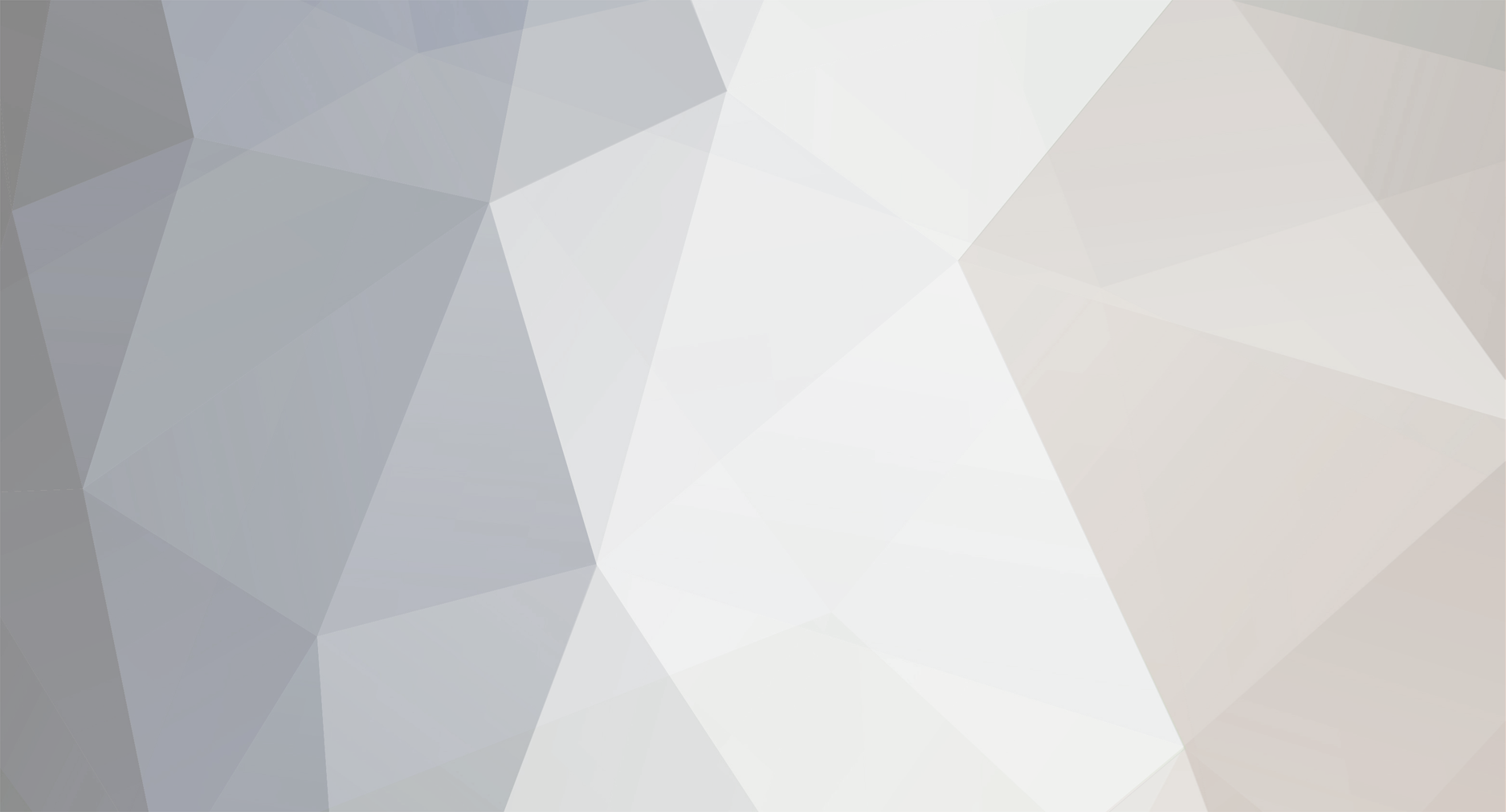
DJTim666
Members-
Posts
212 -
Joined
-
Last visited
Never
Everything posted by DJTim666
-
So lets say we have a large inventory of items(5000+) held in a database. Now lets say 2000 of these items do something different than the last. Which of the following ways is more efficient? Or maybe you have your own suggestion . Store individual code bits in the DB, then pull and run <?php $item_id = $_GET['item_id']; $findItem = $db->query("select `code` from `items` where `item_id` = '{$item_id}'"); if ($findItem) { $itemCode = $db->fetch($findItem); eval ($itemCode['code']); } ?> Store all item codes in an inventory script <?php $item_id = $_GET['item_id']; switch ($item_id){ case 123: //do this break; case 124: //do this break; //continuing on for my 2000 item list } ?> I know the first one looks more efficient, but I've heard its not good to use eval :S
-
Try this inside your <form>; <input type='hidden' name='id' value='someValue /'> Obviously change someValue to whatever value you want passed to your script.
-
Could you please your PHP and include the error.
-
Calling the date() function without parameters will give you a timestamp. This would be the same for the time() function aswell
-
We need some code to work with..?
-
Where you check the email, your $okay variable is spelt $okey. Change that and your script should work fine
-
<td><input type='button' onClick=\"window.location='edit_form_instructions.php?id={$row['instructions_id']}';\" value='Submit' /></td></tr></p>
-
Print your values and confirm you're getting the values you expect.
-
Try this; <?php if (isset($_GET['passkey'])) { // Passkey that got from link $passkey=mysql_real_escape_string($_GET['passkey']); // Retrieve data from table where row that match this passkey $query="SELECT * FROM `temp` WHERE0 `confirmcode` ='{$passkey}'"; $run = mysql_query($query); // If successfully queried if($run){ // Count how many row has this passkey $count=mysql_num_rows($run); // if found this passkey in our database, retrieve data from table if($count==1){ $rows=mysql_fetch_array($run); $username=$rows['username']; $password=$rows['password']; // Insert data that retrieves from temp in to users $sql2="INSERT INTO `users` (`username`, `password`)VALUES('$username', '$password')"; $result2=mysql_query($sql2); } // if not found passkey, display message "Wrong Confirmation code" else { echo "Wrong Confirmation code"; } // if successfully moved data from table temp to table users displays message "Your account has been activated" and delete confirmation code from table if($result2){ echo "Your account has been activated"; // Delete information of this user from table that has this passkey $sql3="DELETE FROM `temp` WHERE `confirmcode` = '$passkey'"; $result3=mysql_query($sql3); } else { die('Error: ' . mysql_error()); } } } ?>
-
[SOLVED] PHP won't write into MySQL database
DJTim666 replied to jonathanchacon's topic in PHP Coding Help
Lmfao. This is a login script. Not a register script. Try creating one of those to first create the users you want to login to your site. I'm sure you can find a few free register scripts on google. -
Anyone see a problem?
-
No, you'll have to change the way you're storing the time in your database if you want to run the query like that. mktime() should be able to help you convert. Edit: I always use a UNIX timestamp to insert dates into the database. It's much more flexible than having an actual date stamp.
-
Your $time variable should be time(). That'll fix your problem.
-
Am I stupid, or what? <?php $date = date("d", $status['created'])==date("d", time())?"Today at ".date("h:i:s A"):(date("d", $status['created'])+1)==date("d", time())?"Yesterday at ".date("h:i:s A", $status['created']):date("d/m/y h:i:s A", $status['created']); ?> It won't say anything but "Yesterday at [date here]". Even though the status its going over was created today. PLEASE HELP!!!
-
<?php // sending query $result = mysql_query("SELECT * FROM supportfeedback WHERE datetime >= $from AND datetime <= $to"); if (!$result) { die("<b>Error: Query failed</b>"); } if ($to = $from) { $label = "dated {$to}"; } elseif ($to <> $from) { $label = "between {$from} and {$to}"; } ?> In the code above. You haven't defined $to and $from. Now unless you have register_globals turned on, this won't work. Try defining those variables and your problem should be solved. Also you must use == when comparing two variables. Using = is for defining variables, not comparing.
-
<?php function convert_text($content) { $reg_ex = array( '/<ul>/s', '/\.jpg">/s', '/\.gif">/s', '/<br>/s' ); $replace_word = array( '<ul class="list">', '.jpg" class="imgright" alt="" />', '.gif" class="imgright" alt="" />', '<br />' ); $content = preg_replace($reg_ex, $replace_word, $content); return $content; } ?> That should work.
-
<?php $stringToSearch = "<a href=\"lalala.com\" title=\"lalala.com\">Go Here</a>"; preg_match("/\<td class\=\"file\"\>\n\<a href\=\"(.*?)\" title\=\"(.*?)\"\>(.*?)\<\/a\>/i", $stingToSearch, $matches); print_r($matches); ?>
-
Try <?php $stringToSearch = "<a href=\"lalala.com\" title=\"lalala.com\">Go Here</a>"; preg_match("/\<a href\=\"(.*?)\" title\=\"(.*?)\"\>(.*?)\<\/a\>/i", $stingToSearch, $matches); print_r($matches); ?> Simple code, really. $matches[0] will be the first pattern, $matches[1] will be the second and so on.
-
Unfortunately that didn't work. EDIT: I done some research and found encodeURIComponent(); which works perfectly. Thanks for the help
-
I've just recently coded some AJAX forums. They are in excellent working order except for the fact that I cannot post question marks. This is the AJAX code I am using to POST to the PHP script; function create(pars, det){ if (det == "topic") pars += "&subject="+$('subject').value+"&message="+$('message').value; else if (det == "post") pars += "&message="+$('message').value; new Ajax.Request("forum_scripts/posting.php", {method: 'post', parameters: pars, onUninitialized: inRequest(), onSuccess: showResponse} ); } And here is the form I am sending to the AJAX; <form action='' onSubmit='return false;'> <table width='65%' id='replyBox'> <tr> <th vAlign='top'>Message:</th> <td><textarea id='message' cols='70' rows='10'></textarea></td> </tr> <tr> <td colspan='2' align='center'><input type='submit' value='Reply to Topic' onClick=\"create('mode=post&t_id={$t_id}&f_id={$topic['f_id']}&start={$start}', 'post');\" /></td> </tr> </table> </form> Help is appreciated.
-
[SOLVED] How to change a certain string to symbols, help!
DJTim666 replied to Madatan's topic in Regex Help
http://www.php.net/str_replace -
Not true.. I'm not 100% sure of the difference between fetch_array and fetch_assoc. However I do know that I use fetch_array all the time and I call with the field names; not numbers. Wouldn't wanna get the newbs confused as soon as they come here, now would we. P.S. kpetsche20, you're just missing the $ in front of your data array as stated in the first reply. -- DJ