' and `lost_password_answer` = '$lost_password_answer'"; $result = mysql_query($query) or die (mysql_error()); $num = mysql_num_rows($result); $array = mysql_fetch_array($result); mysql_close(); if($num == 1) { while ($i < $num) { $name = $array['name']; $psword = $array['psword']; $email = $array['email']; $lost_password_answer = $array['lost_password_answer']; $valid = $array['valid']; // MAIL THE PASSWORD $to = "$email"; $subject = "Your Password"; $message="You recently requested your password be mailed to you\n Thank you for using our service your password is $psword"; $headers = "From: webmaster@affordit.us"; $sent = mail($to, $subject, $message, $headers) ; if($sent) { print "Your password has been sent to $email and it is $psword<br /> <a href=\"index.php\">Back to main page</a>"; } else { print "We encountered an error sending your mail"; } $i++; } } else { print "The values you enterd were false"; } ?>
-
Here's a little code I used to use. It should list all the files inside your directory. Hope it helps.
<?php if($handle = opendir('dirctory/')) //EDIT TO YOUR DIRECTORY { while(false !== ($file = readdir($handle))) { if(($file == ".") || ($file == "..")){}else { $files .= "<tr> <td> $file </td> </tr>\n"; } } closedir($handle); } echo "<table> $files </table>"; ?>
-
That's nothing to do with php... You have to make a sub domain under that domain..
-
while ($cartplog_product_info = $db->fetch_array($cartplog_product_infos) && $i <= 10)
{
$i++;
$cartplog['product_productid'] = intval($cartplog_product_info['productid']);
$cartplog['product_catid'] = intval($cartplog_product_info['catid']);
$cartplog['product_thumb'] = strval($cartplog_product_info['thumb']);
$cartplog['product_title'] = htmlspecialchars_uni($cartplog_product_info['title']);
$cartplog['product_price'] = round(floatval($cartplog_product_info['price']), 2);
eval('$cartplog[\'popular_products\'] .= "' . fetch_template('cartplog_product_item') . '";');
$cartplog['popular_products'] .= '</tr><tr>';
}
-
Hi, I'm working on a page to convert a video file into a .flv file using ffmpeg. I found this script on the net and it didn't relly work, I've been trying to figure it out a few while now and it finnally uploads something but doesn't convert the file.
<?php if(isset($_POST['submit'])) { $moviepath = "movies/"; $flvpath = "flvfiles/"; /***************Load FFMPEG *********************************/ $extension = "ffmpeg"; $extension_soname = $extension . "." . PHP_SHLIB_SUFFIX; $extension_fullname = PHP_EXTENSION_DIR . "/" . $extension_soname; // load extension if (!extension_loaded($extension)) { dl($extension_soname) or die("Can't load extension $extension_fullname\n"); } /*****************Get the path to Extention ****************/ $array_path = explode("/",$_SERVER['SCRIPT_FILENAME']); for ($i=0; $i < sizeof($array_path)-1; $i++) { if(isset($array_path[$i])) { $dynamic_path = $dynamic_path."/".$array_path[$i]; } } /******************Upload and convert video *****************************/ if(isset($_FILES["x_URL"])) { $fileName = $_FILES["x_URL"]["name"]; $fileNameParts = explode(".", $fileName ); $fileExtension = end( $fileNameParts ); if($fileExtension=="avi" || $fileExtension=="wmv" || $fileExtension=="mpeg" || $fileExtension=="mpg" || $fileExtension=="mov" ) { if ( move_uploaded_file($_FILES["x_URL"]["tmp_name"],$moviepath.$_FILES["x_URL"]["name"])) { if( $fileExtension == "wmv" ) { exec("ffmpeg -i /home/dtesters/public_html/".$moviepath."".$fileName."-sameq -acodec mp3 -ar 22050 -ab 32 -f flv -s 320x240 ".$dynamic_path."/".$flvpath."myflv.flv"); } if( $fileExtension == "avi" || $fileExtension=="mpg" || $fileExtension=="mpeg" || $fileExtension=="mov" ) { exec("ffmpeg -i /home/dtesters/public_html//".$moviepath."".$fileName."-sameq -acodec mp3 -ar 22050 -ab 32 -f flv -s 320x240 ".$dynamic_path."/".$flvpath."myflv.flv"); } /******************create thumbnail***************/ exec("ffmpeg -y -i ".$dynamic_path."/".$moviepath."".$fileName."-vframes 1 -ss 00:00:03 -an -vcodec png -f rawvideo -s 110x90 ".$dynamic_path."/".$flvpath."myflv.png"); }else { die("The file was not uploaded"); } }else { die("Please upload file only with avi, wmv, mov or mpg extension!"); } }else { die("File not found"); } } ?> <form name="frm" action="upload.php" method ="post" enctype="multipart/form-data" > <input name="x_URL" type="file" class="form1" size="26" /> <input type="submit" name="submit" value="upload" /> </form>
Thanks for any help,
O1R.
-
1. Yes, google it.
2. Set the font size to a certain % not pixels or points.
3. Yes with HTML,
<a href="link" target="_BLANK">Link</a>
(not valid in xhtml 1.0 strict)
-
try something like:
<?php if(file_exists($_FILES['name'])) { unlink('{$_FILES['name']}'); //now upload it. } else { //mysql query and whatever else you have there. }
-
The MySpace search engine lets you search the MySpace website in different categories as well as the web.
-
If we could see some code it would help, try putting where you want to write to into your file_put_conents function, rather than http://yahoo.com.
-
echo " <a href='{$_SERVER['PHP_SELF']}?pagenum=1&tag={$_GET['tag']}'> <<-First</a> ";
That wont be valid HTML..
echo " <a href='{$_SERVER['PHP_SELF']}?pagenum=1&tag={$_GET['tag']}'> <<-First</a> ";
-
Why do you want to add '?=games' to the querystring? It wont do anything.
-
mysql_query("INSERT INTO `users` (`username`, `password`, `userlevel`, `userid`, `email`) VALUES ('$user', '$pass', '$ulvl', '$uid', '$emil')");
-
You're not selecting any field,
$query = "SELECT * FROM `software` WHERE `name` = 'Maya'"; $result = mysql_query($query); $row = mysql_fetch_array($result); echo "{$row['field']}";
-
I'm a 15 year old male, i can program PHP, Javascript, a little Java, C++, C# and can code HTML and CSS.
-
Not bad at all, i just don't like the thing that sits on the top left with your address and picture, i think it's because the picture is blured.
-
It's quite nice, not really my style.
There's a problem with your tabs along the top:
-
What are you trying to do with $_POST['name$j']? Maybe you were trying to do $_POST['name'] .= $j;
-
Maybe this has been posted before, but i was thinking it would be handy if there was a 'quick reply' feild at the bottom of each topic. I've seen them on other forums and it saves a lot of time in my opinion.
-
Your table should stick to the width it's set to, try using divs instead of tables.
Check the substr manual.
-
I pronounce it 'my' as in mine, S Q L.
PHP SOAP server and client not working....getting error
in PHP Coding Help
Posted
It can't find what you are trying to include.
try putting in your root path..
/www/soap/testserver.wsdl
instead of http://localhost/.....