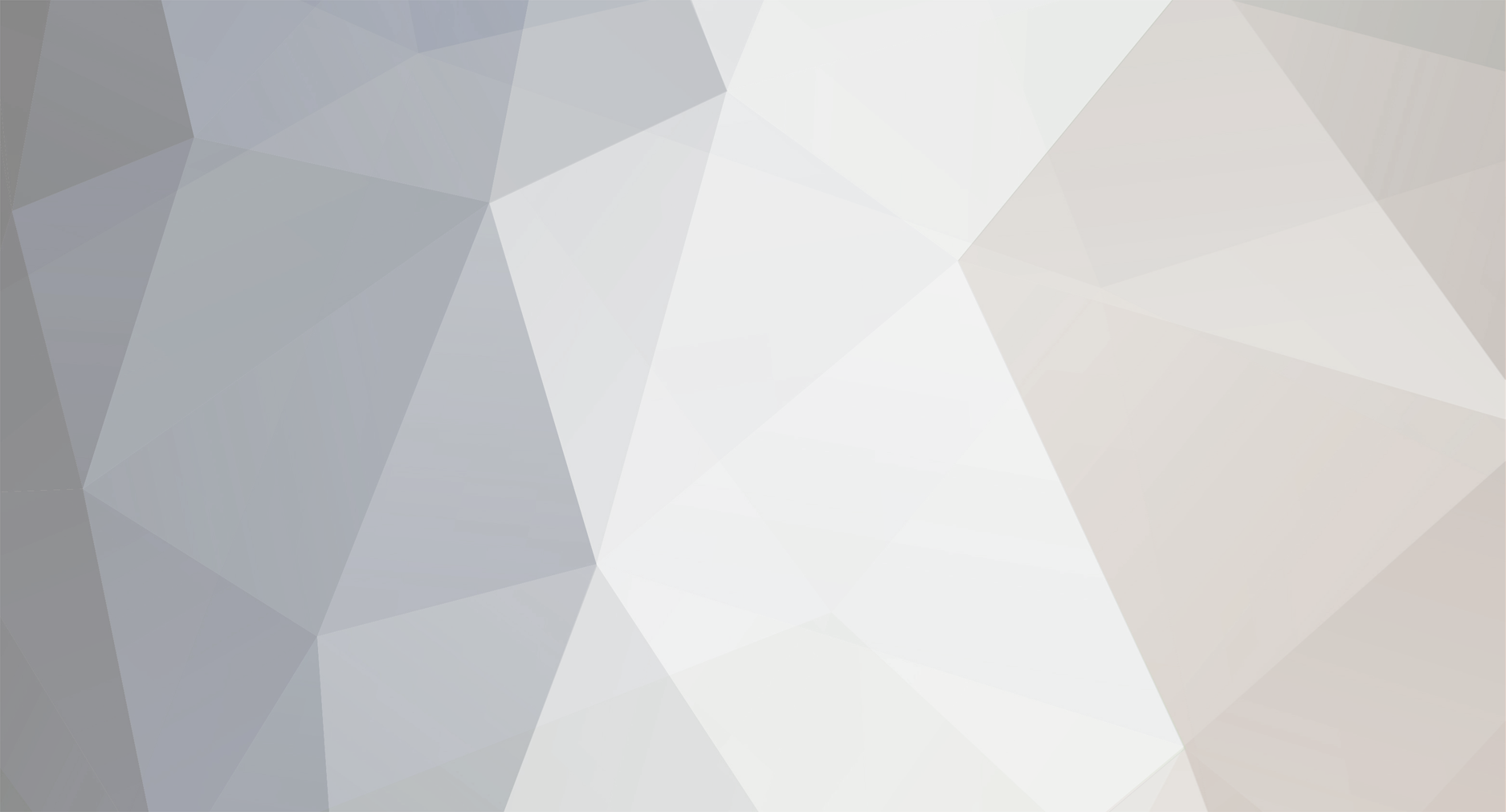
jakebur01
Members-
Posts
885 -
Joined
-
Last visited
Everything posted by jakebur01
-
How do I echo out these rows I am selecting from the zipcode column? function inradius($zip,$radius) { global $db; $query="SELECT * FROM zipData WHERE zipcode='$zip'"; $db->query($query); if($db->affected_rows()<>0) { $db->next_record(); $lat=$db->f("lat"); $lon=$db->f("lon"); $query="SELECT zipcode FROM zipData WHERE (POW((69.1*(lon-\"$lon\")*cos($lat/57.3)),\"2\")+POW((69.1*(lat-\"$lat\")),\"2\"))<($radius*$radius) "; $db->query($query); if($db->affected_rows()<>0) { while($db->next_record()) { $zipArray[$i]=$db->f("zipcode"); $i++; } } }else{ return "Zip Code not found"; } return $zipArray; } // end func } // end class
-
The PHP MD5() function does not encrypt a string in the same way as the standard *nix MD5SUM function. If you want to access an encrypted string in a MySQL database from different programs in *nix, and also from PHP, use the MySQL MD5() function, as in: UPDATE users SET pwd=md5('mypass') WHERE user='myuser'; This will generate the same encrypted string as in PHP md5('mypass').
-
This is one way, it checks for expressions. <?php $email="[email protected]"; if(!eregi("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9- ]+)*(\.[a-z]{2,3})$", $email) { echo "The e-mail was not valid"; } else { echo "The e-mail was valid"; } ?>
-
I am wanting to add a table to my MYsQL Database. With the colums Dealer, Address, City, State, Zip. I am wanting to use this code to find the dealers within a certain radius, depending on what zip code the user types in. [attachment deleted by admin]
-
Am I in the wrong place looking for help on this?
-
No, I need some direction. I don't know where to start. I was hoping someone could help walk me through some of it.
-
Could someone please help me with this?
-
How could I add onto this code to have it retrieve all of the zip codes within radius from another table?
-
Hello, I have this code that returns the distance between two zip codes and will return a radius of zips within so many miles. I am wanting to add onto this code to have it select from a new table that I have all of the rows of zip codes within that range. This new table would have name, address, zip, ect. <?php /* vim: set expandtab tabstop=4 shiftwidth=4: */ // +----------------------------------------------------------------------+ // | Filname: | // +----------------------------------------------------------------------+ // | Copyright (c) http://www.sanisoft.com | // +----------------------------------------------------------------------+ // | Description: | // +----------------------------------------------------------------------+ // | Authors: Original Author <[email protected]> | // | Your Name <[email protected]> | // +----------------------------------------------------------------------+ // // $Id$ include_once ("db_mysql.inc"); include_once ("phpZipLocator.php"); $db = new db_sql; $zipLoc = new zipLocator; $zipOne = 71075; $zipTwo = 23456; $distance = $zipLoc->distance($zipOne,$zipTwo); echo "The distance between $zipOne and $zipTwo is $distance Miles<br>"; $radius = 90; $zipArray = $zipLoc->inradius($zipOne,$radius); echo "There are ",count($zipArray)." Zip codes within $radius Miles of $zipOne"; <?php /* vim: set expandtab tabstop=4 shiftwidth=4: */ // +----------------------------------------------------------------------+ // | Filname: phpZipLocator.php | // +----------------------------------------------------------------------+ // | Copyright (c) http://www.sanisoft.com | // +----------------------------------------------------------------------+ // | License (c) This software is licensed under LGPL | // +----------------------------------------------------------------------+ // | Description: A simple class for finding distances between two zip | // | codes, The distance calculation is based on Zipdy package found | // | at http://www.cryptnet.net/fsp/zipdy/ written by V. Alex Brennen | // | <[email protected]> | // | You can also do radius calculations to find all the zipcodes within | // | the radius of x miles | // +----------------------------------------------------------------------+ // | Authors: Dr Tarique Sani <[email protected]> | // | Girish Nair <[email protected]> | // +----------------------------------------------------------------------+ // // $Id$ class zipLocator { /** * Short description. * This method returns the distance in Miles between two zip codes * Detail description * This method returns the distance in Miles between two zip codes, if either of the zip code is not found and error is retruned * @param zipOne - The first zip code * @param zipTwo - The second zip code * @global db - the database object * @since 1.0 * @access public * @return string * @update */ function distance($zipOne,$zipTwo) { global $db; $query = "SELECT * FROM zipData WHERE zipcode = $zipOne"; $db->query($query); if(!$db->nf()) { return "First Zip Code not found"; }else{ $db->next_record(); $lat1 = $db->f("lat"); $lon1 = $db->f("lon"); } $query = "SELECT * FROM zipData WHERE zipcode = $zipTwo"; $db->query($query); if(!$db->nf()) { return "Second Zip Code not found"; }else{ $db->next_record(); $lat2 = $db->f("lat"); $lon2 = $db->f("lon"); } /* Convert all the degrees to radians */ $lat1 = $this->deg_to_rad($lat1); $lon1 = $this->deg_to_rad($lon1); $lat2 = $this->deg_to_rad($lat2); $lon2 = $this->deg_to_rad($lon2); /* Find the deltas */ $delta_lat = $lat2 - $lat1; $delta_lon = $lon2 - $lon1; /* Find the Great Circle distance */ $temp = pow(sin($delta_lat/2.0),2) + cos($lat1) * cos($lat2) * pow(sin($delta_lon/2.0),2); $EARTH_RADIUS = 3956; $distance = $EARTH_RADIUS * 2 * atan2(sqrt($temp),sqrt(1-$temp)); return $distance; } // end func /** * Short description. * Converts degrees to radians * @param deg - degrees * @global none * @since 1.0 * @access private * @return void * @update */ function deg_to_rad($deg) { $radians = 0.0; $radians = $deg * M_PI/180.0; return($radians); } /** * Short description. * This method retruns an array of zipcodes found with the radius supplied * Detail description * This method returns an array of zipcodes found with the radius supplied in miles, if the zip code is invalid an error string is returned * @param zip - The zip code * @param radius - The radius in miles * @global db - instance of database object * @since 1.0 * @access public * @return array/string * @update date time */ function inradius($zip,$radius) { global $db; $query="SELECT * FROM zipData WHERE zipcode='$zip'"; $db->query($query); if($db->affected_rows()<>0) { $db->next_record(); $lat=$db->f("lat"); $lon=$db->f("lon"); $query="SELECT zipcode FROM zipData WHERE (POW((69.1*(lon-\"$lon\")*cos($lat/57.3)),\"2\")+POW((69.1*(lat-\"$lat\")),\"2\"))<($radius*$radius) "; $db->query($query); if($db->affected_rows()<>0) { while($db->next_record()) { $zipArray[$i]=$db->f("zipcode"); $i++; } } }else{ return "Zip Code not found"; } return $zipArray; } // end func } // end class ?>
-
I found this. It makes it to where ppl are unable to right click. <HEAD> <SCRIPT LANGUAGE="JavaScript1.1"> <!-- Original: Martin Webb ([email protected]) --> <!-- This script and many more are available free online at --> <!-- The JavaScript Source!! http://javascript.internet.com --> <!-- Begin function right(e) { if (navigator.appName == 'Netscape' && (e.which == 3 || e.which == 2)) return false; else if (navigator.appName == 'Microsoft Internet Explorer' && (event.button == 2 || event.button == 3)) { alert("Sorry, you do not have permission to right click."); return false; } return true; } document.onmousedown=right; document.onmouseup=right; if (document.layers) window.captureEvents(Event.MOUSEDOWN); if (document.layers) window.captureEvents(Event.MOUSEUP); window.onmousedown=right; window.onmouseup=right; // End --> </script> </HEAD>
-
Yea, I just need to make it harder for people to get the information though.
-
Does anyone know how to make it to where no one can right click and copy a bunch of important data off of you page?
-
thank you soo much man.
-
mysql_connect("localhost","myuser","mypass"); mysql_select_db("mydatabase"); $now = strtotime(date("Y/m/d H:i:s")); $expire = $now + (60 * 60 * 24 * 30); $values = "'$username','$first','$last','$pass',1,'$now','$expire'"; $cols = "username,first,last,password,billing,created,expire"; $id = db_insert ("users", $cols, $values); mysql_close(); echo "<br><br> Account #$id Created"; $_SESSION ["cur_user"] = $id; echo "<Br><Br>Saving contact Info Record Number: "; I tried mysql_insert, i get :Call to undefined function mysql_insert()
-
I got some code that had the function db_connect and I had to change it to mysql_connect for in to work. I am having trouble with db_insert, what would be another function that would replace this?
-
Could someone please give me a clue so I can figure out whats wrong?
-
if ($payment_type == 'subscription') { make_paypal(1); }
-
if ($step == 'signup4z') { if ($_POST) { $payment = $_POST['payment']; $payment_type = $_POST['payment_type']; echo "<h2>Payment Options</h2>"; if ($payment == 'paypal') { if ($payment_type == 'subscription') { make_paypal(1); } elseif ($payment_type == 'monthly') { make_paypal1(1); } } elseif ($payment == 'stormpay') { if ($payment_type == 'subscription') { make_stormpay(1); } elseif ($payment_type == 'monthly') { make_stormpay1(1); } } }
-
PHP Fatal error: Call to undefined function make_paypal() in C:\Inetpub\UltimateDogginSite.com\couponsite\signup.php on line 264
-
Hello, I have some code that I have to change '<?' to '<?php' for it to work. I have one part of the code that may be encoded base64_encode with the '<?' trapped inside. Is their a snippit of code I could insert to convert all of the code from '<?' to '<?php'? How do I fix this? Thanks, Jake
-
Hey, I am new to php and know a few basics. What would a be a really good book for me that would take me deeper into php?
-
Where would be a good place to find tutorials related to this? I want to learn!! Point me in the right direction.
-
You can see the page that I am trying to get going here: http://www.smithssc.com/dealerlocator.php
-
smithsla is a column in my zip code table that stands for latitude... and smithslo is the longitude column.................. I got in a habit of naming the columns thing that I can rememeber... I was having trouble getting my data to go in to the table in the beginning. I first had my longitude column named Long, I found the word "Long" on the list of words not to use. Since then I have been naming my stuff odd abbeviations and names. The zip code table which I have labeled "zdat1" holds 40,000 zip codes.