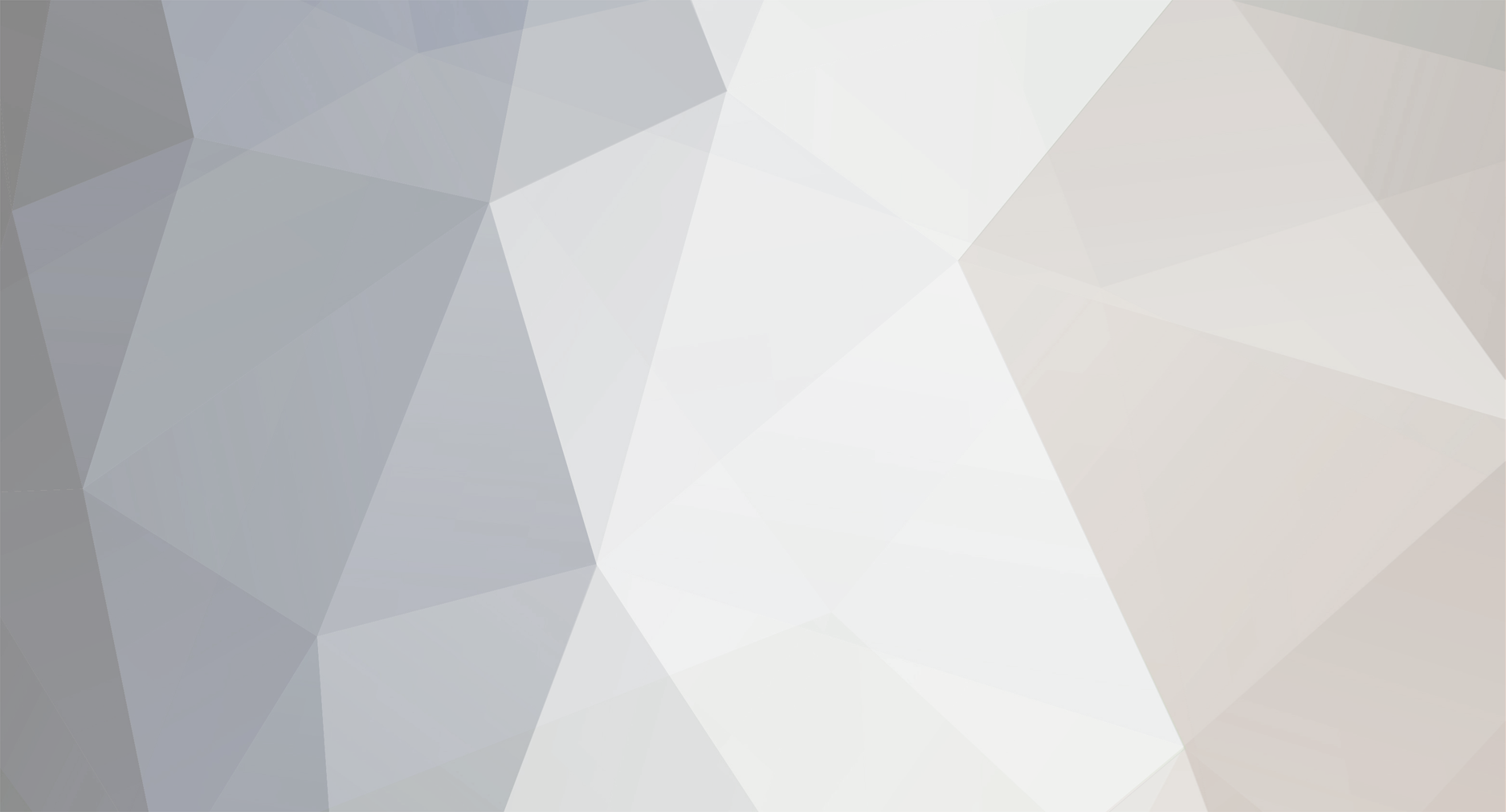
deadimp
Members-
Posts
185 -
Joined
-
Last visited
Never
Everything posted by deadimp
-
It would be better to do the pagination through MySQL LIMITs. That way is more efficient, since the MySQL server only sends you only the data you use to your script - much better than having it send you all of the data, and then cropping it off and leaving it for garbage collection.
-
Google is your friend. Try something like "ajax select". The first result is exactly what you're looking for (though the implementation looks a little iffy).
-
Make a block in Javascript that handles a certain flag returned by your AJAX request, and then either change the document location (better) or create a meta-refresh if you're paranoid (worse). Example: <ajax.php> $data=array('good'=>false, 'list'=>array()); if (mysql_numrows($res)) { $data['good']=true; //... Insert you data or whatever in the list } echo json_encode($data); <stuff.js> function ajax_handle(str) { //... Proper states, etc eval("var data="+str); //Ghetto JSON decode if (data.good) { //... Handle all the data } else document.location="redirect.php"; } That's the basic jist of it.
-
AJAX and AHAH are just different terms for just about the same thing. The Wikipedia article states it's just a subset so there's not much difference. I myself don't use XML in AJAX but instead JSON encoded data. Just because it's not XML doesn't mean it's not AJAX. Ajax is just the accepted name for the design. At least, that's my spin on it. If you want to see examples of what I'm doing go here or look around in the source.
-
What exactly is the problem with the old items showing up when you hit the back button? Does it just show the old response sent by the server (the old page) or does it resend the request and re-process (so that the items are actually re-added)? If the back button is just turning up old cached browser data, don't worry about it. That's a per-browser/user/client preference, something you probably oughtn't mess with. If it's reprocessing it, do something like Gath said, with a redirection. Another method for redirection would be to alter the header's location after some sort of form processing: header("Location: index.php"); Essentially does the same thing as the meta-refresh. Only thing is, you have to make sure that the header information is sent before the main body of it all is, so you'd need to do some organizing with your code.
-
[SOLVED] how to make my jobs easier n smooth?
deadimp replied to zgkhoo's topic in Application Design
Plan what you're doing ahead of time, and revise code that you've already done to see if there's a more clear way to implement it. Planning may involve actually writing something down in a flow chart, jotting down quick notes on a scrap piece of paper, or just simply thinking about it. -
[SOLVED] Force MySQL to use decimal numbers instead of sci. notation
deadimp replied to benjam's topic in MySQL Help
This deals with the MySQL command line console rather than raw data retrieval from MySQL itself. Sadly, I don't use the console (at all), so I don't know how to change the formatting. -
[SOLVED] Force MySQL to use decimal numbers instead of sci. notation
deadimp replied to benjam's topic in MySQL Help
How are you outputting the results from the MySQL query? Are you using the command line tool, PHP, ...? -
Get data from relational key reference to same table [Sort topics by date]
deadimp replied to deadimp's topic in MySQL Help
The left join works now, and I don't know what I was doing before that made it not seem to... Must've been the data I was working with. This is the final solution I came up with: select topic.* from `post` topic left join `post` latest on latest.`id`=topic.`latest_id` where topic.`topic_id`='-1' and topic.`forum_id`='1' group by topic.`id` order by if(topic.`latest_id`='-1', topic.`date`, latest.`date`) desc Thanks! -
Look into the include() series (include, include_once, require, require_once) of functions. What that does is execute the code in that separate file in the scope of the main file. One random thing to keep in mind is that you can have a script return to stop execution, but it only works at that file's level: index.php <?php echo "Hello<br>"; $type='return'; require("test.php"); echo "I'm still alive<br>"; ?> test.php <?php echo "Included script<br>"; if ($type=='return') return; //Have the script return if ($type=='die') die(); //Kill it all echo "Included still alive<br>"; ?> Output for $type='return': Hello Included script I'm still alive $type='die' Hello Included script If you're looking to redirect a page to another immediately (without a meta-refresh), use header("Location: $url")
-
Get data from relational key reference to same table [Sort topics by date]
deadimp replied to deadimp's topic in MySQL Help
How do I have the join account for possibly missing latest posts? I read in the MySQL manual that the left join creates NULL fields for relations that don't exist in table B. I tried it on my localhost with some test data and it didn't return a row for topics without latest posts. The self-reference method isn't ideal for myself, since it would need to know its id ahead of time to use the information. That, or it would need a second query to update `latest_id` to itself. Then again, as I'm thinking about this, maybe I should just stick with the second query, unless someone has a reasonable objection to it. -
Incrementing a number then inserting it into the database problems
deadimp replied to cturner's topic in MySQL Help
If you're just trying to increment the value of a field in a row, just use a simple update expression: update `menu` set `mainid`=`mainid`+1 where `id`=? where `id` is your primary index, and ? is the value of the id. If you want to insert an item into a table with one more than the largest value: insert into `menu` (`mainid`) values (max(`mainid`)+1) The max() statement ought to work. If not, you could use a subquery or sometihng like that. What's the point of this, though? You already have indexing with your auto increment field... -
[SOLVED] Refresh page and pass a value across
deadimp replied to noobstar's topic in Javascript Help
You're getting confused in the ways PHP and Javascript are processed. PHP (PHP: Hypertext Preprocessor) is a server side language. That means that all of that your code is executed and outputted before the file is sent to the client. Javascript is a client side language, meaning that the code is executed at the client's side, hence it's executed after the file is sent, after any server-side processing takes place. In this context, PHP executes all of the PHP code first, and then sends it off: Try putting this line at the end of your code: <?=$artpic?> or <?php echo $artpic?> If you notice, it'll always output the last thing you set it to, "My Article". That's simply the way the code is executed. Try using GET variables (url query). Example: (you don't really need javascript, or radio inputs for that matter) <a href='test.php?var=outside'>Outside Article</a><br> <a href='test.php?var=my'>My Article</a> test.php: <?php $var=$_GET['var']; //This gets the variable from the query if ($var=='outside') echo "Outside article"; else if ($var=='my') echo "My article"; ?> -
It's a basic question, but: What's the best way to have javascript focus on a link without adding the identifier 'clause' to the end of an url? Example: index.html Random html... <a id='anchor' href='...'>Anchor</a> More random html To have a browser focus on the anchor via the url, you just add #anchor to your url. This is what I've come up with: <script language='javascript'> function anchor_focus(name) { var obj=document.getElementById(name); obj.focus(); obj.blur(); } //Example anchor_focus("anchor"); </script> That scrolls it into view, and of course deselects it to mimick appending "#anchor" to the url. It's worked on Firefox, IE, and Opera (all Windows). Any other suggestions?
-
Get data from relational key reference to same table [Sort topics by date]
deadimp replied to deadimp's topic in MySQL Help
Thanks for the help! I've tried out the join approach, screwed around with left/right/inner joins, but I still haven't exactly learned what the difference is and how I can make them do what I want (essentially the problem in all programming). I wanted it to be able to handle `latest_id`=-1, but when I tested out the joins the results only brought up topics with a valid relation (topic.latest_id=post.id). So I ended up just using a basic sub-query as I had before, but also stuck in a conditional (once I learned how to do that): select `t`.* from `post` `t` where `t`.`topic_id`='-1' order by if( `t`.`latest_id`='-1', `date`, (select `date` from `post` where `id`=`t`.`latest_id`) ) desc There's an alternate sub-query I was considering, but I'm not sure it's faster than the first: select max(`date`) from `post` where `topic_id`=`id` Since it would have to go through multiple rows to get all of the data, I'm sure it'd be slower (though by an insignificant amount, most likely). Is there a more aesthetic way to do this with joining, or is the sub-query way the simplest? -
I know it's a bit of a confusing and generic title, but here's my situation: I'm designing some forum software based off of Thacmus, and I want to know the easiest way sort topic in the forum view according to the date on their latest post. All topics / posts are stored in the table `posts`. The fields in focus here are `id`, `date`, `topic_id`, and `latest_id`. `topic_id` is the relational key for a post's topic (if it's a topic itself, this value is -1), and `latest_id` is just a reference to topic's latest post. One implementation I'm thinking of is having the an empty topic's (a topic without replies) latest post just reference to itself, so something like this query could work without any extra voodoo: select from `post` where `topic_id`='-1' order by (select `date` from `post` where `id`=`latest_id`) desc I think this could be done better using a join, but I'm just a MySQL novice. As you see, if the post's latest id references to itself, then it won't return a null date on the nested select statement. Anyone know of a better way? NOTE: I didn't included the forum info in there 'cause that's just implied.
-
Post some more code and possibly an online example. We need some context here.
-
If you want to use CSS, be sure you know how to use CSS. Be sure to know the right question to ask. It looks like you know what to do with PHP, and I'll just elaborate with correct CSS, like jesirose said: <body style="background: url('<?=$image?>')"> You need to have short tags enabled for PHP on your server, and it should be. If not, use <?php echo $image php?>.
-
I didn't see anything in the PHP Manual on Reflection on how you could create anonymous classes, and create_function() has it all defined via strings and doesn't have a natural feel to it. I was doubtful when I posted this, I was just hoping there was some hidden feature in there somewheres. Thanks for the info.
-
Hacking together a sort of Virtual Inheritance in PHP?
deadimp replied to deadimp's topic in Application Design
I'm not sure that delegating parent method calls to actual instances of a parent would work with the structure using DBI in Thacmus... DBI has two 'parts', the static part, which is your basic database wrapper, and the instance part, which has a child class extend it and use it for interfacing with the static part. The main problem I'm thinking of is the dreaded diamond structure. My first example of having a Virtual class extend OrderedItem and UserItem would be an instance of a diamond structure, because both are DBI classes. Each DBI class has its id, the id for its row in its defined table. If I use delegation like that, I would have to make sure that the common date between the virtual base (DBI) is shared equally among them, and that could be a pain. Plus, I would need to make sure that the table they reference to in their database calls (CRUD) would be that of child Virtual class, not their own. That's why I want to stick with simply scoping the parent methods over the child Virtual class, to simply the process of 'data synchronization' across the multiple types of parents. Arg! Too much stuff to think of... Thanks for the help so far. -
Small framework for an 'MVC' + registry pattern
deadimp replied to drcphd's topic in Application Design
There aren't really stupid ideas when you're learning something new... Unless what you're doing is counter-productive (which kind of negates what I just said). I you feel you have a sturdy structure in mind, or that you could develop one over time, just go for it. It's a learning process. If it fails, move on to something else. If you aren't sure of or don't believe in a project you start, don't plan on killing yourself over it. Be flexible. [insert more motivational clichés here] Your implementation of a registry, I'm not entirely sure of... It looks a lot like a singleton interface, which is probably what you're looking for. That, or just use globals / statics (ie. Database::$current/$instance/$default/etc) for your classes. It looks like you have the basic grasp of OOP, and all I can say at this point is browse around the PHP documentation for language/syntax info along with docs on libraries. In addition to that, find yourself a well-written or popular (one doesn't always imply the other ) library, a CMS, framework, some tutorials, what not, and browse around the source some, see what you find. -
I'm just curious: Is there a possibility of anonymous classes with methods in PHP? Something like JavaScript or C++: //JS - Rough sketch var obj={ name: "Bob", explain: function() { return this.name+" es tan padre"; } }; document.write(obj.explain()); //C++ struct { string name; //No ctor because of C++'s definition style string explain() { return name+" es tan padre"; } } obj; obj.name="Bob"; cout << obj.explain(); Each one will output "Bob es tan padre". I know you can use a blank stdClass, a null variable, or an array that's converted using the object operator to create an object, but as far as I know you can only define variables. EDIT: Err... Corrected my JS code. Another thing I'm looking for, if this is even possible in PHP, is inheritance with anonymous classes. I know you can do this in C++: struct Test { virtual void blarg() { cout << "Nadie"; } } ... struct : public Test { void blarg() { Test::blarg(); cout << " es el mejor"; } } bob; Test *ptr=&bob; ptr->blarg(); Output: "Nadie es el mejor" Of course, with C++, the classes are scoped, so you could name a class inside a function or whatever and not really have to worry about collision (which isn't the case with PHP).
-
To fix the way you were doing it before, use the global keyword to access global variables: function DoMore() { global $MySQL; $blah = $MySQL->DoQuery('blah', 'blah2'); $query = $MySQL->FetchArray($blah); return $query; } But if you want a different approach, I'd use the static style (MySQL::query()...) if it's only basic stuff you want. If you're looking for more advanced stuff with your database, do something else. What I'm doing with Thacmus is basing all database classes off of DBI, which also contains static functions for my MySQL wrappers. (The stylesheets for the site are broken, so you might want to view the plain text version)
-
If you're referring to the API style, then I'd use OOP, since you've already done so in the class you posted. Using the global functions 'mysqli_x()' are redundant when you have the link. It'd be easier just to type '$this->link->x()'. Be sure to look into mysqli_result in the documentation also.