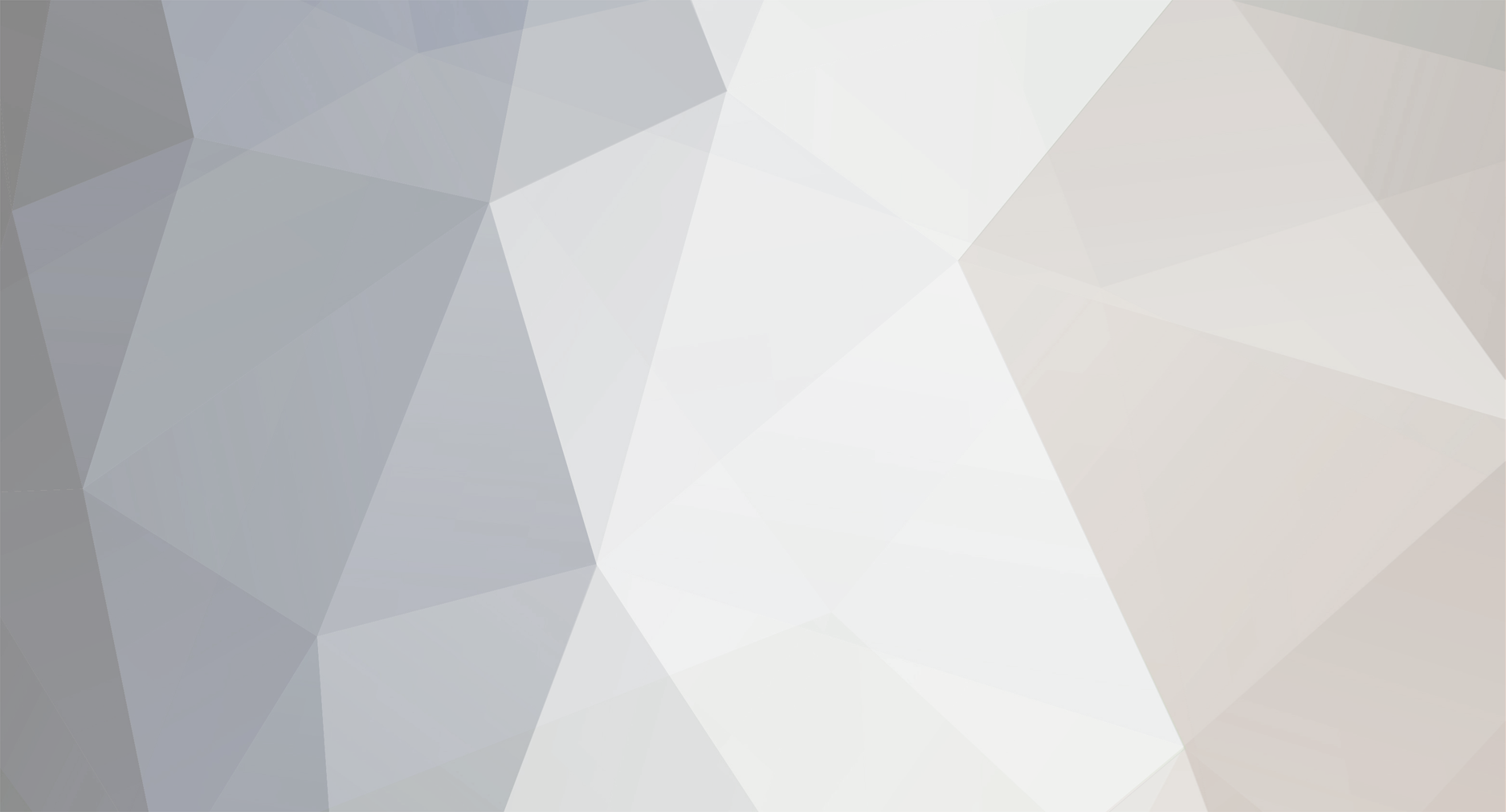
Fadion
-
Posts
1,710 -
Joined
-
Last visited
Posts posted by Fadion
-
-
<?php //get the id from the url and clean it, assuming it is smth like profile.php?id=7 $id = mysql_real_escape_string($_GET['id']); //check if the button is pressed if(array_key_exists('submit', $_POST)){ //clean up the address $address = htmlentities($_POST['address']); //make the query and echo a message $queryEdit = "UPDATE users SET address='$address' WHERE id='$id'"; $resultsEdit = @mysql_query($queryEdit) or die(); echo "Your address was changed successfully"; } //make the query to show the results in the input box $queryShow = "SELECT address FROM users WHERE id='$id'"; $resultsShow = @mysql_query($queryShow) or die(); $valuesShow = mysql_fetch_array($resultsShow); ?> <form name="form1" method="post" action=""> <input type="text" name="address" value="<?php echo $valuesShow['address']; ?>"> <input name="submit" type="submit" value="Change"> </form>
The code was written assuming certain conditions but you can modify it to fit your needs. What it does is display the address in an input box, so if the user wants to change it, he just clicks in the input, changes it and then clicks the submit button, which reloads the same page. When the page reloads, it checks if the button is pressed and if it is the case it modifies the database with the new address. Pretty simple and straightforward. You can modify the styles of the input so it can look a little better then the default.
-
Maybe some code you wrote should help track the problem.
Normaly, for a basic search you should write something like:
$query = "SELECT * FROM table WHERE field LIKE '%$search%'"; $results = @mysql_query($query) or die(); while($values = mysql_fetch_array($results)){ echo $values['column']; }
where $search is the value retrieved from the input.
For searching your two tables you have a set of different possibilites. U can use 2 different queries:
$queryTable1 = "SELECT * FROM table1 WHERE column LIKE '%$search%'"; $queryTable2 = "SELECT * FROM table2 WHERE column LIKE '%$search%'";
Then you execute the query and get the data for each table.
If the tables columns are identical then u can use UNION between the two queries:
$query = "SELECT * FROM table1 WHERE column LIKE '%$search%' UNION SELECT * FROM table2 WHERE column LIKE '%$search%'"
If the case is different than i guess you must use the appropriate JOIN command but im not familiar with those so i cant say much. Hope i helped.
-
This code
echo $result['<td align=center><a href="Image">"Image"</a></td>'];
shouldnt be smth like:
echo "<img src=\"{$result['imagePath']}\" />"
Where 'imagePath' is the path to the thumbnail. You can put the whole thing between <a> tags to link it to the full size image.
Hope i got it right and helped.
How to create the section on login forms which lets users log in forever ?
in PHP Coding Help
Posted
I create my logins system with sessions too, but when you need the system to remember the user, than just stick to cookies.
You can create the cookie with the time limit you want:
For one hour expire:
For ten days expire:
You can set it to expire after 10 years (time()+60*60*24*365*10), so it practically is forever