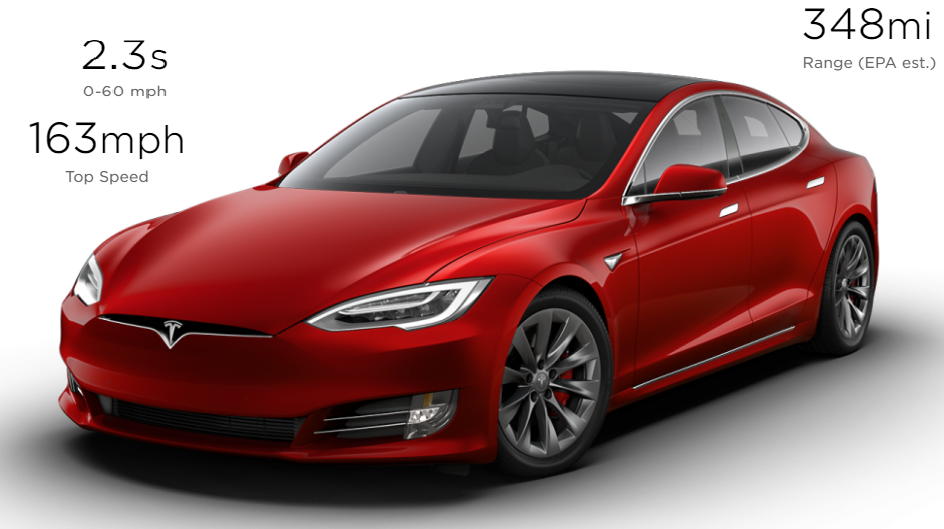
Coreye
Members-
Posts
537 -
Joined
-
Last visited
Everything posted by Coreye
-
Try this code and let me know if any errors appear. <?php ini_set('display_errors', 1); if(!empty($_POST['cont'])) { $species = $_POST['species']; include "db.php"; if(empty($_POST['name']) || empty($_POST['Species'])) { echo "<script>document.write('You must input a species and a name!'); setTimeout(\"window.location.reload()\", 350);</script>"; } if($species == "Predator" || $species == "Alien" || $species == "Marine" && !empty($_POST['name'])) { mysql_query("INSERT INTO `rpg` (`name`, `species`, `player`) VALUES ('{$_POST['name']}', '{$_POST['Species']}', '{$_POST['player']}')") OR die(mysql_error()); } elseif($species == "Predator") { mysql_query("UPDATE `rpg` SET `max_health` = 25, `health` = 25, `attack` = 25, `defense` = 25, `honor` = 20 WHERE `player` = '{$_POST['player']}')") OR die(mysql_error()); } elseif($species == "Alien") { mysql_query("UPDATE `rpg` SET `health` = 20, `max_health` = 20, `attack` = 20 , `defense` = 20, `stealth` = 20 WHERE `player` = '{$_POST['player']}')") OR die(mysql_error()); } elseif($species == "Marine") { mysql_query("UPDATE `rpg` SET `health` = 15, `max_health` = 15, `attack` = 20, `defense` = 20, `melee` = 20 WHERE `player` = '{$_POST['player']}')") OR die(mysql_error()); } else { echo "Invalid Species. Try again!"; } mysql_query("INSERT INTO `bank` (`player`, `amount`) VALUES('{$_SESSION['user_id']}', 5)") OR die(mysql_error()); header('Location: statadd.php'); } ?>
-
You need to define your $username and $password variables. The same with $previous, $next, $update and $delete. <?php error_reporting(E_ALL); $username = $_POST['username']; $password = $_POST['password']; if($username == "xxxxx" && $password == "xxxxxx") { setcookie("username", $username, time()+1200); echo "<h2>Administrator Access Approved</h2><hr>"; echo "You can now update the monthly special."; } else { setcookie("username", "", time()-3600); echo "<h2>Access Denied</h2><hr>"; echo "The User Name and Password you entered are incorrect."; } ?>
-
Hi there, When you say it's not working, what's it doing? Are there any errors?
-
Do other PHP scripts work on your server? Try this code: <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN"> <html> <head> <title>A 'Simple' PHP Calendar</title> </head> <body> <p align="center"><b><a href="./Graded_Project2_Calendar.php">Today</a> is: <?php /* Display today's date via the PHP date() function */ printf($date = time()); ?> <br /><br /> <table width="700" border="1" cellspacing="0" cellpadding="4" align="center"> <tr> <td colspan="7" height="55" align="center" valign="middle"><font size="6"> <?php /* Check for the $Year and $Month parameters. If they are null then we assume that the Year and Month to displayed will be the current Year and Month, and therefore set the $Year and $Month varaibles with the PHP date() function. Otherwise we will used the values passed in. */ if ($Year=="") { $Year = date("Y"); } if ($Month=="") { $Month = date("m"); } /* Display the Month and Year for the Calendar we are going to show. */ switch($Month) { case "1": printf("January"); break; case "2": printf("February"); break; case "3": printf("March"); break; case "4": printf("April"); break; case "5": printf("May"); break; case "6": printf("June"); break; case "7": printf("July"); break; case "8": printf("August"); break; case "9": printf("September"); break; case "10": printf("October"); break; case "11": printf("November"); break; case "12": printf("December"); break; } printf(" %d",$Year); /* Figure out how many days are in this month using the PHP checkdate() Function. We will loop and increment the $Day_Counter until we exceeed the number of Days for the Month [when the checkdate() function then returns False]. */ $Days_In_Month = 1; while (checkdate($Month,$Days_In_Month,$Year)): $Days_In_Month++; endwhile; /* Now we need to figure out which day of the week the first day of the month falls under. */ if ( date("l", mktime(0,0,0,$Month,1,$Year)) == "Sunday" ) { $Day_Of_Week = 1; } if ( date("l", mktime(0,0,0,$Month,1,$Year)) == "Monday" ) { $Day_Of_Week = 2; } if ( date("l", mktime(0,0,0,$Month,1,$Year)) == "Tuesday" ) { $Day_Of_Week = 3; } if ( date("l", mktime(0,0,0,$Month,1,$Year)) == "Wednesday" ) { $Day_Of_Week = 4; } if ( date("l", mktime(0,0,0,$Month,1,$Year)) == "Thursday" ) { $Day_Of_Week = 5; } if ( date("l", mktime(0,0,0,$Month,1,$Year)) == "Friday" ) { $Day_Of_Week = 6; } if ( date("l", mktime(0,0,0,$Month,1,$Year)) == "Saturday" ) { $Day_Of_Week = 7; } ?> </font></td> </tr> <tr> <td align="center" width="100" height="30" bgcolor="#EFEFEF"><b>Sunday</b></td> <td align="center" width="100" bgcolor="#EFEFEF"><b>Monday</b></td> <td align="center" width="100" bgcolor="#EFEFEF"><b>Tuesday</b></td> <td align="center" width="100" bgcolor="#EFEFEF"><b>Wednesday</b></td> <td align="center" width="100" bgcolor="#EFEFEF"><b>Thursday</b></td> <td align="center" width="100" bgcolor="#EFEFEF"><b>Friday</b></td> <td align="center" width="100" bgcolor="#EFEFEF"><b>Saturday</b></td> </tr> <tr> <?php $Row = 1; $Col = 1; $Day_Counter = 1; if ($Day_Of_Week > $Day_Counter) { for($Col = 1; $Col < $Day_Of_Week; $Col++) { printf("<td height=\"100\" valign=\"top\"> </td>"); } for($Col = $Col; $Col <= 7; $Col++) { printf("<td height=\"100\" valign=\"top\"><b>%d</b>", $Day_Counter); printf("</td>"); $Day_Counter++; } $Col = 1; $Row++; } else { printf("<tr>"); for($Col = $Col; $Col <= 7; $Col++) { printf("<td height=\"100\" valign=\"top\"><b>%d</b>", $Day_Counter); printf("</td>"); $Day_Counter++; } $Col = 1; $Row++; printf("</tr>"); } for($Row = $Row; $Row <= 6; $Row++) { printf("<tr>"); for($Col = $Col; $Col <= 7; $Col++) { if($Day_Counter >= $Days_In_Month) { printf("<td height=\"100\" valign=\"top\"> "); } else { printf("<td height=\"100\" valign=\"top\"><b>%d</b>", $Day_Counter); } printf("</td>"); $Day_Counter++; } $Col = 1; printf("</tr>"); } ?> </tr> <tr> <td align="center" valign="middle" height="30" bgcolor="#EFEFEF"> <?php $Last_Year = ($Year-1); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=%d\">%d</a>", $Last_Year , $Month, $Last_Year); ?> </td> <td colspan="5" align="center" valign="middle" bgcolor="#EFEFEF"> <?php printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=1\">Jan.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=2\">Feb.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=3\">Mar.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=4\">Apr.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=5\">May</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=6\">Jun.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=7\">Jul.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=8\">Aug.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=9\">Sep.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=10\">Oct.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=11\">Nov.</a> | ", $Year); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=12\">Dec.</a>", $Year); ?> </td> <td align="center" valign="middle" bgcolor="#EFEFEF"> <?php $Next_Year = ($Year+1); printf("<a href=\"./Graded_Project2_Calendar.php?Year=%d&Month=%d\">%d</a>", $Next_Year , $Month, $Next_Year); ?> </td> </tr> </table> </body> </html>
-
What problems are you having? Post the code and we'll be able to help you better...
-
Hi there, printf$date =time () ; needs to be printf($date = time());
-
Cross Site Scripting: You can submit ">code in the website field. I would check to make sure it's a valid domain name.
-
Full Path Disclosure: http://beta.imuzic.co.uk/download.php?id[] Full Path Disclosure: http://beta.imuzic.co.uk/files/search.php?q[] Full Path Disclosure: http://beta.imuzic.co.uk/static/search/test&page=0 Full Path Disclosure: http://beta.imuzic.co.uk/static/search/test&page[] Full Path Disclosure: http://beta.imuzic.co.uk/files/more.php?id[]
-
http://www.phpfreaks.com/forums/index.php/topic,95867.0.html.
-
Cross Site Scripting(XSS): You can submit code when registering and it will execute on the members page. http://mythscape.freezoka.com/member.php Cross Site Scripting(XSS): You can submit ">code when editing the profile field "website" and it will execute when viewing a profile. http://mythscape.freezoka.com/profile.php?username=test
-
Add error reporting to the top of the script and see what it says. error_reporting(E_ALL); ini_set("display_errors", 1);
-
http://js-fireworks.appspot.com/?msg=PHPFreaks.com - That's awesome, lol.
-
Like I said here, you can also sell domain names on SitePoint.
-
I use FireFox. Chrome looks alright but I have no plans to switch to it .
-
Be sure to read this post: http://www.phpfreaks.com/forums/index.php/topic,232470.0.html.
-
Full Path Disclosure: When you submit ' you get this: Full Path Disclosure: When you submit single letters or strings, you get this:
-
[SOLVED] Only display certain amount of a text variable
Coreye replied to jeff5656's topic in PHP Coding Help
I would check out this thread: http://www.phpfreaks.com/forums/index.php?topic=162428. -
Hey, I just tested that script and it ran fine.
-
Try this: $connectToInboxQuery = "SELECT * FROM `messages` WHERE `touser` = '" . $_SESSION["theUSERNAME"] . "' AND `read` = '0'";
-
Cross Site Scripting (XSS): http://media-script.com/index.php?action=browsev&cat="><marquee><h1>test Cross Site Scripting (XSS): http://media-script.com/index.php?action=addfav&cat="><marquee><h1>test Cross Site Scripting (XSS): You can submit ">code and it will execute when using search. Cross Site Scripting (XSS): You can submit ">code and it will execute when editing your profile. You can submit comments for jokes, videos and games that don't exist. http://media-script.com/index.php?action=joke&id=0 http://media-script.com/index.php?action=video&id=0 http://media-script.com/index.php?action=game&id=0
-
[php] How to check if the input variable is integer?
Coreye replied to michalchojno's topic in PHP Coding Help
Try this: <?php if (empty($val)) { $val = "0"; } if (!ctype_digit($val)) { die("Error"); } ?> -
Getting blank screen when trying to include or redirect >:|
Coreye replied to deleet's topic in PHP Coding Help
I'm not sure if this is the problem but $SESSION['email']=$row['email']; should be $_SESSION['email'] = $row['email']; -
When I use the code above, it works correctly. Is that all of the code?
-
Some thing in your script is adding the word "Error". Post your code and we might be able to tell you .
-
Your error checking doesn't work very well either. Even if they don't fill in all the fields, an email will still be sent. You need to include the mail() function into the if() part.