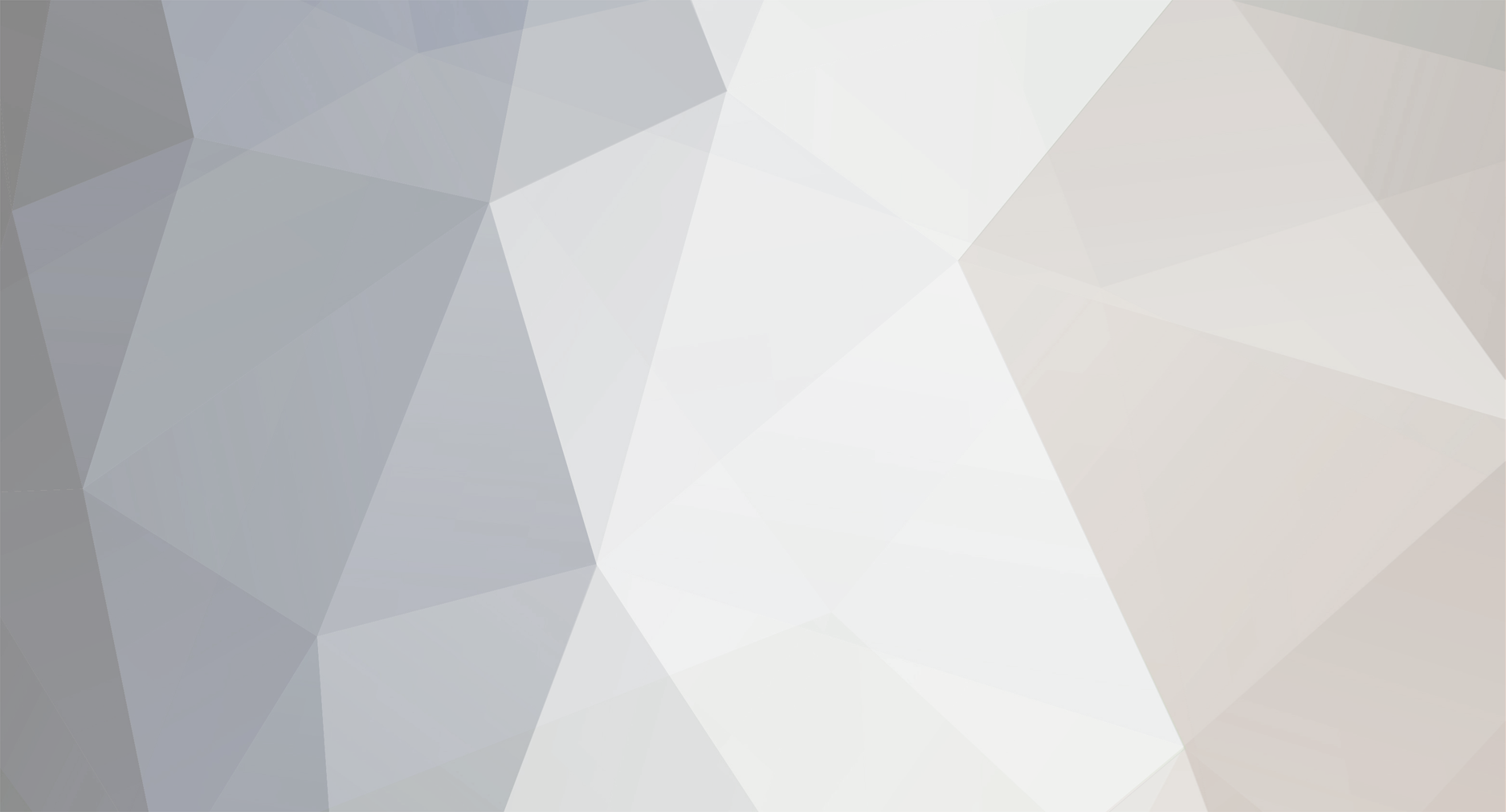
deadimp
-
Posts
185 -
Joined
-
Last visited
Never
Posts posted by deadimp
-
-
Yes there is a way to do it. You can use php to go into the file and edit the parameters and then send the finished text to the browser and get it all to work. This would be dificult and a waste of time.
It isn't that hard to have PHP pass through a css file. The hardest thing I've found about it is finding an IDE that can different from *.php and *.css.php masks so the highlighting doesn't collide (I ended up using *.phtml for PHP/CSS files, though some hosts might not have the extension defined for PHP). The main thing to keep in mind when using PHP with CSS is to make sure the browser knows that its mimetype is CSS, by using:
header("Content-Type: text/css");
Of course, this won't exactly help you with your percentage dilemma. Kinda off-topic...
-
What you need to do is combine the functionality between select() and MyAjaxRequest(). For example, have one link as 'select(this,"main")', where the second argument is used to determine what should be sent to MyAjaxRequest(). If you need more arguments, simply add them.
Remember that the code I gave you was more or less of a template. It's up to you to specialize it. As far as the className thing goes, I really can't say I know. I'll test it out some.
EDIT: I looked around a little, and it seems the best way to remove a class name is to pad className with spaces on both sides, and the use replace to take out the class you're looking for.
-
Instead of having to embed your PHP in your heredoc literals to get the user info at that point, try getting it ahead of time to make things a little more aesthetic.
-
I think there's a library included in PHP called GeoIP that does that for you.
-
Actually, since it's in a string, you don't really need quotes.
But one thing you'll need to observe is the possibility of an SQL-injection attack, meaning someone could rewrite your query to do malicious things - which isn't good. Be sure you escape your data properly.
-
No, you need to keep the href attribute blank (with a '#' so it uses the hand cursor) so that it doesn't change pages. You put your Ajax request in the section I commented in select(). For example, if your page urls are different enough, just have the second argument of the function contain the url stuff, such as "?L=tour.tour&type=start".
The ... is just there as a placeholder. You put your styling information there.
EDIT: I made a syntax error in my previous post. It should be:
select(document.getElementById("link_sel"),"page");
-
Google ought to help you in this respect. Your question's a little too general to give anything specific.
The hardest part in this will be managing the HTML for the table.
-
The easiest way to dynamically highglight a selected link is to store the currently highlighted link, and on a change, deselect the current link and set the current link to that new one. Whenever a link is selected, just add a class name to it so it has those different properties in CSS, and when deselected, remove the class.
The best way to do the whole selection thing is to have the links work on 'onclick', so that they have access to themselves as 'this', whereas using the javascript protocol with href will not give them that access.
Example:
<style> a { ... } a.sel { font-weight: bold; background: ...; } </style> <a href='#' onclick='select(this,"something")'>Something</a><br> <a href='#' onclick='select(this,"else")'>Else</a><br> <a href='#' onclick='select(this,"another")'>And another thing</a><br> <script language='javascript'> var sel=null; function select(obj,page) { //Set the previously selected one back to normal if (sel) sel.className=sel.className.replace(" sel",""); //Replace it - I'm not sure how well this'll do in Firefox... Maybe you ought to look into something like jQuery to see how to manipulate classes better //Set the currently selected one to selected sel=obj; sel.className+=" sel"; //And now do some Ajax stuff according to the page variable } </script>
As for when the main page loads, to select the default page, the best thing to do would be to have PHP, when you're generating the links, have the default one selected (with class='sel'), give it some sort of unique id (like id='link_sel'), and then call your select function like this:
select(document.getElementById("link_sel","page");
EDIT: Looking back over some of this, I'm still unsure about the whole class manipulation thing... Be sure to google that.
-
How do you want them to change in relation to the user's input? You want one to be selected and the other deselected?
-
One thing I want to throw out there: Javascript (as I've tested in IE 6, Firefox, and Opera) has the funcions escape() and unescape() defined, meaning they are built-in functions. Therefore, you don't have to write your own encoding/decoding functions to make your GET/POST data url-safe.
Example:
[code]var title="The End";
var html="Hurrah!<br>And so it goes & goes! I ain't no sore, not @ the world no more. Url: \"index.php?page=etc&stuff=2\"";
url="index.php?page=etc&title="+escape(title)+"&html="+escape(html);[/code]
Nothing complicated about it. -
The reason it's inserted before your table header is because your <span> tag is just floating around in your <table>. You can't exactly have it like that, since your DOM renderer doesn't know where to put in respect to the rows.
Problem with altering rows in a table is, IE's a bitch. Plain and simple. By that, I mean if you try and alter a row's contents via innerHTML (which could involve adding a new row) IE will throw it's hands up and lob out an 'Unknown runtime error'. Now, this isn't a problem if your target audience is people with standards-compliant browsers, but that's highly unlikely.
I've made my own simple Ajax-enabled table (which right now only changes rows, I haven't gotten around to adding new ones, though that won't be too much of challenge in Firefox), and used this concept and ignored IE. That isn't good though, since I'm excluding some people. One solution I'm thinking about right now is splitting the data up into their respective columns (since I've started using JSON encoding, thank God) and using JavaScript DOM control to sprinkle in/alter the data. Fun stuff.
-
I've just tested this on Firefox, so I'm not sure about IE, but it ought to work.
Just create a style element using Javascript and append it to the document body.
Example:
var body=document.body; var extra=document.createElement("style"); extra.innerHTML="a { color: #000; }"; //There are probably other attributes of a <style> tag, but you can use this body.appendChild(extra); //This will place it at the end of the document's body
Wait... Are you only trying to change the color for that one active link?
-
Yeah, that's essentially how I've doing it. Your point on security is correct, in that it can filter out (ie. not respond) to any undefined functions / actions.
As for where I store this, I actually switch between writing the Ajax response in the main script that requests it (by putting the variable 'ajax' in GET) and having a separate page. As to whether which style is better I can't really decide yet. Having the Ajax right next to everything else in the same file makes it easy to change the functionality, but it sometimes becomes cluttered. Having the Ajax response handled in an external file is good if you want to avoid clutter, but you have to be sure to maintain that file and others for that, uh, module.
-
Look into (search for) HTTP/Digest Authentication, and look at the .htaccess files cPanel is creating, if it's creating any.
But why are you allowing a public script into a private folder?
-
I'm sure there are smaller CMS's/frameworks out there that you could probably learn something from. I've started my own, Thacmus, which I'm trying to keep small and compact for hobbyist programmers (like myself) who don't need the industrial strength of the other CMS's (not aiming for quantity, though that doesn't mean that I'm shirking on the quality side). All in all, I'm kind of designing it for rapid application development.
I started the CMS to minimize on repetition in my HTML and PHP, and make it easier on myself since I'm somewhat lazy. I also intend on using it make the website for my other programming projects (a game engine and what not), for my personal website, and for a forum.
Magnitude is something to consider, but you have to figure out what kind of magnitude it is, how many users there'll be (popularity), how complex the structure is, what kind of resources you have, etc. Look into different systems and see what style suits you best. Try developing in different ones, or simply start your own (if you choose to do so).
-
Well, that and efficiency, since it'll bug me that it will have to be connected per-class (or I'll have to make a separate description class, or something like that), though that's not of great importance.
-
The only problem I'm thinking about with the backbone structure would be precedence. Then again, it could be set in how the subject classes are set...
-
Have you checked whether it's the filesize?
Also, why do you store the max filesize in your form? Someone could easily recreate a form and set that limit to whatever they choose. It's better to store the limit in your script.
-
It depends what your situation is. Usually there's a PHP configuration set that limits the size of uploaded files.
-
Notify your host's support system. It could be that they don't allow .mp3 files.
-
I had thought about making the combined OrderedUserItem, which would involve deriving from one of the two, and then incorporating the other's properties and explicitly calling that class's properties using PHP's method invocation (Class::method()) which are still $this-calls.
However, I didn't think that much about using object overloading to try and do all of that. But yeah, running into cases where they share the same function/property (which will happen, since they're both derivates of DBI, database interface) will be somewhat of a pain to resolve.
Thanks for the info!
-
You'll need to format your normal text to HTML, either by using some PHP function or for simply handling line breaks, replace the characters ("\n" (LF on Unix), "\r\n" (CR+LF on Windows)) with the HTML break tag "<br>".
-
The code dbillings posted inserted the file into a database. You would need to add your own resizing code to that.
Look into move_uploaded_file(), and after you've moved it, take your thumbnail function and use it on that file, generating the thumbnail in the relative directory (thumb/, or whatever you've chosen).
-
What you could do is have the range of pages shown be dependent upon the current page you're viewing, much like how the pagination for this forum works. So if you really needed to view the next ten pages, you could just increase the page count from the current by 10, and solve that problem.
Tabbed Menu How To?
in CSS Help
Posted
Google. "css tabs"