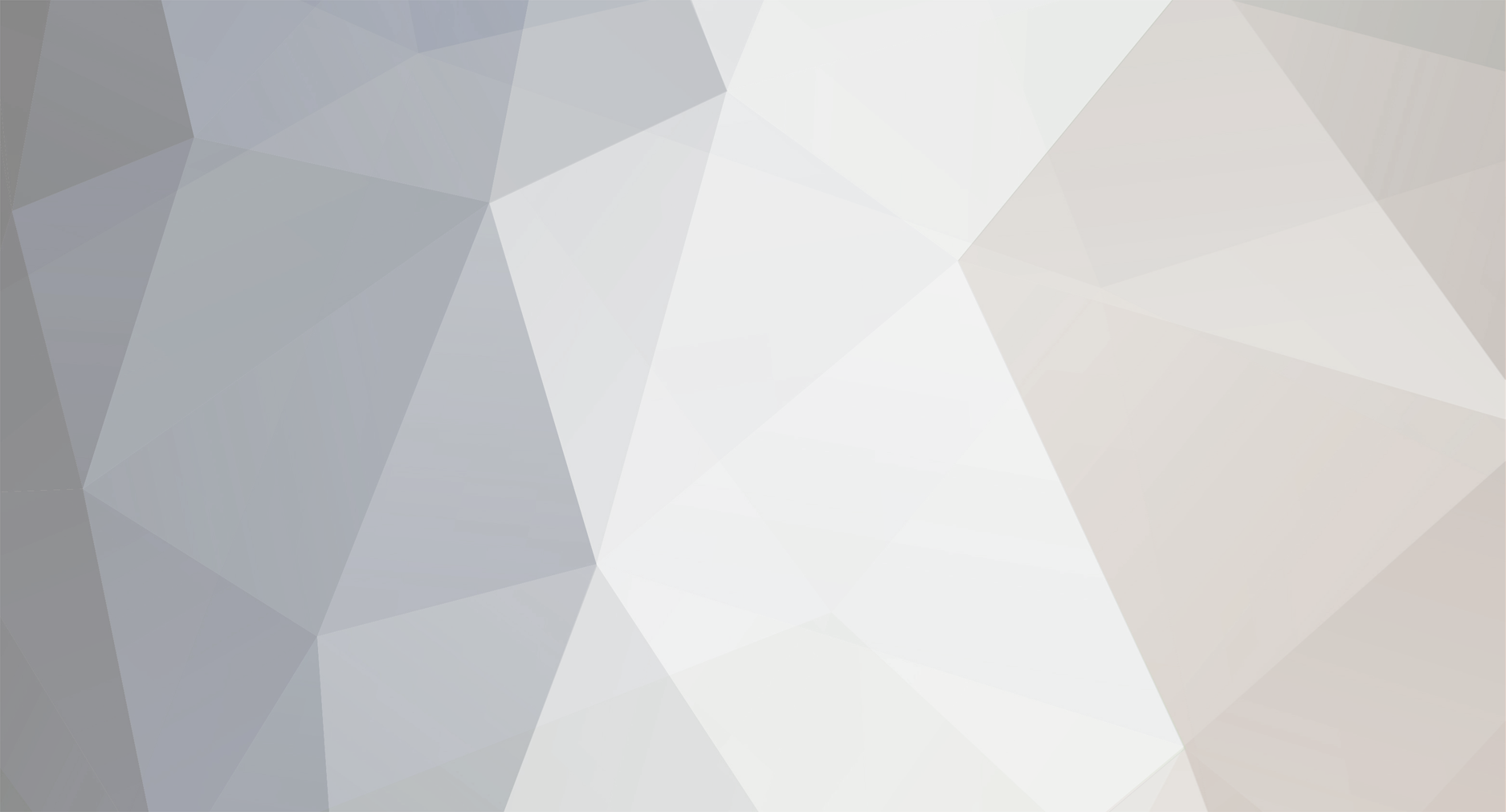
Cobby
Members-
Posts
59 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
Cobby's Achievements

Member (2/5)
0
Reputation
-
Whats with the open curly bracket straight after session_start()?
-
Its a term used to shorten search results, etc. Best google a popular term and check down the bottom of the page (Prev 1 2 3 4 Next). Imagine if every google search result was on the one page. As for the OP, I would suggest die()-ing your major variables so see what their actually values are, or maybe var_dump(). And then doing this inside your condition statements to see if they're actually executing.
-
Just making a very simple MVC-type system for an IPT assignment. A snippet from the bootstrap goes like: <?php ob_start(); @include root . '/header.php'; include root . $request; @include root . '/footer.php'; ob_flush(); ?> I've got output buffering setup so the $request file can still send headers. In header.php, I have things that need to be set in the header (i.e <title></title>, meta information, javascript, css, etc) and I want it so that $request can set stuff to the header.php. I don't really see how, but would it be any easier if I OOP-erised things? I would rather not because the schools servers are running PHP4 (pretty messed up I know) and I doesn't have very good class support. Cheers, Cobby
-
not sure how to structure includes for interdependent classes
Cobby replied to alexweber15's topic in PHP Coding Help
That would involve the Products class being static. Instead consider this: <?php require_once 'Products.php'; class ShoppingCart { protected $_products; public function __construct(){ $this->_products = new Products(); } public function getCategoryProducts($cid){ $products; // load Product IDs from given Category ID foreach($products as $pid){ $category[] = $this->_products->getProduct($pid); } return $category; } } ?> Depending on how you have set up the products class, you might consider simply extending, but the above would be my preffered method. -
Looking for constructive criticism of my simple MVC classes..
Cobby replied to cgm225's topic in PHP Coding Help
I like your code, its very clean, commented and strict. Have you considered using a static registry class? With regards to a template engine, no matter what you do, you can't really get anything better than smarty. But if you just want to create a very basic template engine that can really only do outputting variables, then making you own would be better to save in file size, efficiency, etc. If you are going to create a separate config file, it could be worth while creating it in an XML format and creating a class to handle it. This way you can update settings from within PHP where as you can't do that as easily with a .ini type file. -
"A single connection object can then be used throughout your code, as opposed to creating many additional connection objects which will eat up your resources. That would be the reason I guess." That means if, say you had two classes that both required a database connection you could do something like: <?php class foo { protected $_db; public function __construct(){ $this->_db = DatabaseConnection::getInstance(); } } class bar { protected $_db; public function __construct(){ $this->_db = DatabaseConnection::getInstance(); } } ?> Then instead of creating two connection to the database, it would be like: <?php $db = DatabaseConnection::getInstace()->connect($dbSettings); new foo(); new bar(); ?> Now both classes foo and bar have access to the database, but you only connected once.
-
It could also be a good idea to define method scope as well. Do you understand the difference between public, protected and private? And I assume that the str_ at the start is reference to the variable being a string? I don't think that sort of practice is necessary, PHP is a loosely type language so unofficially specifying the variable type isn't need. Same with the type casting when you set the class variables, its something that you can do, but isn't necessary. I haven't tried it, but if you pass, say an integer as the database username, PHP would convert it to a string anyway.
-
Render a string instead of template file with Smarty
Cobby replied to Cobby's topic in PHP Coding Help
I figured it out, Smarty supports resource plugins, so I created a text one. Now I can just go: <?php $smarty->assign('aVar', 'World'); $tpl = 'Hello {$aVar}!'; $smarty->display("text:$tpl"); // Hello World! ?> -
I think you might be creating a non-terminating loop because you call the function inside the function. <?php function foo($string){ foo($string); die('Will this execute?'); } ?>
-
There isn't really any limitation on how many class you can have defined. Are the images stored in the database? Generally, you would have a class to handle the DB connection and provide a few functions to save a bit of SQL. Then you would have a gallery class which you would pass the instance of the DB class to the constructor and assign it to a property. Then you would have functions in your gallery class that do that actually work, like getAlbums(), getPhoto($photo_id), etc.
-
Hi, I have templates stored in a database that I want to render. At the moment I just generate a temporary file and write the contents of the database query to it, the display that temporary file. <?php $result = $db->query($sql); file_put_contents('temp.tpl', $result['content']); $smarty->display('temp.tpl'); ?> But I don't like doing this as it just seems so unecessary, instead I would rather be like: <?php $result = $db->query($sql); $smarty->render($result['content']); ?> Anyway to do that? Cheers, Cobby
-
[SOLVED] Variables in a string inputted into Class Method
Cobby replied to WBSKI's topic in PHP Coding Help
So is $row a property inside the class? <?php class foo { public function echo($var){ echo $this->{$var}; } } $foo = new foo(); $foo->echo('testVar'); // will display $foo->testVar ?> Cobby -
You could use the __set and __get magic methods, or create a stdClass and assign it to one variable. <?php class foo { private $_data; public function __contruct(){ // TODO: class contructor function } public function __get($key){ return $this->_data[$key]; } public function __set($key, $value){ $this->_data[$key] = $value; } } ?> You can then access data from the class like this: <?php $class = new foo(); $class->test = 'Test Variable'; // performs $this->_data['test'] = 'Test Variable'; echo $class->test; // displays 'Test Variable' ?> stdClass is basically just an alias to an array: <?php $class = new stdClass(); $class->test = 'Test Variable'; // performs $class['test'] = 'Test Variable'; echo $class->test; // displays 'Test Variable' ?> Of course with stdClass you can't define functions, as its just a special type of array. .Cobby
-
Since your so focused on making your own, which is good fun , I highly recommend Zend Framework. Zend Framework is quite easy, but its just there is soo much to learn. I would allow two weeks to learn it thoroughly. With Zend you will find several video tutorials on creating bootstrap, etc (just google) and make sure you look deeply at: Zend_Controller Modular Directory Structure Zend_Loader Zend_Zb Zend_Config / Zend_Log Zend_Auth / Zend_Layout Zend_View / Zend_Layout Zend_Form Integrating Smarty For modular directory structure, see here: http://framework.zend.com/manual/en/zend.controller.modular.html#zend.controller.modular.directories That's the bulk of the essential modules when developing a CMS. More info at http://framework.zend.com/manual/en/ In any case, actual page content should be stored in a database. Create a regex route that routes all requests ending in .html to your content controller. Then your content controller would retrieve the specified page (from the request) from the database, then render it in the content section of your template/layout. Editing would be a similar process, except a different url to route (like /admin/page/:pid) and have a little editor (either TinyMCE or FCKEditor) and when the form is submitted just update the record in the DB. Again, learn Zend Framework, especially if you will be creating other applications down the track. Cobby.