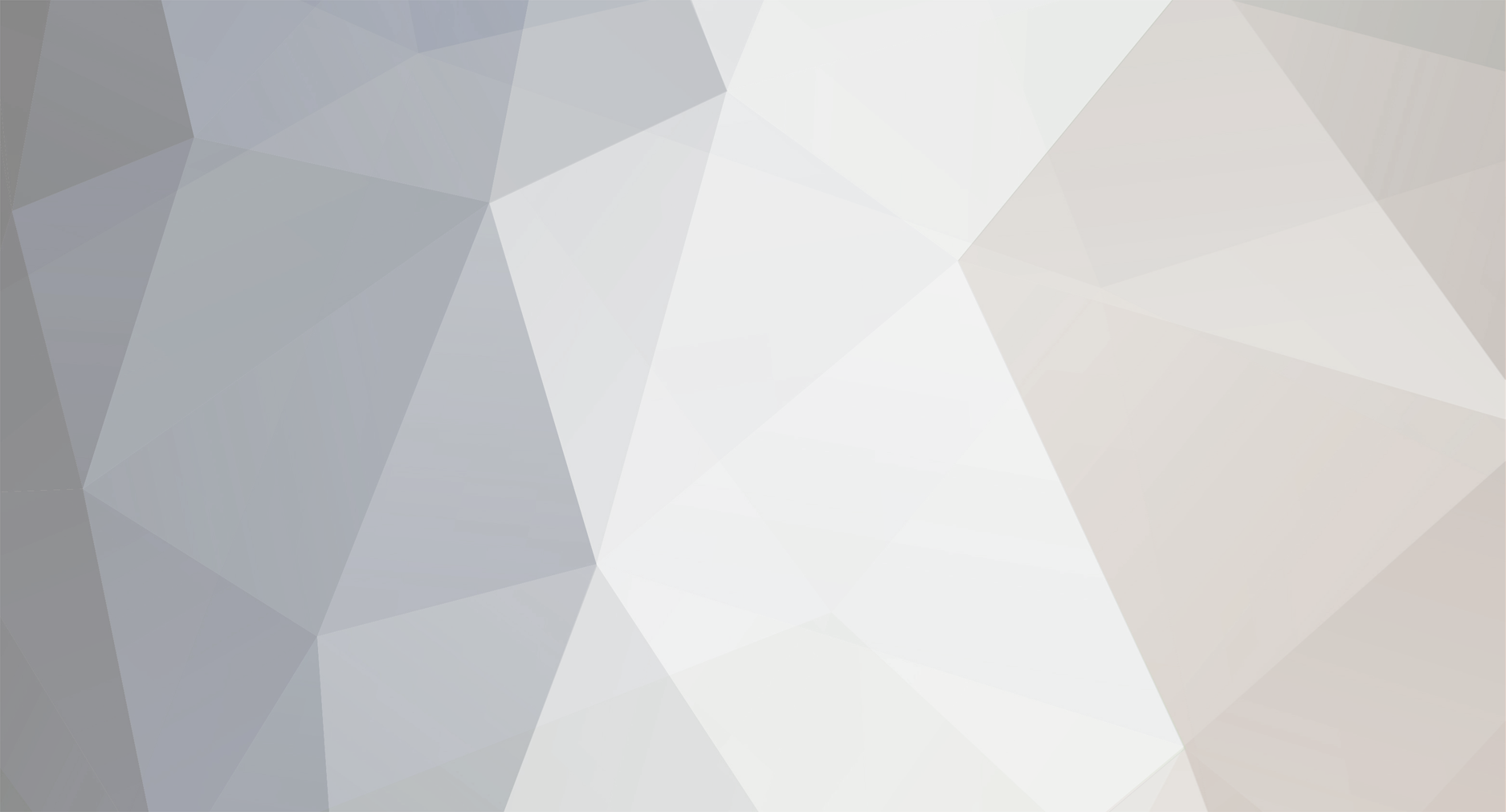
irkevin
-
Posts
295 -
Joined
-
Last visited
Posts posted by irkevin
-
-
well the point it,
I have a config.php page where some variable are set for the website.
If one day i have to change something in this config.php page (example: change $allow = 'no' TO $allow = 'yes'), instead of doing it manually in the file, i would like to do it via a form! Hope that makes sense. lol
-
Bump, No one ever tried this?
-
Well, but i need to write the file and update whatever value is assign to the variable
So if $host = 'somehost', i would like to change it to $host = 'anotherhost' with a form!
-
Hi,
I need some help and some advice on how i should proceed with this.
Let's say i have config.php with those variables:
<?php $host = "localhost"; $user = "username"; $pass = "pass"; $db = "db_name"; $allow = "no"; ?>
I will have a form that will list each of those values in a text input. Now, is it possible to update the values with this form? If i have to set $allow = "yes", will it be possible using a form?
Please give some advice! I searched this forum but didn't get a clue on how to achieve this!
Thanks, any advice is welcome.
Have a nice Day!
-
Just a suggestion.
Try to put this
session_start();
On top of your codes. Just after the opening php tag like this:
<?php session_start(); //the rest of your codes ?>
-
can you post a link to it?
-
I think you're right. I was just googling to see a better way of writing oop and and someone did the same thing as i did, needless to say, he got the same comment. lol.
Anyways, do you have a good place to start learning oop?
-
This is just a basic test since i'm still learning oop..
-
You should create a table for active users and active guests.. Have a look at the code below, this is something i used ..
<?php include('config.php'); // absolute path to your config page $query = "SELECT * FROM users"; $result = mysql_query($query); $u = mysql_num_rows($result); if(!$u) { echo 'No data available'; } else { $time = time(); // set the time $time_out = 300; // timeout $guest_time_out = $time-300; // timeout set to second $member = $_SESSION['username']; // seting a variable for the session $ip = $_SERVER['REMOTE_ADDR']; // variable for the ip adresss // this will check if the current guy is a guest or a logged user //if he is not logged user execute these code if(empty($member)){ // select from the active guest table to see if there is a record //if there is a record, we update the row else we insert the ip in our table $sql = "SELECT * FROM active_guests WHERE ip = '$ip'"; $result = mysql_query($sql,$link); $num = mysql_num_rows($result); //if now rows was found, insert our guest in the active_guests table if($num == 0) { $q = "INSERT INTO active_guests(ip,timestamp)VALUES('$ip','$time')"; $res = mysql_query($q); } else { //if the ip was already there, we update the row $q = "UPDATE active_guests SET timestamp = '$time' WHERE ip = '$ip'"; $res = mysql_query($q); } } else{ //if the user is logged in, we delete the ip of the member in the active guest table so the logged //doing so, the logged in user won't be consided as a guest anymore $del = "DELETE FROM active_guests WHERE ip = '$ip'"; $delresult = mysql_query($del); //now we'll check if the user was already there $sql = "SELECT * FROM active_users WHERE username = '$member'"; $result = mysql_query($sql); $num = mysql_num_rows($result); //if the user was already there,we'll update else we'll insert //so if no rows was found, we'll surely want to add him. right?? if($num == 0) { $sql = "INSERT INTO active_users(username,timestamp)VALUES('$member','$time')"; $result = mysql_query($sql); }else{ // else don't let him go, update the row for him $sql = "UPDATE active_users SET timestamp = '$time' WHERE username = '$member'"; $result = mysql_query($sql); } } // Note that $guest_time_out is the number of seconds a guest is considered as guest $sql4="DELETE FROM active_guests WHERE timestamp < $guest_time_out"; $result4=mysql_query($sql4) or die (mysql_error()); //grab result for visitors or guest $cnx = "SELECT * FROM active_guests"; $resultat = mysql_query($cnx); $visitors = mysql_num_rows($resultat); if($visitors == 1 || $visitors == 0) { echo "<br />Active Guest : <span style='font-weight:bold;'>". $visitors ."</span><br />"; } else { echo "<br />Active Guest(s) : <span style='font-weight:bold;'>". $visitors ."</span> <br />"; } //grab result for our logged in users $grab = "SELECT * FROM active_users"; $result = mysql_query($grab); $count = mysql_num_rows($result); if($count == 1) { echo "Member Online : <span style='font-weight:bold;'>".$count. "</span><br />"; } else { echo "Member(s) Online : <span style='font-weight:bold;'>".$count. "</span><br />"; } } ?>
It is commented everywhere, so you should find your way around
-
Hmm nothing?
-
Array ( [0] => Title number 2 [1] => Content Number 2<br /> [2] => Title number 1 [3] => Content number 1<br /> )
This is what I get
-
Hmm i see.
But now the only thing that bugs me is
When i get the result.. they come like this
Title number 2
Content Number 2
Title number 1
Content number 1
In the page source code, it's like this:
span class="list">Title number 2 </span><br /> <span class="list">Content Number 2<br /></span><br /> <span class="list">Title number 1</span><br /> <span class="list">Content number 1<br /></span><br />
As you can see, ebery span has the same class, since i'm echoing the variable $data in my foreach. Is there anyway I can echo out the title with it's own span and the content with it's own span?
-
I heard it depends on which mail you are using. Some web host mail doesn't accept html..
It might work in hotmail !
-
Hey good timing. lol.. I don't even know why I wrote it in lowercase. I always do it the uppercase way.. Anyways I have one more question.
Is it ok to echo out stuff directly from the class file?
Lets say i want to echo out the number of rows I have. I would I proceed with that?
-
Hi guys,
I'm new to OOP and i would be grateful if someone could review the simple and basic code i wrote and tell me if the technique is good or if i can better it.
What I did was a simple class file call database.php. It basically select some stuff from mysql.. and in my index.php, i've include the class file and spit out all the data.
Below are the codes
database.php
<?php class database{ private $mysql; function __construct(){ $this->mysql = new mysqli('localhost','root','','data'); } //a function to select our stuff function select_query($sql){ if(($result = $this->mysql->query($sql)) != NULL){ while($row = $result->fetch_object()){ $content[] = $row->title; $content[] .= $row->content.'<br />'; } }else{ echo $this->mysql->error; } return $content; } function __destruct(){ $this->mysql->close; } } ?>
index.php
<?php require 'database.php'; $database = new database(); $myData = $database->select_query('select * from contents order by id asc'); ?> <?php foreach($myData as $data): ?> <span class="list"><?php echo $data;?></span><br /> <?php endforeach;?>
Any advice is welcome. Thanks!
-
PFMaBiSmAd got a good point.
short php tags doesn't work quite well if it's not defined in php.ini if im correct and because of this, you'll smash your forehead on your keyboard wondering whats going on!
and btw, Wampserver seems to work well.
-
the semicolumn should be after
$name = stripslashes($_POST['Name']); $email = stripslashes($_POST['Email']); $comments = stripslashes($_POST['Comments']);
-
wow i never knew that stipslashes depends on magic_quotes_gpc :s ... in this case, i believe most servers have this on by default.. If im not mistaken..
-
wouldnt this be fine using strislashes?
-
i might be wrong, but you can also pass php in Javscript..
I did something like this recently
document.getElementById("slideshow").setAttribute("src", "<?php echo $chapter;?>/"+galleryarray[curimg])
-
can you post the actual code you have plz?
-
Here's what i've been looking for
http://www.mu-anime.com/manga_folder.php
Looks like i'm almost done with it..
here is the code
<?php /*####################################################### * * THIS BLOCK OF CODE IS TO FETCH THE FIRST FOLDERS * AND OUTPUT THEM IN OUR DROP DOWN MENU * *********************************************************/ $path = "manga/"; $narray = array(); if ($dir_handle = @opendir($path)) { $i = 0; while (($file = readdir($dir_handle)) !== false) { if ($file != '.' && $file != '..') { $narray[$i] = $file; $i++; } }// end while sort($narray); for($i=0; $i < sizeof($narray); $i++) { $folder = $_GET['folder']; if($folder == $path.$narray[$i]) { $output .= '<option value="manga_folder.php?folder='.$path.$narray[$i].'" selected="selected">'. $narray[$i]. '</option>'; } else { $output .= '<option value="manga_folder.php?folder='.$path.$narray[$i].'">'.$narray[$i].'</option>'; } }// end for loop closedir($dir_handle); } // end dir handle /*####################################################### * * THIS BLOCK OF CODE IS TO FETCH THE SUB FOLDERS * AND OUTPUT THEM IN OUR SECOND DROP DOWN MENU * *********************************************************/ $folder = $_GET['folder']; $thepath = $folder; if ($the_handle = @opendir($thepath)) { while (($thefile = readdir($the_handle)) !== false) { if ($thefile != '.' && $thefile != '..') { if($_GET['chapter'] == $thefile) { $list .= '<option value="manga_folder.php?folder='.$thepath.'&chapter='.$thefile.'" selected="selected">'.$thefile. '</option>'; } else { $list .= '<option value="manga_folder.php?folder='.$thepath.'&chapter='.$thefile.'"> '.$thefile. '</option>'; } } } closedir($the_handle); } /*####################################################### * * THIS BLOCK OF CODE IS TO READ ALL THE FILES * AND OUTPUT THEM AS PICTURE TO READ * *********************************************************/ $chapter = $_GET['folder'].'/'.$_GET['chapter']; $carray = array(); if(isset($chapter)) { if ($the_chapter = @opendir($chapter)) { $i = 0; while (($TheChapter = readdir($the_chapter)) !== false) { if ($TheChapter != '.' && $TheChapter != '..') { $carray[$i] = $TheChapter; $i++; } }// end while sort($carray); for($i = 0; $i < sizeof($carray); $i++) { if(isset($_GET['chapter'])) { $page .= '<option value="'.$chapter.'/'.$carray[$i].'"> Page No:'.$i.'</option>'; } else { $page .= '<option value="'.$chapter.'/'.$carray[$i].'"></option>'; } $gallery .= 'galleryarray['.$i.']="'.$carray[$i].'";'; $jsarray = 'var galleryarray=new Array();'; } closedir($the_chapter); }// end handle } ?>
I know it might look messy to you guys
I'm only good with php and mysql.. and it the first time i've been able to manage something with folders, subfolders and files..
Below is the javascript to get the dropdown to go to the selected folders and Files..
<script type="text/javascript"> <?php echo $jsarray;?> <?php echo $gallery;?> var curimg = 0 function rotateimages() { document.getElementById("slideshow").setAttribute("src", "<?php echo $chapter;?>/"+galleryarray[curimg]) document.getElementById('page').options[curimg+1].setAttribute('selected','selected'); if(curimg < galleryarray.length-1) { curimg = curimg+1; } else { curimg = 0; document.getElementById("slideshow").style.cursor = 'default'; } } function selectManga() { var folder = document.getElementById('choose'); for(i=0;i < folder.selectedIndex; i++) { if(folder.selectedIndex) { document.location = folder.value; } } } function selectChapter() { var chapter = document.getElementById('chapters'); for(i=0; i < chapter.selectedIndex; i++) { if(chapter.selectedIndex) { document.location = chapter.value; } } } function changePage() { var MyPage = document.getElementById('page'); for(i=0; i < MyPage.selectedIndex; i++) { if(MyPage.selectedIndex) { document.getElementById('slideshow').src = MyPage.value; } } } </script>
Oh, if you have some modification that would make the script better, do share plz ..
-
Ok man and thanks for your help
i'll be waiting
-
no one to help?
[SOLVED] update variables in a .php page with a form
in PHP Coding Help
Posted
If i have an Admin Backend, it will then be easier and faster to change a configuration instead of doing it manually, then re upload the file! Anyways, i will try to sort things out!
Thanks for every suggestion