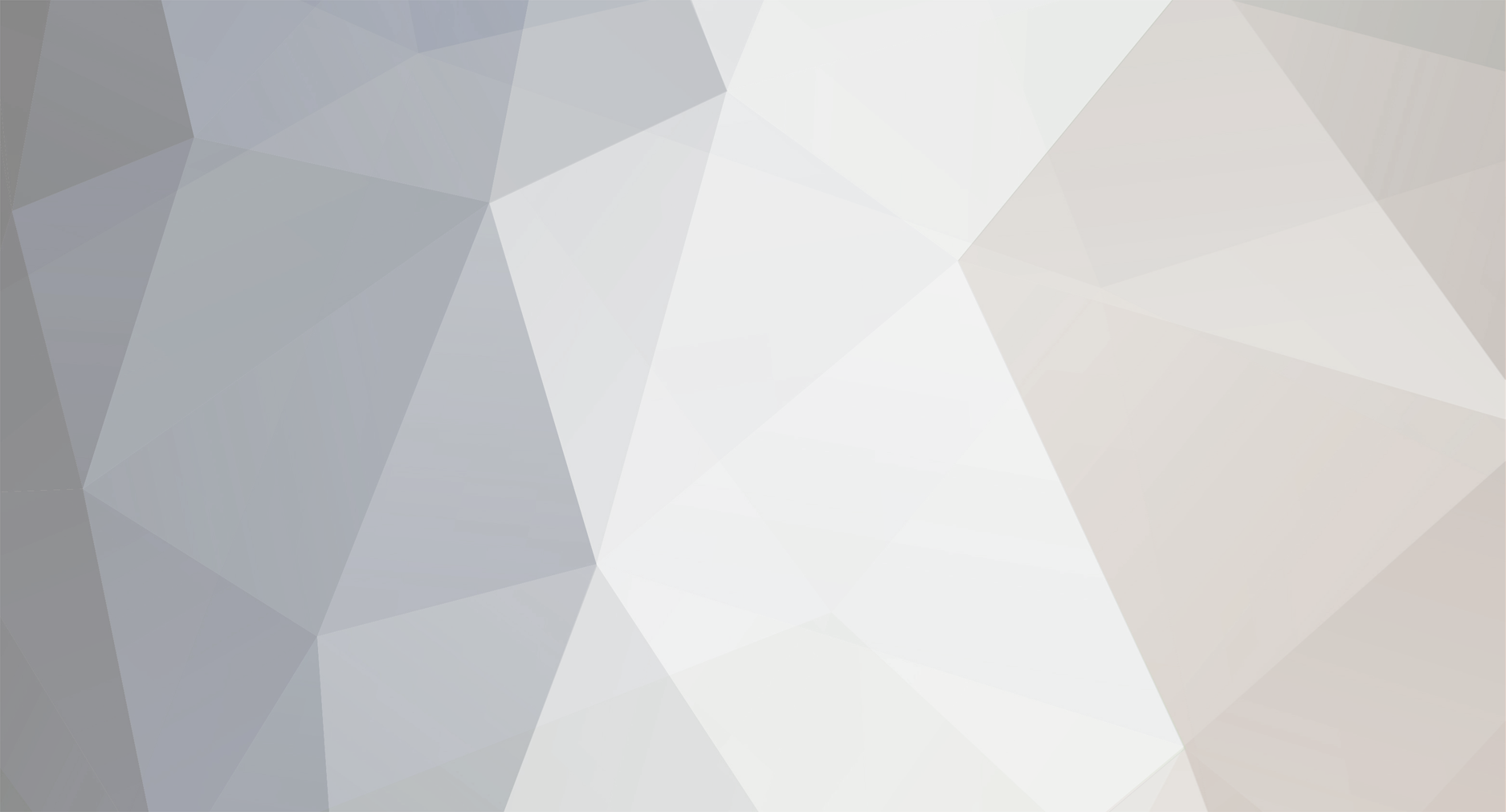
effigy
-
Posts
3,600 -
Joined
-
Last visited
Never
Posts posted by effigy
-
-
Do you want your original XML to remain exactly the same, aside from the sorting factor? In XSLT you can control the format--it can be whatever you want. If you want to leave the original XML structure the same, but make a few changes, you use an identity transform.
-
-
<pre> <?php $start = '<td class="object_desc" dir=rtl align=right valign=top background="iaasds/jxx05.gif" width="554" height="67" style="padding-right:20px;padding-left:35px;">'; $end = '</td>'; preg_match('/' . preg_quote($start) . '(.*?)' . preg_quote($end) . '/', $data, $matches); print_r($matches); ?> </pre>
-
Example #1 Using the xslt_process() to transform an XML file and a XSL file to a new XML file
Example #2 Using the xslt_process() to transform an XML file and a XSL file to a variable containing the resulting XML data
-
-
Have you tried XSLT?
-
You've already taken care of the thanks part. Clicking the "TOPIC SOLVED" button in the bottom left is all that's left.
-
<pre> <?php $data = <<<DATA (great,cool) site, I (like,enjoyed) it i love (apples,oranges,bananas,grapes,tangerines,cake,pie) DATA; echo preg_replace_callback( '/\((.*?)\)/', create_function( '$words', '$words = split(",", $words[1]); return array_rand(array_flip($words));' ), $data ); ?> </pre>
-
<pre> <?php $data = <<<DATA Great site, I like it Cool site, I like it DATA; print_r(preg_split('/site,\s+I\s+|\s+it(?:\s+|\z)/', $data, -1, PREG_SPLIT_NO_EMPTY)); ?> </pre>
-
Without the proper tools, nesting is always an issue. What if the tag has data, do you want to close it rather than remove it?
-
How about tidy?
-
On second thought...
<pre> <?php $str = <<<STR <div class="s_ach_stat">10<img src="../images/achievements/tiny_shield.gif"> (01-13-2009) </div> <span>Explore Storm Peaks</span><span class="achv_desc">Explore Storm Peaks, revealing the covered areas of the world map.</span> STR; $pieces = preg_split('#[()]|\s*<[^>]*>\s*#', $str, -1, PREG_SPLIT_NO_EMPTY); print_r($pieces); ?> </pre>
-
What was wrong with nrg's solution? Below is my simplification: the expression is condensed and the looping is by reference, making the appearance a little more kind.
<pre> <?php $str = <<<STR <div class="s_ach_stat">10<img src="../images/achievements/tiny_shield.gif"> (01-13-2009) </div> <span>Explore Storm Peaks</span><span class="achv_desc">Explore Storm Peaks, revealing the covered areas of the world map.</span> STR; $pieces = preg_split('#\s*<[^>]*>\s*#', $str, -1, PREG_SPLIT_NO_EMPTY); foreach ($pieces as &$piece) { $piece = trim($piece, '()'); } print_r($pieces); ?> </pre>
-
Why does PHP have some functions that have alternative aliases?
My guess is to accommodate those coming from other languages. For example, Perl uses join rather than implode, so I'd much rather use join across the board than remember the idiosyncrasies.
-
preg_split splits a string by expression, discarding the parts that it matches. This expression has two delimiters (//), a character class ([]), a quantifier (+), a shorthand (\s), and two literals (()).
The delimiters contain the expression; the character class matches any one character inside, but the plus quantifier instructs it to match 1 or more; and what we're asking it to match is either "(", ")", or any kind of whitespace (\s).
-
<pre> <?php $str = 'Nick Leigh(1)'; print_r(preg_split('/[()\s]+/', $str, -1, PREG_SPLIT_NO_EMPTY)); ?> </pre>
-
Does Google not have an API for this?
Try %<a[^>]+?href="http://[^"]+"[^>]+?class=l\s%. You can reduce the results with array_slice.
-
Try this:
<pre> <?php $data = <<<DATA <h1>Heading </h1> <pre>Pre </pre> <ul> <ol>A </ol> <ol>B </ol> </ul> <p>P </p> DATA; ### Separate the tags from the data. $pieces = preg_split('%(<[^>]*>)%', $data, -1, PREG_SPLIT_NO_EMPTY | PREG_SPLIT_DELIM_CAPTURE); foreach ($pieces as $piece) { echo htmlspecialchars($piece); } echo '<hr>'; ### Parse. $level = 0; foreach ($pieces as &$piece) { echo "($level) ", htmlspecialchars($piece); ### Determine nesting. if (substr($piece, 0, 1) == '<') { preg_match('%<(?:pre|[ou]l)[^>]*>%i', $piece) ? ++$level : null ; preg_match('%</(?:pre|[ou]l)>%i', $piece) ? --$level : null ; } ### If a level exists, trim trailing white space. elseif ($level) { $piece = rtrim($piece); } } unset($piece); echo '<hr>'; foreach ($pieces as $piece) { echo htmlspecialchars($piece); } ?> </pre>
-
This is an easy task, but nesting would complicate things.
Is there a lot of nesting?
How big are these documents?
-
$pieces = preg_split('/\s{2,}/', $data, -1, PREG_SPLIT_NO_EMPTY); echo join(',', $pieces);
-
Can you not incorporate that into your pattern?
-
This is only a basis as unpaired single quotes (apostrophes) may throw off the detection.
<pre> <?php ### $'s escaped for sake of example. $data = <<<DATA ##div('\$\$title or "\$\$title"'); // current results: '\$\$title or "\$view->title"' // expected results: '\$\$title or "\$\$title"' ##div("\$\$title or '\$\$title'"); // current results: "\$view->title or '\$view->title'" // expected results: "\$view->title or '\$view->title'" DATA; echo '<b>Original:</b><hr>'; echo $data; echo '<br><br><b>Split on Double Quote Pairs:</b><hr>'; $pieces = preg_split('/(".*?")/s', $data, -1, PREG_SPLIT_NO_EMPTY | PREG_SPLIT_DELIM_CAPTURE); print_r($pieces); echo '<br><br><b>Double Quote Replacement:</b><hr>'; $singles = 0; foreach ($pieces as &$piece) { $singles += substr_count($piece, "'"); ### No replacement should be made if: ### 1. The line does not start with a double quote; and ### 2. If the number of single quotes are not even (closed). if (!substr($piece, 0, 1) == '"' || $singles % 2) { continue; } $piece = preg_replace('/"(.*?)"/s', '"====="', $piece); } print_r($pieces); echo '<br><br><b>Reassembled:</b><hr>'; echo join('', $pieces); ?> </pre>
-
<pre> <?php $content = file_get_contents('http://www.bungie.net/stats/halo3/fileshare.aspx?gamertag=HaLo2FrEeEk'); $pieces = explode('<div class="shareTitle">', $content); foreach ($pieces as $piece) { if (!preg_match('%/images/halo3stats/fileshareiconssm/film(?:clip)?s/sm/%', $piece)) { continue; } preg_match('% fileshareitem_titleLink[^>]+? h3fileid=(?P<h3fileid>\d+) [^>]+> (?P<title>.+?) </a> .+? Film\s+Length:\s+ (?P<length>[\d:]+) %xis', $piece, $matches); $result = array(); foreach (array_keys($matches) as $key) { if (!is_numeric($key)) { array_push($result, $matches[$key]); } } print_r($result); } ?> </pre>
-
You may want to try some other tutorials via our resources.
These two paragraphs from your link cover character classes and quantifiers:
To specify a set of acceptable characters in your pattern, you can either build a character class yourself or use a predefined one. A character class lets you represent a bunch of characters as a single item in a regular expression. You can build your own character class by enclosing the acceptable characters in square brackets. A character class matches any one of the characters in the class. For example a character class [abc] matches a, b or c. To define a range of characters, just put the first and last characters in, separated by hyphen. For example, to match all alphanumeric characters: [a-zA-Z0-9]. You can also create a negated character class, which matches any character that is not in the class. To create a negated character class, begin the character class with ^: [^0-9].
The metacharacters +, *, ?, and {} affect the number of times a pattern should be matched. + means "Match one or more of the preceding expression", * means "Match zero or more of the preceding expression", and ? means "Match zero or one of the preceding expression". Curly braces {} can be used differently. With a single integer, {n} means "match exactly n occurrences of the preceding expression", with one integer and a comma, {n,} means "match n or more occurrences of the preceding expression", and with two comma-separated integers {n,m} means "match the previous character if it occurs at least n times, but no more than m times".
And here's a breakdown of the pattern:
NODE EXPLANATION
----------------------------------------------------------------------
( group and capture to \1:
----------------------------------------------------------------------
" '"'
----------------------------------------------------------------------
[^"]+ any character except: '"' (1 or more
times (matching the most amount
possible))
----------------------------------------------------------------------
" '"'
----------------------------------------------------------------------
) end of \1
----------------------------------------------------------------------
displaying items from an XML file in a certain order
in PHP Coding Help
Posted
Look through the Google results I linked. Perhaps this one?