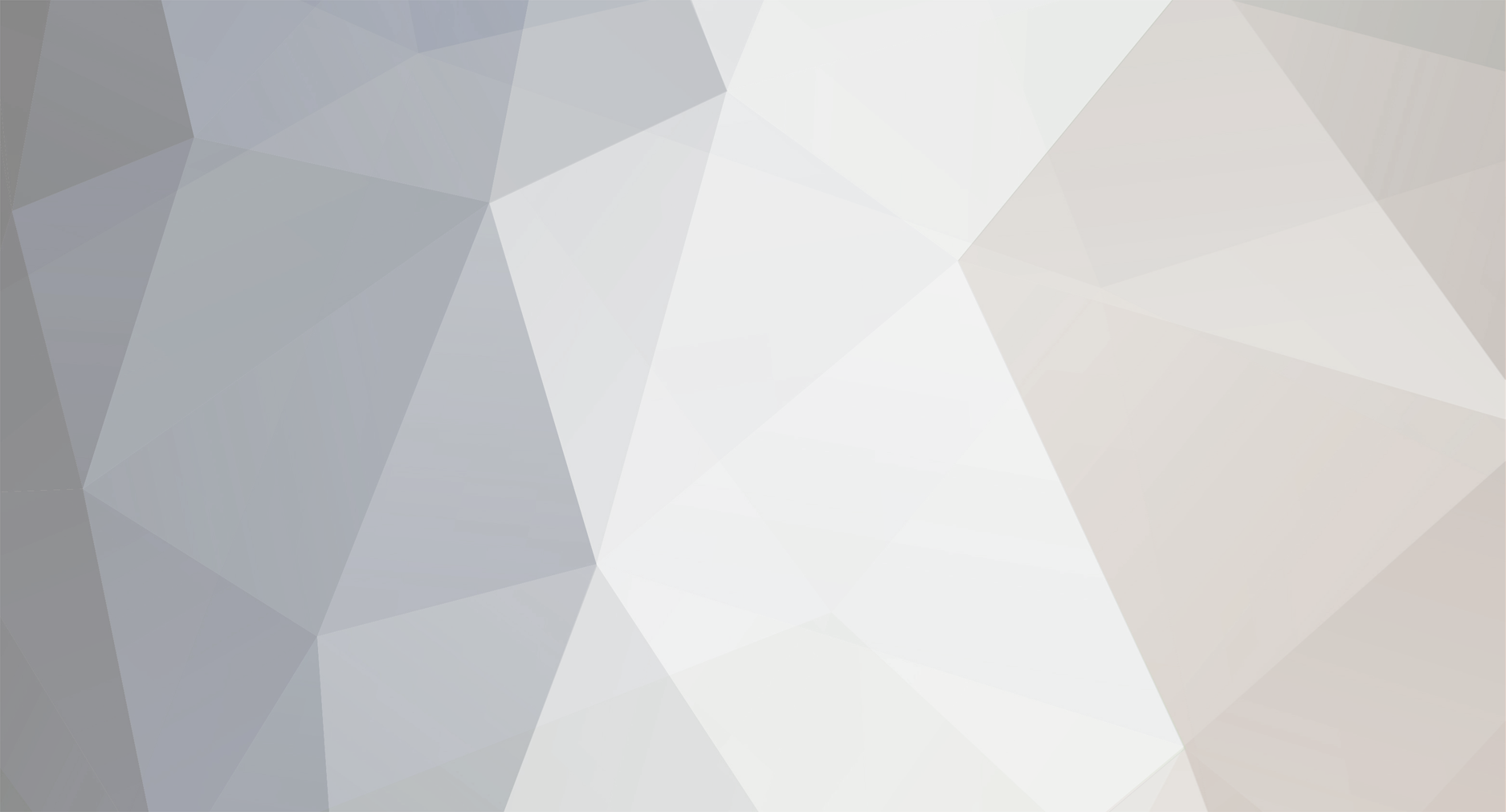
deadimp
-
Posts
185 -
Joined
-
Last visited
Never
Posts posted by deadimp
-
-
They're setting one of the GET/POST variables as an array that is usually a scalar (not array). If you try and manipulate an array as a scalar it'll throw an error and might uncover some vulnerability.
-
Well, not sure what more I can add, so I'll just state why it works for further understanding. You probably already know this, but I'm just avoiding doing homework now.
When arrays are copied (i.e. assigning $test=$array), it goes through the array and copies all primitive data over. Primitive data types are numbers, strings, references, object references, and arrays (fairly certain that they are - subarrays should be copied). Since they are copied and not referenced, changing the primitive data in one array will not affect the other copy.
Note: Object references only refer to objects, and they are the only way to refer to objects. When an object reference is copied, it does not copy/clone the object. That has to be done explicitly. [Applies to PHP 5 only]
Now, if you change data that is referenced in one array, say something in an object, that change will be visible through the reference in the other array, since they're both references.
That's what you are doing with ArrayObject - the iterator returns references to the original object instantiated in the original array, therefore the change is visible through the reference in that array.
Here's just some random examples:
//Basics $array=array(5,15, array(12,7,"pie"), "biscuits", new stdClass()); $test=$array; //All primitives copied $test[1]=18; //was 15 - This does not change the original value in $array $test[2][1]++; //8 $test[3].=" are delicious"; $test[4]->name="Bob"; //Now print out the arrays. All primitive data in $array should remain unchanged. The only different thing will be the stdClass, which will now have the 'name' variable print_r($array); echo "<br>"; print_r($test); echo "<br><br>"; //Try a different approach, same operations $ref=&$array; //referenced $ref[1]=18; //Just wait for it... $ref[2][1]++; $ref[3].=" are delicious"; $ref[4]->color="red"; //Print out - all data should be the same print_r($array); echo "<br>"; print_r($ref); echo "<br><br>"; //And just to screw around $x=15; $array=array($x,"apple",&$x,new stdClass(), array("sub",new stdClass()) ); $test=$array; //Old data in $test left to garbage collector $test[0]=12; $test[1].=" cake"; $test[2]+=58; $test[3]->name="Timmy"; $test[4][0].="array"; $test[4][1]->data=array("good",2,"go"); //Print echo "$x<br>"; print_r($array); echo "<br>"; print_r($test);
Output:
Array ( [0] => 5 [1] => 15 [2] => Array ( [0] => 12 [1] => 7 [2] => pie ) [3] => biscuits [4] => stdClass Object ( [name] => Bob ) ) Array ( [0] => 5 [1] => 18 [2] => Array ( [0] => 12 [1] => 8 [2] => pie ) [3] => biscuits are delicious [4] => stdClass Object ( [name] => Bob ) ) Array ( [0] => 5 [1] => 18 [2] => Array ( [0] => 12 [1] => 8 [2] => pie ) [3] => biscuits are delicious [4] => stdClass Object ( [name] => Bob [color] => red ) ) Array ( [0] => 5 [1] => 18 [2] => Array ( [0] => 12 [1] => 8 [2] => pie ) [3] => biscuits are delicious [4] => stdClass Object ( [name] => Bob [color] => red ) ) 73 Array ( [0] => 15 [1] => apple [2] => 73 [3] => stdClass Object ( [name] => Timmy ) [4] => Array ( [0] => sub [1] => stdClass Object ( [data] => Array ( [0] => good [1] => 2 [2] => go ) ) ) ) Array ( [0] => 12 [1] => apple cake [2] => 73 [3] => stdClass Object ( [name] => Timmy ) [4] => Array ( [0] => subarray [1] => stdClass Object ( [data] => Array ( [0] => good [1] => 2 [2] => go ) ) ) )
-
Sorry, I was thinking that you had designed the iterator object...
I'm not sure how you can change the code so it directly references to your original array variable.
What you might want to do is just see if you can get the data from the iterator, something similar to getSubIterator()... Maybe current() or getArrayCopy().
I'm looking at the reference here.
-
I think I understand what you're saying now...
If you only want to use the email address after the message is sent, you can probably set the Reply-To flag in your email header so that it attaches the return address there, eliminating the need to cross-reference in your database. Therefore, you can probably delete the message, and also remove the mail_sent field. You can also attach more information to your email, such as their IP-address, if abuse or something like that gets to be a problem.
If you want to keep records on your message other than in your email, then you'll want to stay with your original design.
-
You're example ought to look like this, if you're trying to sanitize it before you insert it:
$temp=mysql_real_escape_string($_POST['series']); //Just store it on a temp - You can change this around $q="insert into `series_table` (`seriesName`) values ('$temp')"; mysql_query($q);
That ought to work. The extra variables are just for a little organization. If you want to not use temporaries, you can do something like this:
mysql_query("insert into `series_table` (`seriesName`) values ('".mysql_real_escape_string($_POST['series'])."'");
EDIT: Eh, revraz beat me to it.
does PHP read line by line?If you're asking whether the mysql library (the one you're using now) can execute multiple statements (usually on different lines), then no - though mysqli can. You'll need to have a special function, or split your statements across multiple queries.
-
Just for kicks, this would be the basic functionality of extract($_POST):
foreach ($_POST as $var => &$value) { $$var=&$value; //Sets variable named $var to reference to that value }
This, however, is extremely insecure. The reason this was disabled was for security reasons. If you have code that might depend on variables anybody can overwrite that value just by injecting their own POST/GET values.
As painful as it may be, it's better to manually use $_POST['var'] or $_GET['var'], or even $_REQUEST['var'] if you don't want to differ between those environmental variables.
-
all the validation is done on previous pages. actually the only typed input is the No. of boats, and that is validated on the first page. the rest is all done from selection boxes and and an image upload script.
As revraz said, you might still need to do some solid sanitization of your input at the point of processing. Though you may be validating your input via combo boxes or javascript, there are still ways people can submit information past those security measures.
For instance, someone can send a request to that page and hand-type the POST data, or create an external form directly to that last page, and if you don't sanitize the raw input from POST there's a chance that the information could be dirty.
As for the interfacing with MySQL from PHP, you're on the right track. Just look into different libraries and see which flavors you like. You can use the mysql library which has the common 'mysql_query()' functions, mysqli which is a more object-oriented interface, etc.
-
Yes, the folders have to be empty to be deleted. Usually you use a recursive delete function to go through the whole folder first, delete everything inside it, and then delete the folder, using rmdir(). Be sure you limit the domain of that function, though!
As for giving each individual item a folder, I'm not so sure that'd be a good idea. Unless there's a massive amount of data that goes with each item, it'd be better to make the storage a little more flat. Look into a simple database structure that you can use.
-
As pessimistic as it sounds, you can't really leave it up to end-user honesty to prevent the songs from being illegaly downloaded.
You might need a little more restriction than that, and you might have to end up contacting the company to make sure.
That being said, how are these songs originally available? Are they downloadable via the official website, and if so what disclaimer do they have there?
And to the whole purpose of the site, why would they need to download the song if they already own it? I can understand why someone who already owns a game would want to download a ROM, but as for a song on a computer game that can be ripped, I dunno. Maybe it'd be safer just to give instruction/tools defining how to get the song themselves.
Of course, that's still shaky grounds.
-
Most likely deleting would be more efficient. Less resource usage, less chance of someone gaining access and seeing the information in its raw, unencrypted form.
What I'm wondering is why would the email be sent again after confirmation? Another thing, why do you store the email in the database? I can kinda see why if they need to request another confirmation, but then again if they don't end up confirming their account after a specified time period the user account waiting to be activated should be freed up...
-
We need a little more context. What you're showing here looks like it should work, so the problem might lie in your loop or in the object...
-
The only thing I can think of based off of your code is that you're not passing by reference. Your unset function may just be altering a copy of a part of that array, not the array itself.
Just look into references on the manual and see if that can help you.
Random examples:
//Function arguments function by_value($value) { $value=5; } function by_ref(&$value) { $value=5; } $x=12; by_value($x); //$x is 12 by_ref($x); //$x is 5 //Function return global $test='Hello'; function return_value() { global $test; return $test; } function& return_ref() { global $test; return $test; } $x=return_value(); $x='World'; //$x is 'World', $test is still 'Hello' $x=return_ref(); $x='People'; //$x and $test are 'People'
-
You can use the new object construction as zanus suggested and just use the -> operator, as long as you don't put the static keyword in front of your method declaration. PHP is lenient on calling functions statically. You can call any function using the static member operator :: and it'll work up until a $this reference. That gets a little awkward though, and you've already used the static keyword.
Another way would be to look at call_user_func() and see how you can format callback:
mixed call_user_func ( callback $function [, mixed $parameter [, mixed $... ]] )function - The function to be called. Class methods may also be invoked statically using this function by passing array($classname, $methodname) to this parameter.
So in this context:
$class=getClName(); //Returns a string "C" call_user_func(array($class,"foo")); //Calls C::foo()
You can add whatever parameters you want. Also look at call_user_func_array() if you want some more flexiblity.
EDIT: Fixed the error Anthop pointed out. Otherwise it would have executed C("foo") instead of C::foo().
-
Well, please keep that in mind for later.
-
PHP doesn't appear to have an equivalent to package. How should I organize my PHP trnslation; should I make the entire base a class and have the perl classes internally defined?
Unfortunately, PHP doesn't support embedded classes as pointed out here - though I still don't understand what that person meant exactly by 'type-parametric'...
The only way I can think of is just altering your class name, as ugly as it may seem.
Also, the Perl classes take high advantage of being able to dynamically define object-level variables from within the member functions.PHP doesn't keep a strict watch over object variables. You can define new ones without grief from the engine. The only time it might throw a notice is when you try and read a variable that doesn't exist.
As an example, you can start with an stdClass (a bare object) and just throw in random variables, and it'll work.
$obj=new stdClass(); $obj->name="Bob"; $obj->age=52; print_r($obj);
Should print out: "stdClass ( [name] => Bob, [age] => 52 )" or something like that.
As for method/member functions, it's the same concept:
class Test { function stuff() { $this->name="Bob"; $this->age=52; print_r($this); //Same as above, different class name } } $obj=new Test(); $obj->stuff();
Is the best way to implement this in PHP to have one data array keyed by the values, and insert them as necessary?I don't really get what you're asking here, since that's, well, already implented.
You can use variable variables, though:
$obj=new stdClass(); $var=array('name'=>'Bob','age'=>'52'); foreach ($var as $key => $value) { $obj->$var=$value; //See variable variables in use here, with a sigil ($) being used on the variable after the member access operator (->) } print_r($obj); //Once again, same thing //This actually has the same affect as (object)$var
if you can think of any other caveats that I should look out for in addition to these, please let me know.I don't much about Perl, but I think that it is a little more lenient with its class structure than PHP. I've heard/read that you're able to manipulate your class structures (such as methods, inheritance, etc) run-time in Perl. You can't exactly do that in PHP. Dynamically defining members and using eval() is about as close as PHP gets to that.
Hope I could help with that.
-
The "11" and "submit" comes from the your POST data. Those are the names for the fields on your form, the radio array and your submit button.
You don't sanitize your input at all, and that's extremely insecure. Someone could inject any type of command on their side and it could be executed on your MySQL server.
Making the loop based off of something other than pure POST data would be best. You need to better define your application's limits.
-
Looks pretty good, just the color scheme is the main thing that jumps out, like the others have said.
Also, it looks like you coded some of your JavaScript for IE. You're using document.all for you little ticker, and FF doesn't support that.
-
To do some simple debugging, call print_r() [recursive print] on your foreach iterator and see if it's an object. If it's not a lot of data, post it on here.
-
It depends on your long-term goals, I guess. If you plan on having your library be modular, you might want to look into separating your resize code from your direct media-management code.
Honestly, just uploading/resizing your pictures shouldn't be the main part of your worry. The hardest part is the higher-level stuff, such as how to manage your directories and the different situations that arise from that.
-upload to a temporary directoryThat's already done by PHP/your server. Uploaded files are placed in the temporary folders designated in the PHP/server configuration.
If you're caught up mainly on design, I'd say just go for it. Usually as you go along you'll begin to realize better methods for doing things, and if your project is realitively small and you don't have a strong 'connection' to your existing design it should be easy to revise. Note that this probably isn't the best method for 'industrial' coding, but works really well from a learning standpoint.
-
The "low-level prefetch data" was more or less of a guess on my part - I should have said that before. The basic idea would be like that of Java or an interpreted language, where some of the byte code generated from the script is stored and used again if the raw format of the script (text) hadn't been changed. If you want to learn more on this, you probably ought to go to the Core PHP Hacking forum.
If you're able to view your PHP files on your server by just marking them with the *.php extension (or whatever else you designate in your httpd.conf file).
I'd say more, but I gotta go.
-
Getting the user agent string from the client (browser), like Silverado_NL said, would be your best bet. You could use either javascript or php ($_SERVER['USER_AGENT']) for that.
-
Heh, you mentioned applying your C++ logic to PHP. A post from GDNet: http://www.gamedev.net/community/forums/topic.asp?topic_id=390500
The basic jist of it is, when you're programming in PHP, focus on PHP.
I think I know what you're going through, wondering how persistence might work with your applications. Well, the only thing I have to say is that yes, the scripts are run essentially from scratch on every request. Luckily the monotony of it all can be reduced by using cache, be it low-level prefetch data on your script generated by your PHP engine, or high-level cache structures included in whatever framework you may be using. Still, on each request the script is ran from the top - that's one of the reasons why PHP can be added as an HTTP module, so it doesn't have to restart the engine on each request.
-
What do you mean by adding . or .. to the script? If you're talking about directory listing, I don't see any code that does that.
And a tip: Try to keep your directories relative, to make it easier for cross-platforming. If not that, then at least use standard directory conventions (posix/unix/whatever-style).
-
It doesn't look like you're iterating $i. You might want to look into for loops for that, or a while loop with automatic result iteration, like mysql_fetch(). Ex:
while ($row=mysql_fetch($res)) { print_r($row); echo "<br>"; }
__autoload issues
in PHP Coding Help
Posted
I'm also kinda confused why it can't be global... Global data isn't constant (unless you explicitly define it as such), so you can change it.
This auto-include system seems like it'd take a little more maintainence than the developer normally included the file or conformed to naming conventions.
Then again, centralizing the information would help when you reorganize and you don't have to comb through your files again and change your includes.