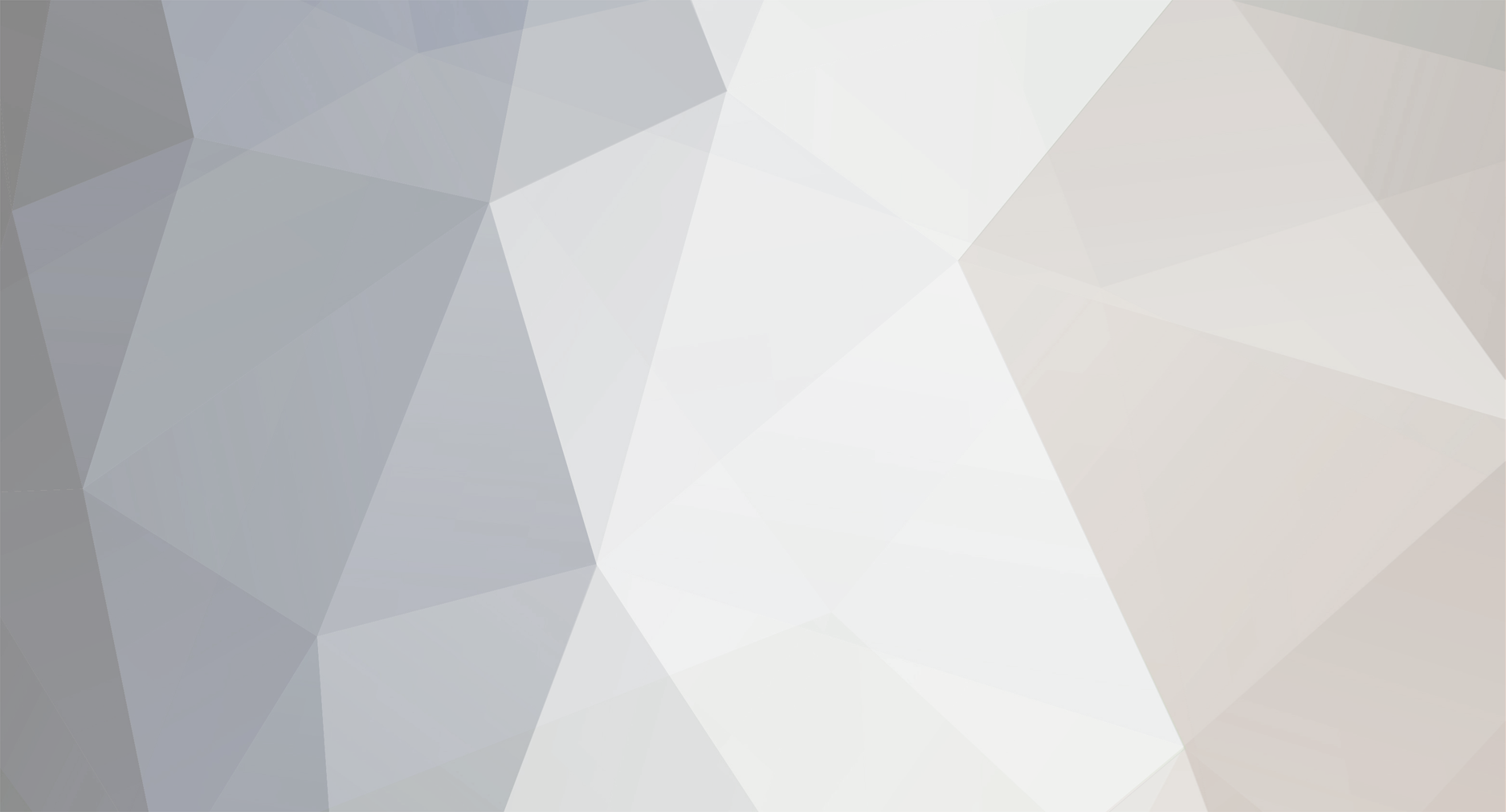
Pastulio
Members-
Posts
85 -
Joined
-
Last visited
Never
Everything posted by Pastulio
-
Yes I'm affraid you are going to have to use a table. But if you really want you can put your div's seperately in each <td> of the table
-
Yes I figured that, thanks though but I put it in there simply for testing purposes right now, there will be another class for the connection later on . Thanks a lot though btw, I will remove the mysql_close also So this would be the final version: <?php /* +---------------------------------------------------+ | | | PHP MySQL login class | | | +---------------------------------------------------+ | Filename : login.php | | Created : 25/06/2007 20:29 | | Created By : Pascal Van Acker (a.k.a Pastulio) | | Email : | | Version : 1.0 | | | +---------------------------------------------------+ */ class login { function verify_User ($username, $password) { $query = "SELECT * FROM `$this -> db_table`"; $result = mysql_query ($query); // Loop our results and search for a match while ($user_Info = mysql_fetch_array($result)) { if ($username == $user_Info['username'] && $password == md5 ($user_Info['password'])){ return true; } else { return false; } } } // END verify_User function user_Logged ($ip) { // Check if the user is already logged $query = "SELECT * FROM `$this -> db_table` WHERE `ip` = '$ip'"; $result = mysql_query ($query); if (mysql_num_rows ($result) > 0) { // User was already logged in return true; } else { // User has not logged in return false; } } // END user_Logged function check_Login ($username, $password, $ip, $remember) { // Check if the user is already logged in if ($this -> user_Logged($ip)){ return true; } else { // verify the user login if (!$this -> verify_User($username, $password)) { return false; } else { // If the user wishes to be remembered, insert him into the database if ($remember == '1') { $query = "UPDATE `$this -> db_table` SET `ip` = '$ip' WHERE `username` = '$username'"; } return true; } } } // END check_Login } /* THE CODE TO INCLUDE IN THE FILE WHERE YOU NEED TO CALL THE LOGIN $login = new login (); // Call the class if ($login->check_Login($username, $password, $ip, $remember)) { // User is logged in } else { // user is not logged in } */ ?>
-
I have created a class that allows you to create a database by calling the class. The problem is I get 2 errors: and I don't know why I'm having this problem because there is no password on my local machine for the MySQL database, and I've clearly set the username when I call the class. Here is the exact code: create_db.php <?php /* +---------------------------------------------------+ | | | PHP MySQL database creation class | | | +---------------------------------------------------+ | Filename : create_db.php | | Created : 25/06/2007 20:29 | | Created By : Pascal Van Acker (a.k.a Pastulio) | | Email : | | Version : 1.0 | | | +---------------------------------------------------+ */ class create_database { // MySQL config var $db_host; // MySQL host (usually 'localhost') var $db_username; // MySQL username var $db_password; // MySQL password var $database; // MySQL database name var $connection; // MySQL connection variable var $db_select; // MySQL selection variable var $db_table; // MySQL table selection function db_Connect () { // Connect to the MySQL server $this -> $connection = mysql_connect ($this -> db_host, $this -> db_username, $this -> db_password); // Test the MySQL connection if (!$this -> connection) { return false; } } // END db_Connect function db_Disconnect () { mysql_close ($this -> connection); } // END db_Disconnect function check_Existence () { $result = mysql_list_dbs ($this -> connection); while ($list = mysql_fetch_array($result)) { $database_list[] = $list['database']; } if (in_array($this -> database, $database_list)) { return true; } else { return false; } } function db_Create () { $query = "CREATE DATABASE `$this -> database`"; $result = mysql_query ($query); if (!$result) { return false; } else { return true; } } // END db_Create function create_database () { if (!$this -> db_Connect()){ return false; } if ($this -> check_Existence ()) { return false; } else { if ($this -> db_Create()){ return true; } else { return false; } } } } /* THE CODE TO INCLUDE IN THE FILE WHERE YOU NEED TO CALL THE DATABASE CREATION */ $create_db = new create_database (); // Call the class $create_db -> host = 'localhost'; // Set the MySQL host $create_db -> db_username = 'root'; // Set the MySQL username $create_db -> db_password = ''; // Set the MySQL password $create_db -> database = 'test_database_01'; // Set the MySQL to be created database name $create_db -> create_database(); $create_db -> db_Disconnect(); ?>
-
I don't really know but it looked quite noob to me, because what if the database could not be selected because of another reason (of which I know absolutely nothing) it would just give a big error. this is my final code: <?php function check_Existence () { $result = mysql_list_dbs ($this -> connection) while ($list = mysql_fetch_array($result)) { $database_list[] = $list['database']; } if (in_array($this -> database, $database_list)) { return true; } else { return false; } } ?>
-
Thanks a lot all *solved*
-
I feel quite stupid now because I don't know what you're talking about ???
-
so I should do something like this?: $db_list = mysql_list_dbs($connection); while ($row = mysql_fetch_array($db_list)) { $database_List[] = $row['database']; } And then use a for loop to check the array contents row by row, or could it be done with one loop?
-
hey everybody, I have a question that should be quite simple. I'm writing a class to create Databases, and I'll also make one to create Tables. But how do I check if a database already exists? just by checking if you can select it like so: // Select the MySQL database $this -> db_select = mysql_select_db ($this -> database); // Test the database selection if (!$this -> db_select) { return false; } (don't mind the "$this" it's coded in OOP but I didn't think I would need to put it in the OOP forum for this simple example) So is this the right way to check it or is there another way?
-
Ok so my deal is, I have just started coding in OOP and I've written a login class. Just wanted you all to have a look at it, and help me out on how to improve it, because I don't want there to be any unneeded code. Thanks in advance, Pastulio Here is my code login.php <?php /* +---------------------------------------------------+ | | | PHP MySQL login class | | | +---------------------------------------------------+ | Filename : login.php | | Created : 25/06/2007 20:29 | | Created By : Pascal Van Acker (a.k.a Pastulio) | | Email : | | Version : 1.0 | | | +---------------------------------------------------+ */ class login { // MySQL config var $host; // MySQL host (usually 'localhost') var $db_username; // MySQL username var $db_password; // MySQL password var $database; // MySQL database var $connection; // MySQL connection variable var $db_select; // MySQL selection variable var $db_table; // MySQL table selection function db_Connect () { // Connect to the MySQL server $this -> $connection = mysql_connect ($this -> host, $this -> db_username, $this -> db_password); // Test the MySQL connection if (!$this -> connection) { return false; } // Select the MySQL database $this -> db_select = mysql_select_db ($this -> database); // Test the database selection if (!$this -> db_select) { return false; } } // END db_Connect function db_Disconnect () { mysql_close ($this -> connection); } // END db_Disconnect function verify_User ($username, $password) { $query = "SELECT * FROM `$this -> db_table`"; $result = mysql_query ($query); // Loop our results and search for a match while ($user_Info = mysql_fetch_array($result)) { if ($username == $user_Info['username'] && $password == md5 ($user_Info['password'])){ return true; } else { return false; } } } // END verify_User function user_Logged ($ip) { // Check if the user is already logged $query = "SELECT * FROM `$this -> db_table` WHERE `ip` = '$ip'"; $result = mysql_query ($query); if (mysql_num_rows ($result) > 0) { // User was already logged in return true; } else { // User has not logged in return false; } } // END user_Logged function check_Login ($username, $password, $ip, $remember) { // If the database connection is not possible, a login cannot be checked if (!$this -> db_Connect) { return false; } // Check if the user is already logged in if ($this -> user_Logged($ip)){ return true; } else { // verify the user login if (!$this -> verify_User($username, $password)) { return false; } else { // If the user wishes to be remembered, insert him into the database if ($remember == '1') { $query = "UPDATE `$this -> db_table` SET `ip` = '$ip' WHERE `username` = '$username'"; } return true; } } $this -> db_Disconnect; } // END check_Login } /* THE CODE TO INCLUDE IN THE FILE WHERE YOU NEED TO CALL THE LOGIN $login = new login (); // Call the class $login -> host = ''; // Set the MySQL host $login -> db_username = ''; // Set the MySQL username $login -> db_password = ''; // Set the MySQL password $login -> database = ''; // Set the MySQL database $login -> db_table = ''; // Set the MySQL table if ($login->check_Login($username, $password, $ip, $remember)) { // User is logged in } else { // user is not logged in } $login -> db_Disconnect(); */ ?>
-
Hello everybody, I am completely new to Js, and I'm trying to do a very simple thing, but don't know how. So in my header I got this but I don't know if I can use the "+ id +" and + topic + variables at the end there (that's problem number 1) <script type="text/javascript"> <!-- confirmDelete (id, topic){ var answer = confirm ("Are you sure you want to delete " + topic + ".") if (answer) window.location="?page=delete&&id=" + id + } // --> </script> Second to call the script I have this <img src=\"del.gif\" onClick=\"confirmDelete($post[id], $post[topic]);\" border=0> The variables are PHP variables for those of you who don't know PHP. Am I wrong somewhere here guys? thanks a lot.
-
I'm not the pro in OOP but I think you just need to do the same thing again. class login{ var $username = ""; var $password = ""; function login($username, $password, $submit){ if(isset($this->submit)){ $this->username = $username; $this->password = $password; } } function querydb($username){ $login->login($this->username); //just doing username for the time being, ill add password when this gets working. echo $login; } }
-
number 1 $result = mysql_query("SELECT * FROM something"); while($row = mysql_fetch_array($result)) { echo $row['title']; ?> HTML source <?php } Number 2 $result = mysql_query("SELECT * FROM something"); while($row = mysql_fetch_array($result)) { echo $row['title']; echo "html source"; }
-
Yes but you store them in the "this->" varibles?
-
Thanks a lot mate . Forgot the name on it so just called it anti-Spam. Since posting a lot of bs on forums is usually called "spamming". sure is a lot to swallow haha, I'll get cracking.
-
use the function nl2br() http://be.php.net/nl2br or str_replace() http://be.php.net/manual/en/function.str-replace.php first one is the fastest.
-
I think it's also "$this->user" and etc...
-
*bump*
-
the function you want to use is either time() http://www.php.net/time or date() http://www.php.net/date
-
ok you beat me to it, bravo
-
Well first of all you'd need a to make a button with an onclick:"" that triggers the function that adds another box in javascript. I'm gonna look something up on google for your specific needs and I'll keep you posted. (I don't know javascript but I'm always willing to learn myself).
-
What exactly are you going for? so a user inputs something and clicks a "button" to get another input box?
-
Ok, so I was writing an anti-spam function that looked a little bit like this: // Anti-Spam function function antiSpam ($passedDay, $passedHour, $passedMinutes, $passedSeconds){ $currentHour = date ("H"); $currentMinutes = date ("i"); $currentSeconds = date ("s") $currentTimeSeconds = ($currentHour * 3600) + ($currentMinutes * 60) + $currentSeconds; $passedTimeSeconds = ($passedHour *3600) + ($passedMinuts * 60) + $passedSeconds; $intervalPP = 120; //interval in seconds $totalTime = $currentTimeSeconds - $passedTimeSeconds; if ($totalTime >= $intervalPP){ $_SESSION['antispam'] = "on"; $_SESSION['time_day'] = date ("d") $_SESSION['time_hour'] = date ("H"); $_SESSION['time_minutes'] = date ("i"); $_SESSION['time_seconds'] = date ("s"); return 1; } } And I though... Well what if somebody happens to post at 23:59 (11:59 PM) and again at 00:00 (12 AM). Then the function wouldn't live up to it's cause. Can anybody help me with this because if I do this for another day, the bug will just scoop over to month and year =/. Thanks a lot guys Edit: just saw I didn't even use the $intervalPP variable fixed in the code now
-
So the error occurs on the last line of my function. Here is the code for my function function checkDate ($day, $month, $year, $ddMonth){ // Februari if ($month == $ddMonth[1]){ if ($day > 29){ return false; } elseif ($day == 29){ // Check if the entered year is a leapyear if ($year % 100 != 0 && ($year % 400 == 0 || $year % 4 == 0)){ return true; } else { return false; } } else { return true; } } // April if ($month == $ddMonth[3]){ if ($day > 30){ return false; } else { return true; } } // June if ($month == $ddMonth[5]){ if ($day > 30){ return false; } else { return true; } } // September if ($month == $ddMonth[8]){ if ($day > 30){ return false; } else { return true; } } // November if ($month == $ddMonth[10]){ if ($day > 30){ return false; } else { return true; } } } // This is where I get the error Now this is where I call the function: $dateValidation = checkDate ($purchaseDay, $purchaseMonth, $purchaseYear, $ddMonth); $ddMonth is an array from a global, and i've also tried to do this: function checkDate ($day, $month, $year, $ddMonth = array()){ but I keep getting this error
-
*bump*