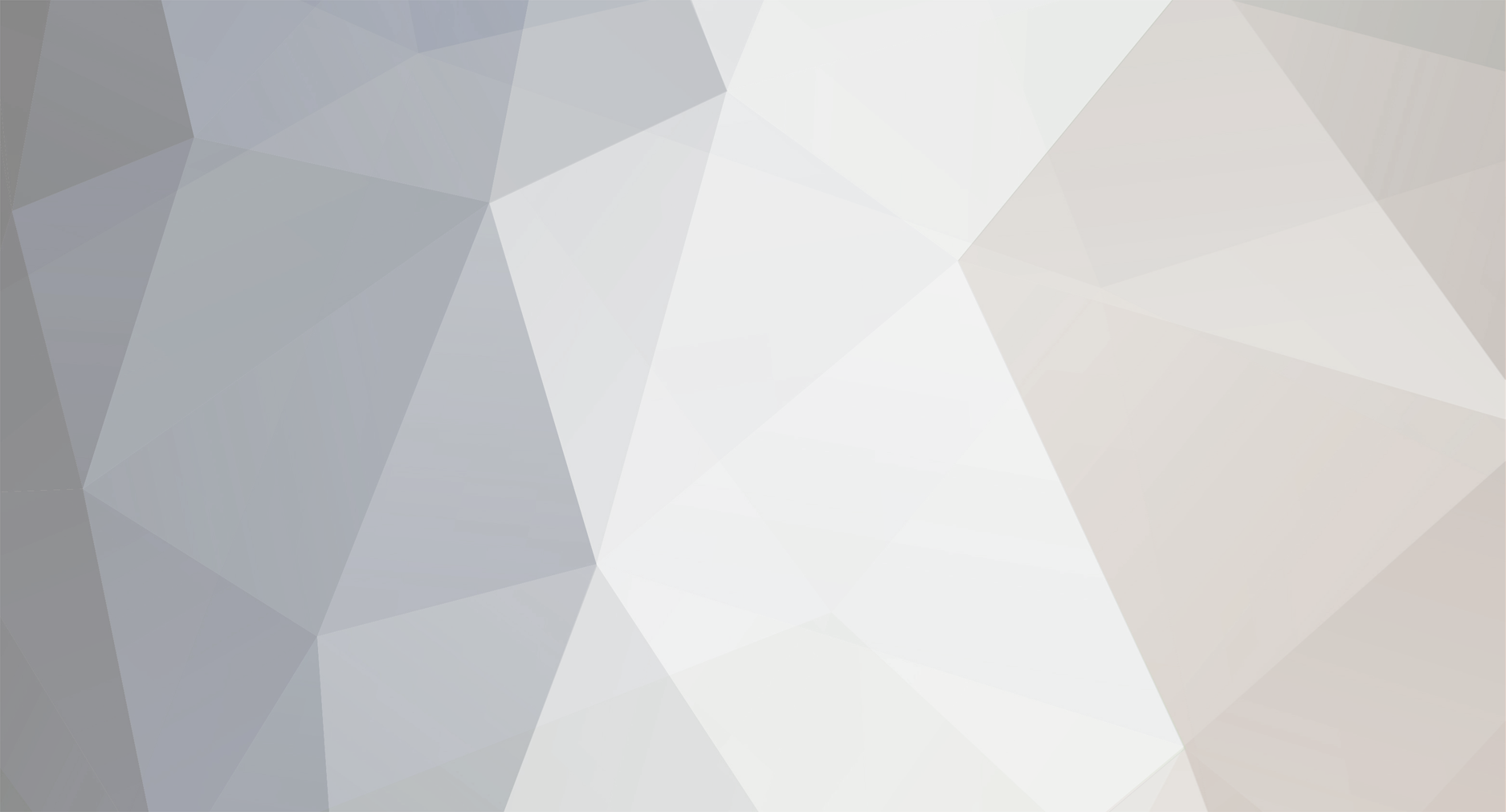
mallen
Members-
Posts
316 -
Joined
-
Last visited
Everything posted by mallen
-
Like this? I need the commas and closing brackets in the correct place. $xml = new AuthnetXML(AUTHNET_LOGIN, AUTHNET_TRANSKEY, AuthnetXML::USE_DEVELOPMENT_SERVER); $xml->createCustomerProfileTransactionRequest(array( 'transaction' => array( 'profileTransAuthCapture' => array( 'amount' => '10.95', 'tax' => array( 'amount' => '1.00', 'name' => 'state sales tax', 'description' => 'your state sales tax' ), 'shipping' => array( 'amount' => '2.00', 'name' => 'ground based shipping', 'description' => 'Ground based 5 to 10 day shipping' ), $i = 1; foreach($Order->Cart->contents as $Item) { $lineItems[] = array ( 'itemId' => $i++, 'name' => $Item->name, 'quantity' => $Item->quantity, 'unitPrice' => $Item->unitprice ); } $whatYouWant = 'lineItems = ' . var_export($lineItems, 1); 'customerProfileId' => '345', 'customerPaymentProfileId' => '675', 'customerShippingAddressId' => '453', 'order' => array( 'invoiceNumber' => 'INV000001', 'description' => 'description of transaction', 'purchaseOrderNumber' => '000001' ), 'taxExempt' => 'false', 'recurringBilling' => 'false', 'cardCode' => '000' ) ), 'extraOptions' => '<![CDATA[x_customer_ip=100.0.0.1]]>' ));
-
It needs to look like this when its sent. $xml = new AuthnetXML(AUTHNET_LOGIN, AUTHNET_TRANSKEY, AuthnetXML::USE_DEVELOPMENT_SERVER); $xml->createCustomerProfileTransactionRequest(array( 'transaction' => array( 'profileTransAuthCapture' => array( 'amount' => '10.95', 'tax' => array( 'amount' => '1.00', 'name' => 'state sales tax', 'description' => 'your state sales tax' ), 'shipping' => array( 'amount' => '2.00', 'name' => 'ground based shipping', 'description' => 'Ground based 5 to 10 day shipping' ), 'lineItems' => array( ////////this is the part I need from here 0 => array( 'itemId' => '1', 'name' => 'widget', 'quantity' => '5', 'unitPrice' => '10.00' ), 1 => array( 'itemId' => '2', 'name' => 'another widget', 'quantity' => '20', 'unitPrice' => '10.00' ) ), /////////////////to here 'customerProfileId' => '345', 'customerPaymentProfileId' => '675', 'customerShippingAddressId' => '453', 'order' => array( 'invoiceNumber' => 'INV000001', 'description' => 'description of transaction', 'purchaseOrderNumber' => '000001' ), 'taxExempt' => 'false', 'recurringBilling' => 'false', 'cardCode' => '000' ) ), 'extraOptions' => '<![CDATA[x_customer_ip=100.0.0.1]]>' ));
-
I am working with a API and need to change the format of the data I send. I need this // Line Items $i = 1; foreach($Order->Cart->contents as $Item) { $_['x_line_item'][] = ($i++)."<|>".substr($Item->name,0,31)."<|>".((sizeof($Item->options) > 1)?" (".substr($Item->option->label,0,253).")":"")."<|>".(int)$Item->quantity."<|>".number_format($Item->unitprice,$this->precision,'.','')."<|>".(($Item->tax)?"Y":"N"); } Converted to this: 'lineItems' => array( 0 => array( 'itemId' => '1', 'name' => $Item->name, 'quantity' => $Item->quantity, 'unitPrice' => $Item->unitprice ), 1 => array( 'itemId' => '2', 'name' => $Item->name, 'quantity' => $Item->quantity, 'unitPrice' => $Item->unitprice ) I tried it like this but I don't have it correct: $i = 1; foreach($Order->Cart->contents as $Item) { 'lineItems' = ($i++). "<|>".substr($Item->name,0,31). "<|>".(int)$Item->quantity. "<|>".number_format($Item->unitPrice,$this->precision,'.',''); }
-
Finally working. John Conde's tutorial and code here was the solution. https://github.com/stymiee/Authorize.Net-XML I had modified this line and had left off USE_DEVELOPMENT_SERVER and then I got the error that the account was in test mode. So atleast it is connecting and creating a profile. Now I will work on other parts such as transactions and integrating to my cart. $xml = new AuthnetXML(AUTHNET_LOGIN, AUTHNET_TRANSKEY,AuthnetXML::USE_DEVELOPMENT_SERVER);
-
Getting closer. $xml = new AuthnetXML(AUTHNET_LOGIN, AUTHNET_TRANSKEY); $xml->createCustomerPaymentProfileRequest(array( 'customerProfileId' => '666', 'paymentProfile' => array( 'billTo' => array( 'firstName' => $Order->Customer->firstname, 'lastName' => $Order->Customer->lastname, 'address' => $Order->Billing->address, 'city' => $Order->Billing->city, 'state' => $Order->Billing->state, 'zip' => $Order->Billing->postcode, 'phoneNumber' => $Order->Customer->phone ), 'payment' => array( 'creditCard' => array( 'cardNumber' => $Order->Billing->card, // 'expirationDate' => date("my",$Order->Billing->cardexpires) 'expirationDate' => '2013-08' ), ), ), 'shipToList' => array( 'firstName' => $Order->Customer->firstname, 'lastName' => $Order->Customer->lastname, 'address' => $Order->Shipping->address, 'city' => $Order->Shipping->city, 'state' => $Order->Shipping->state, 'zip' => $Order->Shipping->postcode, 'phoneNumber' => '(800)555-1234', 'validationMode' => 'testMode' ), )); echo $xml->messages->resultCode; echo $xml->messages->message->code; echo $xml; Get this error: <code>E00003</code> <text>The element 'createCustomerPaymentProfileRequest' in namespace 'AnetApi/xml/v1/schema/AnetApiSchema.xsd' has invalid child element 'shipToList' in namespace 'AnetApi/xml/v1/schema/AnetApiSchema.xsd'. List of possible elements expected: 'validationMode' in namespace 'AnetApi/xml/v1/schema/AnetApiSchema.xsd'.</text> </message> </messages>
-
Thisis the new approach I am using. https://github.com/stymiee/Authorize.Net-XML/blob/master/examples/cim/createCustomerProfileRequest.php Here is my code now. require('config.inc.php'); require('AuthnetXML.class.php'); class AuthorizeNet extends GatewayFramework implements GatewayModule { var $cards = array("visa", "mc", "amex", "disc", "jcb", "dc"); var $liveurl = 'https://secure.authorize.net/gateway/transact.dll'; var $testurl = 'https://secure.authorize.net/gateway/transact.dll'; function AuthorizeNet () { parent::__construct(); $this->setup('login','password','testmode'); } function actions () { add_action('mycart_process_order',array(&$this,'process')); } function process () { $transaction = $this->build(); } function build () { $Order = $this->Order; $xml = new AuthnetXML(AUTHNET_LOGIN, AUTHNET_TRANSKEY); $xml->createCustomerPaymentProfileRequest(array( 'customerProfileId' => '12345', 'paymentProfile' => array( 'billTo' => array( 'firstName' => $Order->Customer->firstname, 'lastName' => $Order->Customer->lastname, 'company' => '', 'address' => $Order->Billing->address, 'city' => $Order->Billing->city, 'state' => $Order->Billing->state, 'zip' => $Order->Billing->postcode, 'country' => 'USA', 'phoneNumber' => $Order->Customer->phone, 'faxNumber' => '' ), 'payment' => array( 'creditCard' => array( 'cardNumber' => $Order->Billing->card, 'expirationDate' => date("my",$Order->Billing->cardexpires) ) ) ), 'validationMode' => 'liveMode' )); } function send ($data) { if ($this->settings['testmode'] == "on") $url = $this->testurl; else $url = $this->liveurl; $url = apply_filters('mycart_authorize_net_url',$url); return $this->response(parent::send($data,$url)); } function settings () { $this->ui->cardmenu(0,array( 'name' => 'cards', 'selected' => $this->settings['cards'] ),$this->cards); $this->ui->text(1,array( 'name' => 'login', 'value' => $this->settings['login'], 'size' => '16', 'label' => __('Enter your AuthorizeNet Login ID.','Mycart') )); $this->ui->password(1,array( 'name' => 'password', 'value' => $this->settings['password'], 'size' => '24', 'label' => __('Enter your AuthorizeNet Password or Transaction Key.','Mycart') )); $this->ui->checkbox(1,array( 'name' => 'testmode', 'checked' => $this->settings['testmode'], 'label' => __('Enable test mode','Mycart') )); } } // END class AuthorizeNet ?> Here is the error I get. Fatal error: Uncaught exception 'AuthnetXMLException' with message 'Connection error: error setting certificate verify locations: CAfile: C:\inetpub\wwwroot\......gateways\AuthorizeNet/ssl /cert.pem CApath: none (77)' in C:\inetpub\wwwroot....\AuthorizeNet\AuthnetXML.class.php:211 Stack trace: #0 C:\inetpub\wwwroot.......\AuthorizeNet\AuthnetXML.class.php(150): AuthnetXML->process() #1 C:\inetpub\wwwroot........\gateways\AuthorizeNet\Authorize.net.php(81): AuthnetXML->__call('createCustomerP...', Array) #2 C:\inetpub\wwwroot.....\gateways\AuthorizeNet\Authorize.net.php(81): AuthnetXML->createCustomerPaymentPr in C:\inetpub\wwwroot......\gateways\AuthorizeNet\AuthnetXML.class.php on line 211
-
I came across this file and it looks like it might be what I am trying to do. https://github.com/stymiee/Authorize.Net-XML/blob/master/examples/cim/createCustomerProfileRequest.php See how all the info is put into an array. How would I use my value that is: $Order->Customer->firstname; and change it to this format? 'firstName' => 'John', can it be like this? 'firstName' => ' $Order->Customer->firstname;'
-
It just gives me that URL error.
-
I never really "fixed" it before. It was using another copy of Authize.Net.php I put all the code to send the XML in a function and it didn't seem to work. I see the function in vars.php which is one of the included files for Authize.net API but need to find a way to get my content to that function. function send_xml_request($content) { global $g_apihost, $g_apipath; return send_request_via_fsockopen($g_apihost,$g_apipath,$content); } There is this thing in Wordpress called add_action that fires the process function I called from here. Also set it to fire build() and send_xml() function actions () { //add_action('mycart_process_order',array(&$this,'process')); add_action('mycart_process_order','process'); add_action('mycart_process_order','build'); add_action('mycart_process_order','send_xml'); }
-
I tried to simplify the transaction just to get it to connect to Authorize.net. THisis what I have now. I put the XML code in a function by itself. Getting Raw request: <?xml version="1.0" encoding="utf-8"?><createCustomerProfileRequest xmlns="AnetApi/xml/v1/schema/AnetApiSchema.xsd"><merchantAuthentication><name>xxxx</name><transactionKey>xxxxx</transactionKey></merchantAuthentication><profile><merchantCustomerId>9999</merchantCustomerId><description>none</description><email>some@somedomain.com</email></profile></createCustomerProfileRequest> Raw response: <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN""http://www.w3.org/TR/html4/strict.dtd"> <HTML><HEAD><TITLE>Bad Request</TITLE> <META HTTP-EQUIV="Content-Type" Content="text/html; charset=us-ascii"></HEAD> <BODY><h2>Bad Request - Invalid URL</h2> <hr><p>HTTP Error 400. The request URL is invalid.</p> </BODY></HTML> require_once 'XML/util.php'; require_once 'XML/vars.php'; require_once 'anet_php_sdk/AuthorizeNet.php'; $METHOD_TO_USE = "CIM"; class AuthorizeNet extends GatewayFramework implements GatewayModule { var $cards = array("visa", "mc", "amex", "disc", "jcb", "dc"); var $liveurl = 'https://test.authorize.net/xml/v1/request.api'; var $testurl = 'https://apitest.authorize.net/xml/v1/request.api'; function AuthorizeNet () { parent::__construct(); $this->setup('login','password','testmode'); } function actions () { add_action('mycart_process_order',array(&$this,'process')); //add_action('mycart_process_order','process'); add_action('mycart_process_order','build'); } function process () { $transaction = $this->build(); } function build () { $this->sendxml(); }//end build function sendxml () { //build xml to post $content = "<?xml version=\"1.0\" encoding=\"utf-8\"?>" . "<createCustomerProfileRequest xmlns=\"AnetApi/xml/v1/schema/AnetApiSchema.xsd\">" . "<merchantAuthentication>" . "<name>xxxx</name>". "<transactionKey>xxxx</transactionKey>" . "</merchantAuthentication>" . "<profile>". "<merchantCustomerId>9999</merchantCustomerId>". // Your own identifier for the customer. "<description>none</description>". "<email>email@somedomain.com</email>". "</profile>". "</createCustomerProfileRequest>"; echo "Raw request: " . htmlspecialchars($content) . "<br><br>"; $response = send_xml_request($content); echo "Raw response: " . htmlspecialchars($response) . "<br><br>"; $parsedresponse = parse_api_response($response); if ("Ok" == $parsedresponse->messages->resultCode) { echo "A transaction was successfully created for customerProfileId <b>" . htmlspecialchars($_POST["customerProfileId"]) . "</b>.<br><br>"; if (isset($parsedresponse->directResponse)) { echo "direct response: <br>" . htmlspecialchars($parsedresponse->directResponse) . "<br><br>"; $directResponseFields = explode(",", $parsedresponse->directResponse); $responseCode = $directResponseFields[0]; // 1 = Approved 2 = Declined 3 = Error $responseReasonCode = $directResponseFields[2]; // See http://www.authorize.net/support/AIM_guide.pdf $responseReasonText = $directResponseFields[3]; $approvalCode = $directResponseFields[4]; // Authorization code $transId = $directResponseFields[6]; if ("1" == $responseCode) echo "The transaction was successful.<br>"; else if ("2" == $responseCode) echo "The transaction was declined.<br>"; else echo "The transaction resulted in an error.<br>"; echo "responseReasonCode = " . htmlspecialchars($responseReasonCode) . "<br>"; echo "responseReasonText = " . htmlspecialchars($responseReasonText) . "<br>"; echo "approvalCode = " . htmlspecialchars($approvalCode) . "<br>"; echo "transId = " . htmlspecialchars($transId) . "<br>"; echo "<br><a href=index.php?customerProfileId=" . urlencode($_POST["customerProfileId"]) . "&customerPaymentProfileId=" . urlencode($_POST["customerPaymentProfileId"]) . "&customerShippingAddressId=" . urlencode($_POST["customerShippingAddressId"]) . ">Continue</a><br>"; } } }//end send xml function send ($data) { // if ($this->settings['testmode'] == "on") $url = $this->testurl; //else $url = $this->liveurl; $url = 'https://test.authorize.net/xml/v1/request.api'; //added $url = apply_filters('mycart_authorize_net_url',$url); return $this->response(parent::send($data,$url)); } function response ($buffer) { $_ = new stdClass(); list($_->code, $_->subcode, $_->reasoncode, $_->reason, $_->authcode, $_->avs, $_->transactionid, $_->invoicenum, $_->description, $_->amount, $_->method, $_->type, $_->customerid, $_->firstname, $_->lastname, $_->company, $_->address, $_->city, $_->state, $_->zip, $_->country, $_->phone, $_->fax, $_->email, $_->ship_to_first_name, $_->ship_to_last_name, $_->ship_to_company, $_->ship_to_address, $_->ship_to_city, $_->ship_to_state, $_->ship_to_zip, $_->ship_to_country, $_->tax, $_->duty, $_->freight, $_->taxexempt, $_->ponum, $_->md5hash, $_->cvv2code, $_->cvv2response) = explode(",",$buffer); return $_; } function settings () { $this->ui->cardmenu(0,array( 'name' => 'cards', 'selected' => $this->settings['cards'] ),$this->cards); $this->ui->text(1,array( 'name' => 'login', 'value' => $this->settings['login'], 'size' => '16', 'label' => __('Enter your AuthorizeNet Login ID.','Mycart') )); $this->ui->password(1,array( 'name' => 'password', 'value' => $this->settings['password'], 'size' => '24', 'label' => __('Enter your AuthorizeNet Password or Transaction Key.','Mycart') )); $this->ui->checkbox(1,array( 'name' => 'testmode', 'checked' => $this->settings['testmode'], 'label' => __('Enable test mode','Mycart') )); } } // END class AuthorizeNet
-
Which line? Just realized it was trying to read another copy of the file I have in the same folder. Anything in the folder it will try to read. So. Now getting Fatal error: Class 'AuthorizeNetCustomer' not found.
-
Thanks requinix. You are always helpful on here. This is the code I have now. I put all my CIM code in the build() function. I get this error: (TESTMODE) The merchant login ID or password is invalid or the account is inactive. include_once ("XML/util.php"); include_once ("XML/vars.php"); class AuthorizeNet extends GatewayFramework implements GatewayModule { var $cards = array("visa", "mc", "amex", "disc", "jcb", "dc"); //var $liveurl = 'https://secure.authorize.net/gateway/transact.dll'; //var $testurl = 'https://secure.authorize.net/gateway/transact.dll'; //worksvar $testurl = 'https://test.authorize.net/gateway/transact.dll'; //var $testurl = 'https://apitest.authorize.net/xml/v1/request.api'; //var $liveurl = 'https://test.authorize.net/xml/v1/request.api'; var $testurl = 'https://apitest.authorize.net/xml/v1/request.api'; function AuthorizeNet () { parent::__construct(); $this->setup('login','password','testmode'); } function actions () { add_action('mycart_process_order',array(&$this,'process')); add_action('mycart_process_order','runxml'); } function process () { $transaction = $this->build(); $Response = $this->send($transaction); if ($Response->code == '1') { // success $this->Order->transaction($this->txnid($Response),'CHARGED'); //return; } elseif ($Response->code == '4') { // flagged for merchant review or risk management $this->Order->transaction($this->txnid($Response),'PENDING'); //return; } else $this->error($Response); } function txnid ($Response) { if (empty($Response->transactionid)) return parent::txnid(); return $Response->transactionid; } function error ($Response) { return new MycartError($Response->reason,'authorize_net_error',MYCART_TRXN_ERR, array('code'=>$Response->reasoncode)); } function build () { //create Auth & Capture Transaction $request = new AuthorizeNetCIM; $customerProfile = new AuthorizeNetCustomer; $customerProfile->description = "Description of customer here"; $customerProfile->merchantCustomerId = 987; //create and add payment profiles and addresses // add payment profile. $paymentProfile = new AuthorizeNetPaymentProfile; $paymentProfile->customerType = "individual"; $paymentProfile->payment->creditCard->cardNumber = $Mycart->Order->Billing->card; $paymentProfile->payment->creditCard->expirationDate = date("my",$Mycart->Order->Billing->cardexpires); $paymentProfile->payment->creditCard->cardCode = $Mycart->Order->Billing->cvv; $customerProfile->paymentProfiles[] = $paymentProfile; $customerProfile->email = $Order->Customer->email; //add a shipping address $address = new AuthorizeNetAddress; $address->firstName = $Mycart->Order->Customer->firstname; $address->lastName = $Mycart->Order->Customer->lasttname; $address->company = "John Doe Company"; $address->address = $Mycart->Order->Shipping->address; $address->city = $Mycart->Order->Shipping->city; $address->state = $Mycart->Order->Shipping->state; $address->zip = $Mycart->Order->Shipping->postcode; $address->country = $Mycart->Order->Shipping->country; $address->phoneNumber = $Mycart->Order->Customer->phone; $response = $request->createCustomerShippingAddress($customerProfileId, $address); $customerAddressId = $response->getCustomerAddressId(); //create the transaction $transaction = new AuthorizeNetTransaction; $transaction->customerProfileId = $customerProfileId; $transaction->customerPaymentProfileId = $paymentProfileId; $transaction->customerShippingAddressId = $customerAddressId; $transaction->amount = $Order->Cart->Totals->total; //$lineItem = new AuthorizeNetLineItem; $transaction->lineItems[] = $lineItem; $response = $request->createCustomerProfileTransaction("AuthCapture", $transaction); $transactionResponse = $response->getTransactionResponse(); $transactionId = $transactionResponse->transaction_id; // Line Items $i = 1; foreach($Order->Cart->contents as $Item) { $transaction->lineItems[] = ($i++)."<|>".substr($Item->name,0,31)."<|>".((sizeof($Item->options) > 1)?" (".substr($Item->option->label,0,253).")":"")."<|>".(int)$Item->quantity."<|>".number_format($Item->unitprice,$this->precision,'.','')."<|>".(($Item->tax)?"Y":"N"); } //build xml to post function runxml() { //header('Location: https://apitest.authorize.net/xml/v1/request.api'); //$request = new AuthorizeNetCIM; //$customerProfile = new AuthorizeNetCustomer; //$customerProfile->description = "Description of customer here"; //$customerProfile->merchantCustomerId = 987; //create and add payment profiles and addresses $content = "<?xml version=\"1.0\" encoding=\"utf-8\"?>" . "<createCustomerPaymentProfileRequest xmlns=\"AnetApi/xml/v1/schema/AnetApiSchema.xsd\">" . MerchantAuthenticationBlock(). "<profile>". "<merchantCustomerId>9999</merchantCustomerId>". // Your own identifier for the customer. "<description>none</description>". "<email></email>". "</profile>". "</createCustomerProfileRequest>"; echo "Raw request: " . htmlspecialchars($content) . "<br><br>"; $response = send_xml_request($content); echo "Raw response: " . htmlspecialchars($response) . "<br><br>"; $parsedresponse = parse_api_response($response); if ("Ok" == $parsedresponse->messages->resultCode) { echo "A transaction was successfully created for customerProfileId <b>" . htmlspecialchars($_POST["customerProfileId"]) . "</b>.<br><br>"; } if (isset($parsedresponse->directResponse)) { echo "direct response: <br>" . htmlspecialchars($parsedresponse->directResponse) . "<br><br>"; $directResponseFields = explode(",", $parsedresponse->directResponse); $responseCode = $directResponseFields[0]; // 1 = Approved 2 = Declined 3 = Error $responseReasonCode = $directResponseFields[2]; // See http://www.authorize.net/support/AIM_guide.pdf $responseReasonText = $directResponseFields[3]; $approvalCode = $directResponseFields[4]; // Authorization code $transId = $directResponseFields[6]; if ("1" == $responseCode) echo "The transaction was successful.<br>"; else if ("2" == $responseCode) echo "The transaction was declined.<br>"; else echo "The transaction resulted in an error.<br>"; echo "responseReasonCode = " . htmlspecialchars($responseReasonCode) . "<br>"; echo "responseReasonText = " . htmlspecialchars($responseReasonText) . "<br>"; echo "approvalCode = " . htmlspecialchars($approvalCode) . "<br>"; echo "transId = " . htmlspecialchars($transId) . "<br>"; } echo "<br><a href=index.php?customerProfileId=" . urlencode($_POST["customerProfileId"]) . "&customerPaymentProfileId=" . urlencode($_POST["customerPaymentProfileId"]) . "&customerShippingAddressId=" . urlencode($_POST["customerShippingAddressId"]) . ">Continue</a><br>"; } } function send ($data) { if ($this->settings['testmode'] == "off") $url = $this->testurl; else $url = $this->liveurl; $url = apply_filters('mycart_authorize_net_url',$url); return $this->response(parent::send($data,$url)); } function response ($buffer) { $_ = new stdClass(); list($_->code, $_->subcode, $_->reasoncode, $_->reason, $_->authcode, $_->avs, $_->transactionid, $_->invoicenum, $_->description, $_->amount, $_->method, $_->type, $_->customerid, $_->firstname, $_->lastname, $_->company, $_->address, $_->city, $_->state, $_->zip, $_->country, $_->phone, $_->fax, $_->email, $_->ship_to_first_name, $_->ship_to_last_name, $_->ship_to_company, $_->ship_to_address, $_->ship_to_city, $_->ship_to_state, $_->ship_to_zip, $_->ship_to_country, $_->tax, $_->duty, $_->freight, $_->taxexempt, $_->ponum, $_->md5hash, $_->cvv2code, $_->cvv2response) = explode(",",$buffer); return $_; } function settings () { $this->ui->cardmenu(0,array( 'name' => 'cards', 'selected' => $this->settings['cards'] ),$this->cards); $this->ui->text(1,array( 'name' => 'login', 'value' => $this->settings['login'], 'size' => '16', 'label' => __('Enter your AuthorizeNet Login ID.','Mycart') )); $this->ui->password(1,array( 'name' => 'password', 'value' => $this->settings['password'], 'size' => '24', 'label' => __('Enter your AuthorizeNet Password or Transaction Key.','Mycart') )); $this->ui->checkbox(1,array( 'name' => 'testmode', 'checked' => $this->settings['testmode'], 'label' => __('Enable test mode','Mycart') )); } } // END class AuthorizeNet
-
Its a Wordpress function http://codex.wordpress.org/Function_Reference/apply_filters does this help?
-
I think URL refers to var $liveurl = 'https://secure.authorize.net/gateway/transact.dll'; var $testurl = 'https://apitest.authorize.net/xml/v1/request.api';
-
Thanks for respoding. It is giving me this error. <?xml version="1.0" encoding="utf-8"?><createCustomerPaymentProfileReq uest xmlns="AnetApi/xml/v1/schema/AnetApiSchema.xsd"><merchantAuthentication><name></name><transactionKey></transactionKey></merchantAuthentication><profile><merchantCustomerId> </merchantCustomerId><description>none</description><email></email></profile></createCustomerProfileRequest> Raw response: <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN""http://www.w3.org/TR/html4/strict.dtd"> <HTML><HEAD><TITLE>Bad Request</TITLE> <META HTTP-EQUIV="Content-Type" Content="text/html; charset=us-ascii"> </HEAD> <BODY><h2>Bad Request - Invalid URL</h2> <hr><p>HTTP Error 400. The request URL is invalid.</p> </BODY></HTML I have tried so many approaches. The key issue is that function Build () that sends the data using AIM. I don't need that anymore. But I can't find a way to send the XML. Since my last post I added this section of code and set it up as a function to run but that just gave me the error above. $content = "<?xml version=\"1.0\" encoding=\"utf-8\"?>" . "<createCustomerPaymentProfileRequest xmlns=\"AnetApi/xml/v1/schema/AnetApiSchema.xsd\">" . MerchantAuthenticationBlock(). "<profile>". "<merchantCustomerId>9999</merchantCustomerId>". // Your own identifier for the customer. "<description>none</description>". "<email></email>". "</profile>". "</createCustomerProfileRequest>"; echo "Raw request: " . htmlspecialchars($content) . "<br><br>"; $response = send_xml_request($content); echo "Raw response: " . htmlspecialchars($response) . "<br><br>"; $parsedresponse = parse_api_response($response); if ("Ok" == $parsedresponse->messages->resultCode) { echo "A transaction was successfully created for customerProfileId <b>" . htmlspecialchars($_POST["customerProfileId"]) . "</b>.<br><br>"; } if (isset($parsedresponse->directResponse)) { echo "direct response: <br>" . htmlspecialchars($parsedresponse->directResponse) . "<br><br>"; $directResponseFields = explode(",", $parsedresponse->directResponse); $responseCode = $directResponseFields[0]; // 1 = Approved 2 = Declined 3 = Error $responseReasonCode = $directResponseFields[2]; // See http://www.authorize.net/support/AIM_guide.pdf $responseReasonText = $directResponseFields[3]; $approvalCode = $directResponseFields[4]; // Authorization code $transId = $directResponseFields[6]; if ("1" == $responseCode) echo "The transaction was successful.<br>"; else if ("2" == $responseCode) echo "The transaction was declined.<br>"; else echo "The transaction resulted in an error.<br>"; echo "responseReasonCode = " . htmlspecialchars($responseReasonCode) . "<br>"; echo "responseReasonText = " . htmlspecialchars($responseReasonText) . "<br>"; echo "approvalCode = " . htmlspecialchars($approvalCode) . "<br>"; echo "transId = " . htmlspecialchars($transId) . "<br>"; } echo "<br><a href=index.php?customerProfileId=" . urlencode($_POST["customerProfileId"]) . "&customerPaymentProfileId=" . urlencode($_POST["customerPaymentProfileId"]) . "&customerShippingAddressId=" . urlencode($_POST["customerShippingAddressId"]) . ">Continue</a><br>"; // }
-
Yes I am trying to create the payment profile , the shipping address and then the transaction. Using CIM becuase I need to store the credit card. I have used the sample code they supply but I just hard coded the values. It created the payment profile and transaction. But can't seem to convert my current shopping cart. See this part in the middle of the code I posted. $paymentProfile = new AuthorizeNetPaymentProfile; $paymentProfile->customerType = "individual"; $paymentProfile->payment->creditCard->cardNumber = $Mycart->Order->Billing->card; $paymentProfile->payment->creditCard->expirationDate = date("my",$Mycart->Order->Billing->cardexpires); $paymentProfile->payment->creditCard->cardCode = $Mycart->Order->Billing->cvv; $customerProfile->paymentProfiles[] = $paymentProfile; $customerProfile->email = $Order->Customer->email; //add a shipping address $address = new AuthorizeNetAddress...... In the sample code they have it like this so I can't figure how to have the values sent over to be sent in XML format. //build xml to post $content = "<?xml version=\"1.0\" encoding=\"utf-8\"?>" . "<createCustomerPaymentProfileRequest xmlns=\"AnetApi/xml/v1/schema/AnetApiSchema.xsd\">" . MerchantAuthenticationBlock(). "<customerProfileId>" . $_POST["customerProfileId"] . "</customerProfileId>". "<paymentProfile>". "<billTo>". "<firstName>John</firstName>". "<lastName>Doe</lastName>". "<phoneNumber>000-000-0000</phoneNumber>". "</billTo>". "<payment>". "<creditCard>". "<cardNumber>4111111111111111</cardNumber>". "<expirationDate>2020-11</expirationDate>". // required format for API is YYYY-MM "</creditCard>". "</payment>". "</paymentProfile>". "<validationMode>liveMode</validationMode>". // or testMode "</createCustomerPaymentProfileRequest>";
-
Thanks. Anyone else have opinion on pear?
-
I have a store that uses Authorize.Net API and its the AIM. I want to convert the process to CIM. The trouble I have looks like this function that sends the fields in an array. CIM doesn't use that format. function build () { $Order = $this->Order; //$_ = array(); // Options //$_['x_test_request'] = ($this->settings['testmode'] == "on")?"TRUE":"FALSE"; // Set "TRUE" while testing //$_['x_login'] = $this->settings['login']; //$_['x_password'] = $this->settings['password']; //$_['x_Delim_Data'] = "TRUE"; //$_['x_type'] = "AUTH_CAPTURE"; // = "AUTH_CAPTURE" AUTH_ONLY; //$_['x_method'] = "CC"; //$_['x_email_customer'] = "TRUE"; //$_['x_merchant_email'] = $this->settings['merchant_email']; //$_['x_customer_ip'] = $_SERVER["REMOTE_ADDR"]; //$_['x_fp_sequence'] = mktime(); //$_['x_fp_timestamp'] = time(); //$_['x_card_num'] = $Order->Billing->card; //$_['x_exp_date'] = date("my",$Order->Billing->cardexpires); //$_['x_card_code'] = $Order->Billing->cvv; //$_['x_amount'] = $Order->Cart->Totals->total; } The entire script: require ('/lib/shared/AuthorizeNetRequest.php'); require ('/lib/shared/AuthorizeNetTypes.php'); require ('/lib/shared/AuthorizeNetXMLResponse.php'); require ('/lib/shared/AuthorizeNetResponse.php'); require ('/lib/AuthorizeNetAIM.php'); require ('/lib/AuthorizeNetCIM.php'); include_once ('XML/util.php'); include_once ('XML/vars.php'); //require ('AuthorizeNetCIM.php'); class AuthorizeNet extends GatewayFramework implements GatewayModule { var $cards = array("visa", "mc", "amex", "disc", "jcb", "dc"); //var $liveurl = 'https://secure.authorize.net/gateway/transact.dll'; var $testurl = 'https://apitest.authorize.net/xml/v1/request.api'; function AuthorizeNet () { parent::__construct(); $this->setup('login','password','testmode'); } function actions () { add_action('mycart_process_order',array(&$this,'process')); } function process () { $transaction = $this->build(); $Response = $this->send($transaction); if ($Response->code == '1') { // success $this->Order->transaction($this->txnid($Response),'CHARGED'); return; } elseif ($Response->code == '4') { // flagged for merchant review or risk management $this->Order->transaction($this->txnid($Response),'PENDING'); return; } else $this->error($Response); } function txnid ($Response) { if (empty($Response->transactionid)) return parent::txnid(); return $Response->transactionid; } function error ($Response) { return new MycartError($Response->reason,'authorize_net_error',MYCART_TRXN_ERR, array('code'=>$Response->reasoncode)); } function build () { $Order = $this->Order; //$_ = array(); // Options //$_['x_test_request'] = ($this->settings['testmode'] == "on")?"TRUE":"FALSE"; // Set "TRUE" while testing //$_['x_login'] = $this->settings['login']; //$_['x_password'] = $this->settings['password']; //$_['x_Delim_Data'] = "TRUE"; //$_['x_type'] = "AUTH_CAPTURE"; // = "AUTH_CAPTURE" AUTH_ONLY; //$_['x_method'] = "CC"; //$_['x_email_customer'] = "TRUE"; //$_['x_merchant_email'] = $this->settings['merchant_email']; //$_['x_customer_ip'] = $_SERVER["REMOTE_ADDR"]; //$_['x_fp_sequence'] = mktime(); //$_['x_fp_timestamp'] = time(); //$_['x_card_num'] = $Order->Billing->card; //$_['x_exp_date'] = date("my",$Order->Billing->cardexpires); //$_['x_card_code'] = $Order->Billing->cvv; //$_['x_amount'] = $Order->Cart->Totals->total; // create Auth & Capture Transaction $request = new AuthorizeNetCIM; $customerProfile = new AuthorizeNetCustomer; $customerProfile->description = "Description of customer here"; $customerProfile->merchantCustomerId = 987; //create and add payment profiles and addresses // add payment profile. $paymentProfile = new AuthorizeNetPaymentProfile; $paymentProfile->customerType = "individual"; $paymentProfile->payment->creditCard->cardNumber = $Mycart->Order->Billing->card; $paymentProfile->payment->creditCard->expirationDate = date("my",$Mycart->Order->Billing->cardexpires); $paymentProfile->payment->creditCard->cardCode = $Mycart->Order->Billing->cvv; $customerProfile->paymentProfiles[] = $paymentProfile; $customerProfile->email = $Order->Customer->email; //add a shipping address $address = new AuthorizeNetAddress; $address->firstName = $Mycart->Order->Customer->firstname; $address->lastName = $Mycart->Order->Customer->lasttname; $address->company = "John Doe Company"; $address->address = $Mycart->Order->Shipping->address; $address->city = $Mycart->Order->Shipping->city; $address->state = $Mycart->Order->Shipping->state; $address->zip = $Mycart->Order->Shipping->postcode; $address->country = $Mycart->Order->Shipping->country; $address->phoneNumber = $Mycart->Order->Customer->phone; $response = $request->createCustomerShippingAddress($customerProfileId, $address); $customerAddressId = $response->getCustomerAddressId(); //create the transaction $transaction = new AuthorizeNetTransaction; $transaction->customerProfileId = $customerProfileId; $transaction->customerPaymentProfileId = $paymentProfileId; $transaction->customerShippingAddressId = $customerAddressId; $transaction->amount = $Order->Cart->Totals->total; $lineItem = new AuthorizeNetLineItem; $transaction->lineItems[] = $lineItem; $response = $request->createCustomerProfileTransaction("AuthCapture", $transaction); $transactionResponse = $response->getTransactionResponse(); $transactionId = $transactionResponse->transaction_id; // Line Items $i = 1; foreach($Order->Cart->contents as $Item) { $transaction->lineItems[] = ($i++)."<|>".substr($Item->name,0,31)."<|>".((sizeof($Item->options) > 1)?" (".substr($Item->option->label,0,253).")":"")."<|>".(int)$Item->quantity."<|>".number_format($Item->unitprice,$this->precision,'.','')."<|>".(($Item->tax)?"Y":"N"); } //return $this->encode($_); } function send ($data) { if ($this->settings['testmode'] == "on") $url = $this->testurl; else $url = $this->liveurl; $url = apply_filters('mycart_authorize_net_url',$url); return $this->response(parent::send($data,$url)); } function response ($buffer) { $_ = new stdClass(); list($_->code, $_->subcode, $_->reasoncode, $_->reason, $_->authcode, $_->avs, $_->transactionid, $_->invoicenum, $_->description, $_->amount, $_->method, $_->type, $_->customerid, $_->firstname, $_->lastname, $_->company, $_->address, $_->city, $_->state, $_->zip, $_->country, $_->phone, $_->fax, $_->email, $_->ship_to_first_name, $_->ship_to_last_name, $_->ship_to_company, $_->ship_to_address, $_->ship_to_city, $_->ship_to_state, $_->ship_to_zip, $_->ship_to_country, $_->tax, $_->duty, $_->freight, $_->taxexempt, $_->ponum, $_->md5hash, $_->cvv2code, $_->cvv2response) = explode(",",$buffer); return $_; } function settings () { $this->ui->cardmenu(0,array( 'name' => 'cards', 'selected' => $this->settings['cards'] ),$this->cards); $this->ui->text(1,array( 'name' => 'login', 'value' => $this->settings['login'], 'size' => '16', 'label' => __('Enter your AuthorizeNet Login ID.','Mycart') )); $this->ui->password(1,array( 'name' => 'password', 'value' => $this->settings['password'], 'size' => '24', 'label' => __('Enter your AuthorizeNet Password or Transaction Key.','Mycart') )); $this->ui->checkbox(1,array( 'name' => 'testmode', 'checked' => $this->settings['testmode'], 'label' => __('Enable test mode','Mycart') )); } } // END class AuthorizeNet
-
I was able to install a package called text highlighter. The instructions didn't say anything about installing it inside the command line. I just renamed the Text_highlighter to "Text" and put it inside the root folder of Pear. Only after I went to the command line and typed "pear install text_highlighter-beta" it installed and also worked. My questionis where am I supposed to put the files from a package? In this case I was confused becuase I think thee is a folder in Pear already called text.
-
object(stdClass)#844 (10) { ["mime"]=> string(10) "image/jpeg" ["size"]=> string(5) "23471" ["storage"]=> string(9) "FSStorage" ["uri"]=> string(43) "cache_215_215_1_100_100_16777215_A1-1.jpg" ["filename"]=> string(43) "cache_215_215_1_100_100_16777215_A1-1.jpg" ["width"]=> string(3) "215" ["height"]=> string(3) "215" ["alt"]=> string(0) "" ["title"]=> string(0) "" ["settings"]=> array(6) { ["width"]=> int(215) ["height"]=> int(215) ["scale"]=> int(1) ["sharpen"]=> int(100) ["quality"]=> int(100) ["fill"]=> int(16777215) } } I got it finally. <?php $newvalue = unserialize($Product->ivalue); echo $newvalue->filename; ?>
-
<?php unserialize($Product->ivalue); print_r($Product->ivalue); ?> I get the same string above and the same if I do var_dump();
-
Thanks Christian F. you are always hepfull on here. I have echo $Product->ivalue; and I get this O:8:"stdClass":10:{s:4:"mime";s:10:"image/jpeg";s:4:"size";s:5:"14737";s:7:"storage";s:9:"FSStorage";s:3:"uri";s:27:"cache_276_260__100_A130.jpg";s:8:"filename";s:27:"cache_276_260_100_A130.jpg";s:5:"width";s:3:"258";s:6:"height";s:3:"260";s:3:"alt";s:0:"";s:5:"title";s:0:"";s:8:"settings";a:6:{s:5:"width";i:276;s:6:"height";i:260;s:5:"scale";i:0;s:7:"sharpen";i:100;s:7:"quality";b:0;s:4:"fill";b:0;}
-
I tried this and still no luck. I need just to echo the image name. <?php $newvalue = unserialize($Product->ivalue); echo $newvalue ?>
-
Ok its a serialized object. How can I extract that image name from it?
-
<?php $array = unserialize($Product->ivalue); echo $array[0]; ?> I tried this and it didn't work.