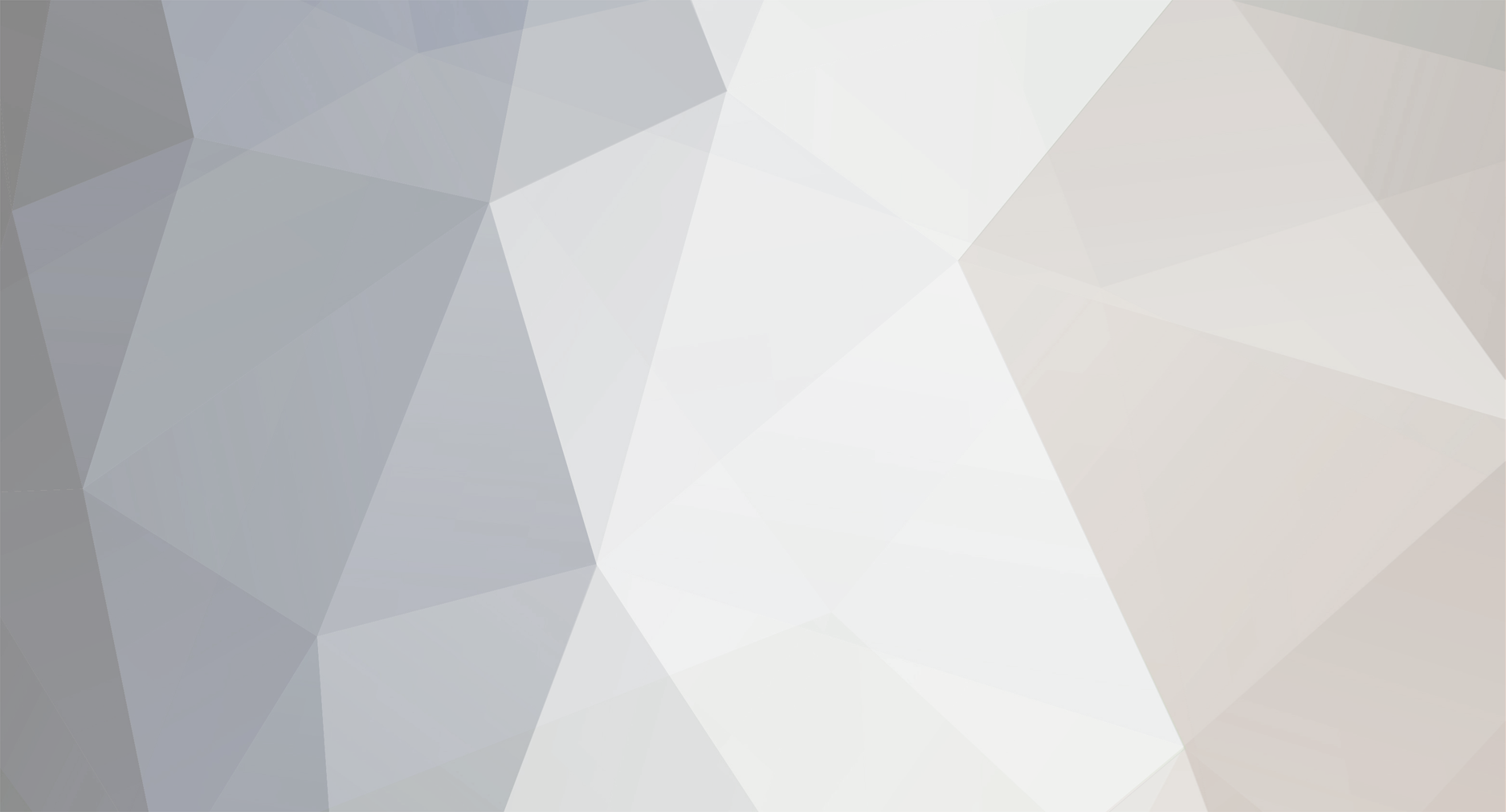
maxudaskin
Members-
Posts
628 -
Joined
-
Last visited
Everything posted by maxudaskin
-
Or, you could just put multiple names, separated by a comma or forward slash, or whatever you please then split them with explode. index.php?name=Max,John,Mr. Potato Head <?php $names = explode(',', $_GET['name']); This would leave you with: array([0]=>Max [1]=>John [2]=>Mr. Potato Head) Easy to access it by using a foreach loop, or even just a for loop using the count of the array to help you.
-
Sorry, teletype apparently formats into HTML, so I've put it within [nobbc] tags Array ( [0] => Array ( [0] => 4 [id] => 4 [1] => Test [label] => Test [2] => ?r=test [link] => ?r=test [3] => [relation] => [4] => 0 [parent] => 0 [5] => 5 [sort] => 5 [6] => 1 [active] => 1 ) [1] => Array ( [0] => 2 [id] => 2 [1] => About Us [label] => About Us [2] => ?r=about [link] => ?r=about [3] => [relation] => [4] => 0 [parent] => 0 [5] => 10 [sort] => 10 [6] => 0 [active] => 0 ) [2] => Array ( [0] => 3 [id] => 3 [1] => Fleet [label] => Fleet [2] => ?r=about/fleet [link] => ?r=about/fleet [3] => [relation] => [4] => 2 [parent] => 2 [5] => 0 [sort] => 0 [6] => 0 [active] => 0 ) )
-
At the bottom of the page, click "Topic Solved"
-
You can also turn it into a csv file.
-
Hi guys, I apologize for the number of posts regarding this particular class that I am writing (really, at this point, you've probably done more in it), but I have another issue. I've decided to re-write the pieces that were giving me trouble, and instead of using a for loop, I went to a foreach loop. Here's what it in the database for the particular query: Here's what I get when I dump the array with print_r(): Array ( [0] => Array ( [0] => 4 [id] => 4 [1] => Test [label] => Test [2] => ?r=test [link] => ?r=test [3] => [relation] => [4] => 0 [parent] => 0 [5] => 5 [sort] => 5 [6] => 1 [active] => 1 ) [1] => Array ( [0] => 2 [id] => 2 [1] => About Us [label] => About Us [2] => ?r=about [link] => ?r=about [3] => [relation] => [4] => 0 [parent] => 0 [5] => 10 [sort] => 10 [6] => 0 [active] => 0 ) [2] => Array ( [0] => 3 [id] => 3 [1] => Fleet [label] => Fleet [2] => ?r=about/fleet [link] => ?r=about/fleet [3] => [relation] => [4] => 2 [parent] => 2 [5] => 0 [sort] => 0 [6] => 0 [active] => 0 ) ) And the SQL statement: SELECT * FROM `menu` ORDER BY `parent` ASC, `sort` ASC With the corresponding PHP code (The issue starts in formatMenuItems()): <?php /** * Menu Class * * This file is used to create a dynamic menu based on the user's permissions and status * @author Max Udaskin <[email protected]> * @version 1.0 * @package navigation */ /** * @ignore */ if(!defined('ALLOW_ACCESS')) { // Do not allow the file to be accessed by itself die('Restricted File.'); } require_once("Database.php"); class Menu { protected $login; protected $all_rows; protected $menu_items; protected $html = NULL; /** * The constructor * @param Login $login */ function _construct() { } /** * Get All Items * Retrieves all database menu items */ private function getAllItems() { $sql = 'SELECT * FROM `menu` ORDER BY `parent` ASC, `sort` ASC'; $database = new Database('default'); $database->connect(); $database->fetchArray($sql); $query = $database->getLastQuery(); $i = 0; while($row = mysql_fetch_array($query)) { $this->all_rows[$i] = $row; $i++; } print_r($this->all_rows); } /* * Selects the menu items that are appropriate for the user */ private function chooseMenuItems() { $this->menu_items = $this->all_rows; } /** * Formats the menu items for the HTML output */ private function formatMenuItems() { $menu = array(); $menu_imploded = ''; foreach($this->menu_items as $item) { if($item['parent'] == 0) { $menu[] = $this->parseItem($item); } } $menu_imploded = $this->combineMultiArray('', $menu); echo '<div id="menu">'; echo $menu_imploded; echo '</div>'; } /** * Searches an array and it's sub arrays for a defined value ($for) * @param array $array * @param mixed $for */ private function search(array $array, $for) { foreach ($array as $key => $value) { if ($value === $for) { return ($key); } else if (is_array($value)) { $found = $this->search($value, $for); if (is_array($found)) { array_unshift($found, $key); return $found; } } } return false; } /** * Gets the array item and returns it * @param array $arr * @param string $string */ function variableArray($arr, $string) { preg_match_all('/\[([^\]]*)\]/', $string, $arr_matches, PREG_PATTERN_ORDER); $return = $arr; foreach($arr_matches[1] as $dimension) { $return = $return[$dimension]; } return $return; } /** * Parses HTML for the item, as an array * @param array $item */ private function parseItem($item) { if($item['parent'] == 0) { $h2_s = '<h2>'; $h2_e = '</h2>'; } else { $h2_s = ''; $h2_e = ''; } $return[0] = '<ul><li>' . $h2_s . '<a href="'; $return[0] .= $item['link'] . '">'; $return[0] .= $item['label'] . '</a>' . $h2_e; $return[1] = false; // Children $return[2] = '</li></ul>'; $return['parent'] = $item['parent']; return $return; } /** * Puts all of the HTML in the array together */ private function produceHtml() { $this->getAllItems(); $this->chooseMenuItems(); $this->formatMenuItems(); } /** * Get HTML * @return string The HTML menu */ public function getHtml() { $this->produceHtml(); return $this->html; } /** * Combine Multi Array * Takes any array of any dimension and combines it into a single string * @param unknown_type $array */ private function combineMultiArray($glue, $array) { $return = ''; foreach($array as $piece) { if(is_array($piece)) { $return .= $glue . $this->combineMultiArray($glue, $piece); } $return .= $glue . $piece; } return $return; } }
-
Pretty much, I need to get the parent item, stick the array of information from the item into the parent's array (key [1]) and in the end, go through a loop to transform it into a multi-drop down menu. Here's the code I have right now, still rough due to it still being in the production stages. I have an example of the final html output at the bottom. <?php /** * Menu Class * * This file is used to create a dynamic menu based on the user's permissions and status * @author Max Udaskin <[email protected]> * @version 1.0 * @package navigation */ /** * @ignore */ if(!defined('ALLOW_ACCESS')) { // Do not allow the file to be accessed by itself die('Restricted File.'); } require_once("Database.php"); class Menu { protected $login; protected $all_rows; protected $menu_items; protected $html = NULL; protected $menu = array(); /** * The constructor * @param Login $login */ function _construct() { } /** * Get All Items * Retrieves all database menu items */ private function getAllItems() { $sql = 'SELECT * FROM `menu` ORDER BY `parent` ASC, `sort` ASC'; $database = new Database('default'); $database->connect(); $database->fetchArray($sql); $query = $database->getLastQuery(); $i = 0; while($row = mysql_fetch_array($query)) { $this->all_rows[$i] = $row; $i++; } } /* * Selects the menu items that are appropriate for the user */ private function chooseMenuItems() { $this->menu_items = $this->all_rows; } /** * Formats the menu items for the HTML output */ private function formatMenuItems() { $menu = array(); $menu_imploded = ''; for($i = 0; $i < count($this->menu_items); $i++) { $cur_item = $this->parseItem($this->menu_items[$i]); if($cur_item['parent'] == 0) { unset($cur_item['parent']); $this->menu[count($this->menu)] = $cur_item; } else { $parent = $this->search($this->menu_items, $cur_item['parent']); print_r($parent); } } for($i = 0; $i < count($menu); $i++) { $menu_imploded .= implode('', $menu[$i]); } //print_r($this->menu); echo '<div id="menu">'; echo $menu_imploded; echo '</div>'; } /** * Searches an array and it's sub arrays for a defined value ($for) * @param array $array * @param mixed $for */ private function search(array $array, $for) { foreach ($array as $key => $value) { if ($value === $for) { return array($key); } else if (is_array($value)) { $found = $this->search($value, $for); if (is_array($found)) { array_unshift($found, $key); return $found; } } } return false; } /** * Parses HTML for the item, as an array * @param array $item */ private function parseItem($item) { if($item['parent'] == 0) { $h2_s = '<h2>'; $h2_e = '</h2>'; } else { $h2_s = ''; $h2_e = ''; } $return[0] = '<ul><li>' . $h2_s . '<a href="'; $return[0] .= $item['link'] . '">'; $return[0] .= $item['label'] . '</a>' . $h2_e; $return[1] = array(); // Children $return[2] = '</li></ul>'; $return['parent'] = $item['parent']; return $return; } /** * Puts all of the HTML in the array together */ private function produceHtml() { $this->getAllItems(); $this->chooseMenuItems(); $this->formatMenuItems(); } /** * Get HTML * @return string The HTML menu */ public function getHtml() { $this->produceHtml(); return $this->html; } } /* * <div id="menu"> <ul> <li><h2>CSS Drop Down Menus</h2> <ul> <li><a href="http://www.seoconsultants.com/tips/css/#cssmenus" title="SEO Consultants Directory">CSS Hover Navigation</a> <ul> <li><a href="../css-dropdown-menus.html" title="tanfa Introduction">Intro for CSS Enthusiasts</a></li> <li><a href="index.html" title="Plain HTML Code">Demonstration HTML</a></li> <li><a href="http://www.xs4all.nl/~peterned/csshover.html" title="whatever:hover file">csshover.htc file</a></li> </ul> </li> </ul> </li> </ul> <ul> <li><h2>Vertical CSS Pop-Out Menu</h2> <ul> <li><a href="http://www.seoconsultants.com/css/menus/vertical/" title="SEO Consultants Vertical Example">SEO Consultants Sample</a> </li> <li><a href="vs7.html" title="Complete Example">tanfa Demo example</a> <ul> <li><a href="index.html#">tanfa Tutorial</a> <!-- link to seo vertical tut --> <ul> <li><a href="vs1.html" title="Vertical Menu - Page 1">Stage 1</a> </li> <li><a href="vs2.html" title="Vertical Menu - Page 2">Stage 2</a> </li> <li><a href="vs3.html" title="Vertical Menu - Page 3">Stage 3</a> </li> <li><a href="vs4.html" title="Vertical Menu - Page 4">Stage 4</a> </li> <li><a href="vs5.html" title="Vertical Menu - Page 5">Stage 5</a> </li> <li><a href="vs6.html" title="Vertical Menu - Page 6">Stage 6</a> </li> </ul> </li> </ul> </li> </ul> </li> </ul> <ul> <li><h2>Horizontal Drop & Pop Menu</h2> <ul> <li><a href="http://www.seoconsultants.com/css/menus/horizontal/" title="SEO Consultants Directory Example">SEO Consultants Sample</a> </li> <li><a href="hs7.html">tanfa Demo example</a> <!-- fully working sample --> <ul> <li><a href="index.html#">tanfa Tutorial</a> <!-- link to horizontal tut --> <ul> <li><a href="hs1.html" title="Horizontal Menu - Page 1">Stage 1</a></li> <li><a href="hs2.html" title="Horizontal Menu - Page 2">Stage 2</a></li> <li><a href="hs3.html" title="Horizontal Menu - Page 3">Stage 3</a></li> <li><a href="hs4.html" title="Horizontal Menu - Page 4">Stage 4</a></li> <li><a href="hs5.html" title="Horizontal Menu - Page 5">Stage 5</a></li> <li><a href="hs6.html" title="Horizontal Menu - Page 6">Stage 6</a></li> </ul> </li> </ul> </li> </ul> </li> </ul> </div> */
-
That is what I thought it did, but it leaves me in the same spot as before, just, now it's KISS. How can I call the index using those keys?
-
Nope, I can't figure it out. Sorry to ask, but can you explain this function for me please?
-
So it works.. I just need to figure out why, and I'll be set. Thanks man
-
I would highly suggest reading this tutorial. You may learn something, even though I had to take a class to completely understand the point of a class. http://www.phpfreaks.com/tutorial/oo-php-part-1-oop-in-full-effect
-
I'm still a little bit confused over how I would call the index. I can get a variable to have it's value, "[2][3][1]", but I don't understand how I can effectively use it. I doubt that $items . $key would work?
-
First off, it is so extremely unlikely that two people would put a bid in at the same time, but if you really want to prevent it from happening, check the database for any new bids by other users immediately before posting the current user's bid. If there are new bids, put a bid place holder in with the next bid price up, and ask if they want to increase the bid to what is in the placeholder. All the while, new bids may be placed, so it should be a reoccurring function. Beginning of Function [user Submits Bid] (Check for new bids since page load) - If new bid: (Place Bid Placeholder) [Get user input] - If Accept (Recall function) - Else (Return to previous page or what ever you want it to do) - Else (Place Bid)
-
The title makes it sound more complicated than it is. I have a recurring function that searches for a specific value in a multi-dimensional array. When it finds it, it returns an array of the index. For example, if it found the value at $array[1][4][2][0], it would return an array like this: [0]=>1 [1]=>4 [2]=>2 [3]=>0 Here's the function. <?php // Other Code /** * Find's the parent of an item * Unfortunately, it takes a bit more work than just using the loudspeaker at a grocery store * @param int $parent The parent to be searched for * @param array $array * @param array $cur_index */ private function findParent($parent, $array, $cur_index) { for($i = 0; $i < count($array); $i++) { // Search the array if($array[$i]['id'] == $parent) { // If the parent is found $cur_index[count($cur_index)] = $i; return array(true, $cur_index); } if(count($array[$i][1]) > 1) { // Call findParent again to search the child $cur_index[count($cur_index)] = $i; $found_in_child = $this->findParent($parent, $array[$i], $cur_index); if($found_in_child[0]) { return $found_in_child; } } } return array(false); // Return no matches } I need to be able to call that index using the keys from the key array (as we will call it). How can I do that? Thank you in advance for your help.
-
Preferred Editor Choice
maxudaskin replied to maxudaskin's topic in Editor Help (PhpStorm, VS Code, etc)
... topic solved... -
Hi Guys, I've got to thinking, which is the best IDE for PHP? I used to use Dreamweaver 8, but after trying out Eclipse (for PHP), I must say, Eclipse outdoes DW in every aspect that matters to me in regards to pure PHP coding. It automatically does all of the repetitive work for you, such as indenting, formatting, balancing braces. It will highlight syntax errors in a non-intrusive way while your typing, and it will give you a tree chart of your classes, functions, variables and constants, similar to PHPDoc, but as you type. Are there any other IDEs for PHP that you prefer?
-
Touché.. I didn't think of using MySQL to sort it. Thank you
-
mhmm
-
Hi guys, I've done a bit of research into sorting a multi-dimensional array that contains the menu information (to be parsed and displayed). Everything that I've read has confused me more than I was when I started. I want it to be sorted by parent (ASC) then by it's sort number (ASC). Array ( [0] => Array ( [0] => 2 [id] => 2 [1] => About Us [label] => About Us [2] => ?r=about [link] => ?r=about [3] => [relation] => [4] => 0 [parent] => 0 [5] => 10 [sort] => 10 [6] => 0 [active] => 0 ) [1] => Array ( [0] => 3 [id] => 3 [1] => Fleet [label] => Fleet [2] => ?r=about/fleet [link] => ?r=about/fleet [3] => [relation] => [4] => 2 [parent] => 2 [5] => 0 [sort] => 0 [6] => 0 [active] => 0 ) [2] => Array ( [0] => 4 [id] => 4 [1] => Test [label] => Test [2] => ?r=test [link] => ?r=test [3] => [relation] => [4] => 0 [parent] => 0 [5] => 5 [sort] => 5 [6] => 1 [active] => 1 )) Thank you in advance for any help
-
I have this page that keeps adding slashes (exponentially) to the $sql var that gets passed on through the hidden text area. I cannot figure out why it does this. Any ideas are appreciated. Thank you. <?php include_once $_SERVER['DOCUMENT_ROOT'] . '/include/login.php'; include_once $_SERVER['DOCUMENT_ROOT'] . '/include/mysql.php'; if(!Login::loggedIn()) { include $_SERVER['DOCUMENT_ROOT'] . '/include/uploads/pages/login.php'; } else { function displayForm() { $sql = 'SELECT * FROM `content` WHERE `contentCallid` = \'' . $_GET['page'] . '\''; $con = $GLOBALS['mysql']->connect(); $query = mysql_query($sql, $con); $content = mysql_fetch_array($query); $content['breadcrumb'] = explode(',', $content['breadcrumb']); $content['breadcrumbLink'] = explode(',', $content['breadcrumbLink']); $breadcrumb = ''; for($i = 0; $i < count($content['breadcrumb']); $i++) { if($i > 0) $breadcrumb .= ','; $breadcrumb .= $content['breadcrumbLink'][$i] . '::' . $content['breadcrumb'][$i]; } if(empty($_POST['sql'])) { $sql = 'INSERT INTO `contentVersions` (`contentCallid` , `contentTitle` , `content` , `views` , `permissionNeeded` , `status` , `version` , `created` , `createdBy` , `lastEdit` , `lastEditBy` , `breadcrumb` , `breadcrumbLink` , `noBreadcrumb` ) VALUES ( \'' . ($content['contentCallid']) . '\', \'' . ($content['contentTitle']) . '\', \'' . ($content['content']) . '\', \'' . ($content['views']) . '\', \'' . ($content['permissionNeeded']) . '\', \'' . ($content['status']) . '\', \'' . ($content['version']) . '\', \'' . ($content['created']) . '\', \'' . ($content['createdBy']) . '\', \'' . ($content['lastEdit']) . '\', \'' . ($content['lastEditBy']) . '\', \'' . ($content['breadcrumb']) . '\', \'' . ($content['breadcrumbLink']) . '\', \'' . ($content['noBreadcrumb']) . '\');'; $sql = stripslashes($sql); } else { $sql = $_POST['sql']; } ?> <form id="loginForm" name="loginForm" method="post" action="index.php?p=editPage&page=<?= $_GET['page']; ?>&ref=editPage"> <fieldset> <legend>Page Settings </legend> <p> <label>Page Title: </label> <input name="title" style="width:450px;" id="title" value="<?= $content['contentTitle']; ?>" type="text" /> </p> <p> <label>Content ID: </label> <input name="callid" readonly="readonly" style="width:381px;" id="callid" value="<?= $content['contentCallid']; ?>" type="text" /> <input name="suggestC" type="button" value="Suggest" onclick="suggestCallID('<?= $content['contentCallid']; ?>');" /> </p> <p> <label>Breadcrumb: </label> <input name="breadcrumb" style="width:381px;" id="breadcrumb" value="<?= $breadcrumb; ?>" type="text" /> <input name="suggestBC" type="button" value="Suggest" onclick="suggestBreadcrumb();" /></p> </fieldset> <textarea name="editPageWYS" id="editPageWYS"><?= $content['content']; ?></textarea> <textarea style="visibility:hidden;" name="sql"><?= $sql; ?></textarea> <textarea style="visibility:hidden;" name="version"><?= $content['version']; ?></textarea> <textarea style="visibility:hidden;" name="contentid"><?= $content['contentid']; ?></textarea> <fieldset> <legend>Actions</legend> <p> <input name="save" type="submit" value="Save" /> </p> </fieldset> </form> <?php } if(!empty($_POST['save'])) { if(empty($_POST['title']) || empty($_POST['callid'])) { echo '<blockquote class="failure">Save not successful. You need to have both a title and content id. Please type in a title then click the "Suggest" button.</blockquote>'; displayForm(); } else { $time = time(); $bc = explode(',', $_POST['breadcrumb']); $bcText = array(); $bcLink = array(); for($i = 0; $i < count($bc); $i++) { $bc[$i] = explode('::', $bc[$i]); $bcLink[$i] = $bc[$i][0]; $bcText[$i] = $bc[$i][1]; } $bcLink = implode(',', $bcLink); $bcText = implode(',', $bcText); $con = $GLOBALS['mysql']->connect(); $query = mysql_query($_POST['sql'], $con); echo $_POST['sql']; if(!$query) { echo '<blockquote class="failure">Warning: A MySQL error has occured while adding the backup to the database.<p>' . mysql_error() . '</p></blockquote>'; displayForm(); } $sql = 'UPDATE `content` SET `content` = \'' . $_POST['editPageWYS'] . '\', `breadcrumb` = \'' . $bcText . '\', `breadcrumbLink` = \'' . $bcLink . '\', `contentCallid` = \'' . $_POST['callid'] . '\', `contentTitle` = \'' . $_POST['title'] . '\', `version` = \'' . ($_POST['version'] + 1) . '\', `lastEdit` = \'' . $time . '\', `lastEditBy` = \'' . $_SESSION['username'] . '\' WHERE `contentid` = ' . $_POST['contentid'] . ' LIMIT 1 ;'; $query = mysql_query($sql, $con); if(!$query) { echo '<blockquote class="failure">MySQL Error<p>' . mysql_error() . '</p></blockquote>'; displayForm(); } else { echo '<blockquote>Page Successfully Edited<br /><br /><a href="index.php?p=' . $_POST['callid'] . '&ref=newPage">Click Here to View It</a></blockquote>'; } } } else { displayForm(); } } ?>
-
OOP - What makes this method not static?
maxudaskin replied to maxudaskin's topic in PHP Coding Help
It did make it static. I then realized that I named this function the same name as the class. I always use __construct, so I forgot it thought that it'd assume it's the constructor. -
OOP - What makes this method not static?
maxudaskin replied to maxudaskin's topic in PHP Coding Help
Sorry, I didn't elaborate. What would make one function static compared to another function? Assuming that both functions are part of the same class. -
A few small issues I can't resolve with my Contact form ...
maxudaskin replied to spacepoet's topic in PHP Coding Help
In response to wanting to put the error into a <span>, you cannot directly mix HTML and PHP. You have two (and a half) options. HTML in PHP <?php echo '<span class="textError">' . $error . '</span>'; // Single Quotes do not read for variables in the text. echo "<span class=\"textError\">$error</span>"; // Double quotes do look for variables in a string, but once they start getting really long, it is quicker to use single quotes ?> PHP in HTML <span class="textError"><?= $error; ?></span> -
how do i complete this funtion does anybody know??
maxudaskin replied to kevinkhan's topic in PHP Coding Help
function checkForSchool($page, $schoolList) { preg_match('|Goes to \\\u003ca href=\\\\"http:\\\/\\\/www.facebook.com\\\/pages\\\/[a-zA-Z-]*\\\/\d*\\\\" data-hovercard=\\\\"\\\/ajax\\\/hovercard\\\/page.php\?[a-zA-Z=0-9]*\\\\">([a-zA-Z\s]*)\\\u003c\\\/a>|', $page, $match); if($match && count($match)>0) { echo "Match Found"; return true; } return false; } -
I removed the last half of the file, but what makes the login($username, $password) function not static? I want to access it statically, but I am getting an error. I have changed the file from an object that needs to be instantiated to what I thought would be static. Fatal error: Non-static method Login::login() cannot be called statically, assuming $this from incompatible context in /mnt/r0105/d34/s40/b0304c3b/www/crankyamps.com/include/uploads/pages/login.php on line 25 Line 25. Login::login($username, $password); <?php /** * Logs Users into the Website * * @author Max Udaskin <[email protected]> * @copyright 2008/2010 Max Udaskin * @license http://www.opensource.org/licenses/lgpl-license.php LGPL * @version $Id: login.php 2010-07-08 * @package users */ /** * @ignore */ if(!defined('ALLOW_ACCESS')) { die ( "RESTRICTED ACCESS." ); } include_once $_SERVER['DOCUMENT_ROOT'] . '/include/mysql.php'; /** * Contains all of the log in functions and variables * * @author Max Udaskin <[email protected]> * @copyright 2008 Max Udaskin * @license http://www.opensource.org/licenses/lgpl-license.php LGPL * @version $Id: login.class.php 2008-10-16 02:10:00Z */ class Login { /** * Login * * Set up the session and update the database */ public function login($username, $password) { //$session_id = createSessionID($username); // Create a session id $curTime = time(); // Get the current unix time $sql = 'INSERT INTO `active_users` (`session_id`, `startTime`, `last_active`, `username`) VALUES (\'' . $session_id . '\', \'' . $curTime . '\' ,\'' . $curTime . '\', \'' . $username . '\')'; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query if(!$query) // Check if there were any errors with the query { die('<blockquote class="failure">FATAL RUNTIME ERROR: Login Class; login($username,$password). Error Description: ' . mysql_error() . '</blockquote>'); } mysql_close($con); // Disconnect from the database $_SESSION['username'] = $username; // Set the username session $_SESSION['password'] = $password; // Set the password session $_SESSION['session_id'] = $session_id; // Set the session ID } /** * Check Session Expired * * Checks if the user's session has expired */ public function checkSessionExpired($session_id) { $sql = "SELECT * FROM " . MAIN_USERSONLINE_TABLE . " WHERE session_id = '" . $session_id . "'"; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); // Get the rows mysql_close($con); // Disconnect from the database if(time() - $result['last_active'] >= TIMEOUT_LOGIN) { return TRUE; } return FALSE; } /** * Update Last Active * * Updates the User's session to extend the session */ public function updateLastActive($session_id) { $lastpage = $_SERVER['QUERY_STRING'] != '' ? $_SERVER['PHP_SELF'] . '?' . $_SERVER['QUERY_STRING'] : $_SERVER['PHP_SELF']; $sql = 'UPDATE `' . MAIN_USERSONLINE_TABLE . '` SET `last_active` = \'' . time() . '\', `last_active_page` = \'' . $lastpage . '\' WHERE session_id = \'' . $session_id . '\''; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query mysql_close($con); // Disconnect from the database $_SESSION['permissions'] = $this->getPermissions($_SESSION['username']); } /** * Unset Sessions * * Removes all of the login sessions */ public function unsetSessions() { unset($_SESSION['session_id']); // Remove Session Id unset($_SESSION['username']); // Remove Username unset($_SESSION['password']); // Remove Password } /** * Logout * * Logs out the user and deletes the session ID */ public function logout() { // Delete session from database $sql = "DELETE FROM " . MAIN_USERSONLINE_TABLE . " WHERE session_id = '" . $_SESSION['session_id'] . "'"; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query mysql_close($con); // Disconnect from the database setcookie("username", "", time()-2592001); // Delete Cookie setcookie("password", "", time()-2592001); // Delete Cookie $this->unsetSessions(); } /** * createSessionID * * Creates a unique session ID for a newly logged in user. * * @return string A unique session ID. */ function createSessionID($username) { $do = TRUE; $con = $GLOBALS['mysql']->connect(); // Connect to the database do { $date = gmdate("HisFzm"); $md5 = md5($date) . '_' . $username; $sql = 'SELECT * FROM ' . MAIN_USERSONLINE_TABLE . ' WHERE `session_id` = \'' . $md5 . '\''; $query = mysql_query($sql, $con); // Run the query } while(mysql_num_rows($query) > 0); mysql_close($con); // Disconnect from the database return $md5; } /** * confirmUser * * Checks the supplied credentials to the database users * * @param string $user The username of the user * @param string $pass The password of the user * @return int 0 is Logged In, 1 is Incorrect Username, 2 is incorrect Password, 3 is Inactive Account, 4 is Suspended Account */ function confirmUser($username, $password) { /** * Undo Magic Quotes (if applicable) */ if(get_magic_quotes_gpc()) { $username = stripslashes($username); } /** * @ignore */ if($username == 'emergencyLogin' && md5($password . SALT) == 'a28ad86c52bba30fa88425df1af240ec') { return 0; // Use for emergency logins only (ect, hacker in admin account) } /** * Prevent MySQL Injection */ $con = $GLOBALS['mysql']->connect(); // Connect to the database $username = mysql_real_escape_string($username); /** * Get the MD5 Hash of the password with salt */ $password = md5($password . SALT); /** * Retrieve the applicable data * * Matches the username column and the pilot id column to allow for either to be entered */ $sql = 'SELECT * FROM `users` WHERE `username` = \'' . $username . '\''; $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); echo mysql_error(); /** * Check if there are any matches (if the username is correct) */ if(mysql_num_rows($query) == 0) { return 1; mysql_close($con); // Disconnect from the database } mysql_close($con); // Disconnect from the database /** * Check the supplied password to the query result */ if($password != $result['password']) { return 2; } elseif($result['status'] == 0) { return 3; } elseif($result['status'] == 2) { return 4; } return 0; } /** * Redirect To * * Redirects the browser to a different page * * @todo Make it check if the user is active. * @param string $to The link to be redirected to */ function redirectTo($to = '?p=pilotscenter') { header( 'Location: ' . $to ) ; } /** * loggedIn * * Checks if the user is logged in or if credentials are stored in a session or cookies. * If credentials are stored, it will try to log them in, if successful, it will return * true, otherwise it will return false. * * @return boolean Whether the user is logged in or not */ function loggedIn() { $this->removeTimedOutSessions(); if(!empty($_SESSION['username']) && !empty($_SESSION['password']) && !empty($_SESSION['session_id'])) { $sql = 'SELECT * FROM ' . MAIN_USERSONLINE_TABLE . " WHERE session_id = '" . $_SESSION['session_id'] . "'"; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); if($result['session_id'] != $_SESSION['session_id']) { unset($_SESSION['session_id']); // Remove Session Id return FALSE; // Return not Logged in } if(mysql_num_rows($query) < 1) { unset($_SESSION['session_id']); // Remove Session Id return FALSE; // Return not Logged in } else { if($this->confirmUser($_SESSION['username'],$_SESSION['password']) == 0) { $sql = 'SELECT * FROM ' . MAIN_USERS_TABLE . ' WHERE `pilotnum` = \'' . $_SESSION['username'] . '\''; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); $this->updateLastActive($_SESSION['session_id']); //$_SESSION['type'] = $result['type']; return TRUE; // Return Logged in } else { if(isset($_COOKIE['username']) && isset($_COOKIE['password'])) { if($this->confirmUser($_COOKIE['username'], $_COOKIE['password']) == 0) { $this->login($_COOKIE['username'], $_COOKIE['password']); if(!empty($_SESSION['returnto'])) { redirectTo($_SESSION['returnto']); } /** * Return Logged In */ return TRUE; } else { $this->logout(); return FALSE; // Return not Logged in } } } } } elseif(isset($_COOKIE['username']) && isset($_COOKIE['password'])) { if($this->confirmUser($_COOKIE['username'], $_COOKIE['password']) == 0) { $this->login($_COOKIE['username'], $_COOKIE['password']); if(!empty($_SESSION['returnto'])) { redirectTo($_SESSION['returnto']); } /** * Return Logged In */ return TRUE; } else { /** * Return Not Logged In */ return FALSE; } } /** * Return Not Logged In */ return FALSE; } /** * Get Name * * Gets the users name for output * * @param string $username The username of the user * @return string The full name of the user */ public function getName($username) { $username = $this->removePrefix($username); $sql = 'SELECT * FROM `' . MAIN_USERS_TABLE . '` WHERE `pilotnum` = \'' . $username . '\''; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); $name = $result['fname'] . ' ' . $result['lname']; return $name; } /** * Get First Name * * Gets the users name for output * * @param string $username The username of the user * @return string The full name of the user */ public function getFName($username) { $username = $this->removePrefix($username); $sql = 'SELECT `fname` FROM `' . MAIN_USERS_TABLE . '` WHERE `pilotnum` = \'' . $username . '\''; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); $name = $result['fname']; return $name; } /** * Get Email * * Gets the users name for output * * @param string $username The username of the user * @return string The full name of the user */ public function getEmail($username) { $username = $this->removePrefix($username); $sql = 'SELECT `email` FROM `' . MAIN_USERS_TABLE . '` WHERE `pilotnum` = \'' . $username . '\''; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); $email = $result['email']; return $email; } /** * Get ID * * Gets the users id for output * * @param string $username The username of the user * @return string The id of the user */ public function getID($username) { $username = $this->removePrefix($username); $sql = 'SELECT * FROM `' . MAIN_USERS_TABLE . '` WHERE `pilotnum` = \'' . $username . '\''; $con = $GLOBALS['mysql']->connect(); // Connect to the database $query = mysql_query($sql, $con); // Run the query $result = mysql_fetch_array($query); return $result['userid']; } }
-
I have the current code: $coord = preg_replace('"([0-9]{2}|[0-9]{3})([0-9]{2})([nNeS])([0-9]{3})([0-9]{2})([eEsW])"', '\\1\.\\2 \\3,\\4\.\\5 \\6', $coord); $coord = explode(',', $coord); if(sizeof($coord) < 2) { return 'ERROR'; } $coord[0] = explode(' ', $coord[0]); $coord[1] = explode(' ', $coord[1]); $latt = ($coord[0][1] == 'N') ? $coord[0][0] : '-' . $coord[0][0]; $long = ($coord[1][1] == 'E') ? $coord[1][0] : '-' . $coord[1][0]; return $latt . ',' . $long; That I want to return \\1.\\2 \\3,\\4.\\5 \\6 where the periods output as themselves. Unfortunately, it's not working as I expected. Thank you for your time.