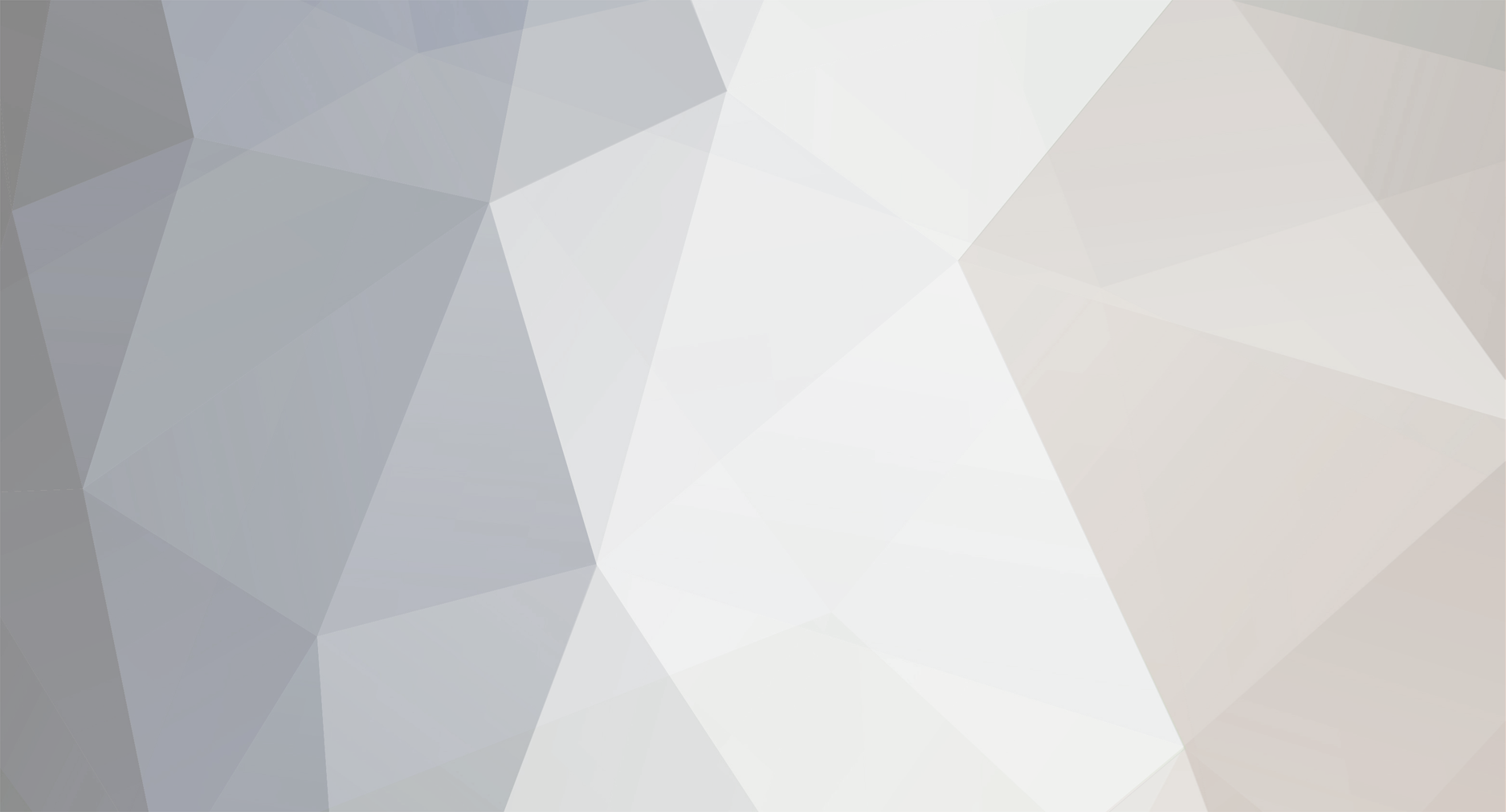
per1os
New Members-
Posts
3,095 -
Joined
-
Last visited
Everything posted by per1os
-
<?php $months = array(1=> array("num" => "01", "name" => "January"), 2 => array("num" => "02", "name" => "February")); // ..etc $currentMonth = 4; $count = 12; $display = ""; while (true) { if (($counter % 2) == 0) { $display .= "<td>" . $months[$currentMonth]['name'] . "</td></tr>"; }else { $display .= "<tr><td>" . $months[$currentMonth]['name'] . "</td>"; // error was here } if ($currentMonth == 12) { $currentMonth = 0; } if ($counter == 12) { break; // kill the loop } $currentMonth++; $counter++; } echo "<table>" . $display . "</table>"; ?> my bad.
-
Put the months into an array. <?php $months = array(1=> array("num" => "01", "name" => "January"), 2 => array("num" => "02", "name" => "February")); // ..etc $currentMonth = 4; $count = 12; $display = ""; while (true) { if (($counter % 2) == 0) { $display .= "<td>" . $months[$currentMonth]['name'] . "</td></tr>"; }else { $display .= "<tr><td>" . $currentMonth[$currentMonth]['name'] . "</td>"; } if ($currentMonth == 12) { $currentMonth = 0; } if ($counter == 12) { break; // kill the loop } $currentMonth++; $counter++; } echo "<table>" . $display . "</table>"; ?> Change 'name' to 'num' to get the number.
-
You definitely do not want register_globals on. But if the CGI is causing problems you can manually turn off the register_globals via htaccess, that or just code as if they were off.
-
Use modulos (%) <?php $currentMonth = 4; $count = 12; $display = ""; while (true) { if (($counter % 2) == 0) { $display .= "<td>" . $currentMonth . "</td></tr>"; }else { $display .= "<tr><td>" . $currentMonth . "</td>"; } if ($currentMonth == 12) { $currentMonth = 0; } if ($counter == 12) { break; // kill the loop } $currentMonth++; $counter++; } echo "<table>" . $display . "</table>"; ?> Something like that?
-
www.php.net/ereg There are a bunch of email validation functions located in the user comments. Or even from www.php.net/eregi If thats what you are looking for, no need to re--invent the wheel.
-
I am sure the spike in 06 was caused by PHP 5. But for the most part the reason is as explained, php4 came out and it sky rocketed from there. PHP 3 really was a joke, with the development of the Zend engine they brought PHP a long ways.
-
Highly doubt it, but give it a try. You have to know that most email clients filter out that stuff or display it as just raw text for that exact reason. I could hi-jack anyone's cookies using javascript/iframe. Which is why it would, and should be disabled. I think your going to be wasting your time pursuing this any further.
-
Umm maybe it helps if you use the right if statement? <?php $val['rank'] = intval($val['rank']); // make sure it is in int. if ($val['rank'] == 4) { echo 'Person is Rank4'; }else elseif ($val['rank'] == 3) { echo 'Person is Rank3'; }else elseif ($val['rank'] == 2) { echo 'Person is Rank2'; }else elseif ($val['rank'] == 1) { echo 'Person is Rank1'; }else elseif ($val['rank'] == 0) { echo 'Person is Rank0'; } else { echo 'Unexpected error please contact the Admin'; } ?> Elseif's are the right type of if's for this statement.
-
Generally means your error_reporting is set to too low or display_errors is turned off. www.php.net/init_set init_set('display_errors', 'on'); error_reporting(E_ALL); Add those to the top and see if an error comes out.
-
That is the best way to use sessions, it removes those annoying ids from the links and put the sessionid into a cookie. This will solve your problem of wanting to not use "session_start" as it would not append the session id to the urls.
-
<?php if ($x == 1) { echo 'here'; }elseif ($x == 2) { echo 'there'; }// ... etc ?> <?php switch ($x) { case '1': echo 'here'; break; case '2': echo 'there'; break; } ?>
-
Shit Out of Luck
-
Nope you are SOL.
-
Thanks. Although that works when I send the email to my address the link is still visible in the status bar.. When the mouse hovers over the link it should say "Click here to continue". You do know that most email clients disallow javascript in the email that is received right? Reason being is because javascript can easily be used to exploit someone's computer. I doubt this would/should work.
-
To be honest you wouldn't see much of a difference unless there are about 1,000+ entries to run it against. I use eregi for my checking works great for me, never had a speed issue.
-
Edit to the above, when using strstr or stristr always use !== FALSE if ($_POST['submit'] && (stristr($string,$spamlist) !== FALSE)) { As it can return 0, which is taken as false, when really the word is there.
-
<?php if (eregi('www.', $message)) { // the period may have to be escaped "\." echo 'Please no spam.'; } ?> Does that not work?
-
for every item in array of items create a new index of $item in the $contents array and if it is already set add one, else set it to one.
-
The best way to go about doing it is storing the file information in the database that way when the user is in you can locate the file and the file does not have to be stored in separate folders for users etc and this way if you want to expand it makes i easy. I would also suggest creating a hash for the filename or some type of combination as to avoid duplicates, and as long as that data is stored in the DB you are set. MySQL I think would be faster if you already have the connection present etc. Either way it will be pretty fast whichever method you choose.
-
You can use Regex which works pretty well, or LIKE with the % wildcard, or if you want FULL TEXT search you can use MATCH and AGAINST. Really it depends on how advanced you want to get.
-
Use session variables or cookies. It is not possible using referrer check due to the fact it is easily spoofed.
-
I think your mistaken. http://us2.php.net/manual/en/function.sort.php asort is for sorting but mainting index association. Sort automaticalyl goes by the natrual sort meaning it will sort numbers before letters etc. sorta is not a function.
-
lol, now the question is, let's say that you retrieve the data from mysql, what format does it come in? Usually you use mysql_fetch_array to retrieve data from mysql. Weird it comes in an array format... Now let's say that you want to process the data from mysql somewhere else on the page instead of just printing it out within the while loop. Say we have state data that could be used multiple times on the page. We can gather the data from mysql into a multi-dimensional array which we then can pass to different functions, say one function is to create a drop down menu, another function is to assign flags to each of the states and the third function is for let's say assigning the state flower. Now you could make 3 trips to the database grabbing the same data, or you could just assign the data to an array and loop through the array, thus saving mysql retrival time. But when you think of that question ask yourself, how is $_SESSION data retrieved and stored? How is $_COOKIE data retrieve and store? Same with GET and POST, also what about $_SERVER or $_REQUEST? All are arrays. It just makes life easier to access them that way. Although it may not be apparent it, I guarantee you, that you will one day realize, wow arrays can make my job a ton easier. I would much rather have my data in an array as appose to multiple variables. I can easily change the index name of an array, the data etc without having to get creative =)
-
lol obviously have not worked much with data. Arrays are very handy in many instances, whether it would be storing state data from a database or just in code IE: <?php $states = array("al" => "Alaska", "CO" => "Colorado"); // Using the array above I can easily loop through it and print out the data in a fashion I like foreach ($states as $abb => $full) { echo $abb . ' is the abbreviation for ' . $full . '<br />'; } // I can also take in just an abbreviation and find that state name echo $states['al'] . " is the state for the abbreviation 'al'<br />"; //Or I can take it a step further and house item data: $items = array(0 => array("item_name" => "Shoes", "price" => "25.00", "quantity" => 32), 1 => array("item_name" => "Robes", "price" => "25.00", "quantity" => 3)); $total = 0; foreach ($items as $id => $item) { $item_total = $item['price'] * $item['quantity']; $total += $item_total; echo "You have chosen to buy " . $item['item_name'] . " at the cost of \$" . $item['price'] . " with a quantity of " . $item['quantity'] . " which is equal to \$" . number_format($item_total, 2) . "<br />"; } echo '<br />Your orders total is $' . number_format($total); ?> Really arrays can make processing large amounts of data easier and efficiently, as you can see I could have 50 items in that array and it will total everything up and print out nice messages to me with few lines of code.