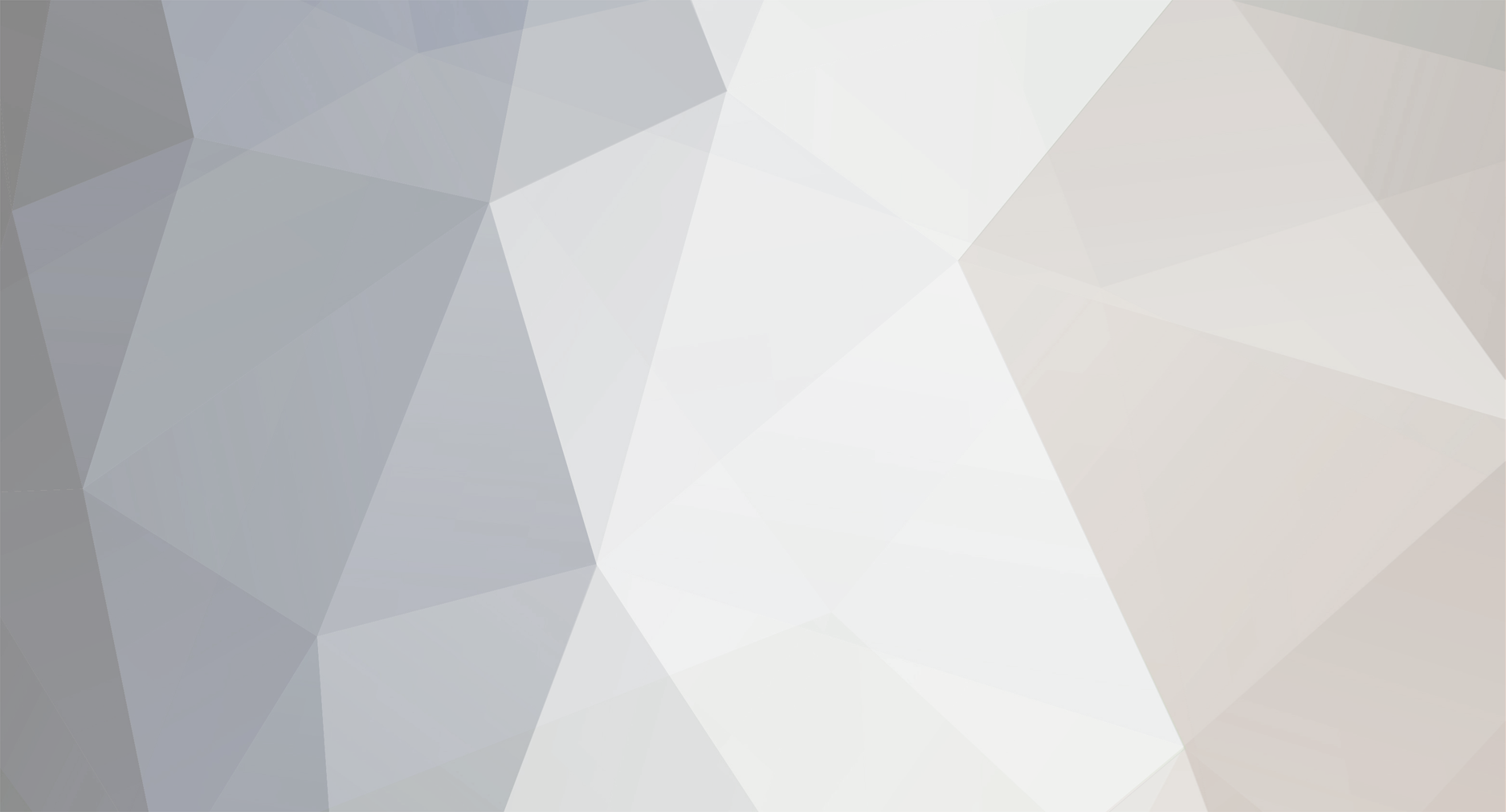
sdi126
Members-
Posts
52 -
Joined
-
Last visited
Everything posted by sdi126
-
PHP does not have tracing built in like ASP.NET does
-
<?$uploadLocation = "schedules/";?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" name="fileForm" id="fileForm" enctype="multipart/form-data"> <input name="upfile" type="file" size="36"> <input class="text" type="submit" name="submitBtn" value="Upload Schedule"> </form> <?php if (isset($_POST['submitBtn'])){ ?> <div id="caption">RESULT</div> <div id="icon2"> </div> <div id="result"> <table width="100%"> <?php $basename = basename($_FILES['upfile']['name']); $filename = (substr_count(".html",$basename) > 0 ) ? $ substr_replace($basename,"",-1); : $basename; $target_path = $uploadLocation .$filename; if(move_uploaded_file($_FILES['upfile']['tmp_name'], $target_path)) { echo "The file: ". basename( $_FILES['upfile']['name']). " has been uploaded!"; } else{ echo "There was an error uploading the file, please try again!"; } ?>
-
The IP address is returned on failure of the function call. I found these on php.net...might work for you: <?php // For Linux... function gethost ($ip) { $host = `host $ip`; return (($host ? end ( explode (' ', $host)) : $ip)); } // For Win32... function nslookup ($ip) { $host = split('Name:',`nslookup $ip`); return ( trim (isset($host[1]) ? str_replace ("\n".'Address: '.$ip, '', $host[1]) : $ip)); } ?>
-
This is the function I use to prevent sql injection: function cleanQuery($string) { if(get_magic_quotes_gpc()) // prevents duplicate backslashes { $string = stripslashes($string); } if (phpversion() >= '4.3.0') { $string = mysql_real_escape_string($string); } else { $string = mysql_escape_string($string); } return $string; }
-
You need to do it as the variable...putting it in quotes is trying to find a form element with the dollar sign in it. $value=$_POST[$value]; Your function should become: function post_data($value){ return $_POST[$value]; }
-
you want to have a look at the mail function. <?php mail('emailto@user.com','subject here','body of the message here...put your $_POST variables');?>
-
Having onsubmit is fine...but first thing you need to do is get rid of the two form declarations you have...there should only be one set of form tags. I am not sure how you are building the dropdown lists though? If the values come from a database...you would just loop through ( like you do to build the options )...but check it against the selected value <?php $_POST["stateList"]; ?> and the current value in the db...if there is a match...you set the "selected" property of the select element (option item). You also need a check around your mysql statement that you call to pull the cities...something like: <?php if(trim($_POST["stateList"]) != ''){ //make call to db here } ?>
-
For question # 1...look at http://www.insidedhtml.com/ie5/htc/ts08/page1.asp For question # 2...this could be limited by setting the width property on the td element such as <?php echo "<tr><td height='200' valign='top' align='left' width='30px'>$evdescription</td></tr>"; ?>
-
There is most likely an error with that php code. If you are using internet explorer go to tools >> internet options >> click on the advanced tab >> under the browsing tab uncheck the box "show friendly http error messages" and then look at the page...it will show you the php error and not "page cannot be displayed"
-
[SOLVED] Problem assigning variables to a session?
sdi126 replied to Solarpitch's topic in PHP Coding Help
Try this: session_write_close(); header('Location: http://mysite.com/somepage.php'); exit(0); The session might not be complete before the next page tries to read it. If this doesn't work I have other suggestions to as this has happened to me before :-\ -
I like drupal.
-
It is because strpos is returning false...it nevers find the position of .pdf if a file is not there... You need to move your else code down like so: if (strpos($filename, '.pdf',1) ) { $pdf_file = $filename; if (file_exists($pdf_file) && is_readable($pdf_file)) { echo "Message: $pdf_file Exists"; } } else { echo "Error Message: $pdf_file does not Exist"; }
-
I like oscommerce...its free...easy to setup and has a lot of mods you can do.
-
[SOLVED] How can I temporarely get more execution time for my php
sdi126 replied to Hardwarez's topic in PHP Coding Help
You can use the set_time_limit function it takes one parameter which is the number of seconds you want to limit your script to. ***Please not that this function has no effect when PHP is running in safe mode. There is no workaround other than turning off safe mode or changing the time limit in the php.ini. -
You would need to loop through all the td tag elements inside of that array also.
-
Echo out the number of rows returned by your sql statement and make sure it is more than one.
-
It just returns a reference to one row. Using array you have access to all the rows returned by the sql statement. Also it is better practice to refer to the variable by name and not by array number....ie $row[4] should be something like $row["freegift"] if your field name was called freegift of course in the table
-
[SOLVED] Execute a certain deed to all entries at once?
sdi126 replied to djfox's topic in PHP Coding Help
The sql code would be something like: I am just going to assume you are using mysql. mysql_query("update table set number = (number - " .$_REQUEST["numberFromForm"].")"); -
Use mysql_fetch_array instead of row while($row = mysql_fetch_array($result))
-
[SOLVED] Execute a certain deed to all entries at once?
sdi126 replied to djfox's topic in PHP Coding Help
Will the field number be the exact same for all entries? Or Will the field number be the same just for the newly inserted rows? -
You are missing the keyword "selected"...both of your if statements are the same. It should be if($row[4] == '$FreeGift') { $auto = '<option selected="selected" value="' . $row[4] . '">' . $row[4] . '</option>'; }
-
Yea that should be an or statement || instead of && So your code would be saying if the extension is not .jpg or not .gif or its bigger than the maximum size you want for uploads...then display the error.
-
Newline characters and not sending emails out
sdi126 replied to greenhawk117's topic in PHP Coding Help
Is this a windows box? If so try \r\n -
What you are trying to do in your example is definetly an ajax solution. Why not just give an input box for the user to enter a coupon code on the confirmation page if they have one. Once they confirm their order do your checking with php and then update the db.
-
Im not sure what the problem is? Do you have an error? You should do your redirect using php like so: header("location: ".$_REQUEST["redirect"]);