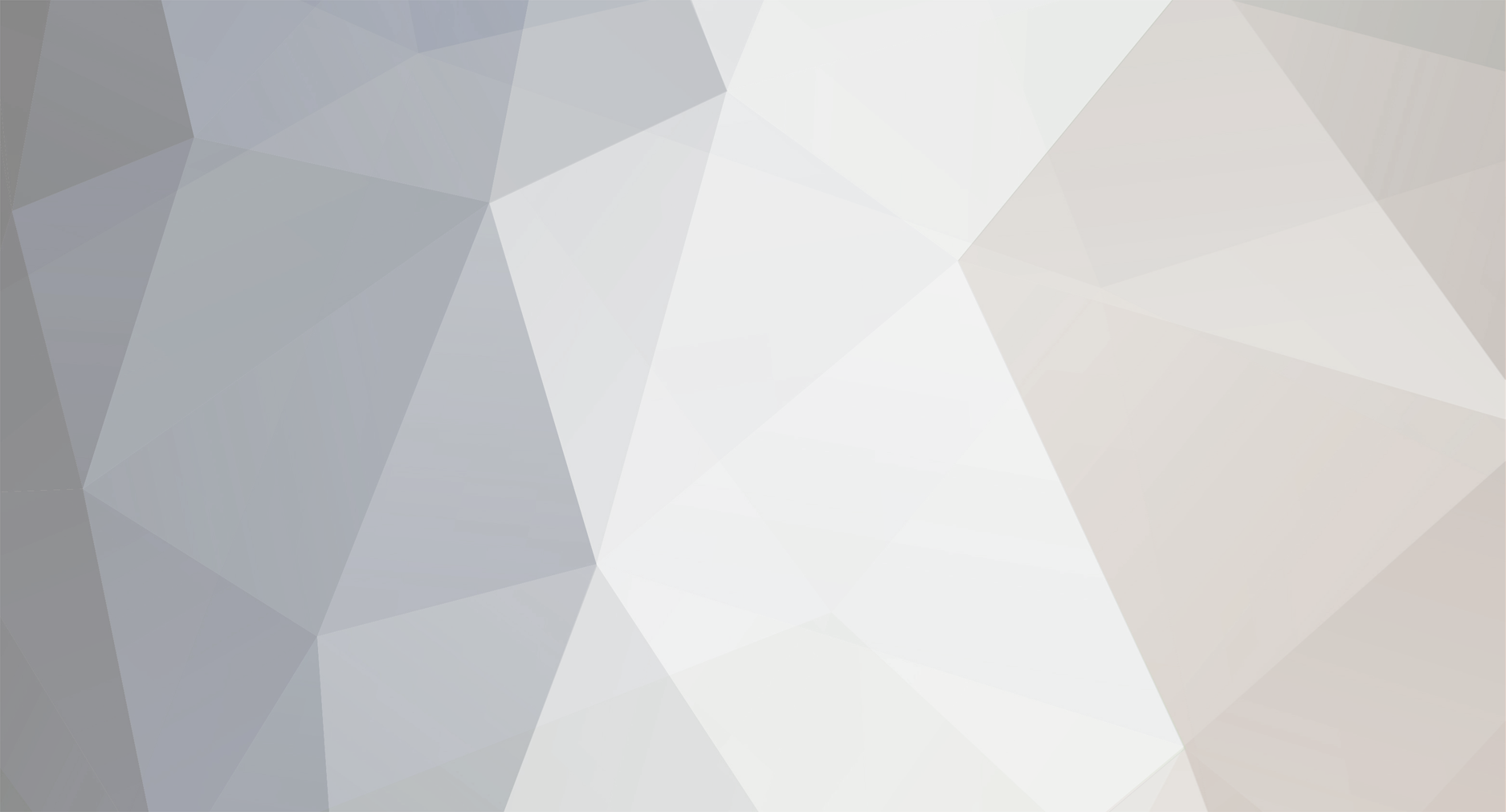
jakebur01
Members-
Posts
885 -
Joined
-
Last visited
Everything posted by jakebur01
-
This is the login-check.php page, which is the only thing check to see if the user is logged in. This is required at the top of all the admin pages. require "client-info.php"; session_start(); $ses_id = session_id(); $db = mysql_connect("localhost",$dbusername,$dbpassword); mysql_select_db($database,$db); $sql="select ses_id from $users_table where user_name like '$login'"; $result=mysql_query($sql,$db); $myrow=mysql_fetch_array($result); if($ses_id==$myrow[ses_id]) {} else { header( 'Location: index.php' ) ; }
-
Hello, I have a friend whose login has been getting hacked into. There is not any evidence that they have been posting a username and password. We are thinking they could be getting in using a cookie or session somehow. <?php require 'client-info.php'; if($submit) { $db = mysql_connect("localhost",$dbusername,$dbpassword); mysql_select_db($database,$db); $sql="select * from $users_table where user_name='$login'"; $result=mysql_query($sql,$db); $myrow=mysql_fetch_array($result); if($myrow['user_password']==$password and $myrow['user_name']==$login) { session_start(); $ses_id = session_id(); $sql2 = "UPDATE $users_table SET ses_id='$ses_id' where user_name like '$login'"; $result2=mysql_query($sql2); require 'admin-panel.php'; } else { echo "<br><br><tr><td align=center><font face=arial size=4 >"; echo "Incorrect login information. Hit your back key to try again."; echo "</font><br><br></td></tr>"; } } else { session_start(); $ses_id = session_id(); $db = mysql_connect("localhost",$dbusername,$dbpassword); mysql_select_db($database,$db); $sql="select ses_id from $users_table where user_name like '$login'"; $result=mysql_query($sql,$db); $myrow=mysql_fetch_array($result); if($ses_id==$myrow[ses_id]) {require 'admin-panel.php';} else { require 'login-form.php';} } ?> Thanks, Jake
-
There are thousands of hosting companies out there. I am trying to get opinions of the best.
-
I could not find a hosting section to post under, so I figured I would be ok posting here in PHP Help. I am currently hosting with a guy who has a windows server that he manages that is located in a data center in Dallas, TX. He is currently charging my clients $20 a month and giving me half. I am considering switching to another host where I do not have to call him for every little thing. I manage about 15 companies, each have PHP & MySQL. I am looking for some insight for the best host for PHP and ease of managing 10 - 15 seperate companies. Thanks, Jake
-
So are you saying that even though the html redirect is at the bottom of the pup page that it could actually execute before the page finishes loading?
-
What is the best way to redirect to a new page after the page has fully loaded? Would this require javascript. I realize I header redirect must be put before all other code, but I need it at the bottom of the script. - Jake
-
[SOLVED] passing where I am at to a new page
jakebur01 replied to jakebur01's topic in PHP Coding Help
Something isn't right. I'm getting PHP Fatal error: Allowed memory size of 67108864 bytes exhausted (tried to allocate 840 bytes). Something else must be out of place. It won't even do one row if I change $rows_per_loop to 1. I also changed if ($limit_end==$total_rows) { $s=$rows_per_loop; } } to if ($limit_end==$total_rows) { $i=$rows_per_loop; } } -
[SOLVED] passing where I am at to a new page
jakebur01 replied to jakebur01's topic in PHP Coding Help
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'OFFSET ''' at line 1 I also added a missing bracket in the first if statement near $_GET. -
I am having trouble putting this together. I have tried a few things, but I have not been able to figure it out. 1. I am trying to run 25 rows. 2. Pass where I am at to the next page using $_GET and run the next set of 25 rows. 3. and continue until finished <?php if (isset($_GET['limit_start'])) { $limit_start=$_GET['limit_start']; $limit_end=$_GET['limit_end']; } ini_set('max_execution_time', '999'); // example of how to use basic selector to retrieve HTML contents include('simple_html_dom.php'); $source_file = "C:/Inetpub/Websites/edit.com/echo.txt"; $fp= fopen("$source_file", "a"); $other="\n"; $db = mysql_connect('localhost', 'xx', 'xx') or die(mysql_error()); mysql_select_db('xx') or die(mysql_error()); $total_rows = mysql_num_rows(mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK'", $db)); $rows_per_loop = 25; $total_loops = ceil($total_rows/$rows_per_loop); // run the loop, while loop counter is less than the total number of loops: for($s=0; $s<$total_loops; $s++) { // get the numbers for the limit, // start is just the current loop number multiplied by the rows per loop // and end is loop counter + 1, multiplied by the rows per loop $limit_start = $rows_per_loop*$s; $limit_end = $rows_per_loop*($s+1); $result = mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK' LIMIT $limit_start, $limit_end") or die(mysql_error()); while($myrow = mysql_fetch_array ($result)) { $zip=$myrow['zip_code']; // get DOM from URL or file $html=file_get_html("http://www.mysite.com/selector.php?transaction=search&template=map_search&search1=0&pwidth=400&pheight=700&proxIconId=400&proxIcons=1&search2=0&search3=1&country=US&searchQuantifier=AND&address=&city=&stateProvince=+&postalCode=$zip&radius=500&x=78&y=16"); $i = 0; $tmp = $html->find('span[class=mqEmp], span[class=Black11]'); $cnt = count($tmp) - 1; foreach($tmp as $e) { if($i > 0 && $i < $cnt){ $outputstring=$e->plaintext; $outputstring=$outputstring. "\t"; fwrite($fp, $outputstring, strlen($outputstring)); if ($i % 8 == 0) { fwrite($fp, $other, strlen($other)); } } $i++; } } // now that we've run those, let's clear the results so that we don't run out of memory. mysql_free_result($result); // show where we are at echo $limit_start,' - ',$limit_end,'<br />'; sleep(1); // give PHP a little nap to keep from overloading server ob_flush(); // as recommended by MadTechie, in case we go beyond the max execution time flush(); // add flush, to make sure it is outputted header( "location:http://www.edit.com/selector.php?limit_start=$limit_start&limit_end=$limit_end" ); } fclose($fp); ?>
-
dude... I edited it so everybody wouldn't see exactly all of my business. 1. I am just trying to run 25 rows. 2. Pass where I am at to the next page using $_GET and run the next set of 25 rows. 3. and continue until finished
-
<?php if (isset($_GET['limit_start'])) { $limit_start=$_GET['limit_start']; $limit_end=$_GET['limit_end']; } ini_set('max_execution_time', '999'); // example of how to use basic selector to retrieve HTML contents include('simple_html_dom.php'); $source_file = "C:/Inetpub/Websites/edit.com/echo.txt"; $fp= fopen("$source_file", "a"); $other="\n"; $db = mysql_connect('localhost', 'xx', 'xx') or die(mysql_error()); mysql_select_db('xx') or die(mysql_error()); $total_rows = mysql_num_rows(mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK'", $db)); $rows_per_loop = 25; $total_loops = ceil($total_rows/$rows_per_loop); // run the loop, while loop counter is less than the total number of loops: for($s=0; $s<$total_loops; $s++) { // get the numbers for the limit, // start is just the current loop number multiplied by the rows per loop // and end is loop counter + 1, multiplied by the rows per loop $limit_start = $rows_per_loop*$s; $limit_end = $rows_per_loop*($s+1); $result = mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK' LIMIT $limit_start, $limit_end") or die(mysql_error()); while($myrow = mysql_fetch_array ($result)) { $zip=$myrow['zip_code']; // get DOM from URL or file $html=file_get_html("http://www.www.edit.com/selector.php?transaction=search&template=map_search&search1=0&pwidth=400&pheight=700&proxIconId=400&proxIcons=1&search2=0&search3=1&country=US&searchQuantifier=AND&address=&city=&stateProvince=+&postalCode=$zip&radius=500&x=78&y=16"); $i = 0; $tmp = $html->find('span[class=mqEmp], span[class=Black11]'); $cnt = count($tmp) - 1; foreach($tmp as $e) { if($i > 0 && $i < $cnt){ $outputstring=$e->plaintext; $outputstring=$outputstring. "\t"; fwrite($fp, $outputstring, strlen($outputstring)); if ($i % 8 == 0) { fwrite($fp, $other, strlen($other)); } } $i++; } } // now that we've run those, let's clear the results so that we don't run out of memory. mysql_free_result($result); // show where we are at echo $limit_start,' - ',$limit_end,'<br />'; sleep(1); // give PHP a little nap to keep from overloading server ob_flush(); // as recommended by MadTechie, in case we go beyond the max execution time flush(); // add flush, to make sure it is outputted header( "location:http://www.edit.com/selector.php?limit_start=$limit_start&limit_end=$limit_end" ); } fclose($fp); ?>
-
I tried this, but it is not functioning right. It is looping through and sending multiple headers without executing it. I added this at the top. if (isset($_GET['limit_start'])) { $limit_start=$_GET['limit_start']; $limit_end=$_GET['limit_end']; } And this at the bottom // now that we've run those, let's clear the results so that we don't run out of memory. mysql_free_result($result); // show where we are at echo $limit_start,' - ',$limit_end,'<br />'; sleep(1); // give PHP a little nap to keep from overloading server ob_flush(); // as recommended by MadTechie, in case we go beyond the max execution time flush(); // add flush, to make sure it is outputted header( "location:http://www.mysite/example.php?limit_start=$limit_start&limit_end=$limit_end" ); } fclose($fp);
-
That's kind of what I already have going. Now I am trying to go to a new page after processing 25 rows and pick up where I left off using $_GET.
-
How could I redirect to myself and use Get to pass the last ID limiting 25 each time? example..... run 25 then redirect to: mypage.php?offset=25 run 25 more then redirect to mypage.php?offset=50 and so on.. $total_rows = mysql_num_rows(mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK'", $db)); $rows_per_loop = 20; $total_loops = ceil($total_rows/$rows_per_loop); // run the loop, while loop counter is less than the total number of loops: for($s=0; $s<$total_loops; $s++) { // get the numbers for the limit, // start is just the current loop number multiplied by the rows per loop // and end is loop counter + 1, multiplied by the rows per loop $limit_start = $rows_per_loop*$s; $limit_end = $rows_per_loop*($s+1); $result = mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK' LIMIT $limit_start, $limit_end") or die(mysql_error()); while($myrow = mysql_fetch_array ($result)) { $zip=$myrow['zip_code']; // get DOM from URL or file $html=file_get_html("http://www.mysite.com/age.asp?transaction=search&template=map_search&search1=0&pwidth=400&pheight=700&proxIconId=400&proxIcons=1&search2=0&search3=1&country=US&searchQuantifier=AND&address=&city=&stateProvince=+&postalCode=$zip&radius=500&x=78&y=16"); $i = 0; $tmp = $html->find('span[class=mqEmp], span[class=Black11]'); $cnt = count($tmp) - 1; foreach($tmp as $e) { if($i > 0 && $i < $cnt){ $outputstring=$e->plaintext; $outputstring=$outputstring. "\t"; fwrite($fp, $outputstring, strlen($outputstring)); if ($i % 8 == 0) { fwrite($fp, $other, strlen($other)); } } $i++; } } // now that we've run those, let's clear the results so that we don't run out of memory. mysql_free_result($result); // show where we are at echo $limit_start,' - ',$limit_end,'<br />'; sleep(1); // give PHP a little nap to keep from overloading server ob_flush(); // as recommended by MadTechie, in case we go beyond the max execution time flush(); // add flush, to make sure it is outputted } fclose($fp);
-
How could I make this like refresh to a new page limiting 25 each time? Like..... run 25 then redirect to: mypage.php?offset=25 run 25 more then redirect to mypage.php?offset=50 and so on..
-
bump
-
Is there any other way to give it a break in between?
-
I have tried that. I was referring to something that would maybe release the memory more often or something.
-
bump...
-
How can I free up the memory on this script to keep from getting " 0 - 20 20 - 40 PHP Fatal error: Allowed memory size of 134217728 bytes exhausted (tried to allocate 1992 bytes) in ?" $total_rows = mysql_num_rows(mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK'", $db)); $rows_per_loop = 20; $total_loops = ceil($total_rows/$rows_per_loop); // run the loop, while loop counter is less than the total number of loops: for($s=0; $s<$total_loops; $s++) { // get the numbers for the limit, // start is just the current loop number multiplied by the rows per loop // and end is loop counter + 1, multiplied by the rows per loop $limit_start = $rows_per_loop*$s; $limit_end = $rows_per_loop*($s+1); $result = mysql_query("SELECT * FROM zip_code where `state_prefix` = 'OK' LIMIT $limit_start, $limit_end") or die(mysql_error()); while($myrow = mysql_fetch_array ($result)) { $zip=$myrow['zip_code']; // get DOM from URL or file $html=file_get_html("http://www.mysite.com/age.asp?transaction=search&template=map_search&search1=0&pwidth=400&pheight=700&proxIconId=400&proxIcons=1&search2=0&search3=1&country=US&searchQuantifier=AND&address=&city=&stateProvince=+&postalCode=$zip&radius=500&x=78&y=16"); $i = 0; $tmp = $html->find('span[class=mqEmp], span[class=Black11]'); $cnt = count($tmp) - 1; foreach($tmp as $e) { if($i > 0 && $i < $cnt){ $outputstring=$e->plaintext; $outputstring=$outputstring. "\t"; fwrite($fp, $outputstring, strlen($outputstring)); if ($i % 8 == 0) { fwrite($fp, $other, strlen($other)); } } $i++; } } // now that we've run those, let's clear the results so that we don't run out of memory. mysql_free_result($result); // show where we are at echo $limit_start,' - ',$limit_end,'<br />'; sleep(1); // give PHP a little nap to keep from overloading server ob_flush(); // as recommended by MadTechie, in case we go beyond the max execution time flush(); // add flush, to make sure it is outputted } fclose($fp);
-
Wow! That's exactly what I was looking for. Thanks a lot man.
-
Yea, but I need it to repeat after every eight rows.
-
I have searched and not been able to figure how to return to a new line after 8 number of loops or tabs when writing to a text file. I would like to write 8 fields then tab to a new line. Something like: $i = 0; $tmp = $html->find('span[class=mqEmp], span[class=Black11]'); $cnt = count($tmp) - 1; foreach($tmp as $e) { if($i > 0 && $i < $cnt){ $outputstring=$e->plaintext . '\t'; fwrite($fp, $outputstring, strlen($outputstring)); // if eighth field, then start a new row something like: fwrite($fp, \n); } $i++; }
-
It is not to bad if I limit it by one state at a time. It is actually faster than I expected after I fixed that little quote issue. I have search and not been able to figure how to tab after x number of loops when writing to a text file. I would like to write 8 fields then tab to a new line. Something like: $i = 0; $tmp = $html->find('span[class=mqEmp], span[class=Black11]'); $cnt = count($tmp) - 1; foreach($tmp as $e) { if($i > 0 && $i < $cnt){ $outputstring=$e->plaintext . '\t'; fwrite($fp, $outputstring, strlen($outputstring)); // if eighth field, then start a new row something like: fwrite($fp, \n); } $i++; }
-
I put double quotes instead of single quotes on file_get_html(), which corrected my $zip variable. But, it is still taking a few seconds even when I use LIMIT 1 in the query.