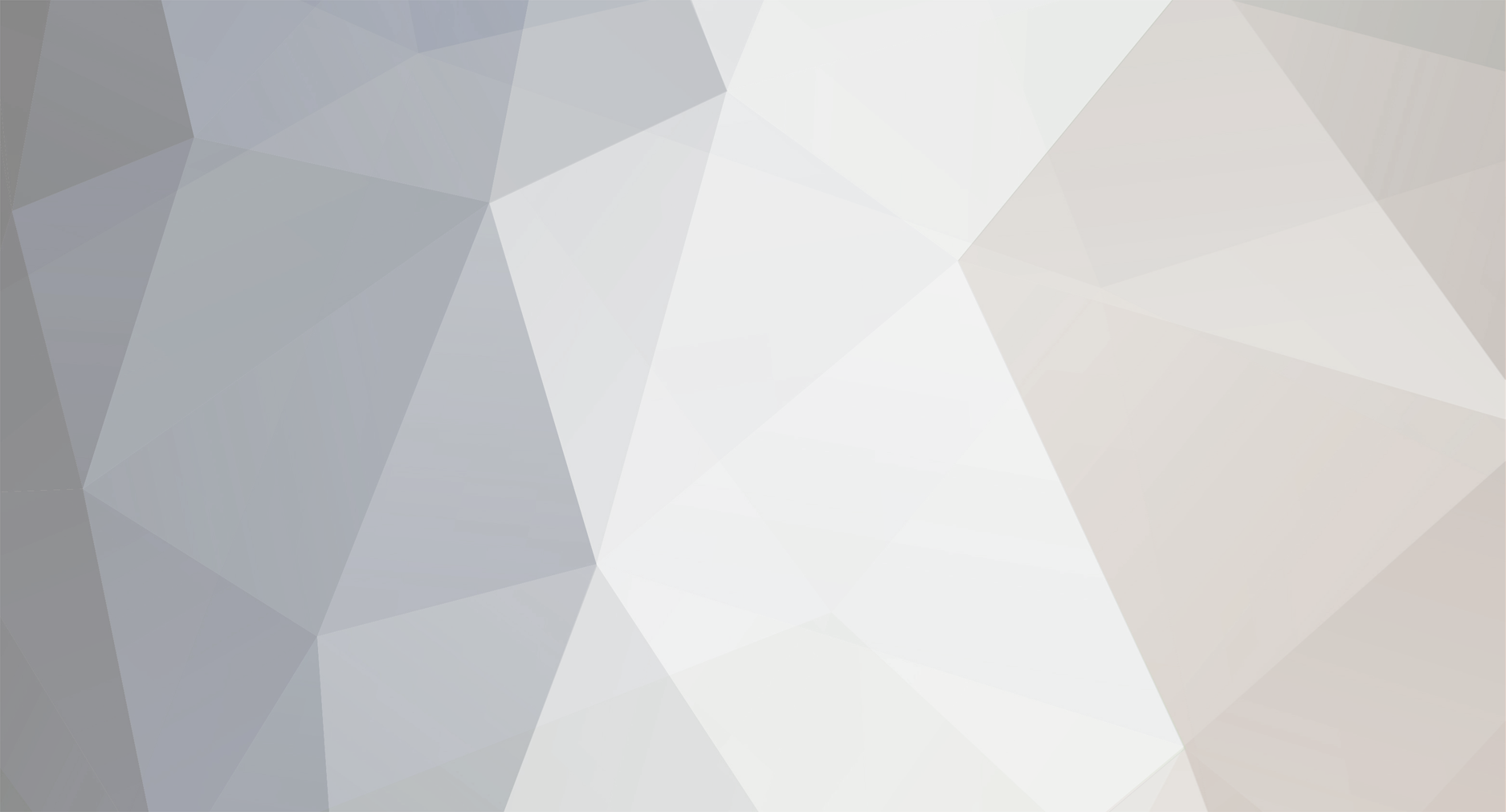
effigy
-
Posts
3,600 -
Joined
-
Last visited
Never
Posts posted by effigy
-
-
$sql = preg_replace("/'(\d+)'/", '$1', $sql);
-
You have a laziness/greediness issue.
-
?! means does not follow.
?= means follows.
?!=, what you have, means does not follow, then a literal =.
-
-
What encoding are you using?
-
-
/(\.|-|\/)+/
Why are you using this and not a character class? This kind of alternation is not optimal.
-
I think it's best to include the + since it doesn't hurt. If the data is coming from a user they could have a typo—"02/27//2009" for instance—which, when split, would create 4 elements rather than 3. However, if there are any delimiters on the ends, this would still create empty elements. I recommend:
preg_split('/\D+/', $string, -1, PREG_SPLIT_NO_EMPTY)
-
You can split on \D+.
-
file_get_contents should be fine. Most editors do; I use Notepad++.
-
<pre> <?php $tests = array( 'BBB', 'BBB; BBB', 'BBB; BBB; BBB; BBB', 'B;', ';B', ';;', ); foreach ($tests as $test) { echo "$test => "; echo preg_match('/\A([^;]+;\s*)*[^;]+\z/', $test) ? 'OK' : 'Not OK' ; echo '<br>'; } ?> </pre>
-
<pre> <?php $data = <<<DATA HOW TO WATER ROSES Start by turning the water on.... HOW TO MOW Start the mower... DATA; print_r(preg_split('/(?:\r?\n)+/', $data)); ?> </pre>
-
You need to use the /m modifier if you want $ to match around all new lines, rather than just the end of the string.
-
/\Apub-\d{16}\z/
-
What's the value of $unicode?
This gives me "réréré":
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8"> <pre> <?php $text = 'r%C3%A9r%C3%A9r%C3%A9'; echo urldecode($text); ?> </pre>
-
"Suddenly"? Perhaps your input and/or code changed? Have you examined the data fully? A hex dump, perhaps?
-
You've bad Unicode. See "Replacement character" here.
-
Your first .*? will capture anything up to prodID, even it has to go beyond another double quote or into another tag.
Try |href="([^"]+prodID=[^"]+)"[^>]*>(.*?)</a>|.
-
Are all of these Kanji? You can use their code point ranges to match them, e.g. preg_match_all('/[\x{3000}-\x{303F}]+/u', $string, $matches);.
-
-
Turn your warnings on: Warning: preg_match_all() expects parameter 2 to be string, array given.
You need to examine the results; I recommend print_r($byname);
-
use lazyiness
'/href="(.*?)"/i'
Even better, be specific: '/href="([^"]*)"/i'
in the regex above, you are taking into consideration escaped quotes
The double quotes were escaped in the regex because they were used to delimit the string; they will match an unescaped quote:
<pre> <?php $str = '"'; echo preg_match("/\"/", $str); ?> </pre>
-
Apparently it requires the delimiter:
preg_match('/' . preg_quote($start, '/') . '(.*?)' . preg_quote($end, '/') . '/', $data, $matches);
-
/(\b(?!(?:else)?if)\S+\b\s*\()/
[SOLVED] preg_match_all returns only one match
in Regex Help
Posted
Regular expressions are not the best tool for nested data, but this works: