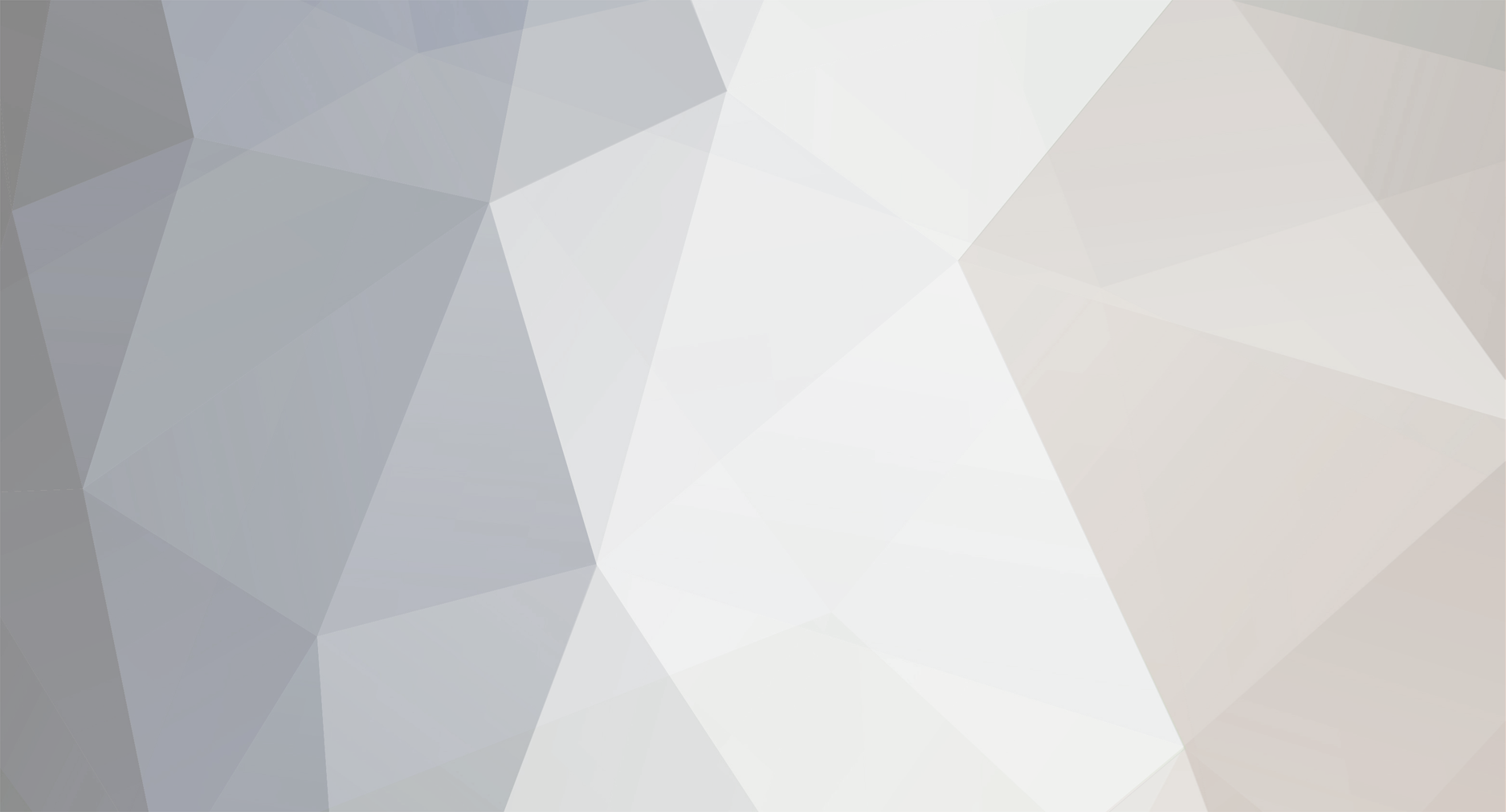
per1os
New Members-
Posts
3,095 -
Joined
-
Last visited
Everything posted by per1os
-
I do not really understand the question you are asking above. But here is a simple table design that may help you see why it would be better not to do it with tons of tables: Create table users ( userid int(11) NOT NULL auto_increment, username varchar(50) NOT NULL, password varchar(55) NOT NULL, email varchar(250) NOT NULL, date_created int(11) NOT NULL, primary key(userid), index(username,email) ); create table logs ( logid int(11) NOT NULL auto_increment, userid int(11) NOT NULL, log_timestamp int(11) NOT NULL, log_item int(5) NOT NULL, primary key(logid), index(userid) ); create table log_items ( logitemid int(5) NOT NULL auto_increment, logitemname varchar(50) NOT NULL, logitemdetail text NOT NULL, primary key(logitemid) ); That is an example of a database in 3NF (3rd normal form) Here is how a new user insert would look: INSERT INTO users(`username`, `password`, `email`, `date_created`) VALUES ('$name', '$password', '$email', '" . time() . "'); Now let's say we want to insert a log for that user, something like this would work... SELECT userid FROM users where username = '$name' SELECT logitemid FROM log_items WHERE logitemname = 'Login'; INSERT INTO logs (`userid`, `log_timestamp`, `log_itemid`, `log_timestamp`) VALUES ('$userid', '$logitemid', " . time() . "); That way everything is nice and neat, you can easily query this back and grab what log action the user did, what time they did it and it can have as many users/logs as needed and can be easily filtered by userid etc. Anyhow hope that helps make it clearer on why you really do not want a ton of tables.
-
Read up on Database Management. You will understand soon enough. Databases are not meant to store 1 row per table. They are meant to store thousands, if not millions of rows per table. The way you are going about it you will have thousands if not millions of tables with 1 row a piece. Its just not even being realistic. Imagine this, if you were laying a roof and had all the tiles scattered and only 1 nail per roof-tile it would not be very effective or efficient and chances are a good breeze would throw off the tiles. You obviously have not even scratched the surface of database management, I wouldn't be surprised if you came back stating that you never even read anything about a database. You will thank me for pushing you away from the current route you are going later on.
-
Trouble inserting session variables into a database
per1os replied to matthewchristianson's topic in PHP Coding Help
Just an FYI, double posting is not cool... http://www.phpfreaks.com/forums/index.php/topic,147422.0.html Especially within minutes of each other... -
www.php.net/curl Use curl. It will work better.
-
mysql_query("INSERT INTO schools VALUES ( '{$_SESSION['school_name']}', '{$_SESSION['postcode']}', '{$_SESSION['techer_name']}', '{$_SESSION['email']}', '{$_SESSION['num_logins']}' )"); Syntax errors, the ' ' on that $_SESSION['index'] is causing the problem.
-
It makes variables url friendly, as an & inside $_REQUEST would throw the url off, url_encode encodes them so they can be passed as they need to be passed.
-
<?php //$x = $_REQUEST['amount']; and then pass the $x via the url to another page like https://xxx.com/natHealing/view_gift.php?x $x = $_REQUEST['amount']; $url = "http://www.site.com/page.php?x=" . url_encode($_REQUEST['amount']); ?> Would work.
-
I would highly suggest reading up on MySQL and even looking for a tutorial/book. The way you are trying to use MySQL is not even plausible and very inefficient and you will be kicking yourself in the butt later for doing it that way. Reading is often times very good in programming. Read up on Database Management, you can store multiple users in 1 table and link them to a "log" table that can log actions etc. if need be.
-
Use the LIKE operator with the % wildcard.
-
mysql_real_escape_string not going into database
per1os replied to suttercain's topic in PHP Coding Help
That is the problem nadeemshafi9. No worries it is solved with that exert. -
Some code works but doing this would solve the problem: $year = isset($_REQUEST['year'])?$_REQUEST['year']:''; Checks if the variable was set if it was it assigns it to year if not is assings '' to year to avoid a notice error.
-
mysql_real_escape_string not going into database
per1os replied to suttercain's topic in PHP Coding Help
I should of looked at the code closer. require ('get_connected.php'); mysql_query ("INSERT INTO canada (f That is AFTER you used mysql_real_escape_string. You need to require the get_connected.php BEFORE you call mysql_real_escape_string. -
mysql_real_escape_string not going into database
per1os replied to suttercain's topic in PHP Coding Help
Try adding this to the top of the page: error_reporting(E_ALL); see if any warning messages are being thrown. -
mysql_real_escape_string not going into database
per1os replied to suttercain's topic in PHP Coding Help
You do have a database connection initiated don't you? -
mysql_real_escape_string not going into database
per1os replied to suttercain's topic in PHP Coding Help
Just a thought, is register_globals on? -
http://en.wikipedia.org/wiki/Fulltext_search Read up. To create a search without you need to the use the LIKE keyword with the wildcard (%) sign. and probably the OR keyword too. Since you do not know what you are doing read a tutorial http://www.designplace.org/scripts.php?page=1&c_id=25
-
On the page that is sending the data, make sure the charset is defined and is what you need it to be, you may try using UTF-8 for the database and the page.
-
CREATE TABLE `houses` ( `id` int(11) NOT NULL auto_increment, `name` varchar(255) default NULL, `email` varchar(255) default NULL, `address` varchar(255) default NULL, `state` varchar(255) default NULL, `city` varchar(255) default NULL, `zip` int(11) default NULL, `type` varchar(255) default NULL, `footage` varchar(255) NOT NULL, `image` varchar(255) NOT NULL, `enabled` tinyint(1) NOT NULL, PRIMARY KEY (`id`), fulltext (address,state,city,zip,footage) ) ENGINE=MyISAM AUTO_INCREMENT=22 DEFAULT CHARSET=latin1 AUTO_INCREMENT=22 ; http://dev.mysql.com/doc/refman/5.0/en/fulltext-search.html You can only use fulltext search on columns that have been indexed as full text.
-
You need to add the fulltext index to address, state, city, zip and footage via phpmyadmin or manual sql.
-
if (!empty($_POST['art_image'])) { $art_image = "'" . $_POST['art_image'] . "'"; } else { $art_image = 'NULL'; } //run the query which adds the data gathered from the form into the database $result = mysql_query("INSERT INTO az_articles (art_title, section, category, issue, art_image, art_text) VALUES ('$art_title','$section', '$category', '$issue'," . $art_image . ",'$art_text')"); You have tried the above code exactly how it is. If so give this a shot if (!empty($_POST['art_image'])) { $art_image = "'" . $_POST['art_image'] . "'"; } else { $art_image = 'NULL'; } //run the query which adds the data gathered from the form into the database $result = mysql_query("INSERT INTO az_articles (art_title, section, category, issue, art_image, art_text) VALUES ('$art_title','$section', '$category', '$issue',NULL,'$art_text')"); Just for a testing purpose and see what comes of it.
-
$result = mysql_query("SELECT * FROM `houses` WHERE MATCH(address, state, city, zip, footage) AGAINST ('$searchstr')") OR DIE(mysql_error()); Change that line out, there is a sql error.
-
Alright here is a shot in the dark, if your query should only return 1 row why put it into a while loop? This could be where the issue comes into play. <?php include('includes/header.php'); $username = $_POST['username']; $password = $_POST['password']; if(isset($_POST['username']) || isset($_POST['password'])){ if (!$link = mysql_connect($sqlserver, $sqluser, $sqlpassword)) { echo 'Could not connect to mysql'; exit; } if (!mysql_select_db($sqldb, $link)) { echo 'Could not select database'; exit; } $query="SELECT * FROM users WHERE username = '$username'"; $result = mysql_query($query); if(mysql_num_rows($result) < 1){echo "<p style=\"color:red\">Incorrect Email/Password Combination.</p>";}else{ $row = mysql_fetch_array($result); if(md5($password) == $row['password']){ $_SESSION['uid'] = $row['uid']; $_SESSION['username'] = $row['username']; $_SESSION['password'] = $row['password']; $_SESSION['email'] = $row['email']; $_SESSION['ip'] = $row['ip']; echo "<br /><p style=\"color:green\">Login successful.</p>"; }else{echo "<p style=\"color:red\">Incorrect Email/Password Combination.</p>";} } } if($_GET['logout'] == "true"){ session_destroy(); echo "<p>Logged Out.</p>"; } echo " <h2>Login</h2> <form method=\"post\" action=\"".$_SERVER['PHP_SELF']."\"> <p>Username:<br /><input type=\"text\" name=\"username\" size=\"30\" /></p> <p>Password:<br /><input type=\"password\" name=\"password\" size=\"30\" /></p> <p><input type=\"submit\" value=\"Log In\" /></p> </form> "; include('includes/footer.php'); ?> As long as usernames are unique, there is no need for the while, and it could be overriding the good setting. Try that.
-
Does your DB Column type allow nulls?
-
I think this is what wildteen means if (isset($_POST['art_image'])) { $art_image = "'" . $_POST['art_image'] . "'"; } else { $art_image = NULL; } //run the query which adds the data gathered from the form into the database $result = mysql_query("INSERT INTO az_articles (art_title, section, category, issue, art_image, art_text) VALUES ('$art_title','$section', '$category', '$issue'," . $art_image . ",'$art_text')"); See if that works.
-
I am not sure if php can read jscript cookies, but you should be able to use document.cookie with javascript (google javascript set cookies to find out more)