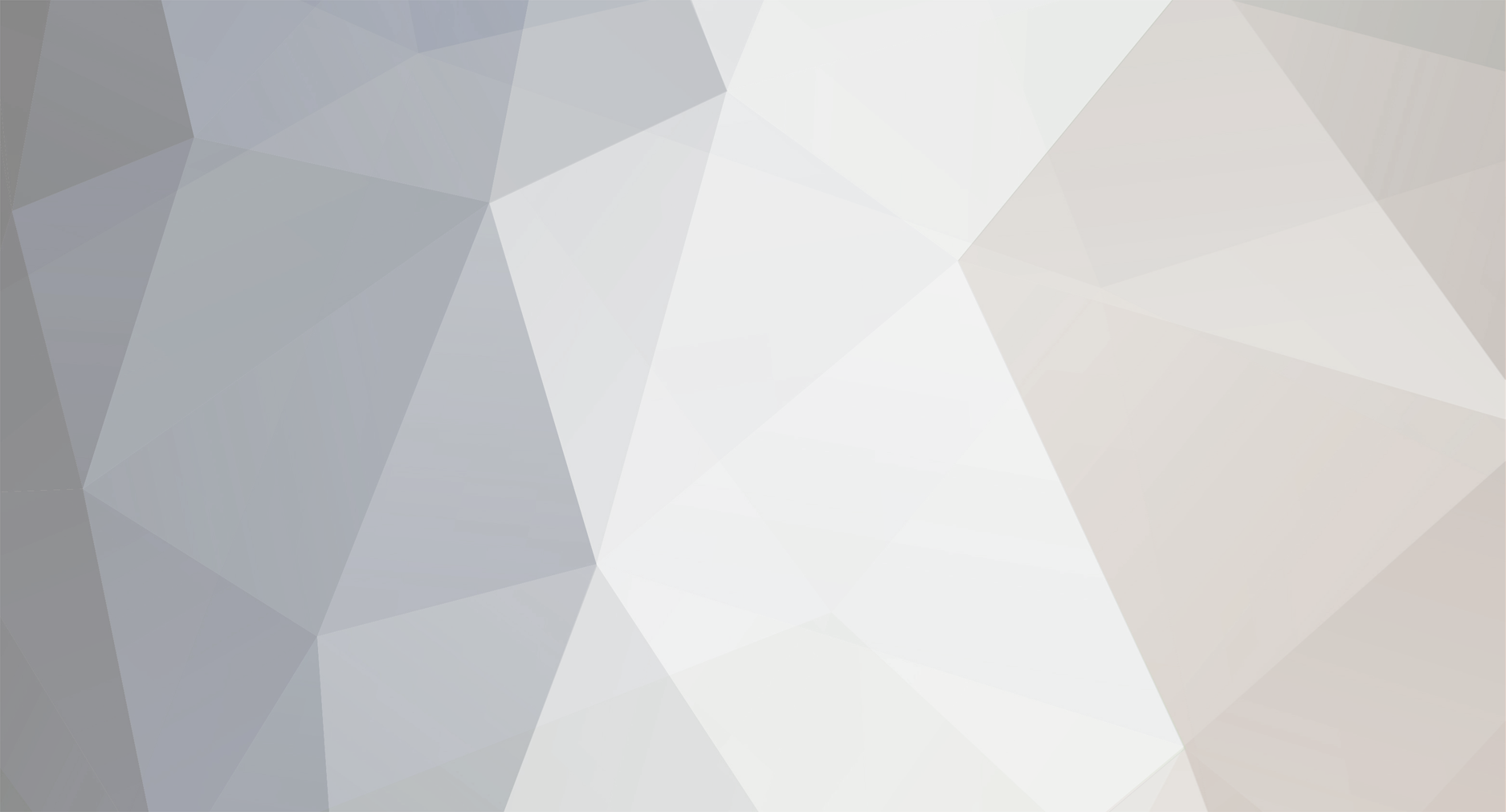
jscix
-
Posts
197 -
Joined
-
Last visited
Never
Posts posted by jscix
-
-
Okay, I just read a basic page on regex. I'm trying to come up with a regex expression for validating an url.
/^http://[.*]\.[a-zA-Z0-9]\.[.{2}]|[.{3}]\/[.*]$/
^
I think this says:
Starting with http://
Followed by any amount of any character .*
Followed by a period \.
Followed by any string (a-z A-Z and 0-9)
Followed by a period \.
Followed by any two characters .{2}
OR
Followed by any three characters .{3}
Followed by a /
Ending with any amount of characters .*$
(Sorry in advance for the questions, I figure i'll never understand this stuff If I just keep borrowing other peoples regex)
I'm just curious how close I was with that? Also im curious about a few things..
1. When do you use brackets? And why? [ ]
2. When do you use Parentheses? And why? ( )
3. What should my regex have looked like, for validating an url?
-
If anyone is interested/cares I figured it out.
(well, someone from www.physicsforums.com told me how to finish it.) ;o
All I was missing was dividing the deviation by the total number of coin tosses. If anyone wants to check it out ,
heres the code.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8" /> <title>Coin Toss</title> </head> <html xmlns="http://www.w3.org/1999/xhtml"> <?php $submit = ($_POST['Toss']); $values = ($_POST['select']); if (!$submit) { ?> <body><center> <p align="center">Coin Toss Deviation <form method="post" action="cointoss.php"> <select name="select"> <option value="none">How many coin tosses?</option> <option value="one">One Hundred</option> <option value="two">One Thousand</option> <option value="three">One-Hundred Thousand</option> </select><input type="submit" name="Toss" value="Toss" /> </form> </center></body></p> </html> <?php } else { switch ($values) { case "none": die ("Select number of tosses"); break; case "one": $total = "100"; break; case "two": $total = "1000"; break; case "three": $total = "100000"; break; } $dev = 0; $i = 0; while ($i < $total) { $cur = mt_rand(1,2); if ($cur == "1") { $dev++; $one++; } if ($cur == "2") { $dev--; $two++; } $i++; } $st = ($dev / $total); print "<center>ones: $one <br>twos: $two<br><br>"; print "<b>total: " . $st . " point deviation.</b><br><br>"; print <<< DUH Average deviation should be 0 points<br> The more tosses, the lower the deviation should be. (Closer to 0)<br> DUH; print "<a href=\"cointoss.php\">Try Again!</a>"; } ?>
-
Okay, What im trying to do is create a create a random number generator, that outputs 1 and 2 (coin toss)
From what I understand, the more coin tosses (the more times I generate a random 1 or 2) the more uniform the pattern should be.
EG: After generating a random 1-2 sequence, 1,000,000 times.. i should have 500,000 (1s) and 500,000 (2s).. am I wrong?
Anyway, I'm not sure what Im doing wrong but the more numbers I generate, the more deviation Im getting.
Here is how i'm doing this.. (I know I probably did this all wrong.. if so someone just tell me lols)
ty in advance
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8" /> <title>Coin Toss</title> </head> <html xmlns="http://www.w3.org/1999/xhtml"> <?php $submit = ($_POST['Toss']); $values = ($_POST['select']); if (!$submit) { ?> <body><center> <p align="center">Coin Toss Deviation <form method="post" action="Cointoss.php"> <select name="select"> <option value="none">How many coin tosses?</option> <option value="one">One Hundred</option> <option value="two">One Thousand</option> <option value="three">One-Hundred Thousand</option> </select><input type="submit" name="Toss" value="Toss" /> </form> </center></body></p> </html> <?php } else { switch ($values) { case "none": die ("Select number of tosses"); break; case "one": $total = "100"; break; case "two": $total = "1000"; break; case "three": $total = "100000"; break; } $dev = 0; $i = 0; while ($i < $total) { $cur = mt_rand(1,2); //print $i . " = $cur <br>"; if ($cur == "1") { $dev++; $one++; } if ($cur == "2") { $dev--; $two++; } $i++; } unset ($submit); print "<center>ones: $one <br>twos: $two<br>"; print "total: " . $dev . " point deviation.<br><br>"; print <<< DUH Average deviation is 0 points<br> The more tosses, the lower the deviation should be. (Closer to 0)<br> DUH; print "<a href=\"Cointoss.php\">Try Again!</a>"; } ?>
-
Warning: session_start(): Cannot send session cache limiter - headers already sent (output started at /home/www/narutoking.com/index.php:3) in /home/www/narutoking.com/common.php on line 3
Means, the file your trying to include, is trying to start a session "Session_Start()", after the page/script your including it in, has already sent data to the page, session_start() has to be the first thing on the page.
so, your best bet is to add in a <?php session_start(); ?> to the top of index.php
-
It seems your not alone with your issue, however:
http://www.google.com/search?source=ig&hl=en&q=ie6+session+issues&btnG=Google+Search
-
Check your users browser types, if their using IE, redirect them to the mozilla download page.
ok sorry, bad joke.
but seriously, I hate ie. die ms...
-
if ((!empty($textfield1)) && (!empty($textfield2))) {
$to = "namet@reaganpower.com";
$subject = "Technical Support request form";
$body = "
\n\n Name: $textfield
\n\n E-Mail Address: $textfield2
\n\n Comments: $textarea";
mail ($to, $subject, $body);
header("Location: http://reaganpower.com/thankyou.html");
} else {
print "Fill out all feilds etc etc";
}
-
lol, wow I didnt catch that at all, ???
$query="INSERT INTO seebergerLogin (username, password, first_name, last_name)VALUES ('$_POST[email_address]','$_POST[password]','$_POST[first_name]','$_POST[last_name]')";
$query is a bad var name for a mysql_query, it looks far too similar
-
I honestly cant see the problem, your sql looks fine, as does the rest of your code.. but im curious why you are working with dirty $_POST Variables, after you have already placed and secured them(Assuming the CLEAN function does this) right here:
$fname = clean($_POST['first_name']);
$lname = clean($_POST['last_name']);
$email = clean($_POST['email_address']);
$pw = clean($_POST['password']);
?
-
yeah, i've created a function to validate the extention,
Function ValidateFileXT($string) { $trimstr = substr($string, -4); $xt = strstr($trimstr, '.'); if (!$xt){ return False; } else { return $xt; } }
eg:
$file = strtolower(basename($_FILES['uploadedfile']['name']));
if (ValidateFileXT($file) == ".mp3") {
//yay
}
but I was thinking more in terms of actual validation, I think I remember reading that the GD lib. Has a function: exif_imagetype()
"exif_imagetype() reads the first bytes of an image and checks its signature."
How difficult would this be to do with an mp3?
-
Okay, This is the first time I've attempted creating anything beyond a simple image upload.
I would appreciate any helpful tips for ways to maximize validation for mp3 files.
Beyond using,
$_FILES["uploadedfile"]["type"]
What ways can I make sure, the file is an valid mp3 file?
Much appreciation in advance..
-
if what your wanting to do is keep the value of the selected option in the list, send the value upon submission using GET
<option<?php if ($get['lesson'] == "whatever") { print " selected=\"selected\"";} ?>> lesson</option>
if the get variable is present it just sets the option feild be be autoselected on load, hope that helps
-
"what are sleepers?"
I would assume he means implements a hidden feature that either grants you access to the file system, or a way to remotely delete the files, in case of non payment
-
I have been teaching myself PHP,SQL (along with dhtml,xml,css,javascript etc) and plan on going to school for either java, or c.
I find programming/web design to be very fun/interesting, So I have some questions for anyone who does this stuff for a living,
How difficult is it to make a decent salery freelancing?
and what advice would you offer a novice interesting in persuring a freelance career in programming?
-
"You have to remember that varchars need single quotes or any string, and to put math problems in parans"
ah, okay. I didn't know this either. Thanks
-
Doh, yeah Im a bit slow today I guess.
The syntax was off,
mysql_query("UPDATE `attend` SET `total` = `total` + 1, `ip` = '$curIP' WHERE `attend`.`eid` = '$eventid'")
works fine. Thanks man.
-
Okay, I'm pretty sure im doing this correct, as it's updating the IP Address, but it isn't incrementing the 'total' feild, the number is staying the same.
Anyone?
mysql_query("UPDATE `attend` SET total=total+1, ip=$curIP WHERE attend.eid = $eventid");
-
basically what you want to do is be as sure as possible, that what is to be saved to your database is what you intend. Anywhere that user-input is allowed, you assume the worst and filter, parse and examine everything.
I'd recommend reading "Essential PHP Security" by chris shiftlett (o'reilly)
-
See if this query works,
$change = mysql_query("ALTER TABLE `movies` CHANGE `directors` `directorrrsss` VARCHAR( 100 ) NOT NULL");
If this doesnt work, I would just install phpMyAdmin ( Anytime you perform a command using their visual interface, it gives you the SQL Code, and has an option to output php formated code to use in your scripts )
-
Okay, I tried a
<?php print_r ($_GET); ?>
I got this(when using your get string):
Array ( [custom_where_did_you_hear_about_us] => dfsfdsf )
So try,
echo $_GET['custom_where_did_you_hear_about_us'];
-
Yes, except `Table` should be the name of your table.
-
no, you dont need it. was just explaining what i meant by type/length
-
sorry spelled it dpAddress instead of jp, but yeah. np
-
ALTER TABLE `TABLE` CHANGE `name` `jpName` VARCHAR( 100 ) NOT NULL ,
CHANGE `address` `dpAddress` VARCHAR( 100 ) NOT NULL
help!! validate a domain
in PHP Coding Help
Posted
regex is a mysterious language created by aliens, imo. I'll never understand it, no matter how much I read ::oo