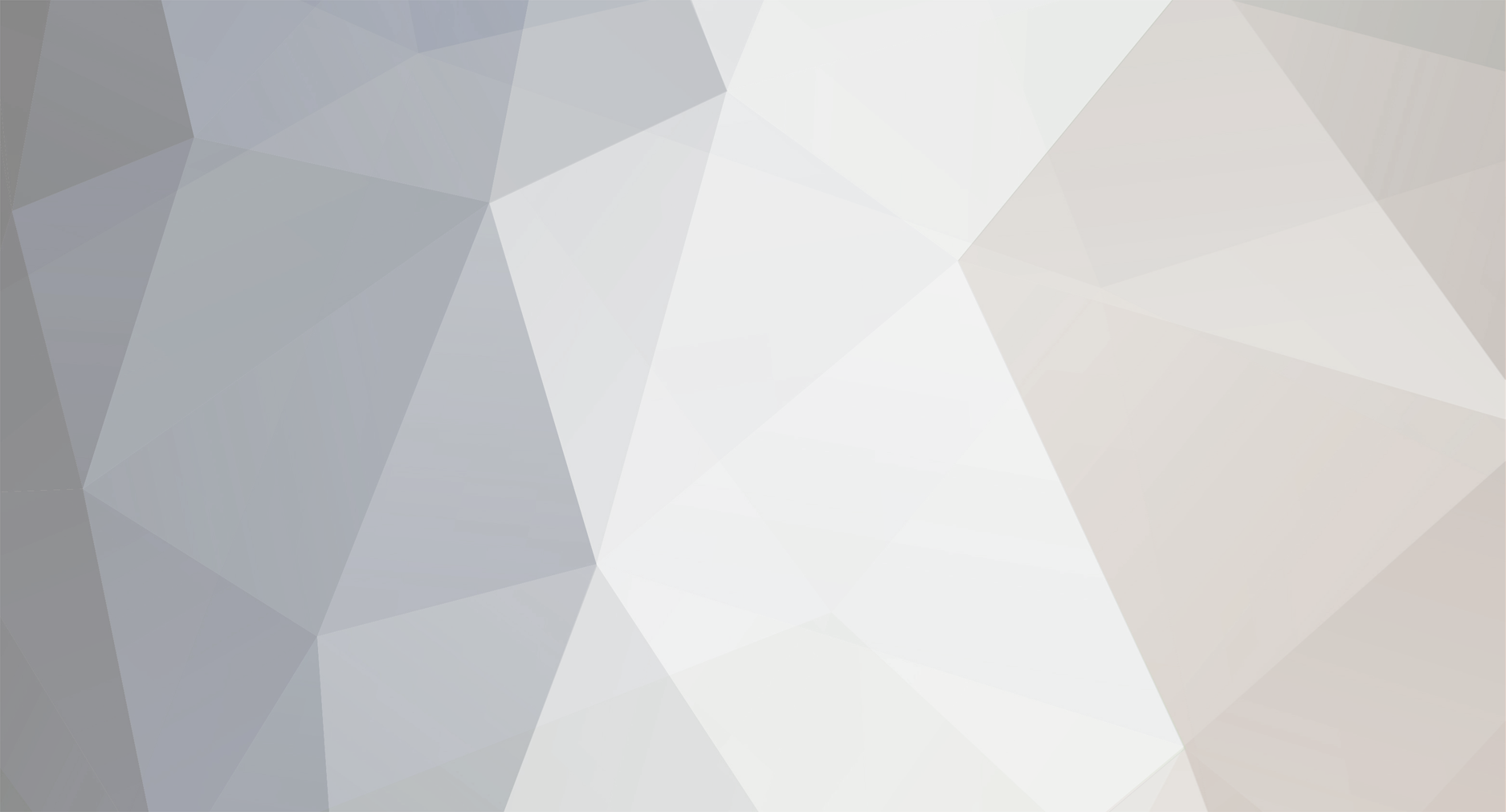
effigy
-
Posts
3,600 -
Joined
-
Last visited
Never
Posts posted by effigy
-
-
If the file is in a Unix format:
<pre> <?php $data = <<<DATA I am a good boy 23. I am a good boy 34. I am a good boy 24. I am a good boy 21. I am a good boy 45. DATA; preg_match('/(\d+)\.$/m', $data, $matches); print_r($matches); ?> </pre>
-
Do you only want the first price? If so, you should use preg_match.
<pre> <?php $data = <<<DATA FareAdult1.00 OFFERAdult1.00 OfferAdult2.00 FareAdult15.00 DATA; function regex_price($data) { preg_match_all('/(?:Fare|OFFER)Adult([\d.]+)/', $data, $result_price, PREG_PATTERN_ORDER); return $result_price; } print_r(regex_price($data)); ?> </pre>
-
$pieces = preg_split('/\S+/', $name);
Effigy.. I'm rather curious.. As I examine your pattern, I can't help but wonder.. Since you are matching any non-space character one or more consecutive times.. is this pretty much a 'beefier' version of:
'/\b\w+\b/' ? (since \w is basically a-zA-Z0-9_ (locale issues aside), I suppose your pattern anticipates a broader range of characters and let's the spaces act as 'word bounderies' or sorts)
Essentially, yes. In many cases preg_split is better because you're being specific about what you do not want, rather than attempting to make a pattern that's versatile enough to match what you do want.
The names are in all sorts of different formats
What problems are you having with the given code? Do you need each variation to look for a middle name?
-
How about this?
$pieces = preg_split('/\S+/', $name);
-
Would noclobber help in the future?
-
Are the names together in one field and you're trying to separate the names, or are you trying to break a single name into pieces?
-
preg uses PCRE patterns; fnmatch uses shell wildcard patterns.
P.S. Always quote variables that are used in expressions: $regex = '/' . preg_quote($search) . '/i';
-
Why are you using a regular expression if you want to insert all of the elements? Why can't you simply use $file from your example? Do you want to break the data apart by pairs of tags? What if these contain other tags?
-
Look here.
-
You need the parentheses. Look at my example again.
What are you trying to do for every element?
-
See this topic. My solution is at the bottom.
-
What is the exact format of this data (try a hex dump)? What are the black question marks--nulls?
-
Here's a start:
preg_match_all('%<(table|area)[^>]*>.*?</\1>%', $data, $matches); print_r($matches);
-
<pre> <?php $data = 'Zend_Db_Table_Abstract'; echo preg_replace('/\b([A-Za-z]+(?:_[A-Za-z]+)+)\b/e', 'join("::", explode("_", "$1"))', $data); ?> </pre>
Update:
...is there a way I can avoid this without knowing how many times $4 will match?
It will use the last match saved into the backreference each time it needs to be used. If a new match is found by capturing parentheses, the previously saved match is overwritten.
-
Do you want to match both, or just IPs (see below)?
<pre> <?php $data = <<<DATA http://subdomain.example.com/index.php or if it's just an IP like: http://72.14.207.99/index.php DATA; preg_match_all('%http://[\d.]+\S+(?<!\p{P})%', $data, $matches); print_r($matches); ?> </pre>
-
Upon review, you'll want to update the split pattern if your data contains escaped quotes: /((?<!\\\)([\'"]).*?(?<!\\\)\2)/
-
Without knowing all of the intricate ins and outs of SQL, that's probably the best approach. My take is below.
You may also want to have a look at Perl's SQL::Statement. SQL::Statement::Structure pulls out the table names for you. Update: There's also SQL_Parser for PHP; I'm not sure what it has to offer.
<pre> <?php $tests = array( "A 'B C' D E F \"G\" H I 'J \"K\" L M' N O P \"Q 'R' S\" T U V 'W X Y' Z", 'A \'B C\' D E F "G" H I \'J "K" L M\' N O P "Q \'R\' S" T U V \'W\' X \'Y\' Z', ); foreach ($tests as $test) { $result = array(); ### Split the string based on quote pairs. $pieces = preg_split('/(([\'"]).*?\2)/', $test, -1, PREG_SPLIT_NO_EMPTY | PREG_SPLIT_DELIM_CAPTURE); $num_pieces = count($pieces); for ($i = 0; $i < $num_pieces; $i++) { $piece = $pieces[$i]; ### Skip lone quotes. if ($piece == '"' || $piece == "'") { continue; } ### Only replace entries that are not quoted. ### Table name is "X" for sake of example. $first = substr($piece, 0, 1); $piece = $first == '"' || $first == "'" ? $piece : preg_replace('/\b(X)\b/', 'prefix_$1', $piece) ; ### Push. array_push($result, $piece); } print_r($result); } ?> </pre>
-
You can also resort to 'conditionals'
Why would you use such complexity for a simple problem?
-
<pre> <?php $data = <<<DATA <img src="http://www.somewhere.com/image.jpg"> Visit www.phpfreaks.com! <a href="http://www.google.com">http://www.google.com</a> See http://www.regular-expressions.info DATA; $pieces = preg_split('/(<[^>]+>)/', $data, -1, PREG_SPLIT_NO_EMPTY | PREG_SPLIT_DELIM_CAPTURE); $num_pieces = count($pieces); for ($i = 0; $i < $num_pieces; $i++) { ### Skip tags or content of <a>. if (substr($pieces[$i], 0, 1) == '<' || array_key_exists($i-1, $pieces) && substr($pieces[$i-1], 0, 3) == '<a ' ){ continue; } $pieces[$i] = preg_replace('% ( (?: (??:https?|ftp)://) | www\. ) \S+ (?<!\p{P}) ) %x', '<a href="$1">$1</a>', $pieces[$i]); } echo htmlspecialchars(join('', $pieces)); ?> </pre>
-
. will not match new lines without the /s modifier. You also need a lazy quantifier: /<ul>(.*?)<ul>/is
-
The parentheses are unnecessary: #/\w+$#.
-
This does not work for me. What version of PHP are you running?
-
I cannot find any information on this. My assumption is that it doesn't exist because there isn't a need for it. A data structure is being built when matching, so it's nice to be able to customize the key, whereas replacing is solely done by the engine.
In the event that your replaces get nasty, the best approach is to use the /x modifier:
/
### 1. Name
(...)
### 2. Address
(...)
### 3. And so forth...
(...)
/x
-
The downside to using \s is that it encompasses all whitespace. This includes spaces, form feeds, new lines, carriage returns, horizontal tabs, and vertical tabs. If you prefer to not see a blank space, you can use \x20.
preg_replace()
in Regex Help
Posted