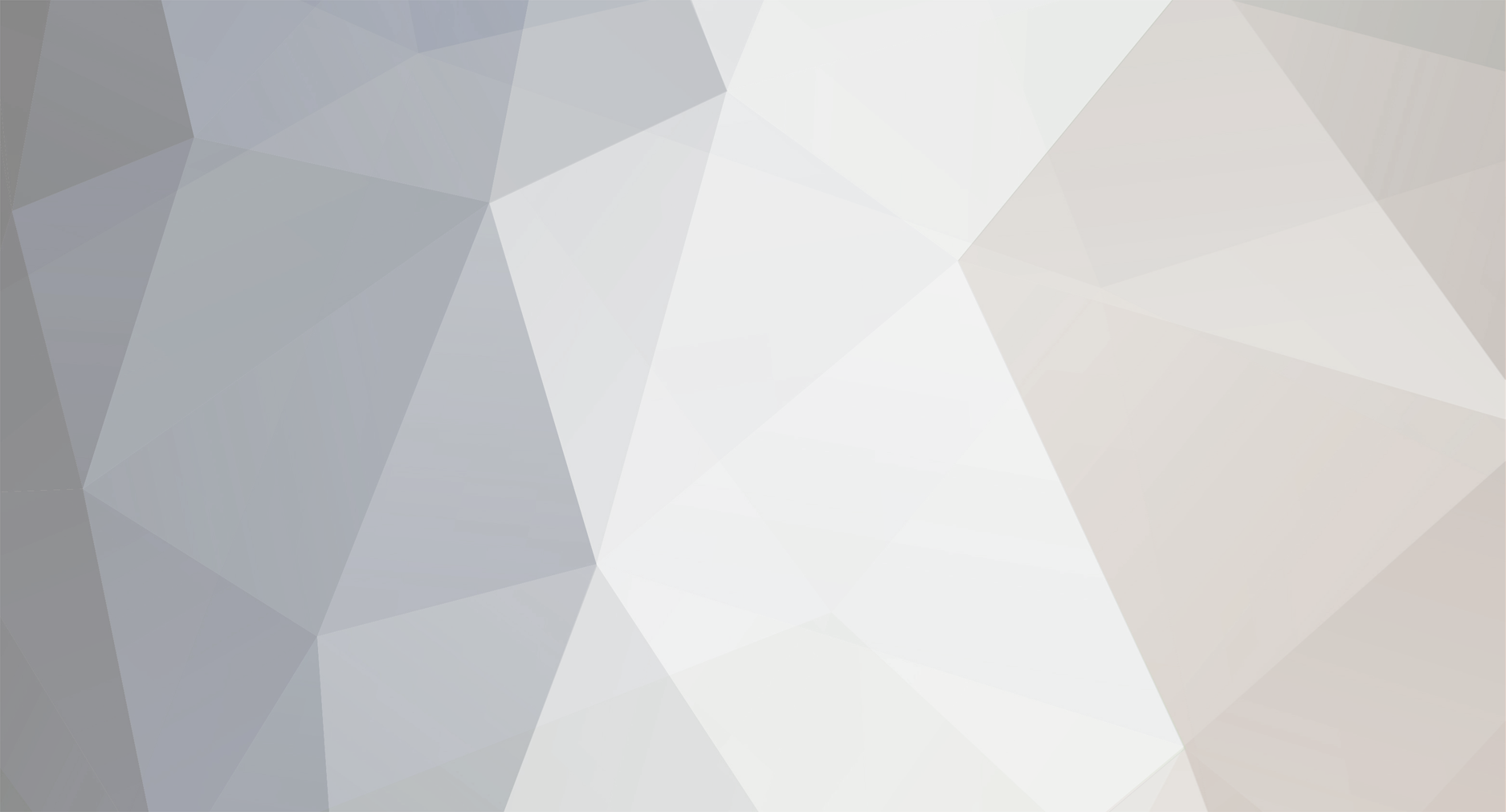
effigy
-
Posts
3,600 -
Joined
-
Last visited
Never
Posts posted by effigy
-
-
What encoding are you working in?
-
must be either a number or a word - number cannot end with .
How do you define a number or word?
-
<pre> <?php $fig = array ( '1,249', '33,182', '33182', '9,333', '3,981,847', '9,149', '3,294', '17,928', '1,413', '2,994' ); $fig = array_map(create_function('$num', 'return preg_replace("/\D/", "", $num);'), $fig); sort($fig); $fig = array_map(create_function('$num', 'return number_format($num);'), $fig); print_r($fig); ?> </pre>
-
One of your <a> tags has content and there are no hrefs? How about !<a[^>]+name="[^"]+"[^>]*>.*?</a>!is?
-
With a flexible approach to a "number" or "word":
<pre> <?php $str = '>< > < >123.< > 999 < >8,88< >eight< > eig.ht < >ni.ne< > nine <'; preg_match_all('#>\s*(?:[\d,.]+(?<!\.)|[a-z.]+)\s*<#is', $str, $matches); print_r($matches); ?> </pre>
-
What is the expected result? I get <h2>Test <p> <a href="test.php">Test2</a>.
<<< is the heredoc syntax.
-
| is a metacharacter in regex. Use \| to match a literal pipe. explode would be better in this instance.
-
The function automatically arrays the captures; observe print_r($match);.
-
You're matching HTML, so you're not going to see it if you print it--the browser is parsing it.
<pre> <?php $str = '</td> </tr> </table>'; preg_match('%</td>\s*</tr>\s*</table>%', $str, $matches); foreach ($matches as &$match) { $match = htmlspecialchars($match); } print_r($matches); ?> </pre>
-
At some point the . was \S to only capture non-whitespace. I can't figure out why this changed in the old posts, but that's what you're after. I've restored this and cleaned up the img addition with alternation:
preg_match_all('% ( (?> ### Protocol or start. (?: (??:https?|ftp)://) | www\. ) ### Body: gobble non-space, [/url], [/img] (??!\[/(?:url|img)\])\S)+ ### Avoid ending punctuation. (?<!\s\p{P}) ) ### Not followed by an url/img end. (?!\[/(?:url|img)\]) ) %x', $str, $matches);
-
Main Entry: hook·er
Pronunciation: \ˈhu̇-kər\
Function: noun
Date: 1567
1 : one that hooks
2 : drink <a hooker of Scotch>
3 : prostitute
-
Can you include a full sample of data since "Chat" and "Username" gave you trouble? Are you simply trying to remove the brackets, or add the text shown in your example as well?
-
Use a character class: #\b(a)(?=\s[aeiou])#i
-
You need to isolate the HTML, similar to this.
-
2. Do you mean like:
$1 =~ /(\w+)\s*=\s*"([^"]+)"/g
(notice the addition of \s*)
Yes.
-
1. You're only going to catch double-quoted attributes.
2. You haven't accounted for rogue white space.
3. There are various modules on CPAN for things like this; my take is below:
use strict; use warnings; use XML::Twig; use Data::Dumper; my $data = <<HTML; <img src="foo" height="1" /> <img src="testing!" height="50" width="200" /> <img height="20" src="lol" /> HTML my @atts; my $twig = XML::Twig->new( twig_roots => { 'img' => sub { push @atts, $_->atts(); } } ); $twig->parse_html($data); print Data::Dumper->Dump([\@atts]);
-
Search for "email valid" in this forum.
-
You could try a split between the two desired patterns--/(?:A|B)/--and move forward from there. If you need additional help, please provide more data.
-
I believe this is what the manual is referring to here:
All values in repeating quantifiers must be less than 65536.
There must be a better way of partitioning or analyzing your data.
-
What about the VIN format indicates that a vehicle is "GVW < 10k lbs"?
-
The whole pattern is stored in index 0, and the parenthetical content is stored in subsequent numbers. array_shift($matches); removed the entire pattern match.
-
<pre> <?php $data = <<<DATA <p><em class="example"> 3.30 </em></p> DATA; preg_match('/<em class="example">\s*(\d{1,2}.\d{2})/', $data, $matches); array_shift($matches); print_r($matches); ?> </pre>
-
<pre> <?php $string = 'My Item, N-female'; echo preg_replace('/,(?=\s+.{3})/s', '', $string); ?> </pre>
-
<pre> <?php $tests = array( ### Pass. 'username:secret@host', 'username:secret:username@host', 'username:secret@host:000/username', 'username:secret:username@host:000/username', ### Fail. 'username:secret@host/', 'username:secret:username:etc@host', 'username:secret@host:username', 'username@host:000/username', 'username:secret:username@host:000@username', ); foreach ($tests as $test) { echo $test, ' <b>'; echo preg_match('% \A [^:@/]+:[^:@/]+(?::[^:@/]+)? @[^:@/]+ (?::\d+/.+)? \z %x', $test) ? 'Pass' : 'Fail'; echo '</b><br/>'; } ?> </pre>
How to replace werid chars
in PHP Coding Help
Posted
Word's "smart quotes" should not translate like this. Can you elaborate? All of those characters are in Windows-1252, where only "â" carries over to Latin-1.