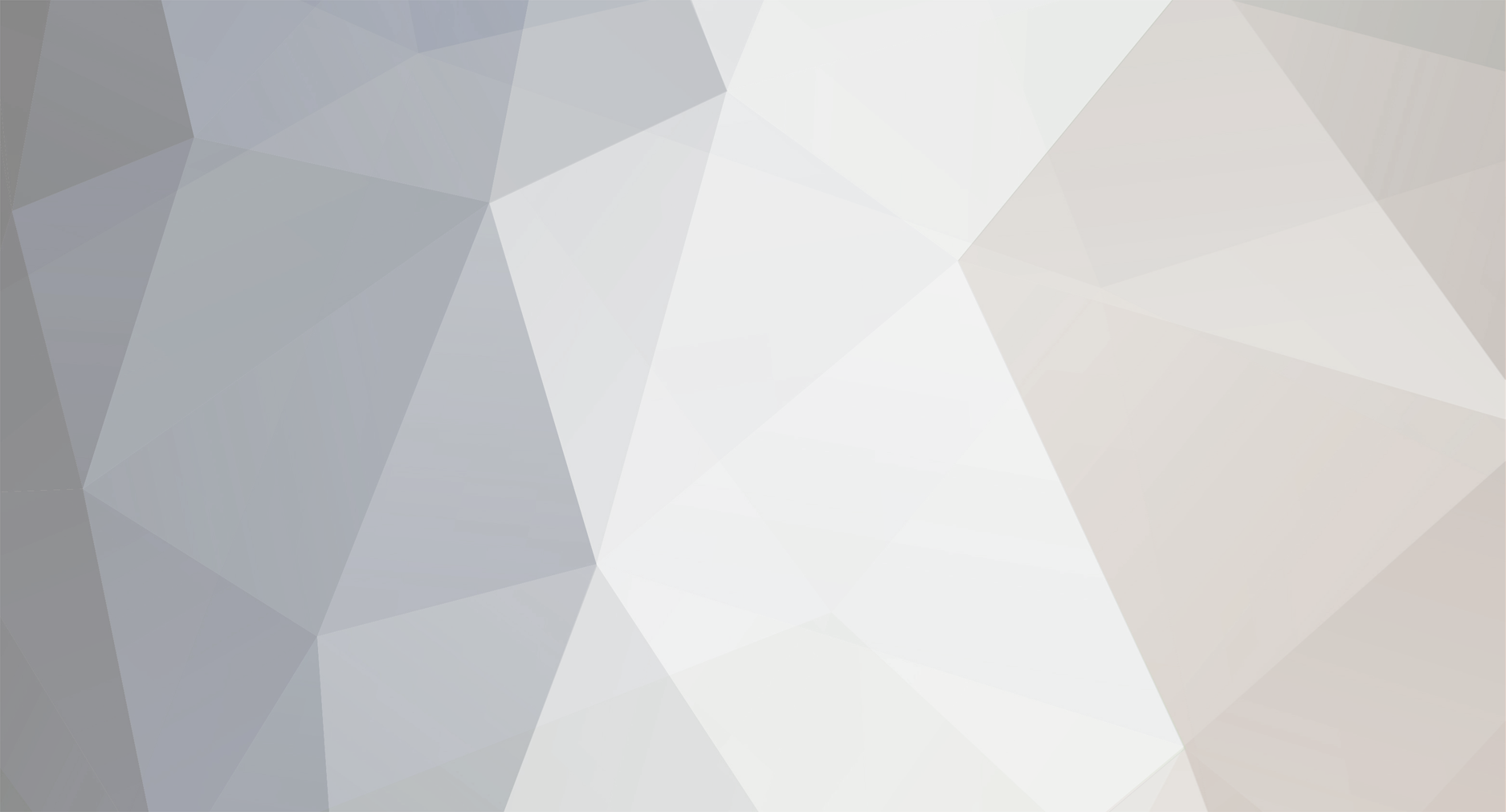
jakebur01
Members-
Posts
885 -
Joined
-
Last visited
Everything posted by jakebur01
-
Thanks. It works good all except for trunicating the file. Fatal error: Call to undefined function get_put_contents() in C:\Inetpub\wwwroot\smithsadvantage\failed_messages.php on line 39
-
I am going to use odbc to look at the inventory file and grab the Name, Account #, and Telephone number associated with the returned address. Then, insert it all into a MySQL database. Then, every evening I am going to schedule a php script that will run and look at the table every night. If there is any data in it, I will have it e-mail one of the ladies in the office then trunicate the table. If there is not any data in the table, it will do nothing.
-
I found this: $myFile = "testFile.txt"; $fh = fopen($myFile, 'r'); $theData = fgets($fh); fclose($fh); echo $theData; But, I am not sure that this will loop through each line??
-
I have a program that is storing failed, returned messages into a text file. I would like PHP to look at each address and handle it, then trunicate the text file, in other words wipe it clean. Here is an example of how it is storing the addresses into the text file: [email protected] [email protected] [email protected] [email protected]
-
This is really what I am looking to do. Get the failed address, query the customer database to get the customers info based on the failed address, then store this line into the MySQL failed_messages table. Then, ever so often I can send this list to someone in the office who can correct these wrong addresses. <?php include_once('inc/data.inc'); $failedaddress= $popmonger->FailedAddress; if (!$conn) {exit("Connection Failed: " . $conn);} $sql="SELECT CUSTOMER_NUM, CUSTOMER_NAME, PHONE_1 FROM AR_CUST_MAST WHERE EMAIL_1 = '$failedaddress'"; //print $sql; $rs=odbc_exec($conn,$sql); if (!$rs) {exit("Error in SQL");} setlocale(LC_MONETARY, 'en_US'); while (odbc_fetch_row($rs)) { $acct=odbc_result($rs,"CUSTOMER_NUM"); $name=odbc_result($rs,"CUSTOMER_NAME"); $phone=odbc_result($rs,"PHONE_1"); } $acct =mysql_real_escape_string($acct); $name =mysql_real_escape_string($name); $phone =mysql_real_escape_string($phone); $query = "insert into failed_messages values ('0','$acct', '$name', '$phone', '$failedaddress')"; mysql_query($query, $db); ?>
-
I changed it, but I still get the same error in the log file. And the data does not insert. <?php include_once('inc/data.inc'); $query = "insert into failed_messages values ('0','0', '0', '0', '0')"; mysql_query($query, $db) or die('could not query'); ?> [attachment deleted by admin]
-
I know this is not an error that php generates. But, I am using a program called popmonger that handles failed messages and it will run a php script to handle the message. I have it pointed to my php script. And in the log file it is throwing this error: Name cannot begin with the '' character, hexadecimal value 0x20. Line 1, position 12. Code I am using for testing: <?php include_once('inc/data.inc'); $query = "insert into failed_messages values ('0','0', '0', '0', '0')" or die('Could not insert'); mysql_query($query, $db) or die('could not query'); ?>
-
Count how many months it is back to November.
jakebur01 replied to jakebur01's topic in PHP Coding Help
Thanks. I will change it to your update and test it out. So, far this code below is working great. I wound up changing $prevEnd to 15 since it will always be SALES_PD_15. $curEnd = 27; // 27 $curStart = 27 - abs(11-date('m')); // 27 - 6 = 21 $prevEnd = 15; // 15 $prevStart = $prevEnd - abs(11-date('m'));//15-6=9 $McurTotal = 0; $MprevTotal = 0; while (odbc_fetch_row($rs)) { for ($col = $curStart; $col <= $curEnd; $col++) { if ($col == 1) $colName = 'SALES_OLDEST_PD1'; elseif($col == 26) $colName = 'SALES_LAST_PD_26'; elseif($col == 27) $colName = 'SALES_CURR_PD_27'; else $colName = 'SALES_PD_' . $col; $McurTotal += odbc_result($rs,$colName); } for ($col = $prevStart; $col <= $prevEnd; $col++) { if ($col == 1) $colName = 'SALES_OLDEST_PD1'; elseif($col == 26) $colName = 'SALES_LAST_PD_26'; elseif($col == 27) $colName = 'SALES_CURR_PD_27'; else $colName = 'SALES_PD_' . $col; $MprevTotal += odbc_result($rs,$colName); } $M27 += odbc_result($rs,"SALES_CURR_PD_27"); $M15 += odbc_result($rs,"SALES_PD_15"); } /* $MyearDiff= 100-($MprevTotal*100/$McurTotal); $MmonthDiff= 100-($M15*100/$M27); $MyearDiff = number_format($MyearDiff, 2, '.', ','); $MmonthDiff = number_format($MmonthDiff, 2, '.', ','); */ $McurTotal = number_format($McurTotal, 2, '.', ','); $MprevTotal = number_format($MprevTotal, 2, '.', ','); $M27 = number_format($M27, 2, '.', ','); $M15 = number_format($M15, 2, '.', ','); -
Count how many months it is back to November.
jakebur01 replied to jakebur01's topic in PHP Coding Help
Thank you. That is exactly was I was looking for. I changed $prevEnd to equal 15. $curEnd = 27; // 27 $curStart = 27 - abs(11-date('m')); // 27 - 6 = 21 $prevEnd = 15;// 15 $prevStart = $prevEnd - 11; // 20 - 11 = 9 I have a question. Looking to the future... Like when the date becomes November of this year, will "abs(11-date('m'))" equal zero? October of this year would equal 13.... And December of this year would equal 1? -
Count how many months it is back to November.
jakebur01 replied to jakebur01's topic in PHP Coding Help
In a round about way, this is what I'm trying to do. Append the numbers between the $start and $end variables to the "$M". Loop incrementing and totaling those odbc variables. while(loop $backtoNovember"7 times") { $MCYTD += append $M to numbers $MCYTDstart through $MCYTDend in order to total up the variables; } So, this would output something like. $MCYTD = $M21 + $MCYTD; $MCYTD = $M22 + $MCYTD; $MCYTD = $M23 + $MCYTD; $MCYTD = $M24 + $MCYTD; $MCYTD = $M25 + $MCYTD; $MCYTD = $M26 + $MCYTD; $MCYTD = $M27 + $MCYTD; _________________ Giving me the total Current Year to Date Sales. Which is stored in $MCYTD. -
Count how many months it is back to November.
jakebur01 replied to jakebur01's topic in PHP Coding Help
Just to clarify. In the example of today's date. $MCYTD would equal the sum of $M21 - $M27. $MLYTD would equal the sum of $M9 - $M15. -
Count how many months it is back to November.
jakebur01 replied to jakebur01's topic in PHP Coding Help
Ok, I think I'm getting somewhere now. Now I need to total the columns that are between $MLYTDstart and $MLYTDend, store it at variable $MLYTD. And total the columns between $MCYTDstart and $MCYTDend, store it at variable $MCYTD. I am not sure how to do this. I have the columns in variables $M1 - $M27. Do I need to do some kind of for loop or while loop and append the numbers to $M to total up the range? while (odbc_fetch_row($rs)) { $M1 += odbc_result($rs,"SALES_OLDEST_PD1"); $M2 += odbc_result($rs,"SALES_PD_2"); $M3 += odbc_result($rs,"SALES_PD_3"); $M4 += odbc_result($rs,"SALES_PD_4"); $M5 += odbc_result($rs,"SALES_PD_5"); $M6 += odbc_result($rs,"SALES_PD_6"); $M7 += odbc_result($rs,"SALES_PD_7"); $mate += odbc_result($rs,"SALES_PD_8"); $M9 += odbc_result($rs,"SALES_PD_9"); $M10 += odbc_result($rs,"SALES_PD_10"); $M11 += odbc_result($rs,"SALES_PD_11"); $M12 += odbc_result($rs,"SALES_PD_12"); $M13 += odbc_result($rs,"SALES_PD_13"); $M14 += odbc_result($rs,"SALES_PD_14"); $M15 += odbc_result($rs,"SALES_PD_15"); $M16 += odbc_result($rs,"SALES_PD_16"); $M17 += odbc_result($rs,"SALES_PD_17"); $M18 += odbc_result($rs,"SALES_PD_18"); $M19 += odbc_result($rs,"SALES_PD_19"); $M20 += odbc_result($rs,"SALES_PD_20"); $M21 += odbc_result($rs,"SALES_PD_21"); $M22 += odbc_result($rs,"SALES_PD_22"); $M23 += odbc_result($rs,"SALES_PD_23"); $M24 += odbc_result($rs,"SALES_PD_24"); $M25 += odbc_result($rs,"SALES_PD_25"); $M26 += odbc_result($rs,"SALES_LAST_PD_26"); $M27 += odbc_result($rs,"SALES_CURR_PD_27"); } $MCYTDstart=27-$backtoNovember; $MCYTDend=27; $MLYTDstart=$MCYTDstart - 12; $MLYTDend=$MLYTDstart + $backtoNovember; -
Count how many months it is back to November.
jakebur01 replied to jakebur01's topic in PHP Coding Help
No, it will always stay the same. November is the beginning of fiscal year for a business. I'm just trying to count back to November. -
Count how many months it is back to November.
jakebur01 replied to jakebur01's topic in PHP Coding Help
What about this? $start = date("m"); if($start<"11") { $backtoNovember= $start + 1; } elseif($start=="11") { $backtoNovember="0"; } else { $backtoNovember="1"; } -
Just to make things a lot simpler, I decided to start a new topic. My question is, how can I count how many months it is back to the past November? Example: May 2011 back to November would return 6. Example: Dec 2011 back to November would return 1. Example: October 2011 back to November would return 11. Jake
-
That's the way PHP was for me when I first starting dealing with it. I finally went and bought a good book on it and started from the beginning and gradually worked my way through it. It actually had a shopping cart in the back of it. By the time I got to the shopping cart, I knew my way around in the code.
-
You might could implement some sort of redirect or page refresh into some of your pages. Put it within an if statement so it doesn't continuously refresh or reload over and over. This is javascript, but I use it sometimes after a database insert or form submit to keep people from being able to refresh the page or hit the back button and submit the same form twice. <script type="text/javascript"> <!-- window.location = "http://www.google.com/" //--> </script>
-
Find a shopping cart tutorial. Build a basic cart that works off a MySQL table then add this other stuff in later. It would be that hard to do what your wanting to do, but you should build your foundation first.
-
Or rather find the number of how many months it is back to November? Example: If I could calculate that November is 6 months back. Store it in $backtoNov. I could subtract 6 from 27. That would help me in knowing that last November would be column SALES_PD_21. * The sum of the totals between SALES_CUR_PD and SALES_PD_21 would give me (CYTD)"current year to date sales". I know that 27 - 12 is 15, giving me the current month a year ago as SALES_PD_15. I could subtract $backtoNov from this, which is 6 in this case, giving me 9. So, last year to date's starting year in November would be SALES_PD_9. * The sum of the totals between SALES_PD_15 and SALES_PD_9 would give me (LYTD)"last year to date sales".
-
Well just as a starting place, how do I find last November? $Currmonth = mktime(0, 0, 0, date("m"), date("d"), date("y")); echo date("m", $Currmonth);
-
No. I can't do much of anything with it. It was built in a language called ProvideX. So, I do as much cool stuff as I can using PHP and the ProvideX ODBC. I've been using wget with a scheduled task to generate e-mail reports.
-
I have a table with Territory Representative Sales. It has columns that go back 26 months. The columns are SALES_OLDEST_PD_1, SALES_PD_2, SALES_PD_3, ..... SALES_PD_25, SALES_LAST_PD_26, & SALES_CURR_PD_27. SALES_CURR_PD_27 is the current month. I want to build a table with "Last Year To Date Sales" (LYTD), "Current Year To Date Sales" (CYTD), (May 2011), and (May 2010). I know that (May 2011) will be SALES_CURR_PD_27 and (May 2010) will be SALES_PD_15. The trick is that the sales year starts in November. So, I need to figure out a way to match the table columns with "Nov. 09 - May. 10 TOTAL" for (LYTD) and "Nov. 10 - May. 2011 TOTAL" for (CYTD). I am familiar with using mktime() to subtract months. But, I am not sure where to start yet on this project. Jake while (odbc_fetch_row($rs)) { $M1 += odbc_result($rs,"SALES_OLDEST_PD1"); $M2 += odbc_result($rs,"SALES_PD2"); $M3 += odbc_result($rs,"SALES_PD3"); $M4 += odbc_result($rs,"SALES_PD4"); $M5 += odbc_result($rs,"SALES_PD5"); $M6 += odbc_result($rs,"SALES_PD6"); $M7 += odbc_result($rs,"SALES_PD7"); $mate += odbc_result($rs,"SALES_PD8"); $M9 += odbc_result($rs,"SALES_PD9"); $M10 += odbc_result($rs,"SALES_PD10"); $M11 += odbc_result($rs,"SALES_PD11"); $M12 += odbc_result($rs,"SALES_PD12"); $M13 += odbc_result($rs,"SALES_PD13"); $M14 += odbc_result($rs,"SALES_PD14"); $M15 += odbc_result($rs,"SALES_PD15"); $M16 += odbc_result($rs,"SALES_PD16"); $M17 += odbc_result($rs,"SALES_PD17"); $M18 += odbc_result($rs,"SALES_PD18"); $M19 += odbc_result($rs,"SALES_PD19"); $M20 += odbc_result($rs,"SALES_PD20"); $M21 += odbc_result($rs,"SALES_PD21"); $M22 += odbc_result($rs,"SALES_PD22"); $M23 += odbc_result($rs,"SALES_PD23"); $M24 += odbc_result($rs,"SALES_PD24"); $M25 += odbc_result($rs,"SALES_PD25"); $M26 += odbc_result($rs,"SALES_LAST_PD26"); $M27 += odbc_result($rs,"SALES_CURR_PD27"); } Note: By the way SMF is changing $M"8" to $M"ate". lol
-
In your html form, put action="your_page.php". At the top of your page, put something like. if(isset($_POST['name'])) { $name =$_POST['name']; //connect to sql //then //mysql_query("UPDATE name WHERE `user` = '$user'"); }
-
bump
-
I am having trouble transferring data from provideX ODBC to MySQL using Navicat. So, I am wanting to write a php script that will write all of the data to a text file, then do a MySQL "DATA LOAD INFILE". I am VERY limited as far as the SQL functions that are available to me with the ODBC connection. The table does not have a special unique identifier other than the item number. This table I am pulling from has a little over 100,000 items. How can I get php to process the full 100,000 rows. I thought about getting it to process 5,000 rows sorted by item #> break > pass the last item to the next page > then process the next batch of 5,000 sorted by item # where the item is greater than the item processed on the last page. ?? Jake I have not tested this code yet. I just kind of threw it together. <?php set_time_limit(900); ini_set('max_execution_time', '999'); $myFile = "item_master.txt"; unlink($myFile); $fp = fopen("item_master.txt", "w"); require("..\inc/data.inc"); if (!$conn) {exit("Connection Failed: " . $conn);} $sql="SELECT ITEM_NUM, DESCRIPTION_1, DESCRIPTION_2, ITEM_CLASS, ALPHA_SORT, STANDARD_PACK, GL_TABLE, PRIMARY_VND_NUM, VENDOR_ITEM_NUM, ACTIVE, ITEM_PRICE_CLS FROM ic_inventry_mast"; $rs=odbc_exec($conn,$sql); if (!$rs) {exit("Error in SQL");} while (odbc_fetch_row($rs)) { $ITEM_NUM=trim(odbc_result($rs,"ITEM_NUM")); $DESCRIPTION_1=trim(odbc_result($rs,"DESCRIPTION_1")); $DESCRIPTION_2=trim(odbc_result($rs,"DESCRIPTION_2")); $ITEM_CLASS=trim(odbc_result($rs,"ITEM_CLASS")); $ALPHA_SORT=trim(odbc_result($rs,"ALPHA_SORT")); $STANDARD_PACK=trim(odbc_result($rs,"STANDARD_PACK")); $GL_TABLE=trim(odbc_result($rs,"GL_TABLE")); $PRIMARY_VND_NUM=trim(odbc_result($rs,"PRIMARY_VND_NUM")); $VENDOR_ITEM_NUM=trim(odbc_result($rs,"VENDOR_ITEM_NUM")); $ACTIVE=trim(odbc_result($rs,"ACTIVE")); $ITEM_PRICE_CLS=trim(odbc_result($rs,"ITEM_PRICE_CLS")); $ITEM_NUM=str_replace('@','',$ITEM_NUM); $DESCRIPTION_1=str_replace('@','',$DESCRIPTION_1); $DESCRIPTION_2=str_replace('@','',$DESCRIPTION_2); $ITEM_CLASS=str_replace('@','',$ITEM_CLASS); $ALPHA_SORT=str_replace('@','',$ALPHA_SORT); $STANDARD_PACK=str_replace('@','',$STANDARD_PACK); $GL_TABLE=str_replace('@','',$GL_TABLE); $PRIMARY_VND_NUM=str_replace('@','',$PRIMARY_VND_NUM); $VENDOR_ITEM_NUM=str_replace('@','',$VENDOR_ITEM_NUM); $ACTIVE=str_replace('@','',$ACTIVE); $ITEM_PRICE_CLS=str_replace('@','',$ITEM_PRICE_CLS); $row="$ITEM_NUM@$DESCRIPTION_1@$DESCRIPTION_2@$ITEM_CLASS@$ALPHA_SORT@$STANDARD_PACK@$GL_TABLE@$PRIMARY_VND_NUM@$VENDOR_ITEM_NUM@$ACTIVE@$ITEM_PRICE_CLS\r\n"; fwrite($fp, $row); } fclose($fp); ?>