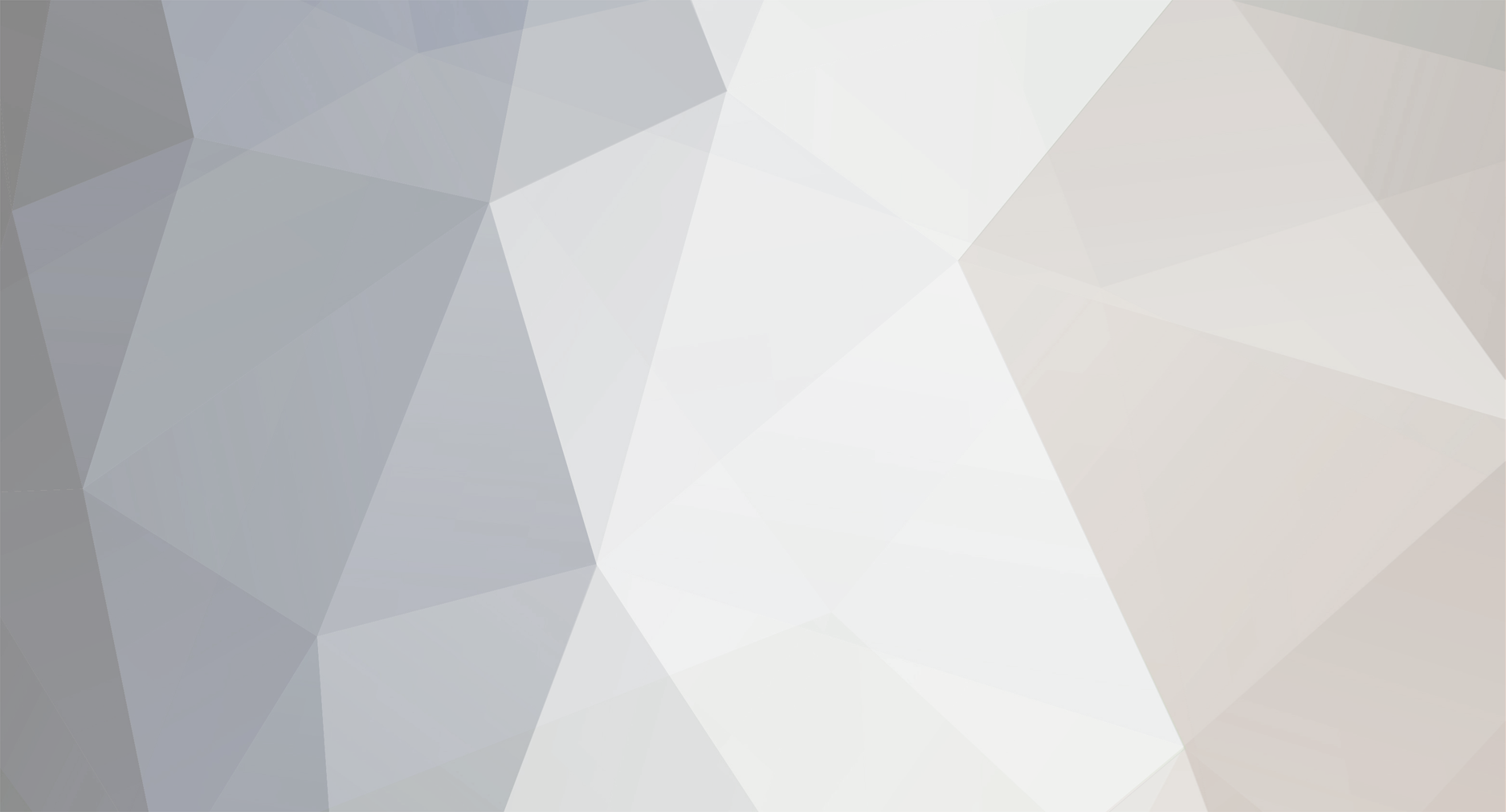
per1os
New Members-
Posts
3,095 -
Joined
-
Last visited
Everything posted by per1os
-
No, just stating that. As for the problem, thats not really a problem at all. I have classes I store in session that uses session variables for verification etc. Never had a problem with it, and I never serialized it. I guess I could but yea.
-
Since you are on a shared server, I would highly recommened you look into http://us2.php.net/manual/en/function.session-save-path.php session_save_path() As quoted from that page: Implementing this on your server would mean that you set where the session files are being stored. Create a directory on your server like /tmp/sess/ and use that for the storing of session files. This helps prevent a HUGE security leak that could happen, especially since you do not validate the username/password everytime you simply set the value of "loggedin" which means all I have to do is if I am on that server is get the file, modify it and I am validated for any page on your website. At any rate, I would try to implement this and if all else fails for the session destroy stuff you can manually delete the session file using www.php.net/unlink Shared hosting is very iffy, it sounds like they disabled permissions to delete the session data, with good reason to. Closing the browser will kill the session anyways. The goal is to kill it without having to close the browser.
-
<?php $page = $_SERVER['PHP_SELF']; echo 'You are currently on ' . $page; ?>
-
<?php session_start(); include("dbconnect.php"); // track logout time in statistics if user logged in if($_SESSION['online'] && $_SESSION['LoginStatus']){ @mysql_query("UPDATE stats_ppl_online SET logout_time=now() WHERE session_id='".session_id()."'"); } // kill session foreach ($_SESSION as $key => $val) { $_SESSION[$key] = null; unset($_SESSION[$key]); // here would of been the parse error. } setcookie(session_name(), session_id(), 1, '/'); unset($_SESSION); session_destroy(); //header("Location: goodbye.php"); echo("<script type='text/javascript'>parent.location='goodbye.php'</script>"); //exit(); ?> If that does not completely destroy the session, maybe you have write/delete issues on the server? Are you using a shared server?
-
Why are you using pconnect? From what I know it is unnecessary and takes a toll on your server. There is nothing wrong with closing mysql connections and re-opening them on the page call. Using that pconnect, if you had 100 users your site may stop functioning because of it. Unless there is a specific reason for using it I would stray away from it and just use the regular mysql_connect.
-
You don't even need to serialize it. Just make sure that the class definition comes before session_start()
-
That would of given a parse error, here is a fix <?php session_start(); include("dbconnect.php"); // track logout time in statistics if user logged in if($_SESSION['online'] && $_SESSION['LoginStatus']){ @mysql_query("UPDATE stats_ppl_online SET logout_time=now() WHERE session_id='".session_id()."'"); } // kill session foreach ($_SESSION as $key => $val) { $_SESSION[$key] = null; unset($_SESSION[$key]); // here would of been the parse error. } if (isset($_COOKIE[session_name()])) { setcookie(session_name(), '', time()-42000, '/'); } session_destroy(); //header("Location: goodbye.php"); echo("<script type='text/javascript'>parent.location='goodbye.php'</script>"); //exit(); ?> But what are you doing on goodbye.php to display that output?
-
<?php session_start(); include("dbconnect.php"); // track logout time in statistics if user logged in if($_SESSION['online'] && $_SESSION['LoginStatus']){ @mysql_query("UPDATE stats_ppl_online SET logout_time=now() WHERE session_id='".session_id()."'"); } // kill session foreach ($_SESSION as $key => $val) { $_SESSION[$key] = null; unset($_SESSION[$key]; } if (isset($_COOKIE[session_name()])) { setcookie(session_name(), '', time()-42000, '/'); } session_destroy(); //header("Location: goodbye.php"); echo("<script type='text/javascript'>parent.location='goodbye.php'</script>"); //exit(); ?> Try that out and see where it gets you.
-
<?php session_start(); session_destroy(); echo '<script type="text/javacsript">window.location=\'http://www.yoursite.com/path/to/goodbye.php\';</script>'; ?> Replace the yoursite and path with the full path to goodbye.php see if that works.
-
Shouldn't that if statement be within the while loop?
-
www.google.com Search for PHP Captcha.
-
<?php session_start(); session_destroy(); echo '<script type="text/javacsript">location.href=\'goodbye.php\';</script>'; ?>
-
Int's are different than strings. Try this to convert that int to a string: $count194++; $count194 = "$count194"; for($x=0;$x<strlen($count194);$x++) { echo $count194[$x] . "<br />"; } See if that works.
-
You want to google Pagination or look at the tutorials section of PHPFreaks for Pagination. That is what you want.
-
Doesn't matter if you can't see how. Sometimes headers and sessions do not get along. For instance I bet if you tried setting a session variable than do a header redirect to a page that should display that value it probably would not work. Give that a try and see if I am right, if I am than the problem lies within the header redirect. If I am not, than well we know it is not the header causing the issue.
-
$count194++; Why are you adding one to a "string". Thats why it produces "1".
-
[SOLVED] Sort/Re-arranging a Multidimension Array
per1os replied to albertdumb's topic in PHP Coding Help
<?php foreach ($dept_array as $array) { $new_array[$array['dep_airport']][] = $array['arr_airport']; } print_r($new_array); ?> See if that works for ya. -
The header redirect could be toying with you. Try using a javascript redirect instead and see if that works.
-
How is that even possible? Doesn't that say that it returns nothing? It says it returns true on success and false on failure. But since it is a "void" type it means it really doesn't have to return anything. But either way it works like a void function does, the code is executed and you are not really informed if it worked or not. Meaning it is meant to just close the session, it does not need to tell you if it worked or not. As for the PM, probably but I never save my messages sent. Sorry bud.
-
[SOLVED] Variable not passing to included class
per1os replied to devknob's topic in PHP Coding Help
$this->un = $_SESSION['uid']; If you assign $_SESSION['uid'] to $uid, $uid is not a global variable, where as $_SESSION is. Meaning that you cannot use $uid outside of the scope, where the class would be outside of the scope. Change the line above and it should work. -
www.php.net/session_write_close Try that before the header redirect. It is a known problem for some people
-
What is usually contained in site_in, can you also post the database structure with maybe a few fields from the DB?
-
both fields false but else statement not executing
per1os replied to Foser's topic in PHP Coding Help
if (mysql_num_rows($result) > 0 or die(mysql_error())){ Thats why you are getting a blank page, there is no error on the mysql part and since you entered the data wrong it goes to the or portion. <?php //starting SESSION session_start(); //Lazy Login if ($_SESSION['LOGGEDIN'] == TRUE){ header("Location: main/index.php");} //SQL Configuration file require("config.php"); //when pressed submit execute the FOLOWING if (isset($_POST['submit'])){ // USER TYPED INFORMATION $user = mysql_real_escape_string($_POST['username']); $password = sha1(md5($_POST['password'])); // Searching DB for username and password Match $result = mysql_query("SELECT * FROM user_info WHERE username = '$user' and password = '$password'") or die(mysql_error()); if (mysql_num_rows($result) > 0){ // SETTING UP SESSIONS $_SESSION['LOGGEDIN'] = TRUE; $_SESSION['UNAME'] = $user; //Go To Member Control Panel if ($_SESSION['LOGGEDIN'] = TRUE){ header("Location: main/index.php"); exit; }} // IF EVERYTHING ELSE IS FALSE EXECUTE THIS: else { echo "You have not entered the correct data. You may try again.";}} ?> The above should hit the else. -
http://www.sitepoint.com/article/hierarchical-data-database That site should help you out.
-
require_once("config.php"); $connection = mysql_connect($host, $user, $pwd) or die("&error1=".mysql_error()); mysql_select_db($db, $connection) OR DIE("Selection Error:" . mysql_error() . "<br /> For Database: $db"); See what that gets ya.