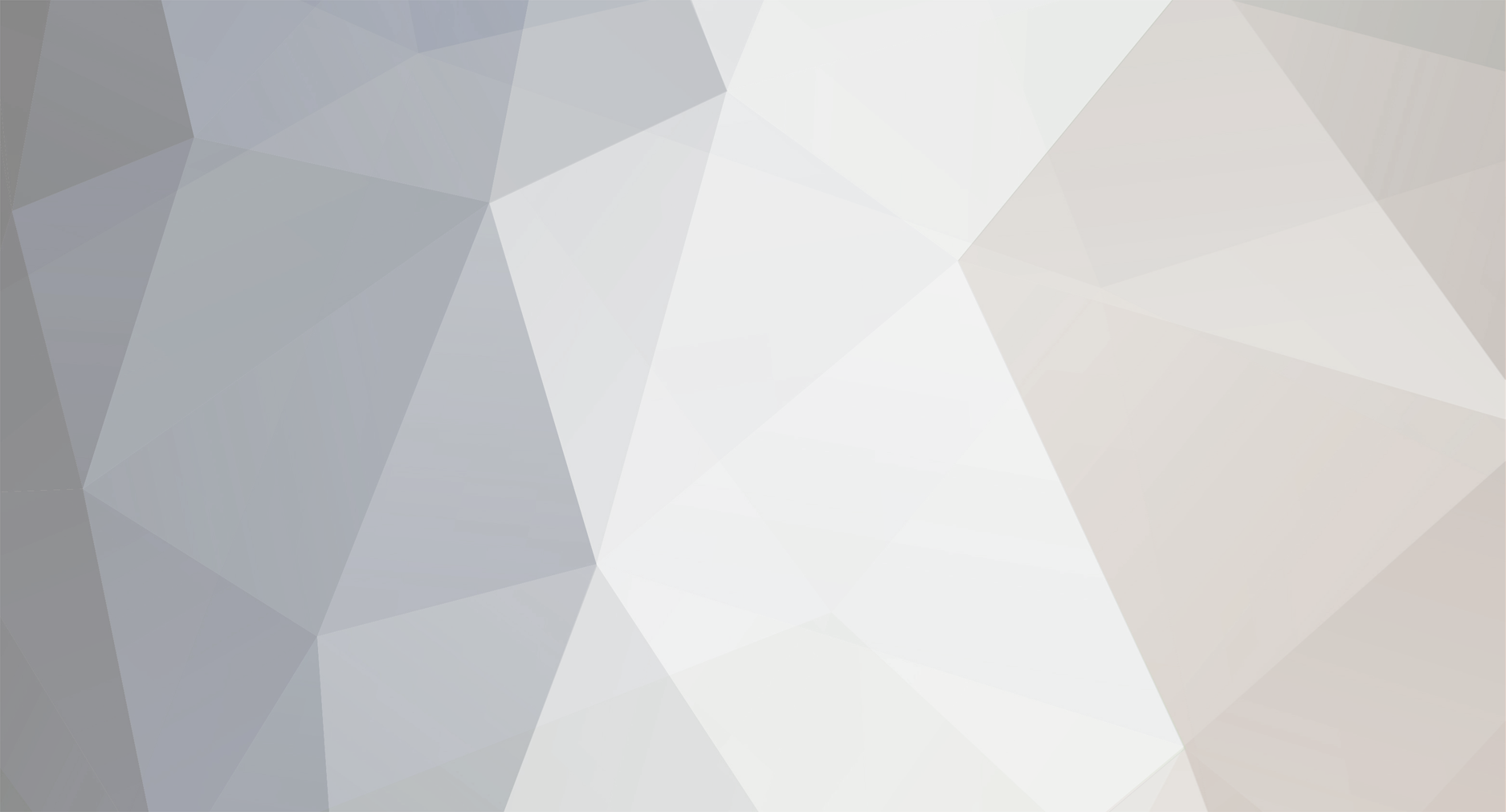
irkevin
-
Posts
295 -
Joined
-
Last visited
Posts posted by irkevin
-
-
ive noticed an extra semicolumn after the insert query
"INSERT INTO `b33_3176976_lunadb`.`DB_Guides` (`gid`, `uname`, `gname`, `icon`, `desc`, `cat`, `body`, `score`) VALUES (NULL, \'$_POST['name']\', \'$_POST['guidename']\', \'$_POST['icon']\', \'$_POST['description']\', \'$_POST['cat']\', \'$_POST['body']\', \'0\');";
try this
$con = mysql_connect("xxxxxxxxxxx","xxxxxxxx","xxxxxxxxxxx"); if (!$con) { die('Could not connect: ' . mysql_error()); }else{ echo "Connected to DB"; } $sql = "INSERT INTO `b33_3176976_lunadb`.`DB_Guides` (`gid`, `uname`, `gname`, `icon`, `desc`, `cat`, `body`, `score`) VALUES (NULL, \'$_POST['name']\', \'$_POST['guidename']\', \'$_POST['icon']\', \'$_POST['description']\', \'$_POST['cat']\', \'$_POST['body']\', \'0\')"; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } echo "1 record added"; mysql_close($con);
-
i noticed something..
if my link is like this -> index.php?action=register
when i change return BASE_URL.$match[1].'/'.$extra;
to return BASE_URL.$extra;
it worked welll....
but if my link is like this
http://www.mysite.com/page.php
it doesn't work.. i get only the domain name of my site without the page.php
-
i've been able to remove /page/ by changing this:
$extra .= $paramEx[0].'/'.$paramEx[1].'/';
to this:
$extra .= $paramEx[1].'/';
but still, the index is here.. dont have a clue how to remove it.. i should use an if statement maybe but dont know how
-
thnx for the reply but there is no variable $string in the script, so which variable should i pass instead of $string? Any Idea?
-
Hi
I have a code that will convert my Dynamic urls to static.. I've already used mod_rewrite which is ok but now have to use php to convert them according to the rewrited one.
However with php, instead of having this:
http://www.mu-anime.com/page/OngoingAnime/
I'm having this:
http://www.mu-anime.com/index/page/OngoingAnime/
The 'index' is to much there and i dont have a clue how to remove it. Below are the relevant codes
init.php
define('BASE_URL', 'http://www.mu-anime.com/'); // this you must use as an absolute path to the application root, ex: /folder1/folder2/ if (isset($_SERVER['PATH_INFO'])) { $url = substr($_SERVER['PATH_INFO'], 1); $urlParts = explode('/', $url); if ($urlParts[count($urlParts) - 1] == '') array_pop($urlParts); $urlPartsCount = count($urlParts); if ($urlPartsCount % 2 != 0) { $urlPartsCount++; } for ($i = 0; $i < $urlPartsCount; $i += 2) { $_GET[$urlParts[$i]] = $urlParts[$i + 1]; } } $urlPatterns = array( '~'.preg_quote(BASE_URL).'([^\.]+[0-9a-zA-Z]+).php(\?([0-9a-zA-Z]+[^#"\']*))?~i', ); ob_start();
deinit.php
function urlRewriteCallback($match) { $extra = ''; if ($match[3]) { $params = explode('&', $match[3]); if ($params[0] == '') array_shift($params); foreach ($params as $param) { $paramEx = explode('=', $param); $extra .= $paramEx[0].'/'.$paramEx[1].'/'; } } return BASE_URL.$match[1].'/'.$extra; } $pageContents = ob_get_contents(); ob_end_clean(); echo preg_replace_callback($urlPatterns, 'urlRewriteCallback', $pageContents);
Someone help me with this please
-
Well, there might ber some sort of solution, but that should give you an idea..
<?php $my_pages = array('home', 'music', 'stuffs', 'aboutus'); // check that $_GET['page'] is present, if its not we'll setup a default page if(isset($_GET['page'])) && !empty($_GET['page'])) { $requested_page = strtolower($_GET['page']); } else // $_GET['page'] not found or is empty, so we'll setup a default page to be requested { $requested_page = 'home'; } // setup an array of all your sites pages $my_pages = array('home', 'music', 'stuffs', 'aboutus'); $requested_page = strtolower($_GET['page']); // make sue the request page is in $my_Pages array if(in_array($requested_page, $my_pages)) { // the requested page will be included from a folder called my_pages in your websites root folder. // I assume the files to be included are html files include $_SERVER['DOCUMENT_ROOT'] . '/my_pages/' . $requested_page . 'html'; } else { // requested_page not found die( 'The requested page (' . $requested_page ') was not found!' ); } ?>
-
you might want to try this
$page = $_GET['page']; if(isset($page)) { switch($page) { case 'home': echo "this is home"; break; case 'page2': echo "this is page2"; break; case 'page3': echo "this is page3"; break; } } else { echo "this is home page which is the default"; } <a href="index.php">home</a> <a href="index.php?page=page2">Page 2</a> <a href="index.php?page=page3">Page 3</a>
try this and let me know
-
i was always wondering..
is php5 compatible on earlier version of php?
-
can u show how ur table is structured?
-
well i think there's a lot of factor to consider. if your server supports php5, what's the use?
-
not sure it will work
but try to return the row variable
<?php function get_user_name_from_db($user_id) { $qry = "SELECT * FROM user_tbl where user_id = '$user_id' "; $result = mysql_query( $qry ) or die(mysql_error()); $row = mysql_fetch_assoc($result)or die (mysql_error()); $user_name = $row['last_name'] . ", " . $row['first_name']; return $row; } ?>
i dont guarantee this. it's been a while since i didnt touch php
-
are you using a form for that?
-
first why are you passing the variable bewteen quotes?
foreach ($sqlbkups as $sqlbkup) { echo "$sqlbkup"; // why is this between quotes? huh. }
what is on line 21?
-
why dont you just use
if ($checkresult){ echo "<br /><br /><font size='5pt' face='comic sans ms'><b>Your horse has been retired.</b></font>"; } else { echo "<BR><BR><font size='5pt' face='comic sans ms'><B>You do not own this horse or the horse is not old enough to be retired.</b></font>"; }
-
i don't see $horseid anywhere in your script.. if it's from the link, you should add something like this maybe
$horseid = $_GET['horseid'];
-
can you give a link to see what you are doing?
this should have been good
<?php $word="hello i am reddarrow"; $test=str_replace(" "," ",$word); echo $test; ?>
-
still no one :S
-
bump..
no one to help?
-
ob_start..? well i dont think it would work with that..
any more suggestion?
i heard it could be use with preg_replace, don't know if it's true.. hope someone has the answer to this
-
thars correct.. but i also need to put them that way on my website if i want users to navigate mu site using static urls.. how do i do this when having stuff coming from database?
there must be a way to do this in php also.
-
Bump ..no one?
-
Well, what do you recommend to use for doing this?
I'm trying to make my links more friendly for google.. SEF i supose.
I've been able to use htaccess to convert the links from dynamic to static.. but now, i have to convert them on my website also so that people can access the static one. .
Do you get it now?
-
Hi everyone,
I need some help to understand how preg_replace work.. Right now, i have a link which is dynamic, like so
http://www.mu-anime.com/index.php?page=CompletedSeries&id=1
I want to use preg_replace to convert it like so
http://www.mu-anime.com/completed/1.html
I used .htaccess, but now i have to convert the link on my website also and have no idea how to start .. Any help plz?
-
You might want to use javascript for that.
Just google for Javascript DropDown Navigation
[SOLVED] help with replace dynamic url into static url
in Regex Help
Posted
Fixed
here's how i did it.. again it was a str_replace
on deinit.php: