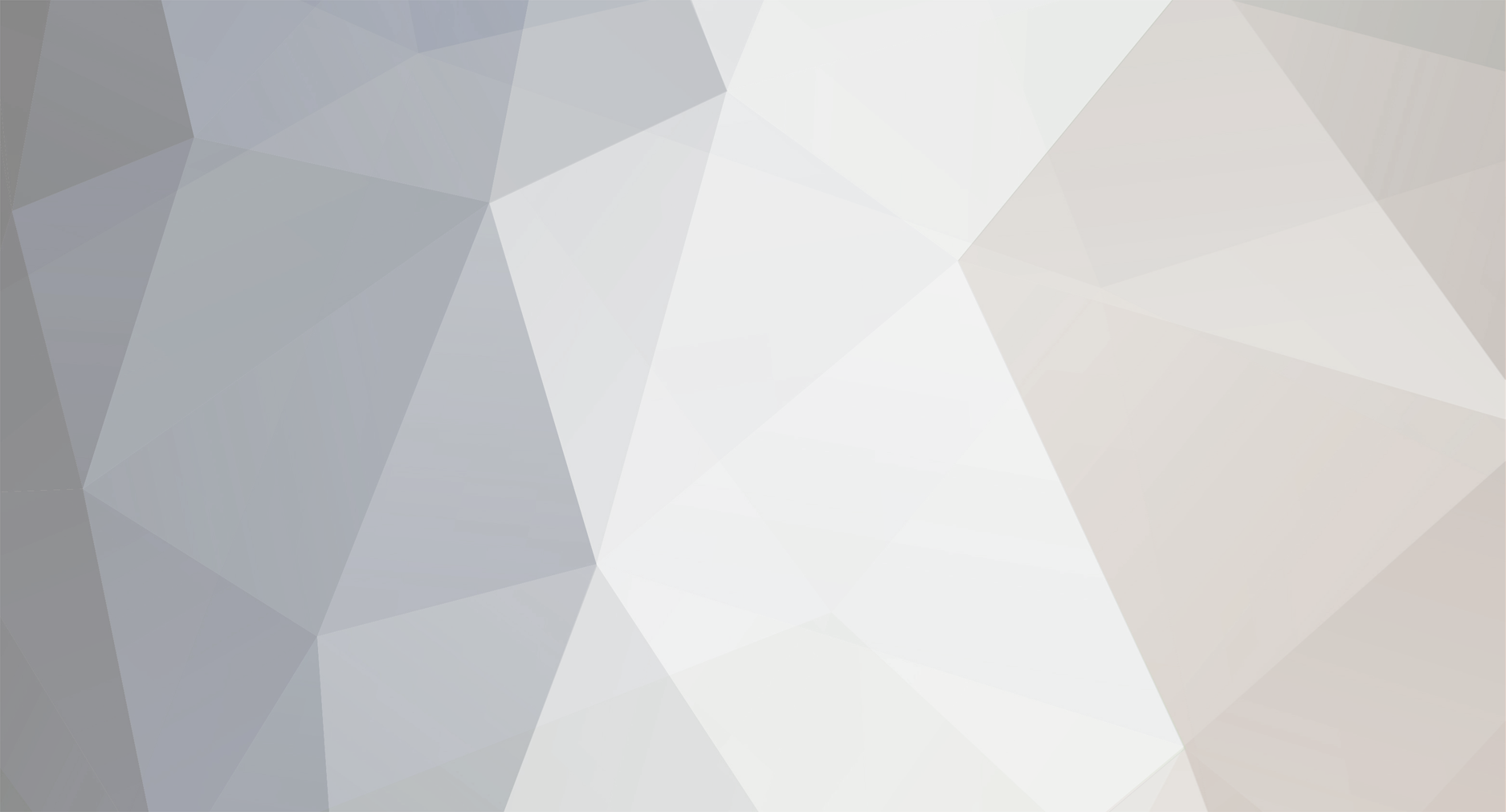
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
header That is probably the best way to redirect in php.
-
<?php $folder = "greckle/"; // set this to be "folder/" if the script is included in a folder define("DOC_ROOT", $_SERVER['DOCUMENT_ROOT'] . "/" . $folder); define("SITEURL", "http://" . $_SERVER['HTTP_HOST'] . "/" . $folder); The DOC_ROOT is for including php files with include See above, the $folder is the folder containing the script. That should fix you up.
-
I always set constants for siteurl and document url using: $_SERVER['DOCUMENT_ROOT'] and $_SERVER['HTTP_HOST']; IE: <?php $folder = ""; // set this to be "/folder" if the script is included in a folder define("DOC_ROOT", $_SERVER['DOCUMENT_ROOT'] . "/"); define("SITEURL", "http://" . $_SERVER['HTTP_HOST'] . "/" . $folder); Then in your script just use this: <LINK REL=StyleSheet HREF="<?php echo SITEURL; ?>style.css" TYPE="text/css"> That way it keeps the site dynamic and you can put it on any server in any directory and it works
-
One option is to use sessions, record what page it came from and redirect back. The other option is to use Javascripts history.go(-1) feature, but if you are posting data, that can be messy. I would suggest the sessions, so set like "return_page" to be each page, then just redirect back to that page on success using header I even think that $_SERVER['HTTP_REFERRER'] might even store that information for you.
-
As far as I know there is no way to prevent that. However doing the redirect after data submital, should erase the post data. So even if they go back it should not duplicate the data. But the history deal, I do not think is possible. If it is, you would have to use JavaScript I am sure to accomplish that.
-
This is how you should do it, instead of 2 querys as stated. <?php if (isset($_COOKIE['ID_my_site'])) { $username = $_COOKIE['ID_my_site']; $sql = "UPDATE `users` SET `village` = 'Akatsuki' WHERE username = '$username' AND skill > 5000000"; mysql_query($sql) or die(mysql_error()); } As long as there is a username in the cookie that should work. (I would store the username in session as appose to a cookie but yea.)
-
I highly doubt this is a PHP issue, this is more related to CSS/HTML. php does not have anything to do with the client side other than providing data.
-
$target_path = $target_path . basename( $_FILES['uploadedfile']['name']); I think that should be: $target_path = $target_path . basename( $_FILES['uploadedfile']['tmp_name']);
-
<?php $logged_string = $_SERVER['REMOTE_ADDR'] . "|" . date("j M Y g:i a"); $file = fopen("userIP.log", "a"); fputs($file, $logged_string, strlen($logged_string)); fclose($file); ?> That is the proper usage, $REMOTE_ADDR assumes register_globals is on, which is should be off due to security risks. Use $_SERVER to access it instead. As far as that, it should capture and IP just fine and write it to a log file, or if you have a database make a field called IP and save it to the database.
-
[SOLVED] Separating a predefined date that is stored in a database?
premiso replied to newbreed65's topic in PHP Coding Help
First question. Where are you pulling the dates out of the database in that script? As far as storing dates in a database, store them as the actual date and don't do 3 separate fields. It is easier to modify a date as a timestamp than as 3 separate fields. As mentioned above you would use either the explode and strtotime functions to separate your date into the sections you want, day/month/year and then set it much like you have done with the $_POST, except with the data from the database. -
they may work...but they violate XHTML/CSS standards To add to that, using a browser that conforms to standards, such as FireFox, this (I think this is true) will display different results due to the ID. IE would probably display it correctly since it violates the standards. Best coding practices is to conform to the standards, even though it "may work" later down the line it may not work.
-
[SOLVED] copy id from one database to another database
premiso replied to mikeabe's topic in PHP Coding Help
That code should work, what you have to worry about is someone with the same name, such as "John Smith", this will set every student with the name "john smith" to the last id done. Look at the ones that are not updating, check that there is not an extra space or something in the name. If you use phpMyAdmin, maybe do a select on both the databases using the logic you have above and see what pulls out and why. -
Not sure if REQUEST_URI will do it. But if it will. <p>I'm sorry <strong>http://www.mywebsite.com/<?php echo $_SERVER['REQUEST_URI']; ?></strong> cannot be found. That should do it.
-
Are you sure? Is this guy wrong then? http://bytes.com/groups/php/10541-session-gc_maxlifetime Think about it, what good would sessions do if you can only stay on a site max 20 minutes period and you have to re-login after 20 minutes. The activity re-activates the session, look at banking sites etc. As long as you do something within the 20 minutes you stay logged in. Once you do not you are automatically logged out cause the session timed out. Think about the word "timed out" itself. A session timeout means that it did not receive a response in x amount of time, thus it timesout, if you send something to the server you essentially sent it a "ping" and told it you are still here, so the timer resets to 20 minutes. But as stated above, try it out for yourself, the only true way to know is testing it on your own.
-
The idea behind SEO is to have keywords part of the URL. What I usually do is take something unique, such as the artist name, and convert it to an seo url and store this in the DB to access it. So let's say you have something like "Jack's High End Band" for an artitst title. I would create something to make it "jacks-high-end-band" Then create the url http://yoursite.com/artist/jacks-high-end-band Then you just select * from tbl where seo_artist = 'jacks-high-end-band'; So basically when an artist is inserted you have a function in your code to convert the artist to seoFriendlyArtist and return a string like the above. Anyhow just figured I would throw that out there incase you were interested =) If you would like a function that converts stuff to seofriendly urls let me know and I will clean up my function and post it here. Wait a minute....I thought the apache server converts the url into an easy to read url. I thought you just have to put the rules in a htaccess file? It does, but if you want SEO friendly URLS to help boost your seo ranking, than you want to have the artist in the url. It is the same setup, except on the artist.php page you check the returned get data against an "Seo_artist" field in the DB. If you do not care about that, artist/id will work just fine for you.
-
More of a server question as appose to PHP. I am sure it is possible, most likely use a cron job to copy files from server a to server b every x minutes. As far as the database connection to both sites, that would tie up a bunch of space, probably be better to do a script that does a mysql dump every 6 hours or something like that then upload it to the other site and insert that data via command line. But yea, it is alot of work and could possibly take a tole on your server having them clone each other. For the database you could possibly create a file that connects to that servers DB and each time a query is ran that inserts/updates on your site write it to a log and the script runs that log via cron every x minutes to push the updates to the other server.
-
<?php $res = mysql_query("SELECT * FROM message_table"); if (mysql_num_rows($res) > 0) { echo 'Display bar here'; } That is probably what you are looking for.
-
SELECT * FROM message_table WHERE x=x LIMIT 1 That should pull out the last one entered by default, replace the x=x if you had a where statement there before and of course the message_table part with your table name. If that does not work, try this one: SELECT * FROM message_table WHERE x=x order by ID desc LIMIT 1 where ID is the unique table identifier. (Primary Key) EDIT: As for the table structure, you should at least have a date and a primary key column broadcastid int NOT NULL auto_increment broadcastmessage blob null broadcasttime timestamp NOT NULL default CURRENT_TIMESTAMP (you may need to look this up) Then order it by timestamp or id, whichever.
-
The session will last as long as there is activity. So if session_start() is called anywhere in that 30 minutes it is extended by another 30 minutes. No need to regenerate the id.
-
[SOLVED] Use array values as function parameters
premiso replied to blogical's topic in PHP Coding Help
call_user_func I think that is the function you are looking for... -
If they kept backups, then they would be able to help you. Chances are they did not if you did not pay extra for it. The short answer is no. Since you overwritten the file (I am sure) it does not act the same as delete and moves it to a trash bin. My bet is it is lost for good.
-
Just curious have you tried str_replace also? As I believe trim only removes it from the end or beginning of the string (not sure of this though).
-
The idea behind SEO is to have keywords part of the URL. What I usually do is take something unique, such as the artist name, and convert it to an seo url and store this in the DB to access it. So let's say you have something like "Jack's High End Band" for an artitst title. I would create something to make it "jacks-high-end-band" Then create the url http://yoursite.com/artist/jacks-high-end-band Then you just select * from tbl where seo_artist = 'jacks-high-end-band'; So basically when an artist is inserted you have a function in your code to convert the artist to seoFriendlyArtist and return a string like the above. Anyhow just figured I would throw that out there incase you were interested =) If you would like a function that converts stuff to seofriendly urls let me know and I will clean up my function and post it here.
-
If you want to avoid this next time I would suggest setting up a local SVN server on your machine. This will allow you to keep backups etc each time you change the file. (SVN - SubVersion Control) Tortoise SVN is a free and great SVN tool.
-
Are you sure it is only a "\n" and not a "\r\n" or vice versa? $key_trim = trim($keyword_array[$count_row], "\r\n"); I think that would/should work if that is the case.