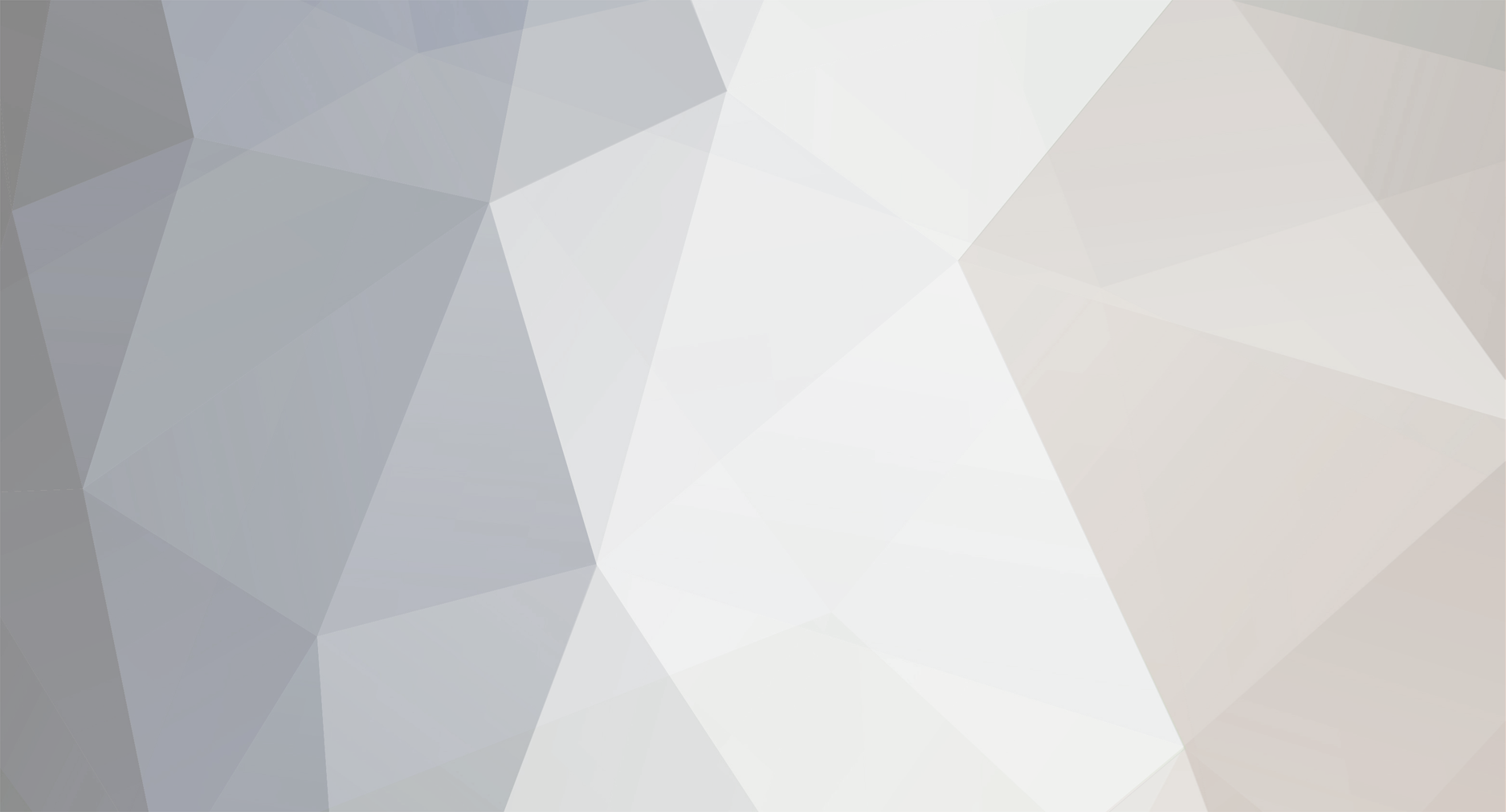
webguync
-
Posts
951 -
Joined
-
Last visited
Posts posted by webguync
-
-
I need to come up with a better way to do this. Currently I have a script which is a form and the user enters their email address, and their password in the database is sent to the email address. Problem is the password is MD5 hashed, so it's hashed when sent to their email. I am sure there is a better way to do this. Also, I am capturing a Security Question and Answer in the initial profile form that the user fills out, so I need to incorporate this as an extra layer of security. Please let me know of the methods for doing this.
Thanks in advance!
-
thanks, this is a bit confusing b/c I am storing info in a temp table, moving it and then deleting the temp table info. I have adjusted my code and now have this. Want to make sure I am doing it right.
<? session_start(); include('config.php'); // Passkey t from link $passkey=$_GET['passkey']; $tbl_name1="Profile_temp"; // Retrieve data from table where row matches passkey $sql1="SELECT * FROM $tbl_name1 WHERE confirm_code ='$passkey'"; $result1=mysql_query($sql1); // If successfully queried if($result1){ // Count how many row has this passkey $count=mysql_num_rows($result1); // if passkey is found retrieve info from temporary DB if($count==1){ $rows=mysql_fetch_array($result1); $FirstName=$rows['FirstName']; $LastName=$rows['LastName']; $UserName=$rows['UserName']; $Password= md5($rows['Password']); $Password2=md5($rows['Password2']); $email=$rows['email']; $Zip=$rows['Zip']; $Birthday=$rows['Birthday']; $Security=$rows['Security']; $Security2=$rows['Security2']; $tbl_name2="Profile"; // Insert data that retrieves from "temp_members_db" into table "registered_members" $sql2="INSERT INTO $tbl_name2(`FirstName`,`LastName`,`Username`,`Password`,`Password2`,`email`,`Zip`,`Birthday`,`Security`,`Security2`) VALUES ('$FirstName','$LastName','$UserName','$Password','$Password2','$email','$Zip','$Birthday','$Security','$Security2')"; //echo $sql2; $result2=mysql_query($sql2) or die(mysql_error()); } // if passkey is not found, display message "Wrong Confirmation code" else { echo "<h2>Sorry, Your passkey was not found.</h2>"; } $sql3="SELECT FROM $tbl_name2(`id`,`FirstName`,`LastName`,`Username`,`Password`,`Password2`,`email`,`Zip`,`Birthday`,`Security`,`Security2`) VALUES ('$FirstName','$LastName','$UserName','$Password','$Password2','$email','$Zip','$Birthday','$Security','$Security2')"; $result3=mysql_query($sql3) or die(mysql_error()); while ($row = mysql_fetch_assoc($result3)) { $_SESSION['id'] = $row['id']; $_SESSION['Username']=$user_name; } // if successfully moved data from table"temp_members_db" to table "registered_members" displays message "Your account has been activated" and don't forget to delete confirmation code from table "temp_members_db" if($result2){ echo "<h2>Your account has been activated</h2>"; echo"<p>You may now upload a profile picture</p>"; // Delete information of this user from table "temp_members_db" that has this passkey $sql4="DELETE FROM $tbl_name1 WHERE confirm_code = '$passkey'"; $result4=mysql_query($sql4) or die(mysql_error()); } } ?>
how would I handle the multiple results? Can I combine them or do I have to do separate if statements?
if($result2,$result3){ echo "<h2>Your account has been activated, $user_name</h2>"; echo"<p>You may now upload a profile picture</p>"; }
-
I am having some problems with part of the code. What is going on is a user registers info with a form and is sent an email with a confirmation link. The info that was in a temp DB table is moved to a member table. All of this works fine, but I am trying to be able to store an ID and username associated with their passkey info and echo that variable out. This part I am having trouble with. Code is here.
<? session_start(); include('config.php'); // Passkey t from link $passkey=$_GET['passkey']; $tbl_name1="Profile_temp"; // Retrieve data from table where row matches passkey $sql1="SELECT * FROM $tbl_name1 WHERE confirm_code ='$passkey'"; $result1=mysql_query($sql1); // If successfully queried if($result1){ // Count how many row has this passkey $count=mysql_num_rows($result1); // if passkey is found retrieve info from temporary DB if($count==1){ $rows=mysql_fetch_array($result1); $FirstName=$rows['FirstName']; $LastName=$rows['LastName']; $UserName=$rows['UserName']; $Password= md5($rows['Password']); $Password2=md5($rows['Password2']); $email=$rows['email']; $Zip=$rows['Zip']; $Birthday=$rows['Birthday']; $Security=$rows['Security']; $Security2=$rows['Security2']; $tbl_name2="Profile"; // Insert data that retrieves from "temp_members_db" into table "registered_members" $sql2="INSERT INTO $tbl_name2(`FirstName`,`LastName`,`Username`,`Password`,`Password2`,`email`,`Zip`,`Birthday`,`Security`,`Security2`) VALUES ('$FirstName','$LastName','$UserName','$Password','$Password2','$email','$Zip','$Birthday','$Security','$Security2')"; //echo $sql2; $result2=mysql_query($sql2); } // if passkey is not found, display message "Wrong Confirmation code" else { echo "<h2>Sorry, Your passkey was not found.</h2>"; } while ($row = mysql_fetch_assoc($result2)) { $_SESSION['id'] = $row['id']; $_SESSION['UserName']=$user_name; } // if successfully moved data from table"temp_members_db" to table "registered_members" displays message "Your account has been activated" and don't forget to delete confirmation code from table "temp_members_db" if($result2){ echo "<h2>Your account has been activated, </h2>"; echo "$user_name"; echo"<p>You may now upload a profile picture</p>"; // Delete information of this user from table "temp_members_db" that has this passkey $sql3="DELETE FROM $tbl_name1 WHERE confirm_code = '$passkey'"; $result3=mysql_query($sql3); } } ?>
getting an error for this part
while ($row = mysql_fetch_assoc($result2)) { $_SESSION['id'] = $row['id']; $_SESSION['UserName']=$user_name; }
the error is "Warning: mysql_fetch_assoc(): supplied argument is not a valid MySQL result resource in confirmation.php on line 62"
-
ok thanks. Looks like Google is s bit help so I will check out those scripts and post if I encounter any problems.
-
thanks, can you give me an idea of how to do this. Never done it before (obviously).
-
I've never actually done a username password, retrieval script before so need a little help. In the profile form the user is submitting username/password/name/email etc. into a MySQL DB along with a security question and answer. Is it just a matter of creating a form which does a check against the database and sends out an email to the user with their password? The password is hashed with MD5, so how would I send out an un-hashed PW?
thanks!
-
any ideas on my header error? Does this code always need to be within the <head></head> tags?
-
thanks again. I am now getting this error.
Warning: Cannot modify header information - headers already sent by (output started at /new_site/inc/inc.header.php:11) in /new_site/register.php on line 229
not sure why I get this b/c this line is the only place I am using a header redirect. Any ideas?
Header("Location: register.php?error=1");
-
I think I am a little confused with where to place the code again. Here is the error I am now getting.
"Fatal error: Function name must be a string in new_site/register.php on line 185"
and here is my current code
<?PHP //session_start(); require_once "formvalidator.php"; $show_form=true; if (!isset($_POST['Submit'])) { $human_number1 = rand(1, 12); $human_number2 = rand(1, 38); $human_answer = $human_number1 + $human_number2; $_SESSION['check_answer'] = $human_answer; } if(isset($_POST['Submit'])) { if (!isset($_SESSION['check_answer'])) { echo "<p>Error: Answer session not set</p>"; } if($_POST['math'] != $_SESSION['check_answer']) { echo "<p>You did not pass the human check.</p>"; exit(); } $validator = new FormValidator(); $validator->addValidation("FirstName","req","Please fill in FirstName"); $validator->addValidation("LastName","req","Please fill in LastName"); $validator->addValidation("UserName","req","Please fill in UserName"); $validator->addValidation("Password","req","Please fill in a Password"); $validator->addValidation("Password2","req","Please re-enter your password"); $validator->addValidation("Password2","eqelmnt=Password","Your passwords do not match!"); $validator->addValidation("email","email","The input for Email should be a valid email value"); $validator->addValidation("email","req","Please fill in Email"); $validator->addValidation("Zip","req","Please fill in your Zip Code"); $validator->addValidation("Security","req","Please fill in your Security Question"); $validator->addValidation("Security2","req","Please fill in your Security Answer"); if($validator->ValidateForm()) { $con = mysql_connect("localhost","uname","pw") or die('Could not connect: ' . mysql_error()); mysql_select_db("DB_Name") or die(mysql_error()); $FirstName=mysql_real_escape_string($_POST['FirstName']); //This value has to be the same as in the HTML form file $LastName=mysql_real_escape_string($_POST['LastName']); //This value has to be the same as in the HTML form file $UserName=mysql_real_escape_string($_POST['UserName']); //This value has to be the same as in the HTML form file $Password= md5($_POST['Password']); //This value has to be the same as in the HTML form file $Password2= md5($_POST['Password2']); //This value has to be the same as in the HTML form file $email=mysql_real_escape_string($_POST['email']); //This value has to be the same as in the HTML form file $Zip=mysql_real_escape_string($_POST['Zip']); //This value has to be the same as in the HTML form file $Birthday=mysql_real_escape_string($_POST['Birthday']); //This value has to be the same as in the HTML form file $Security=mysql_real_escape_string($_POST['Security']); //This value has to be the same as in the HTML form file $Security2=mysql_real_escape_string($_POST['Security2']); //This value has to be the same as in the HTML form file $sql="select * from Profile where username = '$UserName'"; $result = mysql_query( $sql, $con ) or die( "ERR: SQL 1" ); if ($_REQUEST('error') == 1){ echo "Sorry that user name already exist!"; } if(!mysql_num_rows($result)){ $sql="INSERT INTO Profile (`FirstName`,`LastName`,`Username`,`Password`,`Password2`,`email`,`Zip`,`Birthday`,`Security`,`Security2`) VALUES ('$FirstName','$LastName','$UserName','$Password','$Password2','$email','$Zip','$Birthday','$Security','$Security2')"; //echo $sql; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } else{ mail('email@gmail.com','A profile has been submitted!',$FirstName.' has submitted their profile',$body); echo "<h3>Your profile information has been submitted successfully.</h3>"; } } else{ Header("Location: register.php?error=1"); } mysql_close($con); $show_form=false; } else { echo "<h3 class='ErrorTitle'>Validation Errors:</h3>"; $error_hash = $validator->GetErrors(); foreach($error_hash as $inpname => $inp_err) { echo "<p class='errors'>$inpname : $inp_err</p>\n"; } } } if(true == $show_form) { ?> <form name="test" id="ContactForm" method="POST" accept-charset="UTF-8" action="?>"> <fieldset> <div class='normal_field'><label for="LastName">First Name</label></div> <div class='element_label'> <input type='text' name='FirstName' size='20'> </div> <div class='normal_field'><label for="LastName">Last Name</label></div> <div class='element_label'> <input type='text' name='LastName' size='20'> </div> </fieldset> <fieldset> <div class='normal_field'><label for="UserName">User Name</label></div> <div class='element_label'> <input type='text' name='UserName' size='20'> </div> <div class='normal_field'><label for="Password">Password</label></div> <div class='element_label'> <input type='password' name='Password' size='20'> </div> <div class='normal_field'><label for="Password2">Re-Enter Password</label></div> <div class='element_label'> <input type='password' name='Password2' size='20'> </div> <div class='normal_field'><label for="Email">Email</label></div> <div class='element_label'> <input type='text' name='email' size='20'> </div> </fieldset> <fieldset> <div class='normal_field'><label for="Zip">Zip Code</label></div> <div class='element_label'> <input type='text' name='Zip' size='20'> </div> <div class='normal_field'><label for="Birthday">Birthday(mm/dd/yyyy format)</label></div> <div class='element_label'> <input type='text' name='Birthday' size='20'> </div> <div class='normal_field'><label for="Security">Security Question</label></div> <div class='element_label'> <input type='text' name='Security' size='20'> </div> <div class='normal_field'><label for="Security2">Security Answer</label></div> <div class='element_label'> <input type='text' name='Security2' size='20'> </div> <div class='normal_field'><label for="math">What is <?php echo $human_number1." + ".$human_number2. "?"; ?></label></div> <div class='element_label'> <input type='text' name='math' size='20'> </div> </fieldset> <div id="agree"> <label for="tos"> <input type="checkbox" id="tos" name="tos" value="yes" /> I have read and agree to the <a href="ajax/serviceterms.html" id="terms">Terms of Service</a>. </label> </div> <fieldset> <div id="service-terms" class="box rounded-all"></div> <div class="controls"> <input id="submit" type="submit" name="Submit" value="CREATE PROFILE"/> </div> </fieldset> </form> <?PHP }//true == $show_form ?>
specifically line 185 is this
if ($_REQUEST('error') == 1){ echo "Sorry that user name already exist!"; }
-
thanks. Would the
Header("Location: <url of form>?error=1")
go right after
echo "<h2>Sorry, that username already exist!</h2>";
or should
if ($_REQUEST('error') == 1){ echo "<h2>Sorry that username already exist!</h2>"; }
replace this.
else{ echo "<h2>Sorry, that username already exist!</h2>"; }
-
thanks, the username check appears to work now. One more thing though, if the username exist and they get the error. what is the best way to display the form again?
-
with the code I have now, I just get the generic MySQL error. "Error: Duplicate entry 'UserName' for key 'usr'". UserName being the duplicate username in the database. I don't get the customized echo. that I put in. Here is the current code.
<?PHP //session_start(); require_once "formvalidator.php"; $show_form=true; if (!isset($_POST['Submit'])) { $human_number1 = rand(1, 12); $human_number2 = rand(1, 38); $human_answer = $human_number1 + $human_number2; $_SESSION['check_answer'] = $human_answer; } if(isset($_POST['Submit'])) { if (!isset($_SESSION['check_answer'])) { echo "<p>Error: Answer session not set</p>"; } if($_POST['math'] != $_SESSION['check_answer']) { echo "<p>You did not pass the human check.</p>"; exit(); } $validator = new FormValidator(); $validator->addValidation("FirstName","req","Please fill in FirstName"); $validator->addValidation("LastName","req","Please fill in LastName"); $validator->addValidation("UserName","req","Please fill in UserName"); $validator->addValidation("Password","req","Please fill in a Password"); $validator->addValidation("Password2","req","Please re-enter your password"); $validator->addValidation("Password2","eqelmnt=Password","Your passwords do not match!"); $validator->addValidation("email","email","The input for Email should be a valid email value"); $validator->addValidation("email","req","Please fill in Email"); $validator->addValidation("Zip","req","Please fill in your Zip Code"); $validator->addValidation("Security","req","Please fill in your Security Question"); $validator->addValidation("Security2","req","Please fill in your Security Answer"); if($validator->ValidateForm()) { $con = mysql_connect("localhost","username","password") or die('Could not connect: ' . mysql_error()); mysql_select_db("DBName") or die(mysql_error()); $FirstName=mysql_real_escape_string($_POST['FirstName']); //This value has to be the same as in the HTML form file $LastName=mysql_real_escape_string($_POST['LastName']); //This value has to be the same as in the HTML form file $UserName=mysql_real_escape_string($_POST['UserName']); //This value has to be the same as in the HTML form file $Password= md5($_POST['Password']); //This value has to be the same as in the HTML form file $Password2= md5($_POST['Password2']); //This value has to be the same as in the HTML form file $email=mysql_real_escape_string($_POST['email']); //This value has to be the same as in the HTML form file $Zip=mysql_real_escape_string($_POST['Zip']); //This value has to be the same as in the HTML form file $Birthday=mysql_real_escape_string($_POST['Birthday']); //This value has to be the same as in the HTML form file $Security=mysql_real_escape_string($_POST['Security']); //This value has to be the same as in the HTML form file $Security2=mysql_real_escape_string($_POST['Security2']); //This value has to be the same as in the HTML form file $sql="select * from Profile where username = '$username'"; $result = mysql_query( $sql, $con ) or die( "ERR: SQL 1" ); if(mysql_num_rows($result) == 0) { $sql="INSERT INTO Profile (`FirstName`,`LastName`,`Username`,`Password`,`Password2`,`email`,`Zip`,`Birthday`,`Security`,`Security2`) VALUES ('$FirstName','$LastName','$UserName','$Password','$Password2','$email','$Zip','$Birthday','$Security','$Security2')"; //echo $sql; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } else{ mail('email@gmail.com','A profile has been submitted!',$FirstName.' has submitted their profile',$body); echo "<h3>Your profile information has been submitted successfully.</h3>"; } mysql_close($con); $show_form=false; } else { echo "<h3 class='ErrorTitle'>Validation Errors:</h3>"; echo "<h3>That username already exist!</h3>"; $error_hash = $validator->GetErrors(); foreach($error_hash as $inpname => $inp_err) { echo "<p class='errors'>$inpname : $inp_err</p>\n"; } } } } if(true == $show_form) { ?> <form name="test" id="ContactForm" method="POST" accept-charset="UTF-8" action="?>"> <fieldset> <div class='normal_field'><label for="LastName">First Name</label></div> <div class='element_label'> <input type='text' name='FirstName' size='20'> </div> <div class='normal_field'><label for="LastName">Last Name</label></div> <div class='element_label'> <input type='text' name='LastName' size='20'> </div> </fieldset> <fieldset> <div class='normal_field'><label for="UserName">User Name</label></div> <div class='element_label'> <input type='text' name='UserName' size='20'> </div> <div class='normal_field'><label for="Password">Password</label></div> <div class='element_label'> <input type='password' name='Password' size='20'> </div> <div class='normal_field'><label for="Password2">Re-Enter Password</label></div> <div class='element_label'> <input type='password' name='Password2' size='20'> </div> <div class='normal_field'><label for="Email">Email</label></div> <div class='element_label'> <input type='text' name='email' size='20'> </div> </fieldset> <fieldset> <div class='normal_field'><label for="Zip">Zip Code</label></div> <div class='element_label'> <input type='text' name='Zip' size='20'> </div> <div class='normal_field'><label for="Birthday">Birthday(mm/dd/yyyy format)</label></div> <div class='element_label'> <input type='text' name='Birthday' size='20'> </div> <div class='normal_field'><label for="Security">Security Question</label></div> <div class='element_label'> <input type='text' name='Security' size='20'> </div> <div class='normal_field'><label for="Security2">Security Answer</label></div> <div class='element_label'> <input type='text' name='Security2' size='20'> </div> <div class='normal_field'><label for="math">What is <?php echo $human_number1." + ".$human_number2. "?"; ?></label></div> <div class='element_label'> <input type='text' name='math' size='20'> </div> </fieldset> <div id="agree"> <label for="tos"> <input type="checkbox" id="tos" name="tos" value="yes" /> I have read and agree to the <a href="ajax/serviceterms.html" id="terms">Terms of Service</a>. </label> </div> <fieldset> <div id="service-terms" class="box rounded-all"></div> <div class="controls"> <input id="submit" type="submit" name="Submit" value="CREATE PROFILE"/> </div> </fieldset> </form> <?PHP }//true == $show_form ?>
-
Hi, I need to insert some code into my current form code which will check to see if a username exist and if so will display an echo message. If it does not exist will post the form (assuming everything else is filled in correctly). I have tried some code in a few places but it doesn't work correctly as I get the username message exist no matter what. I think I am inserting the code into the wrong area, so need assistance as to how to incorporate the username check code.
$sql="select * from Profile where username = '$username'; $result = mysql_query( $sql, $conn ) or die( "ERR: SQL 1" ); if(mysql_num_rows($result)!=0) { process form } else { echo "That username already exist!"; }
the current code of the form
<?PHP //session_start(); require_once "formvalidator.php"; $show_form=true; if (!isset($_POST['Submit'])) { $human_number1 = rand(1, 12); $human_number2 = rand(1, 38); $human_answer = $human_number1 + $human_number2; $_SESSION['check_answer'] = $human_answer; } if(isset($_POST['Submit'])) { if (!isset($_SESSION['check_answer'])) { echo "<p>Error: Answer session not set</p>"; } if($_POST['math'] != $_SESSION['check_answer']) { echo "<p>You did not pass the human check.</p>"; exit(); } $validator = new FormValidator(); $validator->addValidation("FirstName","req","Please fill in FirstName"); $validator->addValidation("LastName","req","Please fill in LastName"); $validator->addValidation("UserName","req","Please fill in UserName"); $validator->addValidation("Password","req","Please fill in a Password"); $validator->addValidation("Password2","req","Please re-enter your password"); $validator->addValidation("Password2","eqelmnt=Password","Your passwords do not match!"); $validator->addValidation("email","email","The input for Email should be a valid email value"); $validator->addValidation("email","req","Please fill in Email"); $validator->addValidation("Zip","req","Please fill in your Zip Code"); $validator->addValidation("Security","req","Please fill in your Security Question"); $validator->addValidation("Security2","req","Please fill in your Security Answer"); if($validator->ValidateForm()) { $con = mysql_connect("localhost","uname","pw") or die('Could not connect: ' . mysql_error()); mysql_select_db("beatthis_beatthis") or die(mysql_error()); $FirstName=mysql_real_escape_string($_POST['FirstName']); //This value has to be the same as in the HTML form file $LastName=mysql_real_escape_string($_POST['LastName']); //This value has to be the same as in the HTML form file $UserName=mysql_real_escape_string($_POST['UserName']); //This value has to be the same as in the HTML form file $Password= md5($_POST['Password']); //This value has to be the same as in the HTML form file $Password2= md5($_POST['Password2']); //This value has to be the same as in the HTML form file $email=mysql_real_escape_string($_POST['email']); //This value has to be the same as in the HTML form file $Zip=mysql_real_escape_string($_POST['Zip']); //This value has to be the same as in the HTML form file $Birthday=mysql_real_escape_string($_POST['Birthday']); //This value has to be the same as in the HTML form file $Security=mysql_real_escape_string($_POST['Security']); //This value has to be the same as in the HTML form file $Security2=mysql_real_escape_string($_POST['Security2']); //This value has to be the same as in the HTML form file $sql="INSERT INTO Profile (`FirstName`,`LastName`,`Username`,`Password`,`Password2`,`email`,`Zip`,`Birthday`,`Security`,`Security2`) VALUES ('$FirstName','$LastName','$UserName','$Password','$Password2','$email','$Zip','$Birthday','$Security','$Security2')"; //echo $sql; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } else{ mail('email@gmail.com','A profile has been submitted!',$FirstName.' has submitted their profile',$body); echo "<h3>Your profile information has been submitted successfully.</h3>"; } mysql_close($con); $show_form=false; } else { echo "<h3 class='ErrorTitle'>Validation Errors:</h3>"; $error_hash = $validator->GetErrors(); foreach($error_hash as $inpname => $inp_err) { echo "<p class='errors'>$inpname : $inp_err</p>\n"; } } } if(true == $show_form) { ?>
-
thanks. Seems to work now. One other thing I need help with in regards to this. Is there a way to display the form if there is an error with the human check? Right now the error displays, but no form.
-
thanks, still a problem though. The form submits even if the value is wrong in the math field.
-
so it should be ok, putting this code in the header in an include file which is on every page?
<!-- PHP to start a SESSION and redirect to Profile page once profile info is submitted --> <?php session_start(); ?> <?php if($_POST['Submit']) { header("Location:Profile.php"); } ?>
-
thanks, I get the error msg. though even if I type in the correct value. How can I debug why this is happening?
-
any ideas on this problem? Is there another way to redirect other than by header()?
-
Hi,
I want to add another form field to determine if a user is human and not spam bots. I looked into CAPTCHA, but I think those are annoying. I was thinking about just having the user enter (5+5) and if the answer is not ten, then displaying a incorrect value message. If correct submit form and info to the Database. Not sure how to do that with what I currently have coded though so need some help with that part. Here is the current code.
<?PHP require_once "formvalidator.php"; $show_form=true; if(isset($_POST['Submit'])) { $validator = new FormValidator(); $validator->addValidation("FirstName","req","Please fill in FirstName"); $validator->addValidation("LastName","req","Please fill in LastName"); $validator->addValidation("UserName","req","Please fill in UserName"); $validator->addValidation("Password","req","Please fill in a Password"); $validator->addValidation("Password2","req","Please re-enter your password"); $validator->addValidation("Password2","eqelmnt=Password","Your passwords do not match!"); $validator->addValidation("email","email","The input for Email should be a valid email value"); $validator->addValidation("email","req","Please fill in Email"); $validator->addValidation("Zip","req","Please fill in your Zip Code"); $validator->addValidation("Security","req","Please fill in your Security Question"); $validator->addValidation("Security2","req","Please fill in your Security Answer"); if($validator->ValidateForm()) { $con = mysql_connect("localhost","beatthis","Jim2Drew!") or die('Could not connect: ' . mysql_error()); mysql_select_db("beatthis_beatthis") or die(mysql_error()); $FirstName=mysql_real_escape_string($_POST['FirstName']); //This value has to be the same as in the HTML form file $LastName=mysql_real_escape_string($_POST['LastName']); //This value has to be the same as in the HTML form file $UserName=mysql_real_escape_string($_POST['UserName']); //This value has to be the same as in the HTML form file $Password= md5($_POST['Password']); //This value has to be the same as in the HTML form file $Password2= md5($_POST['Password2']); //This value has to be the same as in the HTML form file $email=mysql_real_escape_string($_POST['email']); //This value has to be the same as in the HTML form file $Zip=mysql_real_escape_string($_POST['Zip']); //This value has to be the same as in the HTML form file $Birthday=mysql_real_escape_string($_POST['Birthday']); //This value has to be the same as in the HTML form file $Security=mysql_real_escape_string($_POST['Security']); //This value has to be the same as in the HTML form file $Security2=mysql_real_escape_string($_POST['Security2']); //This value has to be the same as in the HTML form file $sql="INSERT INTO Profile (`FirstName`,`LastName`,`Username`,`Password`,`Password2`,`email`,`Zip`,`Birthday`,`Security`,`Security2`) VALUES ('$FirstName','$LastName','$UserName','$Password','$Password2','$email','$Zip','$Birthday','$Security','$Security2')"; //echo $sql; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } else{ mail('webguync@gmail.com','A profile has been submitted!',$FirstName.' has submitted their profile',$body); echo "<h3>Your profile information has been submitted successfully.</h3>"; } mysql_close($con); $show_form=false; } else { echo "<h3 class='ErrorTitle'>Validation Errors:</h3>"; $error_hash = $validator->GetErrors(); foreach($error_hash as $inpname => $inp_err) { echo "<p class='errors'>$inpname : $inp_err</p>\n"; } } } if(true == $show_form) { ?> <form name="test" id="ContactForm" method="POST" accept-charset="UTF-8" action="<?php echo $_SERVER['PHP_SELF'];?>"> <fieldset> <div class='normal_field'><label for="LastName">First Name</label></div> <div class='element_label'> <input type='text' name='FirstName' size='20'> </div> <div class='normal_field'><label for="LastName">Last Name</label></div> <div class='element_label'> <input type='text' name='LastName' size='20'> </div> </fieldset> <fieldset> <div class='normal_field'><label for="UserName">User Name</label></div> <div class='element_label'> <input type='text' name='UserName' size='20'> </div> <div class='normal_field'><label for="Password">Password</label></div> <div class='element_label'> <input type='password' name='Password' size='20'> </div> <div class='normal_field'><label for="Password2">Re-Enter Password</label></div> <div class='element_label'> <input type='password' name='Password2' size='20'> </div> <div class='normal_field'><label for="Email">Email</label></div> <div class='element_label'> <input type='text' name='email' size='20'> </div> </fieldset> <fieldset> <div class='normal_field'><label for="Zip">Zip Code</label></div> <div class='element_label'> <input type='text' name='Zip' size='20'> </div> <div class='normal_field'><label for="Birthday">Birthday(mm/dd/yyyy format)</label></div> <div class='element_label'> <input type='text' name='Birthday' size='20'> </div> <div class='normal_field'><label for="Security">Security Question</label></div> <div class='element_label'> <input type='text' name='Security' size='20'> </div> <div class='normal_field'><label for="Security2">Security Answer</label></div> <div class='element_label'> <input type='text' name='Security2' size='20'> </div> <div class='normal_field'><label for="math">What is 5 + 5?</label></div> <div class='element_label'> <input type='text' name='math' size='20'> </div> </fieldset> <div id="agree"> <label for="tos"> <input type="checkbox" id="tos" name="tos" value="yes" /> I have read and agree to the <a href="ajax/serviceterms.html" id="terms">Terms of Service</a>. </label> </div> <fieldset> <div id="service-terms" class="box rounded-all"></div> <div class="controls"> <input id="submit" type="submit" name="Submit" value="CREATE PROFILE"/> </div> </fieldset> </form> <?PHP }//true == $show_form ?>
-
what I want to do is check to see if the username exist and if it does echo "Username already please choose another one" and once the username is unique then submit the form.
-
I added this to the top of my page before any of the HTML code begins.
<?php session_start(); ?> <?php if($_POST['Submit']) { header("Location:Profile.php"); } ?>
but it doesn't seem to work and I am getting the 'headers already sent warning msg.
the header is part of an include and is on every page. Is this the problem?
-
I tried doing the header in the else statement if the form is submitted successfully, but I get this error.
Warning: Cannot modify header information - headers already sent by (output started at /inc/inc.header.php:7) in /new_site/register.php on line 86
so I guess I need to put something in the actual header of the document to redirect if the form was successfully submitted?
-
thanks hoping to be able to redirect not after a user signs in but after they fill out a form successfully, that is why I want to be able to redirect within an if statement.
if no validation errors submit form and redirect after successful submission, else don't submit and display validation errors.
Thanks in advance.
-
I need some help with a form being successfully submitted redirecting to a secure page. I already have the form code working and just need the part to redirect within an else statement. Also need to redirect a user back to the form page if they try and visit the secure page without being a member.
thanks in advance.
best way to handle a lost username/password
in PHP Coding Help
Posted
thanks, anyway you can post some code to get me started? I have done something similar for registration, but not for password recovery.